Unlocking Java: Optimize Memory Usage with Smart GC Strategies
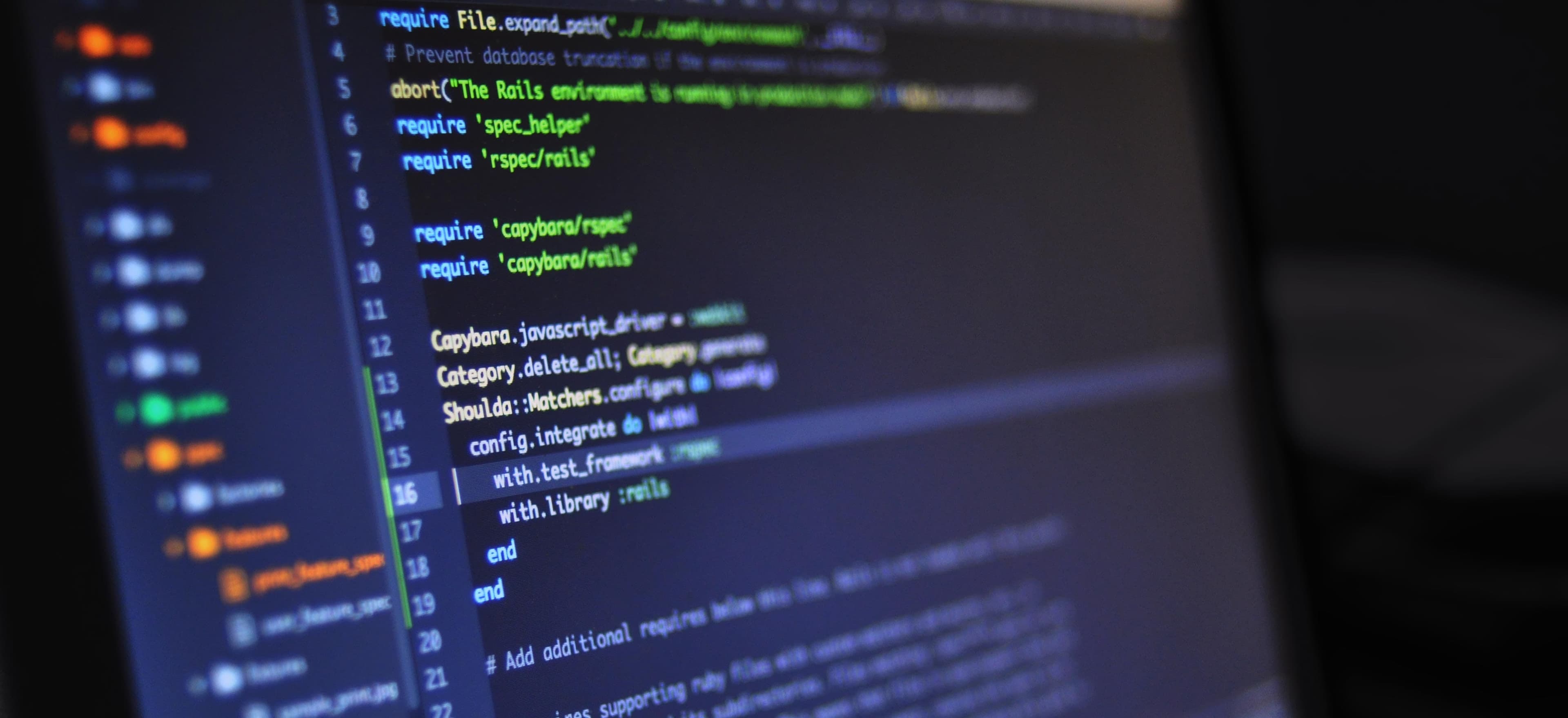
- Published on
Unlocking Java: Optimize Memory Usage with Smart GC Strategies
Java is one of the most widely-used programming languages in the world today, known for its efficiency, portability, and robustness. However, with great power comes great responsibility—specifically, the need to manage memory effectively. Any experienced Java developer knows that memory management can make or break the performance of an application. One of the unsung heroes of memory management in Java is the Garbage Collector (GC). In this blog post, we will explore how you can optimize memory usage in Java through smart GC strategies.
Understanding the Java Garbage Collector
What is Garbage Collection?
Garbage Collection (GC) is an automatic memory management process that helps in reclaiming memory from objects that are no longer in use. In Java, this process runs in the background, freeing developers from the manual memory management burden found in languages like C or C++. However, the GC can also impact application performance if not properly configured—this is where smart strategies come into play.
