Debugging Java: Tackling Timeouts Effectively
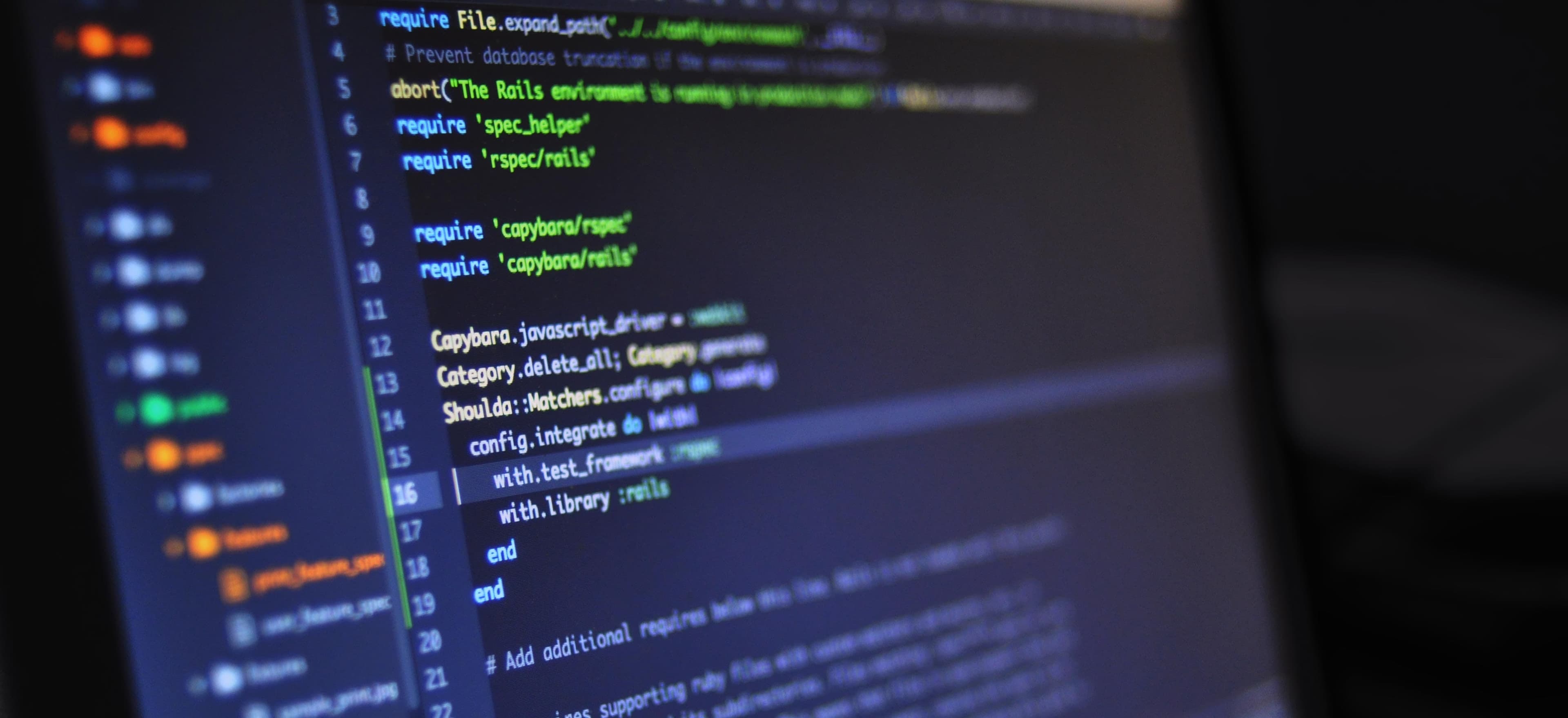
- Published on
Debugging Java: Tackling Timeouts Effectively
Timeouts are a common issue that can plague Java applications, especially when interacting with network resources, databases, or asynchronous operations. Whether you're developing a microservice, a web application, or any distributed system, understanding and addressing timeouts is vital to ensuring a smooth user experience. In this blog post, we'll dive deep into how to effectively debug Java applications facing timeout issues. We will discuss the reasons leading to timeouts, share best practices in exception handling, explore practical debugging techniques, and illustrate everything with code snippets.
What Causes Timeouts?
Timeouts can occur due to numerous reasons, including:
- Slow Network Conditions: Insufficient bandwidth or high latency can delay responses from external services.
- Database Performance: Long-running queries can lead to connection timeouts.
- External Service Failures: Availability issues with third-party APIs can contribute to waiting times.
- Server Load: High loads on your application server can lead to delayed response times, causing timeouts.
Understanding these causes is the first step toward implementing effective debugging strategies.
