Common Maven Mistakes Newbies Make in Java EE 7 Projects
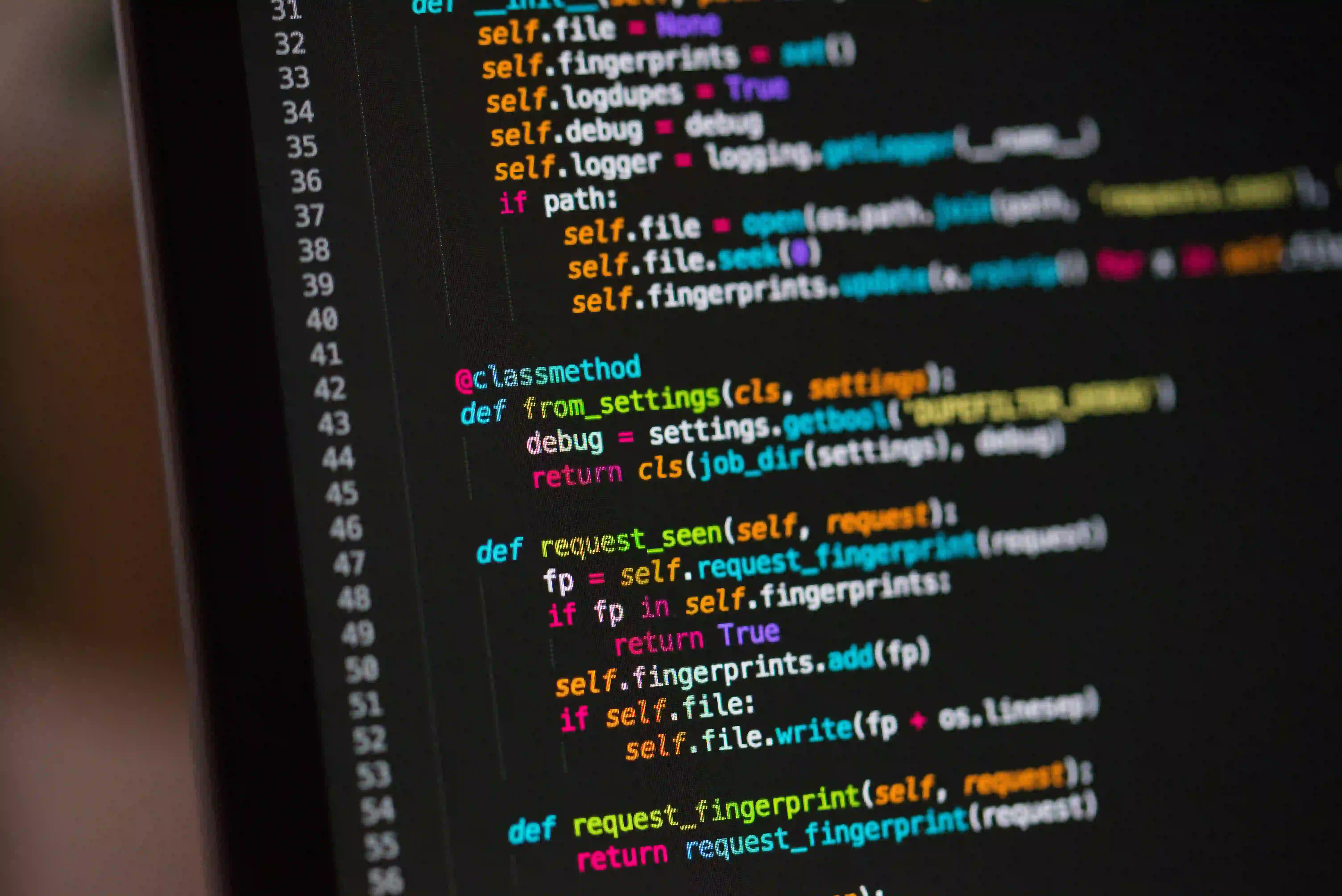
Common Maven Mistakes Newbies Make in Java EE 7 Projects
Maven is a popular build automation tool used primarily for Java projects. However, as with any powerful tool, beginners frequently make mistakes that can hinder productivity and cause frustration. In this blog post, we will discuss common mistakes that newcomers make while using Maven in Java EE 7 projects and offer guidance on how to avoid them. Understanding these pitfalls can help you build efficient, maintainable applications faster.
Table of Contents
- Misunderstanding the POM File
- Ignoring Dependency Management
- Incorrect Version Management
- Improper Lifecycle Management
- Not Using Profiles
- Forgetting to Use the Right Plugin
- Overcomplicating the Build Process
- Conclusion
Understanding the Basics of Maven
Before diving into the common mistakes, it’s valuable to understand Maven's core concept: the POM file (Project Object Model). This file serves as the main configuration file for a Maven project and dictates how your project is built, its dependencies, and various plugin configurations.