The Role of Garbage Collectors
Java provides several types of Garbage Collectors, each tailored to different use cases. You can choose a Garbage Collector based on:
- Application size (small vs. large)
- Throughput requirements
- Pause time requirements
The most commonly used GC techniques include:
- Serial GC
- Parallel GC
- Concurrent Mark-Sweep (CMS) GC
- G1 (Garbage-First) GC
- ZGC and Shenandoah
Understanding how these collectors work will enable you to make informed judgments about their configurations.
Popular Garbage Collectors: A Deeper Dive
Serial Garbage Collector
The Serial GC is straightforward—it uses a single thread for garbage collection. It is best suited for small applications with little memory footprint. While it minimizes overhead, its inability to handle larger datasets limits its usability in more extensive systems.
Code Example: Setting Serial GC in Java
java -XX:+UseSerialGC -jar YourApplication.jar
Parallel Garbage Collector
As the name suggests, the Parallel GC employs multiple threads for performing garbage collection, ideally maximizing throughput. However, it can yield longer pause times, making it less appropriate for applications requiring low latency.
Code Example: Enabling Parallel GC in Java
java -XX:+UseParallelGC -jar YourApplication.jar
Concurrent Mark-Sweep (CMS) Collector
The CMS Collector aims to minimize pause times by performing most of its work concurrently with the application threads. It’s a popular choice for applications sensitive to latency.
Code Example: Configuring CMS in Java
java -XX:+UseConcMarkSweepGC -jar YourApplication.jar
G1 Garbage Collector
G1 GC is designed for large heap applications, partitioning the heap into regions instead of traditional generations. It combines the benefits of both throughput and low pause times.
Code Example: Using G1 GC in Java
java -XX:+UseG1GC -jar YourApplication.jar
ZGC and Shenandoah
Introduced in Java 11 and 12 respectively, these collectors are designed for low-latency applications. They are suitable for applications requiring a very high level of responsiveness, even with enormous heap sizes.
Code Example: Activating ZGC
java -XX:+UseZGC -jar YourApplication.jar
Key GC Tuning Strategies
While selecting the appropriate garbage collector is essential, tweaking GC parameters can further enhance performance.
1. Heap Size Configuration
One of the most efficient ways to boost garbage collection performance is to adjust the heap size. Start by setting the initial and maximum heap size according to your application needs.
Code Example: Configuring Heap Size
java -Xms512m -Xmx4g -jar YourApplication.jar
2. Set the New Generation Size
The size of the new generation can significantly influence your application performance. Allocating sufficient space helps reduce stop-the-world events.
Code Example: Configuring New Generation Size
java -Xmn256m -jar YourApplication.jar
3. GC Logging
Enabling GC logging will help you collect data during execution, allowing you to analyze the garbage collection behavior of your application.
Code Example: Enabling GC Logging
java -Xlog:gc*:file=gc.log -jar YourApplication.jar
4. Adjusting Young and Old Generation Ratios
Tuning the ratio of Young to Old generations can also improve performance. You can specify this using -XX:SurvivorRatio
.
Code Example: Setting Survivor Ratio
java -XX:SurvivorRatio=4 -jar YourApplication.jar
Performance Monitoring Tools
1. VisualVM
VisualVM is a powerful monitoring tool that comes with JDK and allows you to visualize heap and garbage collection activity. It is an excellent tool for profiling your applications and understanding memory usage patterns.
2. Java Mission Control (JMC)
Java Mission Control is an advanced tool that allows profiling and diagnostics of Java applications at runtime. It is particularly useful for analyzing GC performance.
Best Practices for Memory Management
-
Use the Right GC for Your Use Case: Always determine the best GC based on your application’s specific needs.
-
Profile First, Tune Later: Use monitoring tools to gather data before making aggressive changes.
-
Understand Object Lifetimes: Design your applications to create short-lived objects to take advantage of the Young generation efficiently.
-
Beware of Memory Leaks: Keep an eye on memory usage over time to catch any potential leaks.
-
Avoid Unnecessary Object Creation: Reuse objects whenever possible. This reduces the load on your garbage collector.
-
Keep Collections Lean: Use collections that fit your data needs. For example, prefer ArrayList over LinkedList if you are frequently accessing elements.
To Wrap Things Up
Mastering memory management in Java via Garbage Collection is a complex yet rewarding endeavor. By selecting the right collector, tuning parameters, and employing best practices, you can significantly enhance your application's performance and responsiveness. Remember that there's no one-size-fits-all solution; analyze your requirements and adapt strategies accordingly.
In a world where applications must perform optimally to maintain user satisfaction, the strategies discussed here could be the keys to unlocking better memory efficiency in Java.
For further reading on Java's memory management, check the official Java documentation on Garbage Collection.
Feel free to follow this blog for more deep dives into Java technology and programming best practices!
Checkout our other articles