Setting Up a Timeout
Before diving deeper, let's see how you can set a timeout in a typical Java application. This example demonstrates working with an HttpURLConnection
:
import java.io.IOException;
import java.net.HttpURLConnection;
import java.net.URL;
public class HttpClient {
public void connect(String urlString) {
HttpURLConnection connection = null;
try {
URL url = new URL(urlString);
connection = (HttpURLConnection) url.openConnection();
connection.setConnectTimeout(5000); // Set connection timeout to 5 seconds
connection.setReadTimeout(5000); // Set read timeout to 5 seconds
connection.connect();
System.out.println("Connected successfully.");
} catch (IOException e) {
System.out.println("Connection timed out: " + e.getMessage());
} finally {
if (connection != null) {
connection.disconnect();
}
}
}
}
Code Explanation
-
setConnectTimeout(5000): This method sets the maximum time to wait while establishing a connection. If the timeout expires before the connection is established, a
SocketTimeoutException
is thrown. -
setReadTimeout(5000): This method sets the maximum time to wait for data to be available for reading. Similarly, this results in a
SocketTimeoutException
.
In this context, we ensure that we catch any IOExceptions
thrown due to timeouts, allowing us to debug further.
Debugging Timeouts
When dealing with timeout issues, follow a systematic approach to isolate the problem:
1. Monitor Logs
Implement comprehensive logging in your application. Logging the total time taken for requests, along with timestamps, can help you identify problematic patterns.
import java.io.IOException;
import java.net.HttpURLConnection;
import java.net.URL;
public class HttpClient {
public void connect(String urlString) {
long startTime = System.currentTimeMillis();
HttpURLConnection connection = null;
try {
URL url = new URL(urlString);
connection = (HttpURLConnection) url.openConnection();
connection.setConnectTimeout(5000);
connection.setReadTimeout(5000);
connection.connect();
long endTime = System.currentTimeMillis();
System.out.println("Connected successfully in " + (endTime - startTime) + " ms.");
} catch (IOException e) {
long endTime = System.currentTimeMillis();
System.out.println("Connection failed after " + (endTime - startTime) + " ms: " + e.getMessage());
} finally {
if (connection != null) {
connection.disconnect();
}
}
}
}
2. Network Debugging Tools
Use tools like curl
or Postman to test the endpoints manually to check if the timeout persists outside your Java application. If the endpoint is consistently slow or failing, the issue lies in the external service, not your code.
3. Analyze Thread Dumps
When running in a production environment, take thread dumps when the application is experiencing timeouts. Tools like VisualVM can help visualize the state of your Java application. Look for:
- Threads waiting on I/O operations.
- Threads that are blocked due to not being able to access synchronized resources.
4. Performance Profiling
Running a profiler on your Java application can help identify bottlenecks. Tools such as YourKit or JProfiler can expose which methods take the longest and it allows you to focus your debugging efforts.
5. Timeout Settings Review
Review your timeout settings. Ensure they are appropriate for your application context. Sometimes, simply increasing the timeout limit can be a valid quick fix.
Exception Management
Effective handling of SocketTimeoutException
and related exceptions is crucial when tackling timeout issues. Ideally, your application should not crash due to these exceptions. Instead, a graceful recovery mechanism can enhance user experience.
Retry Mechanism
Implementing a retry mechanism can help when a request times out due to transient issues.
public void connectWithRetry(String urlString, int retries) {
for (int attempt = 0; attempt < retries; attempt++) {
long startTime = System.currentTimeMillis();
HttpURLConnection connection = null;
try {
URL url = new URL(urlString);
connection = (HttpURLConnection) url.openConnection();
connection.setConnectTimeout(5000);
connection.setReadTimeout(5000);
connection.connect();
long endTime = System.currentTimeMillis();
System.out.println("Connected successfully in " + (endTime - startTime) + " ms.");
return; // Successful connection, exit the method
} catch (SocketTimeoutException e) {
System.out.println("Retry #" + (attempt + 1) + " failed: " + e.getMessage());
} catch (IOException e) {
System.out.println("Connection failed: " + e.getMessage());
} finally {
if (connection != null) {
connection.disconnect();
}
}
// Exponential backoff
try {
Thread.sleep((long) Math.pow(2, attempt) * 1000);
} catch (InterruptedException ie) {
Thread.currentThread().interrupt();
}
}
System.out.println("All retries failed for URL: " + urlString);
}
Code Explanation
-
Retry Loop: The
for
loop allows us to attempt connecting multiple times. -
Exponential Backoff: The
Thread.sleep()
method introduces increasing delays between retries, making it less likely that we face the same timeout again immediately.
Implementing a resilience pattern like this ensures that your application remains robust even when interacting with unreliable services.
The Bottom Line
Debugging Java applications with timeout issues requires a multi-faceted approach. From logging to performance profiling, utilizing the right tools can dramatically simplify the process. Always ensure that you have a solid strategy for handling exceptions for an enhanced user experience.
For additional information, you can explore resources like Java Network Programming or Effective Java for deeper insights into best practices that can help with debugging and overall application design.
By addressing timeout issues proactively and employing effective debugging techniques, your Java applications will be far more robust, responsive, and user-friendly. Happy coding!
Checkout our other articles