Misunderstanding the POM File
Mistake 1: Not Structuring the POM Correctly
Maven relies heavily on the POM.xml file, the backbone of the project. A well-structured POM can save time and prevent errors.
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.example</groupId>
<artifactId>my-java-ee7-project</artifactId>
<version>0.1-SNAPSHOT</version>
<packaging>war</packaging>
<dependencies>
<!-- Add your dependencies here -->
</dependencies>
<build>
<plugins>
<!-- Add plugins here -->
</plugins>
</build>
</project>
Why It Matters
Proper structure is crucial because it dictates how your project is managed. Missing or misplaced tags will lead to build failures, making your development process inefficient. For a deeper understanding of POM file structure, check out the official Maven Documentation.
Ignoring Dependency Management
Mistake 2: Manual Dependency Resolution
New developers often forget that Maven can handle dependencies automatically. Placing all dependencies in the POM without understanding transitive dependencies can lead to version conflicts.
<dependencies>
<dependency>
<groupId>javax</groupId>
<artifactId>javaee-api</artifactId>
<version>7.0</version>
<scope>provided</scope>
</dependency>
</dependencies>
Why It Matters
By utilizing Maven’s dependency management capabilities, you can avoid manually locating and downloading JAR files, saving time and ensuring consistency across your project.
Incorrect Version Management
Mistake 3: Hardcoding Dependency Versions
While it may seem convenient to hardcode versions, this practice can lead to difficulties when updating or changing versions.
<dependency>
<groupId>org.hibernate</groupId>
<artifactId>hibernate-core</artifactId>
<version>5.4.2.Final</version> <!-- Hardcoded version -->
</dependency>
Why It Matters
Hardcoding versions can result in challenges when you later want to upgrade. Instead, utilize properties:
<properties>
<hibernate.version>5.4.2.Final</hibernate.version>
</properties>
<dependency>
<groupId>org.hibernate</groupId>
<artifactId>hibernate-core</artifactId>
<version>${hibernate.version}</version>
</dependency>
Using properties helps maintain a single point of control over your versioning system.
Improper Lifecycle Management
Mistake 4: Lack of Understanding of Build Lifecycle
Maven has a four-phase build lifecycle which includes validate, compile, test, and package, among others. Beginners often skip phases or do not fully understand their significance.
Why It Matters
Understanding Maven’s lifecycle ensures that all tasks are run in the correct order, making builds more efficient and reducing errors. Not aligning tasks within the lifecycle can lead to runtime issues as certain tasks may not execute as expected.
Not Using Profiles
Mistake 5: Overlooking the Use of Profiles
Profiles allow you to customize builds for different environments (e.g., development, testing, production). Not using profiles means you might end up using the same configuration across different environments, leading to potential issues.
<profiles>
<profile>
<id>dev</id>
<properties>
<env>development</env>
</properties>
</profile>
<profile>
<id>prod</id>
<properties>
<env>production</env>
</properties>
</profile>
</profiles>
Why It Matters
Using profiles streamlines your build process for various environments and prevents configuration errors. For comprehensive usage examples, refer to the Maven Profiles Guide.
Forgetting to Use the Right Plugin
Mistake 6: Not Recognizing Relevant Plugins
Maven plugins are tools that enhance the capabilities of Maven, specifically for your project’s needs. Newbies often overlook the necessity of certain plugins.
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-compiler-plugin</artifactId>
<version>3.8.1</version>
<configuration>
<source>1.8</source>
<target>1.8</target>
</configuration>
</plugin>
</plugins>
</build>
Why It Matters
Using the correct plugins will help tailor the build process, making it more efficient and suitable for your specific requirements. Always research needed plugins based on your project specifications.
Overcomplicating the Build Process
Mistake 7: Adding Unnecessary Complexity
Beginners sometimes add overly complex configurations, leading to confusing builds. Keep it simple.
Why It Matters
A clean, straightforward configuration is easier to maintain and reduces the likelihood of errors. As a rule of thumb, add complexity only when needed. Refer to the Maven Best Practices Guide for tips on keeping your POM clean.
A Final Look
Maven is an essential tool for Java EE 7 projects, but making these common mistakes can slow down your development process. Understanding the POM file's structure, managing dependencies, utilizing profiles, and recognizing the importance of plugins are crucial steps for an effective build environment.
By avoiding these pitfalls, newcomers can enhance productivity and focus more on development rather than troubleshooting build issues. For more detailed explanations on specific Maven topics, feel free to visit the Maven Official Site.
Happy coding, and may your Java EE projects build smoothly!