How to Resolve Arquillian Configuration Issues in Java 8
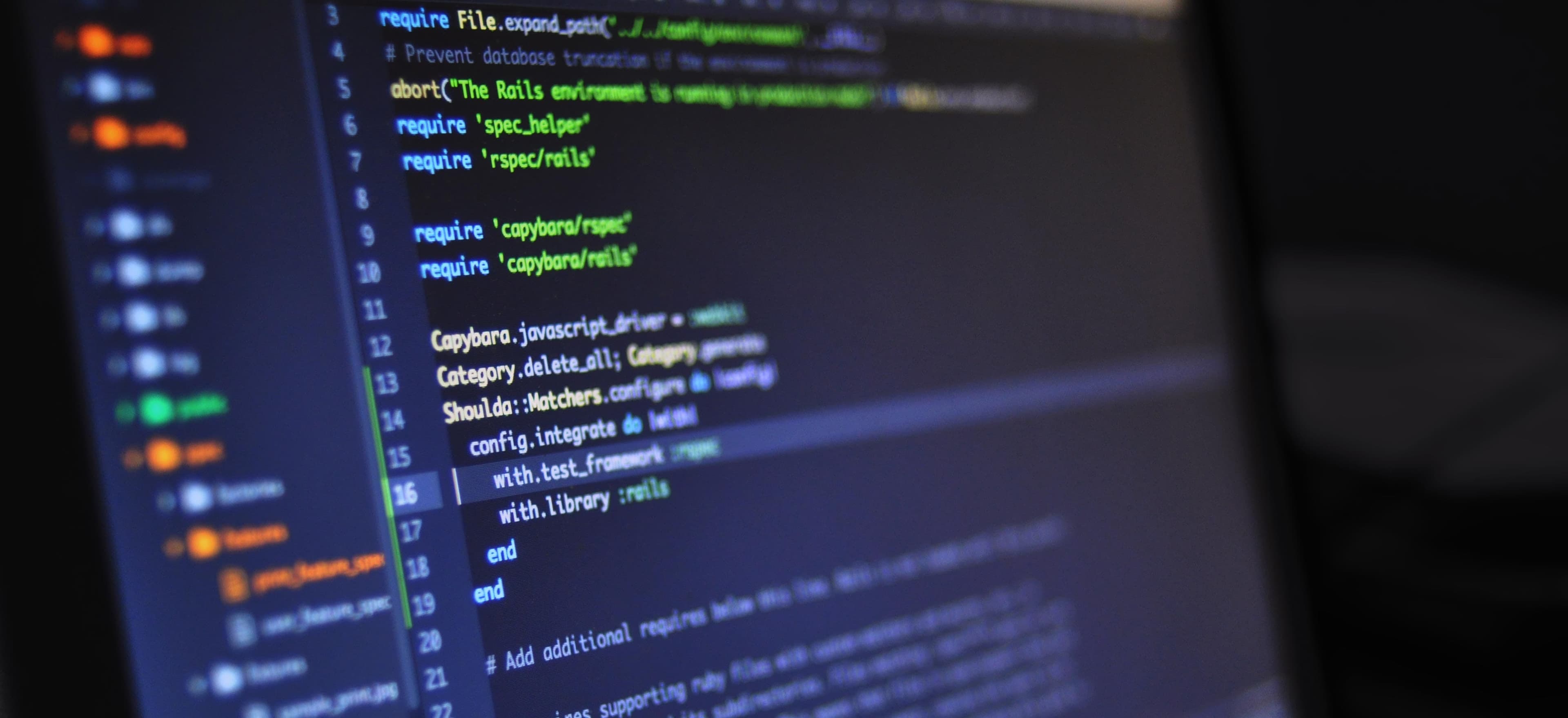
- Published on
How to Resolve Arquillian Configuration Issues in Java 8
When working on Java EE projects, testing can often feel like a daunting task, especially when dealing with deployment and integration tests. Arquillian is a powerful framework designed to ease this process by allowing developers to write integration tests that can run in the same environment as the application. However, configuring Arquillian can sometimes result in various issues that can stall your development process. In this blog post, we will explore common Arquillian configuration challenges in Java 8 and provide solutions to overcome them.
What is Arquillian?
