Common Pitfalls When Creating PostgreSQL Schema Pre-Liquibase
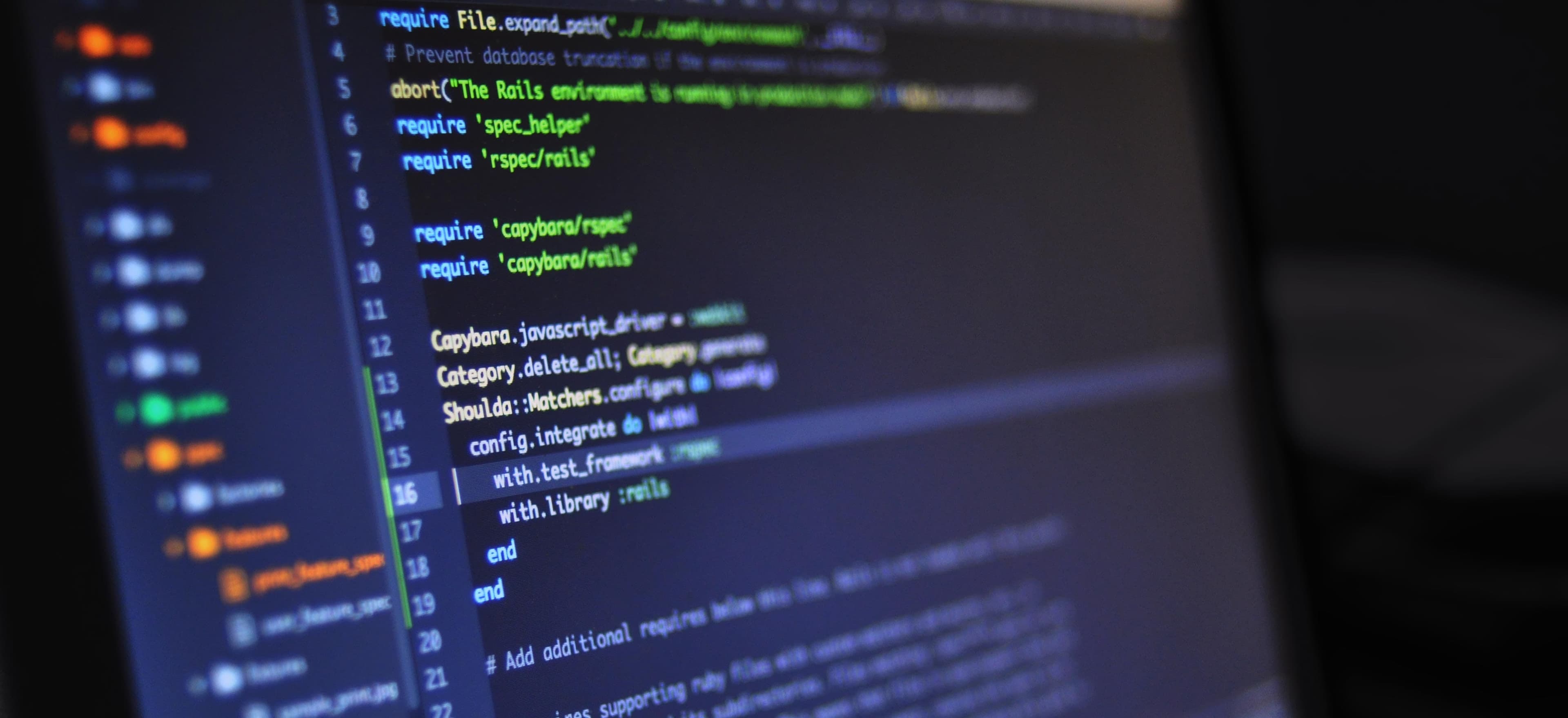
- Published on
Common Pitfalls When Creating PostgreSQL Schema Pre-Liquibase
Creating a robust PostgreSQL schema is crucial for the efficient operation of your database. However, there are several pitfalls developers might encounter when designing their schemas, particularly before utilizing tools like Liquibase for version control and schema management. This blog post will delve into these common pitfalls, how to avoid them, and offer some best practices along the way.
1. Lack of a Clear Naming Convention
One of the most fundamental aspects of a well-structured schema is a consistent naming convention. In PostgreSQL, not having a clear naming strategy can lead to confusion and make the database challenging to work with.
Why Naming Conventions Matter
Effective naming conventions improve readability and maintainability. For example, consider the following naming formats for tables:
- Bad Table Names:
tblUsers
,DataYouMightNeed
. - Good Table Names:
users
,order_items
.
Recommended Practices
- Lowercase: Prefer lower case for tables and columns to avoid case sensitivity issues.
- Descriptive Names: Use names that represent what the table or column is intended to store.
- Prefix/Suffix Usage: Apply prefixes/suffixes to signify the type of entity, such as
tbl_
for tables or_id
for identifiers.
2. Ignoring Data Types
Choosing inappropriate data types can lead to performance issues and complications in application logic.
