Avoiding Deadlocks in Java's Explicit Locking Mechanism
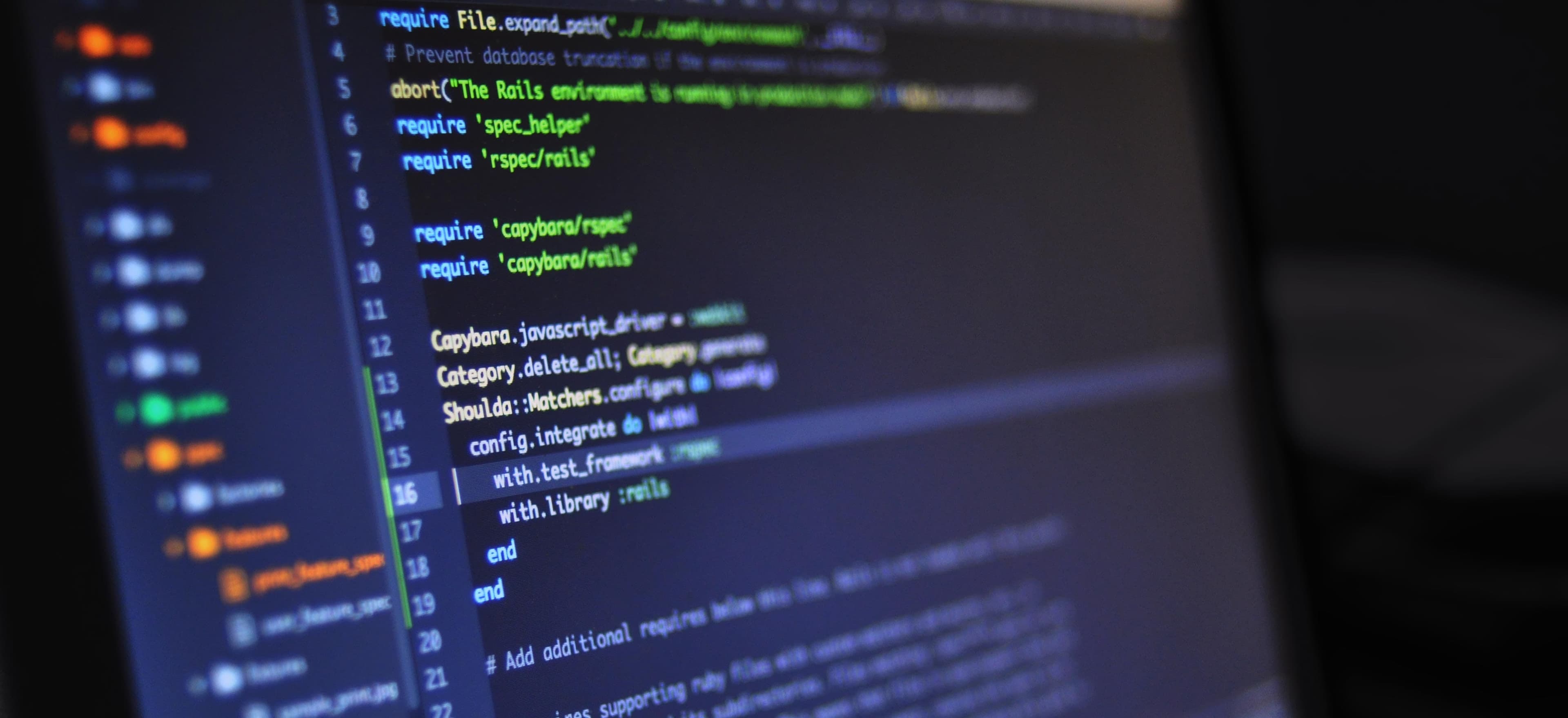
- Published on
Avoiding Deadlocks in Java's Explicit Locking Mechanism
Deadlocks are a common problem when dealing with concurrent programming. In Java, the explicit locking mechanism provides powerful features, but it also introduces the risk of deadlocks if not managed carefully. This blog post will explore the concept of deadlocks, how they can occur with Java's explicit locking, and best practices for avoiding them.
Understanding Deadlocks
A deadlock occurs when two or more threads are waiting for each other to release locks, leading to a situation where none of the threads can proceed. The classic example involves two threads, each holding a lock that the other thread needs.
Here's a simple illustration:
- Thread A holds Lock 1 and needs Lock 2 to proceed.
- Thread B holds Lock 2 and needs Lock 1 to continue.
Neither thread can proceed, resulting in a deadlock.
