State vs Strategy: Choosing the Right Design Pattern in Java
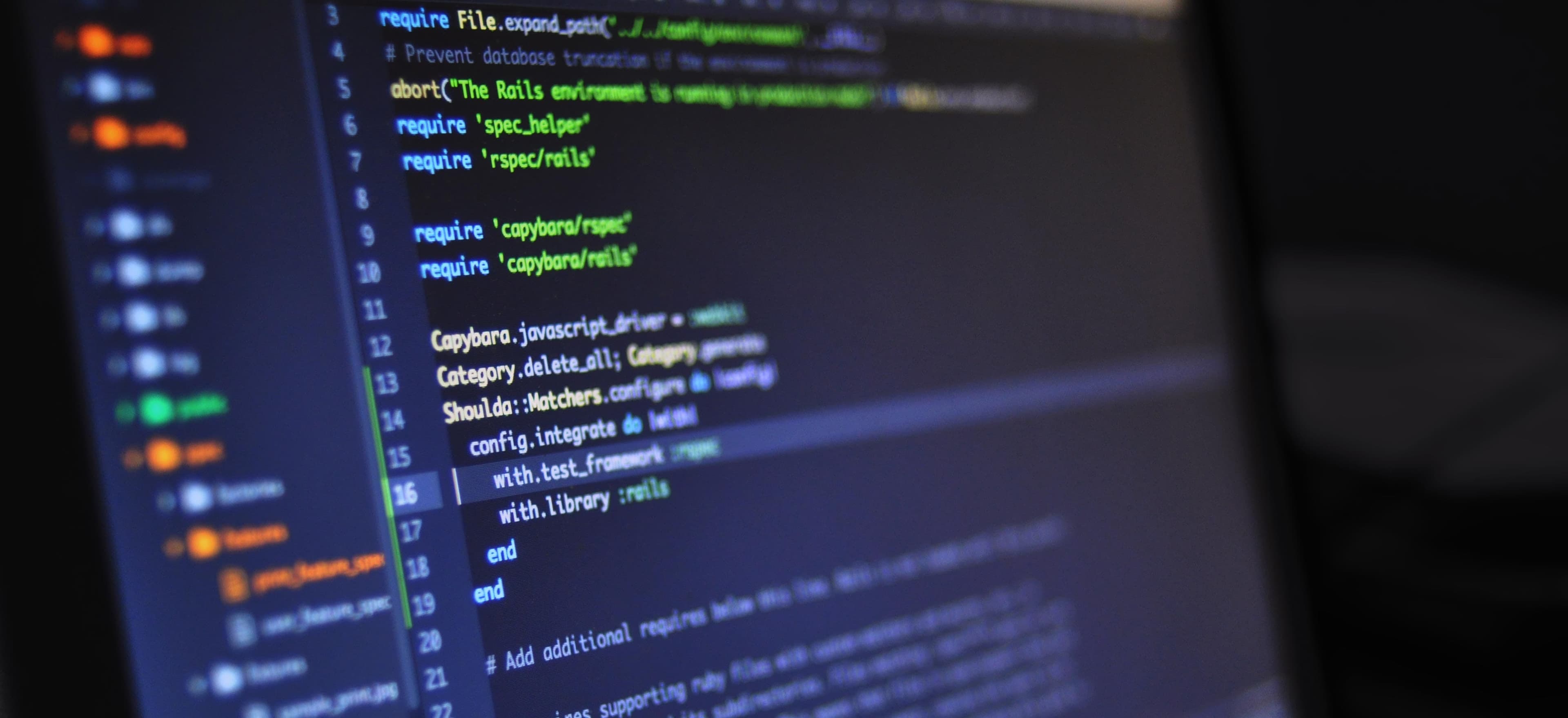
- Published on
State vs Strategy: Choosing the Right Design Pattern in Java
When developing software, choosing the appropriate design pattern is crucial to creating an adaptable and maintainable codebase. Among the numerous design patterns available, the State and Strategy patterns often cause confusion due to their similar principles and purpose. This article explores the differences and use cases for both State and Strategy design patterns in Java, helping you determine which pattern fits your needs better.
What Are Design Patterns?
Before diving into the specifics of State and Strategy patterns, let's define what design patterns are. Design patterns are established and reusable solutions to common software design problems. They promote best practices in programming, encouraging code reusability, maintainability, and flexibility.
Understanding the State Pattern
The State pattern is a behavioral design pattern that allows an object to change its behavior when its internal state changes. It enables an object to appear to change its class. This pattern is particularly useful in applications where the object's behavior should change based on its state.
When to Use the State Pattern
Use the State pattern when:
- An object's behavior is dependent on its state.
- You have many conditional statements managing an object's state.