Common Mistakes
- Using
TEXT
instead ofVARCHAR(255)
when the length is known. - Employing
FLOAT
for currency values, whereDECIMAL
is more precise.
Example Code Snippet
CREATE TABLE products (
id SERIAL PRIMARY KEY,
name VARCHAR(255) NOT NULL,
price DECIMAL(10, 2) NOT NULL
);
Why This Matters: The DECIMAL(10, 2)
ensures that the price retains two decimal places, making it ideal for currency.
3. Failure to Normalize Data
Normalization is the process of organizing data to reduce redundancy. Failing to normalize can lead to data anomalies and an inefficient database structure.
Example of Non-Normalized vs. Normalized Data
Non-Normalized Schema:
CREATE TABLE orders (
id SERIAL PRIMARY KEY,
product_name VARCHAR(255),
price DECIMAL(10, 2),
customer_name VARCHAR(255),
customer_address VARCHAR(255)
);
Normalized Schema:
CREATE TABLE customers (
id SERIAL PRIMARY KEY,
name VARCHAR(255) NOT NULL,
address VARCHAR(255)
);
CREATE TABLE orders (
id SERIAL PRIMARY KEY,
customer_id INT REFERENCES customers(id),
product_name VARCHAR(255),
price DECIMAL(10, 2)
);
Why Normalize?: The normalized approach minimizes duplication (e.g., customer information) and allows for easier updates and better database integrity.
4. Unindexed Columns
Indexes are crucial for speeding up data retrieval. Neglecting to create indexes on frequently searched columns can severely degrade performance.
Common Mistake
Not indexing foreign keys can lead to slow join operations.
Example Code Snippet
CREATE TABLE orders (
id SERIAL PRIMARY KEY,
customer_id INT REFERENCES customers(id),
product_name VARCHAR(255),
price DECIMAL(10, 2),
created_at TIMESTAMP DEFAULT NOW(),
INDEX idx_customer_id (customer_id) -- Adding index on foreign key
);
Why Indexing is Important: In our example, idx_customer_id
helps the system quickly find all orders related to a specific customer, enhancing query performance.
5. Ignoring Foreign Key Constraints
Foreign key constraints ensure data integrity by enforcing relationships between tables. Ignoring these constraints can lead to orphaned records or inconsistent data.
Example Code Snippet
CREATE TABLE products (
id SERIAL PRIMARY KEY,
name VARCHAR(255) NOT NULL
);
CREATE TABLE order_items (
id SERIAL PRIMARY KEY,
order_id INT REFERENCES orders(id) ON DELETE CASCADE,
product_id INT REFERENCES products(id),
quantity INT NOT NULL
);
Why Foreign Keys Matter: The foreign key constraints help maintain referential integrity. The ON DELETE CASCADE
option ensures that if an order is deleted, all associated order items are automatically removed.
6. Insufficient Documentation
Lack of documentation makes it difficult for others (or even your future self) to understand the database schema after some time.
Recommended Practices
- Document each table's purpose.
- Explain the relationships between different tables.
- Maintain an entity-relationship diagram (ERD).
Using Liquibase for Documentation
Liquibase allows you to create change logs that serve as documentation. By maintaining these logs, you ensure that the schema changes are always well-documented and easily traceable.
7. Hardcoding Values in the Schema
Hardcoding values into the schema design can lead to issues with flexibility and scalability. It can also make migrating to new environments cumbersome.
Example of Hardcoding Issues
Bad Practice:
CREATE TABLE users (
id SERIAL PRIMARY KEY,
name VARCHAR(100),
email VARCHAR(255) UNIQUE,
user_type CHAR(1) DEFAULT 'C' -- Hardcoded User Type
);
Better Practice:
Using a separate lookup table for user types:
CREATE TABLE user_types (
id SERIAL PRIMARY KEY,
type_name VARCHAR(50) NOT NULL
);
CREATE TABLE users (
id SERIAL PRIMARY KEY,
name VARCHAR(100),
email VARCHAR(255) UNIQUE,
user_type_id INT REFERENCES user_types(id)
);
Why This Matters: By separating user types into its own table, you can easily modify the types without changing the schema. This enhances flexibility in your application.
8. Not Planning for Future Growth
Schema design should anticipate future requirements. Failing to incorporate flexibility can lead to significant overhead when changes are needed.
Example Scenario
Consider adding a column for user roles after the schema is in use. If this is not planned for, you may find yourself in complicated arrangements.
Best Practice
Regularly review the schema design with team members to anticipate and accommodate future changes or requirements. You might use UML diagrams or other planning tools to help visualize and discuss future needs.
The Closing Argument
Creating an optimal PostgreSQL schema requires careful thought and planning. By understanding these common pitfalls and implementing best practices, you can build a more efficient, maintainable, and scalable database design.
Additional Resources
To deepen your understanding of PostgreSQL schema design, consider the following resources:
Remember, a good schema design not only affects current performance but sets the groundwork for future evolution. By avoiding these common pitfalls, you are better positioned to create a schema that stands the test of time.