How Java's Explicit Locking Works
Java provides the java.util.concurrent.locks
package, which offers explicit locking via the Lock
interface. Unlike synchronized blocks, which rely on intrinsic locks, explicit locks provide a higher degree of flexibility in concurrency control.
Here's a quick example of how to use explicit locking:
import java.util.concurrent.locks.Lock;
import java.util.concurrent.locks.ReentrantLock;
public class Counter {
private int count = 0;
private final Lock lock = new ReentrantLock();
public void increment() {
lock.lock(); // Acquire the lock
try {
count++;
} finally {
lock.unlock(); // Always release the lock
}
}
public int getCount() {
return count;
}
}
Why Deadlocks Occur with Explicit Locking
Deadlocks can occur with explicit locks due to the way multiple locks are acquired. If two threads acquire locks in a different order, deadlocking becomes a possibility:
- Thread 1 acquires Lock A.
- Thread 2 acquires Lock B.
- Thread 1 tries to acquire Lock B, while Thread 2 tries to acquire Lock A.
To visualize this, consider the following scenario:
public class DeadlockExample {
private final Lock lock1 = new ReentrantLock();
private final Lock lock2 = new ReentrantLock();
public void methodA() {
lock1.lock();
try {
Thread.sleep(50); // Simulating some work
methodB(); // Calling another method
} catch (InterruptedException e) {
e.printStackTrace();
} finally {
lock1.unlock();
}
}
public void methodB() {
lock2.lock();
try {
// Some logic for method B
} finally {
lock2.unlock();
}
}
}
public class DeadlockDemo {
public static void main(String[] args) {
DeadlockExample example = new DeadlockExample();
Thread thread1 = new Thread(example::methodA);
Thread thread2 = new Thread(example::methodB);
thread1.start();
thread2.start();
}
}
In this example, if thread1
locks lock1
and tries to enter methodB
, while thread2
has already locked lock2
, then both threads will be unable to proceed, thus causing a deadlock.
Best Practices for Avoiding Deadlocks
Understanding how deadlocks can arise is crucial. However, awareness alone is insufficient to prevent them. Below are some best practices that can significantly reduce the risk of deadlocks in your Java applications:
1. Lock Ordering
Enforce a global order in the acquisition of locks. This means that all threads must acquire locks in the same order. By doing so, you eliminate the scenario where two threads might deadlock each other.
public class OrderedLockingExample {
private final Lock lock1 = new ReentrantLock();
private final Lock lock2 = new ReentrantLock();
public void methodA() {
lock1.lock();
try {
lock2.lock();
try {
// Critical section
} finally {
lock2.unlock();
}
} finally {
lock1.unlock();
}
}
public void methodB() {
lock1.lock();
try {
lock2.lock();
try {
// Critical section
} finally {
lock2.unlock();
}
} finally {
lock1.unlock();
}
}
}
2. Try-Lock with Timeouts
Instead of using lock()
which can potentially lead to deadlocks, use the tryLock(long timeout, TimeUnit unit)
method. This will attempt to acquire the lock and will back off if it fails to acquire the lock within the specified time.
import java.util.concurrent.TimeUnit;
public void safeMethod() {
boolean acquired = false;
try {
acquired = lock1.tryLock(100, TimeUnit.MILLISECONDS);
if (acquired) {
// Proceed with locked logic
} else {
// Handle inability to acquire lock
}
} catch (InterruptedException e) {
Thread.currentThread().interrupt(); // Restore the interrupted status
} finally {
if (acquired) {
lock1.unlock();
}
}
}
3. Avoid Nested Locks
If possible, avoid locking within a lock. If a method that acquires a lock can call another method that also acquires a lock, you increase the risk of deadlocks. You can restructure your code to do as much work as possible before acquiring the locks.
4. Lock Timeout Strategies
Implementing a timeout strategy where threads only block for a limited amount of time before giving up on acquiring a lock can help mitigate deadlocks.
5. Utilize Higher-Level Concurrency Utilities
Consider using higher-level concurrency constructs provided by the java.util.concurrent
package, such as Semaphore
or CountDownLatch
, depending on your scenario. These are designed to manage common concurrency issues and can potentially avoid intricate locking situations.
My Closing Thoughts on the Matter
Deadlocks can be a significant issue in concurrent programming, especially in Java with its explicit locking mechanism. By understanding how deadlocks occur, using best practices to enforce lock ordering, utilizing timeouts, and making use of higher-level concurrency utilities, you can significantly reduce the chances of deadlock in your applications.
For more information on Java concurrency, consider checking out Java Concurrency in Practice. You can also refer to the Oracle Java Documentation for deeper insights into the java.util.concurrent
package.
Happy coding!
Checkout our other articles