Common Pitfalls When Integrating Quartz Scheduler with MySQL
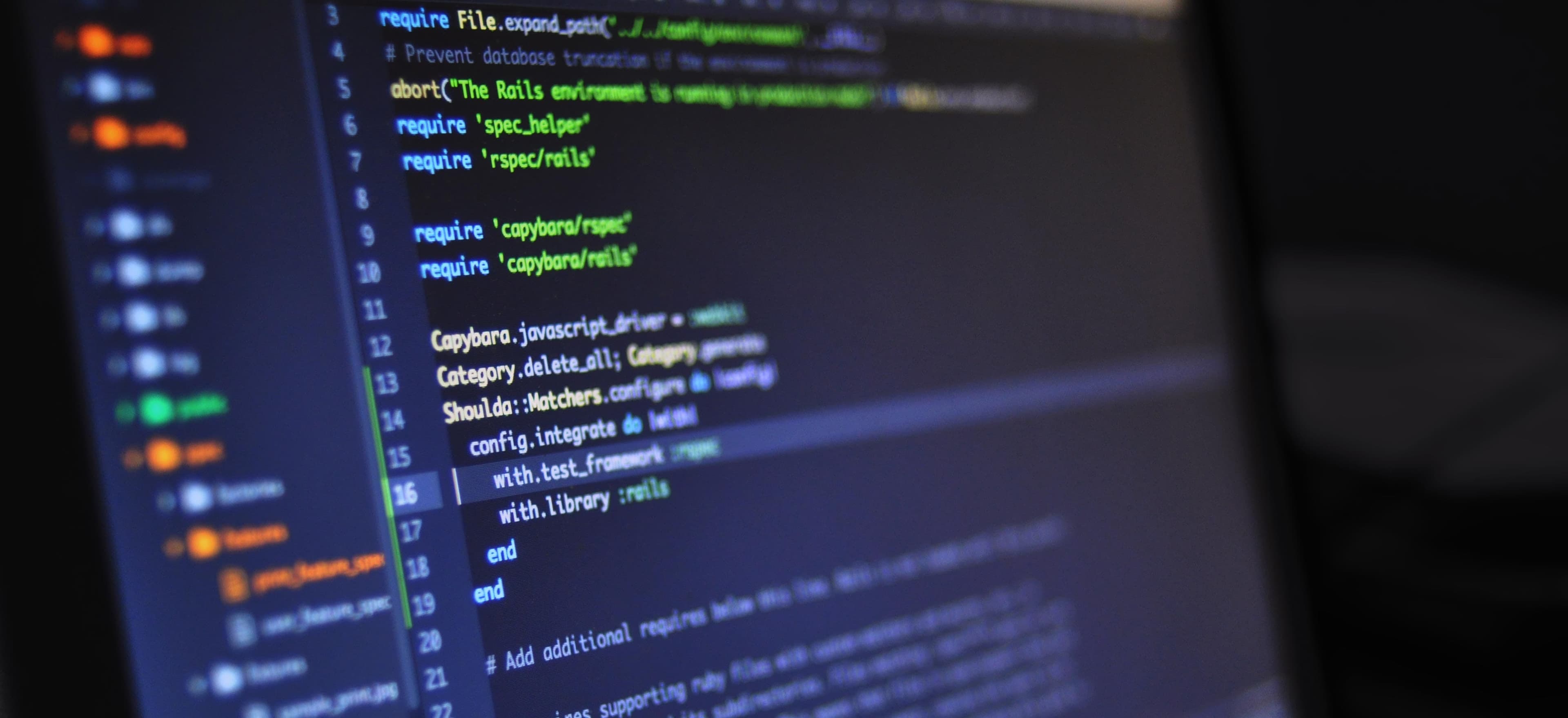
- Published on
Common Pitfalls When Integrating Quartz Scheduler with MySQL
Integrating Quartz Scheduler with MySQL can significantly enhance your application's ability to handle scheduled tasks. However, there are several common pitfalls developers may encounter during this integration. This blog post will explore those pitfalls, provide best practices to avoid them, and include actionable code snippets for clarity. Let's dig into the details!
Understanding Quartz Scheduler and MySQL
Quartz Scheduler is an open-source job scheduling library for Java applications. By leveraging the capabilities of Quartz Scheduler, developers can create and manage complex scheduled jobs, such as sending emails, performing database cleanups, or executing long-running processes at predefined intervals.
Why Choose MySQL?
MySQL is one of the most popular relational database management systems. It is known for its speed, reliability, and ease of use. Using MySQL with Quartz Scheduler allows for persistent job storage, which is crucial for applications that cannot afford the loss of scheduled jobs in case of application crashes or server restarts.