Arquillian is an open-source testing platform for Java EE applications. It simplifies the process of setting up a testing environment by enabling developers to run tests in the actual container. This means you can test your Java EE application in a real-world scenario, which can reveal potential issues that unit tests may overlook.
Arquillian integrates seamlessly with various containers such as GlassFish, WildFly, Payara, and TomEE, making it a valuable tool for modern Java developers.
Common Configuration Issues in Arquillian
- Maven Dependency Issues
- Container Configuration Issues
- Test Packaging Issues
- Network Configuration Issues
- Classloader Issues
Let’s dive deeper into each of these issues and explore how to resolve them.
1. Maven Dependency Issues
One of the most frequent issues developers encounter with Arquillian is related to Maven dependencies. It is crucial to have the correct version of Arquillian and the container adapters.
Solution
Ensure that you have the correct dependencies defined in your pom.xml
. Below is an example of how to configure Arquillian with WildFly:
<dependencies>
<dependency>
<groupId>org.jboss.arquillian.junit</groupId>
<artifactId>arquillian-junit-container</artifactId>
<version>1.7.0.Final</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.jboss.arquillian.wildfly</groupId>
<artifactId>arquillian-wildfly-embedded-1.0.0.Final</artifactId>
<scope>test</scope>
</dependency>
<dependency>
<groupId>javax.enterprise</groupId>
<artifactId>cdi-api</artifactId>
<version>1.2</version>
<scope>provided</scope>
</dependency>
</dependencies>
Why This Matters: Specifying the correct versions synchronizes your project with Arquillian and avoids versioning conflicts that can lead to runtime failures.
2. Container Configuration Issues
If you are running into issues where Arquillian cannot start your desired container, it often means your container configurations are incorrect.
Solution
Check your Arquillian configuration file, typically located in src/test/resources
. For WildFly, the file would be named arquillian.xml
:
<arquillian xmlns="http://jboss.org/schema/arquillian">
<container qualifier="wildfly-remote" default="true">
<configuration>
<property name="jboss.url">http://localhost:9990</property>
<property name="jboss.username">admin</property>
<property name="jboss.password">password</property>
</configuration>
</container>
</arquillian>
Why This Matters: This file is crucial as it defines the connection parameters and other settings that Arquillian needs to connect to the container.
3. Test Packaging Issues
Another common issue arises when the test class is not packaged correctly, leading to class not found exceptions when tests are run.
Solution
Ensure you are using the maven-surefire-plugin
to run your tests. The configuration below ensures all test classes are picked up:
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-surefire-plugin</artifactId>
<version>2.22.2</version>
<configuration>
<includes>
<include>**/*Test.java</include>
<include>**/*Tests.java</include>
</includes>
</configuration>
</plugin>
</plugins>
</build>
Why This Matters: This configuration guarantees that your tests are detected by Maven, preventing missed test executions that can disrupt your development lifecycle.
4. Network Configuration Issues
Arquillian typically interacts with a remote server, and improper network settings can lead to connection failures.
Solution
Check your firewall or network policies. You need to ensure that the port you're using for the server (like 9990 for WildFly) is open and accessible. Also, if you are running tests in a Docker container, you might want to configure Docker networking appropriately.
Why This Matters: Proper network configuration ensures that the Arquillian can interact with the container without interruptions.
5. Classloader Issues
Conflicts in classloading can lead to NoClassDefFoundErrors or ClassNotFoundExceptions.
Solution
To deal with this, include the necessary libraries and dependencies within the arquillian.xml
file. You can also adjust your project's structure to avoid these conflicts.
<arquillian>
<container qualifier="wildfly-remote">
<configuration>
<property name="jboss.wildfly.ear">myapplication.ear</property>
</configuration>
</container>
</arquillian>
Why This Matters: Managing classloader issues allows your tests to run smoothly, minimizing runtime errors linked to missing classes.
Additional Resources
If you are looking for more information on Arquillian and its configurations, check out the following resources:
A Final Look
Resolving Arquillian configuration issues in Java 8 does not have to be a significant hurdle for your development process. By understanding the common pitfalls and implementing appropriate solutions, you can ensure a smoother testing experience. Remember to keep your dependencies updated, verify container configurations, adhere to proper packaging practices, maintain open network ports, and manage classloader dependencies.
With these strategies in hand, you can focus on what truly matters: building higher-quality Java EE applications with robust testing frameworks. Happy coding!
Checkout our other articles