Why Builder Pattern Can Complicate Your Tests
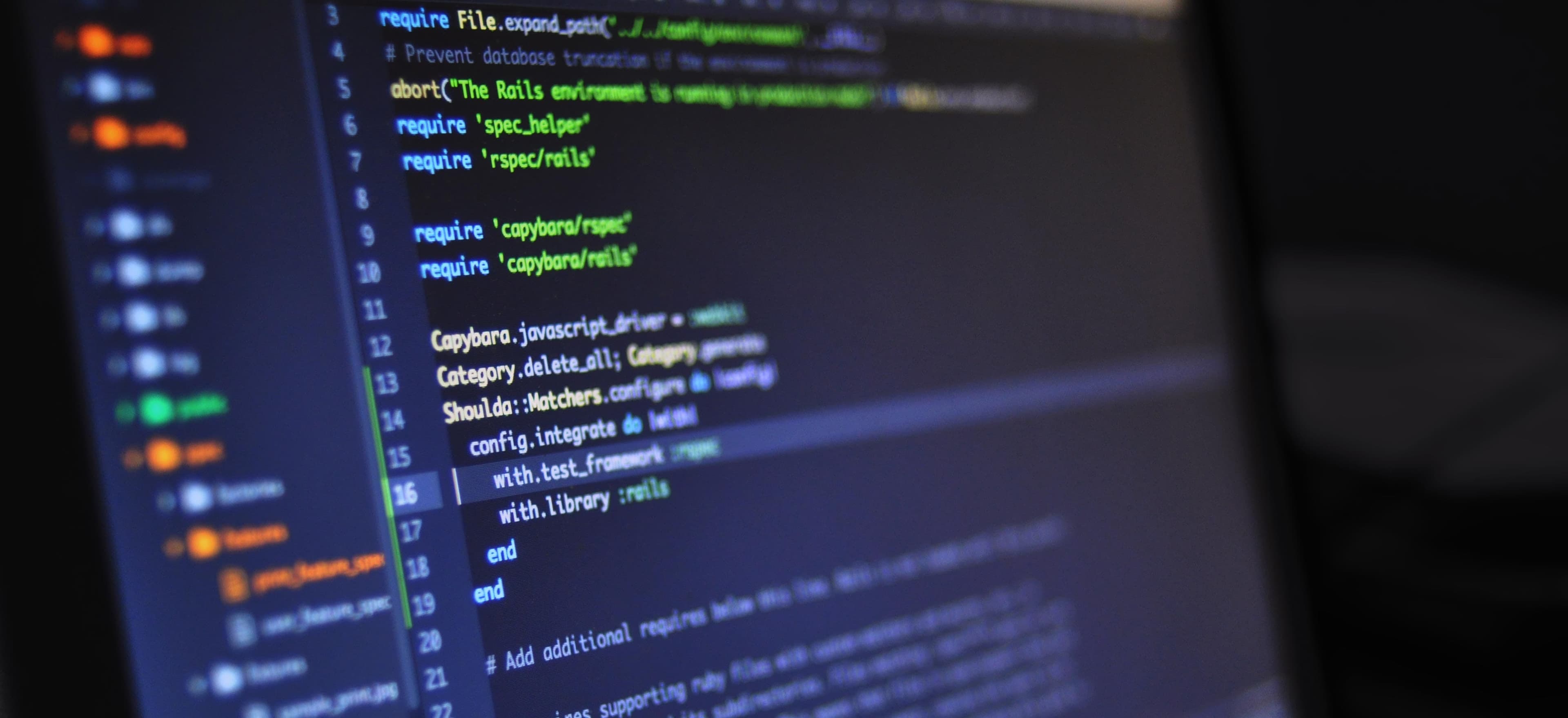
- Published on
Why the Builder Pattern Can Complicate Your Tests
In software design, patterns are essential for facilitating maintainable and readable code. The Builder Pattern stands out for its ability to construct complex objects step by step. It separates the construction of a product from its representation, which can be incredibly beneficial. However, it contains pitfalls, especially when it comes to testing.
This post will delve into why the Builder Pattern may add complexity to your tests, and how to navigate these challenges effectively while staying aligned with best practices.