- You want to encapsulate state-specific behavior and make it easier to maintain.
Implementation of the State Pattern in Java
Let's explore an example that clarifies the State design pattern:
// Context class
class Context {
private State state;
public Context(State state) {
this.state = state;
}
public void setState(State state) {
this.state = state;
}
public void request() {
state.handle(this);
}
}
// State interface
interface State {
void handle(Context context);
}
// Concrete States
class ConcreteStateA implements State {
public void handle(Context context) {
System.out.println("State A handling request.");
context.setState(new ConcreteStateB());
}
}
class ConcreteStateB implements State {
public void handle(Context context) {
System.out.println("State B handling request.");
context.setState(new ConcreteStateA());
}
}
// Client code
public class StatePatternDemo {
public static void main(String[] args) {
Context context = new Context(new ConcreteStateA());
context.request(); // Output: State A handling request.
context.request(); // Output: State B handling request.
}
}
Explanation of the Code
- Context Class: The
Context
class manages the current state. It provides a method for the client to request state-specific behavior. - State Interface: The
State
interface defines the contract for concrete states to implement. - Concrete States:
ConcreteStateA
andConcreteStateB
provide their unique implementation of thehandle
method, which also changes the current state of the context. - Client Code: The
StatePatternDemo
is where the context is initialized, and state transitions occur.
Understanding the Strategy Pattern
The Strategy pattern, another behavioral design pattern, defines a family of algorithms, encapsulates each one, and makes them interchangeable. The Strategy pattern lets the algorithm vary independently from clients that use it.
When to Use the Strategy Pattern
Utilize the Strategy pattern when:
- You have multiple algorithms or strategies that can be applied to a particular context.
- You want to reduce conditional statements that determine which algorithm to execute.
- You need to select an algorithm at runtime.
Implementation of the Strategy Pattern in Java
Here's a sample implementation of the Strategy pattern:
// Strategy interface
interface Strategy {
int execute(int a, int b);
}
// Concrete Strategy A
class Addition implements Strategy {
public int execute(int a, int b) {
return a + b;
}
}
// Concrete Strategy B
class Subtraction implements Strategy {
public int execute(int a, int b) {
return a - b;
}
}
// Context class
class Context {
private Strategy strategy;
public void setStrategy(Strategy strategy) {
this.strategy = strategy;
}
public int executeStrategy(int a, int b) {
return strategy.execute(a, b);
}
}
// Client code
public class StrategyPatternDemo {
public static void main(String[] args) {
Context context = new Context();
context.setStrategy(new Addition());
System.out.println("10 + 5 = " + context.executeStrategy(10, 5)); // Output: 15
context.setStrategy(new Subtraction());
System.out.println("10 - 5 = " + context.executeStrategy(10, 5)); // Output: 5
}
}
Explanation of the Code
- Strategy Interface: The
Strategy
interface defines the methodexecute
that all concrete strategies must implement. - Concrete Strategies: The
Addition
andSubtraction
classes implement theexecute
method with their respective algorithms. - Context Class: The
Context
class refers to aStrategy
and allows changing the strategy at runtime. - Client Code: The
StrategyPatternDemo
initializes the context and tests the various strategies.
Key Differences Between State and Strategy Patterns
While both patterns belong to the behavioral category and encapsulate behaviors, they serve different purposes:
-
Purpose:
- State Pattern: Manages state-related behavior; transitions between states; the state determines the behavior.
- Strategy Pattern: Defines interchangeable strategies; allows the algorithm to vary independently from the client.
-
Behavior Change:
- State Pattern: Behavior changes based on the state of the context.
- Strategy Pattern: Behavior changes based on the selected strategy.
-
Internal Context:
- State Pattern: The context maintains state information.
- Strategy Pattern: The context does not track the state; it can switch strategies at any point.
Choosing Between State and Strategy
Choosing between the State and Strategy patterns often depends on the problem at hand. Here are some key factors:
- Complexity: If the system has complex states with multiple state transitions and behaviors, the State pattern is more appropriate.
- Flexibility: If the system requires flexibility concerning algorithms or behaviors that change frequently, the Strategy pattern is the go-to solution.
The Closing Argument
Understanding the nuances between the State and Strategy design patterns can greatly enhance your software design capabilities. Both patterns encapsulate behavior and can contribute to cleaner, more maintainable code, but their applications and purposes are fundamentally different.
By aligning your design choice with the specific operational needs of your application, you can significantly improve your code's maintainability and agility. For more in-depth information regarding design patterns, consider visiting Refactoring Guru or DZone.
Now, with a clearer understanding of when to use the State and Strategy patterns, you're better equipped to tackle the challenges of designing robust applications in Java!
Checkout our other articles