Common Pitfalls
1. Missing Database Configuration
One of the most common pitfalls is failing to configure the datasource correctly in quartz.properties
. Here’s a basic configuration example:
org.quartz.scheduler.instanceName = MyScheduler
org.quartz.scheduler.instanceId = AUTO
# Configure the Quartz Scheduler to use JDBC JobStore
org.quartz.jobStore.class = org.quartz.impl.jdbcjobstore.JobStoreTX
org.quartz.jobStore.driverDelegateClass = org.quartz.impl.jdbcjobstore.StdJDBCDelegate
org.quartz.jobStore.dataSource = myDS
org.quartz.jobStore.tablePrefix = QRTZ_
org.quartz.jobStore.isClustered = true
# Specify the datasource
org.quartz.dataSource.myDS.driver = com.mysql.cj.jdbc.Driver
org.quartz.dataSource.myDS.URL = jdbc:mysql://localhost:3306/quartz_db
org.quartz.dataSource.myDS.user = your_db_user
org.quartz.dataSource.myDS.password = your_db_password
org.quartz.dataSource.myDS.maxConnections = 5
Why It Matters: Incorrect configurations can lead to runtime errors. Ensure the database URL, user credentials, and driver class are correct to avoid connection issues.
2. Incorrect Job Store Table Prefix
By default, Quartz creates a set of tables to store job and trigger data. Ensure that the tablePrefix
in your configuration matches the tables in your database. If you fail to set or match the prefix correctly:
Tables that Quartz creates:
- QRTZ_JOB_DETAILS
- QRTZ_TRIGGERS
- QRTZ_SIMPLE_TRIGGERS
If you have these tables named differently, configure as follows:
org.quartz.jobStore.tablePrefix = CUSTOM_PREFIX_
Why It Matters: Mismatched prefixes will cause Quartz not to find the scheduled jobs and triggers, resulting in errors.
3. Ensuring Database Schema Compatibility
Update your database schema to match the version of Quartz Scheduler you're using. You can find the scripts for creating the necessary tables in the quartz-2.x.x.jar
file. Make sure to insert the correct version.
-- Example SQL to create the Quartz tables:
CREATE TABLE IF NOT EXISTS QRTZ_JOB_DETAILS (
SCHED_NAME VARCHAR(120) NOT NULL,
JOB_NAME VARCHAR(200) NOT NULL,
JOB_GROUP VARCHAR(200) NOT NULL,
DESCRIPTION VARCHAR(250) NULL,
JOB_CLASS_NAME VARCHAR(250) NOT NULL,
IS_DURABLE VARCHAR(1) NOT NULL,
IS_NONCONCURRENT VARCHAR(1) NOT NULL,
IS_UPDATE_DATA VARCHAR(1) NOT NULL,
DATA VARCHAR(4000) NULL,
REQUESTS_RECOVERY VARCHAR(1) NOT NULL,
PRIMARY KEY (SCHED_NAME, JOB_NAME, JOB_GROUP)
);
Why It Matters: If the database schema does not match the expectations of the scheduler, Quartz will fail to operate properly.
4. Job Misconfiguration
Another pitfall is the misconfiguration of jobs themselves. Ensuring your jobs are overloaded correctly is crucial for maintaining operational integrity.
import org.quartz.Job;
import org.quartz.JobExecutionContext;
import org.quartz.JobExecutionException;
public class MyJob implements Job {
@Override
public void execute(JobExecutionContext context) throws JobExecutionException {
System.out.println("Executing MyJob at: " + new java.util.Date());
// perform your job's task here
}
}
Why It Matters: If jobs are not properly configured, they may either not run or execute with errors. Debugging job execution can become complex without clear configuration.
5. Transaction Management
When using MySQL with Quartz, pay attention to transaction management. Using Spring's @Transactional
annotation can help manage transactions effectively.
import org.springframework.transaction.annotation.Transactional;
@Transactional
public void executeJob(MyJob job) {
// Job execution logic
}
Why It Matters: Transaction management is crucial to ensuring your application’s jobs proceed correctly. If jobs fail, not handling transactions may lead to inconsistencies.
6. Handling Time Zones
Quartz Scheduler uses UTC by default. Failure to account for time zone differences can result in undesired job execution times.
Use the following setting in quartz.properties
:
org.quartz.scheduler.timeZone = UTC
Or, in code:
import org.quartz.TriggerBuilder;
import org.quartz.SimpleScheduleBuilder;
TriggerBuilder<SimpleTrigger> triggerBuilder = TriggerBuilder.newTrigger()
.withIdentity("myTrigger", "group1")
.startAt(DateBuilder.dateOf(15, 10, 10, 1, 1, 2023))
.withSchedule(SimpleScheduleBuilder.simpleSchedule()
.withIntervalInMinutes(2)
.repeatForever());
Why It Matters: Incorrect time zone settings can result in missed jobs or jobs executing at unexpected times.
7. Clustering Configuration
If you're deploying in a clustered environment, ensure that routing and clustering are configured for Quartz Scheduler. For example, set isClustered
to true:
org.quartz.jobStore.isClustered = true
Proper clustering ensures job execution across multiple nodes without duplication.
Why It Matters: Failure to configure clustering correctly could result in jobs running multiple times across different nodes, leading to undesirable performance impacts.
The Last Word
Integrating Quartz Scheduler with MySQL offers powerful scheduling capabilities. However, several common pitfalls arise during this integration. Be vigilant in addressing database configurations, job management, transaction handling, and time zones. By understanding these challenges and implementing best practices, you’ll enhance the stability and reliability of your application.
For more insights on using Quartz Scheduler, check out the Quartz Scheduler Documentation. If you want to dive deeper into Spring and Quartz integration, the Spring Framework Documentation is a great resource.
Happy coding!
Checkout our other articles