Transforming Legacy Code: The Power of Setter Accessors
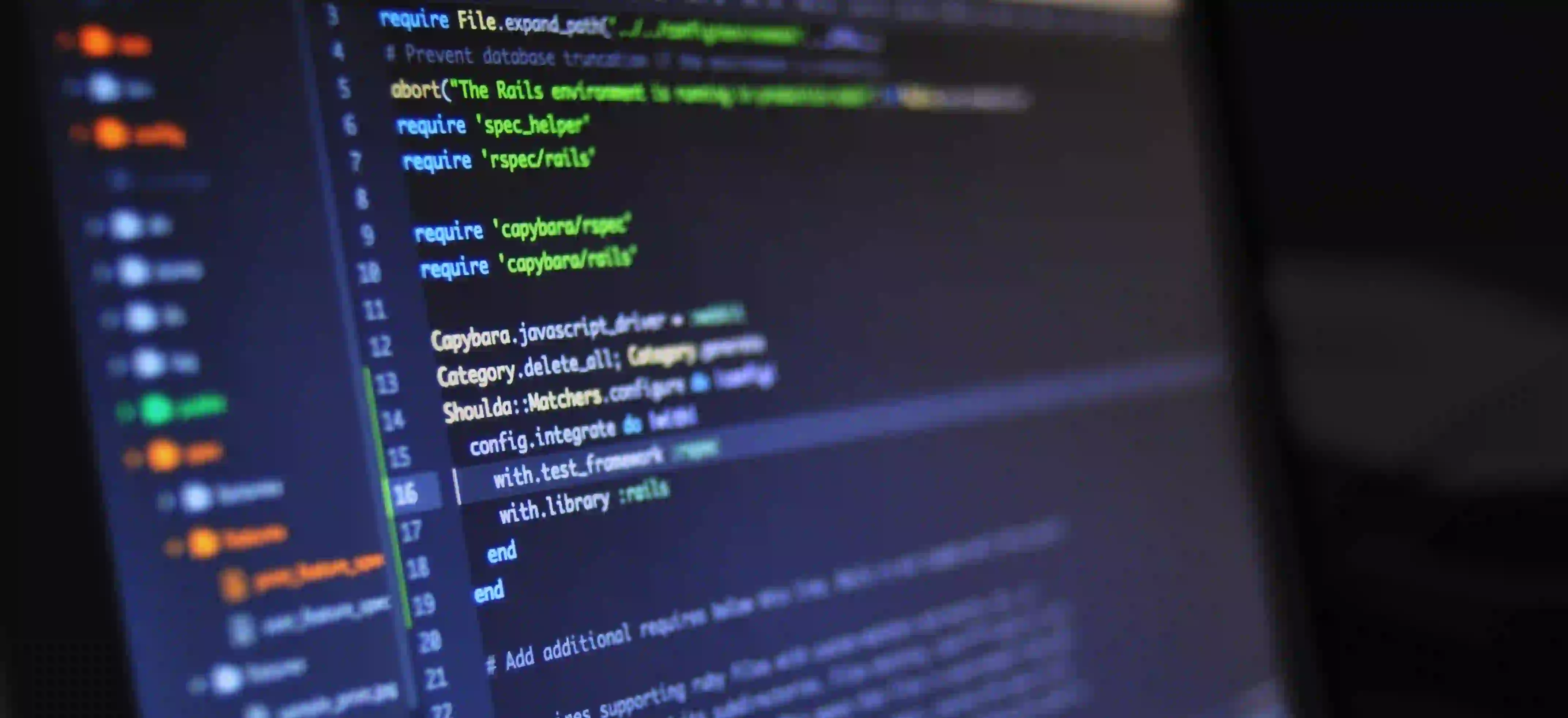
Transforming Legacy Code: The Power of Setter Accessors
In the rapidly evolving world of software development, legacy code can often pose significant challenges. These challenges range from maintaining existing functionalities to adapting to modern development practices. Fortunately, one of the tools developers can use to ease the management and transformation of legacy code is the concept of setter accessors. This blog post explores the importance of setter accessors in Java, illustrates how to implement them effectively, and discusses their advantages.
Understanding Legacy Code
Before diving into setter accessors, let's clarify what legacy code means. Typically, legacy code refers to code that is no longer maintained or updated, often because it was written in outdated languages or practices. Many organizations grapple with systems that were built using past methodologies—systems that are now cumbersome or difficult to adapt to new requirements.
