Mastering Functional Style: Overcoming Common Pitfalls
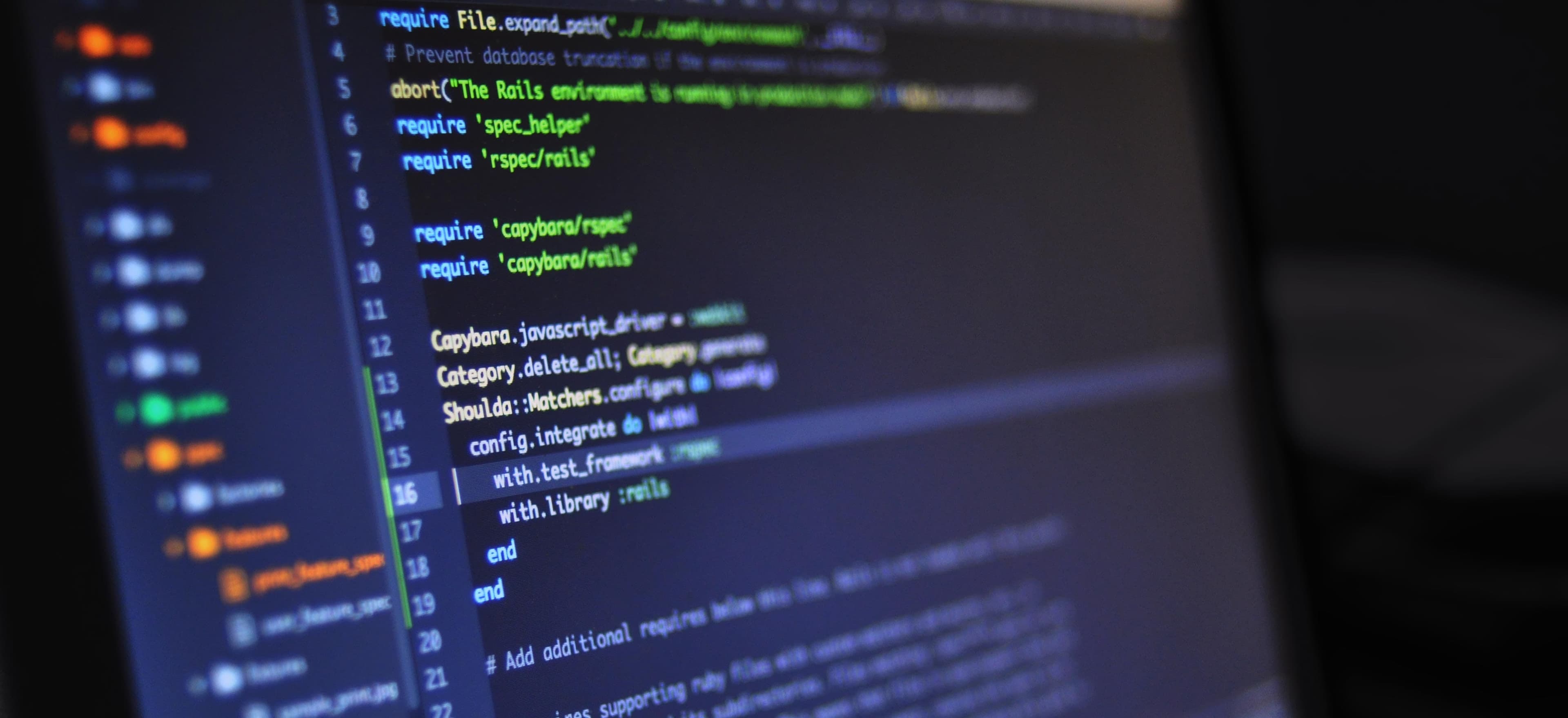
- Published on
Mastering Functional Style: Overcoming Common Pitfalls in Java
The paradigm of functional programming has gained significant traction in the Java community, especially with the introduction of Java 8 and its landmarks such as Lambda Expressions, Streams, and the Optional class. While adopting a functional style can improve code readability and encourage immutability, many developers encounter specific pitfalls. This blog post will guide you through the essential aspects of functional programming in Java while highlighting common errors and effective strategies to overcome them.
What is Functional Programming?
