Mastering Maven: Common Management Pitfalls to Avoid
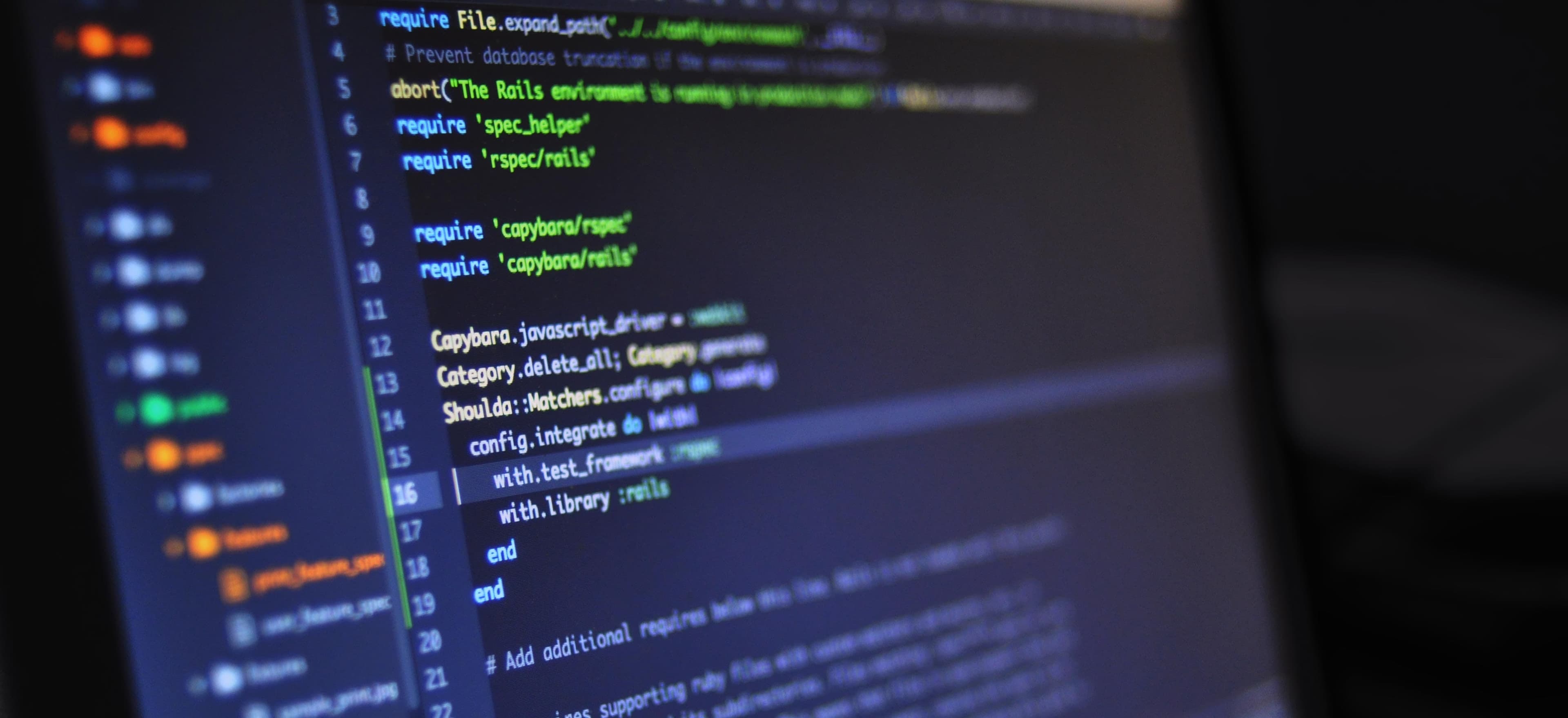
- Published on
Mastering Maven: Common Management Pitfalls to Avoid
Maven is an essential tool for many Java developers, serving as a project management and comprehension tool. Despite its benefits, developers often stumble upon common management pitfalls that can derail their projects. In this blog post, we’ll explore these potential traps, provide solutions, and how you can streamline your Java projects using Maven.
Understanding Maven
Before diving into pitfalls, let’s quickly review what Maven does. At its core, Maven simplifies the build process for Java projects. It manages dependencies, facilitates project structure, and enables easy integration with other tools.
In a typical Maven project, you’ll find a pom.xml
(Project Object Model) file, which is the cornerstone of Maven's project management. In this XML file, you define all project configurations, dependencies, plugins, and other crucial information.