Key Challenges of Legacy Code:
- Lack of Documentation: Older systems often lack proper documentation which makes understanding the architecture and functionality a struggle.
- Tight Coupling: Code written years ago may contain components that are tightly interconnected, making changes risky and difficult.
- Outdated Practices: Legacy code can include outdated coding practices that don't follow modern standards, leading to inefficiency and increased technical debt.
The Role of Setter Accessors
Setter accessors, or setter methods, are a principle of encapsulation in object-oriented programming. They provide a method of modifying the value of a private variable safely and efficiently. When you declare a variable as private, it cannot be accessed directly from outside the class, which preserves the integrity of its data.
Here's a summary of the benefits setter accessors can offer in the context of legacy code:
- Controlled Access: Setter accessors allow you to control how variables are modified. You can enforce rules, validations, or other logic.
- Maintainability: By using setter accessors, you can change the underlying implementation without impacting the classes that use it, thus improving maintainability.
- Comprehensive Debugging: Setters can provide hooks for logging changes, making it easier to debug complex systems.
Example: Implementing Setter Accessors
Let's look at an instance where implementing setter accessors can improve code clarity and maintainability. Assume we have an old class for managing user data.
Legacy Code Excerpt
public class User {
public String name;
public String email;
public User(String name, String email) {
this.name = name;
this.email = email;
}
}
In the code above, both name
and email
are public variables. This approach lacks encapsulation, and any part of the program can modify these variables, leading to potential data integrity issues.
Transforming with Setter Accessors
Let's transform the legacy code to utilize setter accessors:
public class User {
private String name;
private String email;
public User(String name, String email) {
this.setName(name);
this.setEmail(email);
}
public String getName() {
return name;
}
public void setName(String name) {
if (name != null && !name.trim().isEmpty()) {
this.name = name;
} else {
throw new IllegalArgumentException("Name cannot be null or empty");
}
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
if (email != null && email.contains("@")) {
this.email = email;
} else {
throw new IllegalArgumentException("Email must be valid");
}
}
}
Why Use Setter Accessors Here?
- Private Variables: The variables
name
andemail
are now private, adhering to the principle of encapsulation, which hides their internal representation. - Input Validation: The setter methods include basic validation to ensure that inputs follow certain rules. This prevents the internal state from becoming corrupt.
- Clear Data Handling: By using the setter methods during object construction, we are ensuring that only proper values are set right from the start.
Advantages of Setter Accessors
Now that we’ve discussed the transformation, let's delve into the advantages of using setter accessors more thoroughly:
1. Encapsulation
The first and foremost benefit of setter accessors is their emphasis on encapsulation. This principle is a core pillar of object-oriented design. Encapsulation fosters a clear interface for classes, allowing users to understand how to interact with them without revealing the internal workings.
2. Improved Validations
Setter accessors enable you to introduce business logic during object manipulation. This can prevent invalid data from compromising the application's integrity. In our example, we handled null or invalid inputs, thus reducing the risk of runtime errors.
3. Flexibility and Scalability
Suppose you later decide to add logging or transformation when a user's details change. If you have used setter accessors, this can be done easily without altering external code. The original contract (methods exposed) remains intact.
public void setEmail(String email) {
// Log the change
System.out.println("Changing email from " + this.email + " to " + email);
if (email != null && email.contains("@")) {
this.email = email;
} else {
throw new IllegalArgumentException("Email must be valid");
}
}
4. Easier refactoring
Over time, business needs change, and the initial design may become outdated. Using setter methods makes refactoring less painful because you can change how data is stored or validated without affecting other parts of the program relying on your getters and setters.
Wrapping Up
Transitioning legacy code to adopt modern object-oriented practices like setter accessors is a progressive step in ensuring the longevity and maintainability of your software. By encapsulating data and enforcing validation, we can reduce complexity, optimize performance, and enhance clarity in the system.
Additionally, applying these principles allows for a smoother transition when updating legacy systems, aligning them with contemporary development practices. For more in-depth insights on refining legacy systems, you can check out Martin Fowler's article on Refactoring or explore object-oriented design patterns that facilitate cleaner code.
Don't let legacy code hold your development back; embrace setter accessors and make your codebase a better place to work!