Functional programming is a programming paradigm that treats computation as the evaluation of mathematical functions. It avoids changing-state and mutable data. Within the Java ecosystem, functional programming enables developers to use higher-order functions, immutability concepts, and declarative-style coding.
Key Features of Functional Style in Java
- Lambda Expressions: Shortened syntax for anonymous functions that allow you to instantiate functional interfaces.
- Streams: An abstraction that allows complex data processing operations, such as filtering and mapping.
- Optionals: A container object used to define a value that may or may not be present, helping to avoid
NullPointerException
.
Common Pitfalls in Functional Style
- Excessive Use of Lambdas
- Complicated Stream Operations
- Ignoring Immutability
- Overusing Optional
1. Excessive Use of Lambdas
While Lambdas can make your code more concise, overusing them often leads to a reduction in readability. It is crucial to maintain a balance between conciseness and clarity.
Example:
List<String> names = Arrays.asList("Alice", "Bob", "Charlie");
// Excessive use of a lambda expression
List<String> filteredNames = names.stream()
.filter(name -> name.startsWith("A"))
.map(name -> name.toUpperCase())
.collect(Collectors.toList());
The above code is fine, but consider defining a method reference or a separate method if the logic becomes complex:
public static String filterAndUpperCase(String name) {
return name.toUpperCase();
}
List<String> filteredNames = names.stream()
.filter(name -> name.startsWith("A"))
.map(MyClass::filterAndUpperCase)
.collect(Collectors.toList());
This approach improves modularity and readability, easing unit testing for the filterAndUpperCase
method.
2. Complicated Stream Operations
Using Java streams can lead to complex chains of operations, often resulting in difficult-to-read code if mismanaged. Avoid creating long, intricate pipelines by breaking them down into manageable parts.
Example of complexity:
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5);
// Complicated chain
List<Integer> result = numbers.stream()
.filter(n -> n % 2 == 0)
.map(n -> n * n)
.filter(n -> n > 4)
.collect(Collectors.toList());
Instead:
List<Integer> evenNumbers = numbers.stream()
.filter(n -> n % 2 == 0)
.collect(Collectors.toList());
List<Integer> squaredNumbers = evenNumbers.stream()
.map(n -> n * n)
.filter(n -> n > 4)
.collect(Collectors.toList());
Breaking the chain promotes understanding and maintainability. It allows other developers (or you in the future) to navigate the logic seamlessly.
3. Ignoring Immutability
In Java, while functional programming emphasizes immutability, many developers sometimes overlook this principle, leading to unpredictable results.
Example:
List<String> names = new ArrayList<>(Arrays.asList("Alice", "Bob", "Charlie"));
// Attempting to modify the list
names.stream()
.filter(name -> name.startsWith("A"))
.forEach(name -> names.remove(name)); // Mutability issue
This results in a ConcurrentModificationException
. Instead, consider using a purely functional approach:
List<String> immutableNames = List.of("Alice", "Bob", "Charlie");
List<String> filteredNames = immutableNames.stream()
.filter(name -> name.startsWith("A"))
.collect(Collectors.toList());
Using List.of()
creates an immutable list, preventing state changes during stream operations.
4. Overusing Optional
The Optional class is an excellent feature for avoiding null references. However, overusing it can lead to more complexity than necessary.
Example of over-reliance:
Optional<String> optionalName = Optional.ofNullable(getName());
optionalName.ifPresent(name -> System.out.println(name.toUpperCase()));
Instead, consider simpler checks or default values:
String name = getName();
if (name != null) {
System.out.println(name.toUpperCase());
} else {
System.out.println("Default Name");
}
Using Optional should primarily be reserved for method return types, not field types or method parameters.
Closing Remarks
Mastering functional programming in Java involves understanding its principles and avoiding common pitfalls. A functional approach fosters clearer code, but it requires a disciplined and thoughtful practice.
Developers should remain wary of overusing features like Lambdas and Streams, embracing readability with clarity. Keeping immutability and judiciously utilizing Optional will enhance code reliability and maintainability.
To deepen your understanding and see practical examples, consider reading Oracle's official Java documentation for functional interfaces or dive into a comprehensive tutorial on Java Streams.
By following these guidelines, your journey toward mastering functional programming in Java will not only be fruitful but also exciting. Happy coding!