Example of a pom.xml
File
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.example</groupId>
<artifactId>my-app</artifactId>
<version>1.0-SNAPSHOT</version>
<dependencies>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-core</artifactId>
<version>5.3.8</version>
</dependency>
</dependencies>
</project>
In the above example, you define a simple Maven project that depends on the Spring framework. The pom.xml
acts as a central point for dependency management and other configurations.
Common Maven Pitfalls to Avoid
1. Ignoring Dependency Management
One of the most frequent mistakes developers make is overlooking dependency management. Failing to manage your dependencies effectively can lead to “dependency hell,” where conflicting versions of libraries create runtime issues.
Solution:
Always specify versions explicitly in your pom.xml
. You can also use dependency management techniques to control versions across different modules.
For instance, if you're using multiple modules within your project, you can define dependencies in the parent POM and reference them in child POMs:
<dependencyManagement>
<dependencies>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-core</artifactId>
<version>5.3.8</version>
</dependency>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.13.2</version>
</dependency>
</dependencies>
</dependencyManagement>
This method ensures every module uses the specified version, thus mitigating conflicts.
2. Not Cleaning Up Old Dependencies
Maven allows for easy management of dependencies, but many developers forget to clean up unused dependencies. Over time, as projects evolve, old dependencies linger, potentially introducing security vulnerabilities and making the project unnecessarily heavy.
Solution:
Regularly review and update your dependencies. Use the mvn dependency:analyze
command to find any unused dependencies.
3. Incorrectly Configuring the Build Lifecycle
Maven's build lifecycle consists of several phases, including validate, compile, test, package, and deploy. Misconfiguring these phases can lead to incomplete builds or missing artifacts.
Solution:
Understand and utilize Maven's lifecycle features. Use the maven-clean-plugin
to clean your project before every build, ensuring a clean slate.
Example of the use in the pom.xml
:
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-clean-plugin</artifactId>
<version>3.1.0</version>
<executions>
<execution>
<id>default-clean</id>
<phase>pre-clean</phase>
<goals>
<goal>clean</goal>
</goals>
</execution>
</executions>
</plugin>
</plugins>
</build>
4. Not Leveraging Profiles
Maven profiles allow you to customize builds for different environments (e.g., development, testing, production). Many developers neglect this feature and end up with configurations hardwired into their pom.xml
.
Solution:
Define profiles for different environments, making it easier to switch configurations based on your needs:
<profiles>
<profile>
<id>dev</id>
<properties>
<env>development</env>
</properties>
</profile>
<profile>
<id>prod</id>
<properties>
<env>production</env>
</properties>
</profile>
</profiles>
To activate a profile, you can use the command:
mvn clean install -Pdev
5. Overusing Plugin Defaults
Maven plugins provide additional functionality, but developers often overlook configuring their defaults, leading to unoptimized builds and missed features.
Solution:
Review and customize plugin configurations to leverage their full potential. For example, the Surefire Plugin for unit testing can be tailored to output test reports.
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-surefire-plugin</artifactId>
<version>2.22.2</version>
<configuration>
<includes>
<include>**/*Test.java</include>
</includes>
<properties>
<property>
<name>listener</name>
<value>org.apache.maven.surefire.report.SimpleReportFormatter</value>
</property>
</properties>
</configuration>
</plugin>
</plugins>
</build>
6. Neglecting Documentation and Comments
As your project grows, maintaining good documentation becomes crucial. However, many developers skimp on documentation, which can make it challenging for team members (or even yourself) to understand the project later.
Solution:
Comment your pom.xml
and add a README.md file explaining the project structure, key dependencies, and build instructions. Good documentation fosters collaboration and streamlines onboarding.
7. Failing to Manage Local Repository
Maven caches your downloaded artifacts in the local repository. Over time, this repository can become cluttered and inefficient, slowing down build processes.
Solution:
Periodically clean your local .m2
repository. You can remove unused libraries manually or use commands to automatically manage it. For example, you can delete specific versions that are no longer needed.
Closing Remarks
Maven is a powerful asset for Java developers when used correctly. By being aware of the common pitfalls outlined in this post, you can manage your Maven projects more effectively and avoid common issues that can arise throughout the development process.
To learn more about Maven, visit the Maven Official Documentation and Baeldung on Maven.
By understanding and implementing best practices in your Maven projects, you can ensure a smoother and more reliable development experience. Happy building!