Understanding the Builder Pattern
Before diving into its implications on testing, let’s start with a brief overview of the Builder Pattern.
What is the Builder Pattern?
The Builder Pattern is a creational design pattern that allows for constructing an object step by step. It is particularly useful when an object needs to be created in a series of steps, which varies based on the input parameters.
Example of the Builder Pattern
Here’s a simple example in Java using a Car
object.
public class Car {
private String make;
private String model;
private int year;
private String color;
// Private constructor to enforce the use of the Builder
private Car(CarBuilder builder) {
this.make = builder.make;
this.model = builder.model;
this.year = builder.year;
this.color = builder.color;
}
public static class CarBuilder {
private String make;
private String model;
private int year;
private String color;
public CarBuilder setMake(String make) {
this.make = make;
return this;
}
public CarBuilder setModel(String model) {
this.model = model;
return this;
}
public CarBuilder setYear(int year) {
this.year = year;
return this;
}
public CarBuilder setColor(String color) {
this.color = color;
return this;
}
public Car build() {
return new Car(this);
}
}
}
Why Use the Builder Pattern?
- Clarity: It enhances code readability and clarity.
- Flexibility: Allows for the creation of various object configurations without overloads.
- Immutability: The immutable objects encourage safe sharing of instances across threads.
Complications Introduced
While the Builder Pattern offers these advantages, it also complicates testing scenarios. Below are the primary concerns:
1. Increased Complexity
When you utilize a Builder, you often introduce additional classes and functionality. This complexity can make your tests harder to read and interpret.
Code Example
Consider the following test case for creating a Car
object.
import org.junit.jupiter.api.Test;
public class CarTest {
@Test
void testCarCreation() {
Car myCar = new Car.CarBuilder()
.setMake("Toyota")
.setModel("Corolla")
.setYear(2022)
.setColor("Blue")
.build();
assertEquals("Toyota", myCar.getMake());
// Additional assertions can follow...
}
}
This scenario requires more setup and cognitive load than testing a simple constructor.
2. Fragile Tests
When builders introduce additional layers, a change in the underlying implementation can lead to fragile tests. Suppose we decide to add validation logic within the build
method, the existing tests may fail even if the object returned is technically correct.
Example of Fragile Test
If you modify the CarBuilder
to include validation:
public Car build() {
if (year < 1886) { // The first car was invented in 1886
throw new IllegalStateException("Year must be 1886 or later.");
}
return new Car(this);
}
Now, existing tests may throw unexpected exceptions, despite valid input, thus leading to a maintenance nightmare.
3. Heavier Mocking
In situations where a builder cannot provide default values, tests can often produce a significant amount of boilerplate code. If you modify your builder’s API, you may need to update many tests that instantiate the Builder.
Add mocks when necessary:
import static org.mockito.Mockito.*;
public class CarServiceTest {
@Test
void testCarService() {
CarBuilder carBuilderMock = mock(CarBuilder.class);
when(carBuilderMock.build()).thenReturn(new Car(/* parameters */));
// Actual service test logic...
}
}
This mock complexity further raises the barrier for newcomers to easily grasp your tests.
Strategies to Mitigate Complications
While the Builder Pattern can create complications, there are ways to alleviate the burden:
1. Use Default Values
You can incorporate sensible defaults in your builder to reduce setup code in tests. Users can override only necessary fields.
public CarBuilder setColor(String color) {
this.color = (color == null) ? "Black" : color; // Default color
return this;
}
2. Keep Testing Simple
Concentrate on testing behavior rather than implementation. If object creation complexities escalate, consider abstracting the creation logic into a factory.
3. Utilize Parameterized Tests
If you need to test different configurations, consider using parameterized tests to reduce test redundancy.
4. Write Comprehensive Tests
While it may seem counterintuitive, more extensive test cases—it can incorporate various scenarios without needing to alter existing tests when changes occur.
To Wrap Things Up
The Builder Pattern has its merits: enhancing clarity, flexibility, and promoting immutability in object creation. However, it also brings testing complexity, fragility, and mocking overhead.
To achieve the benefits while minimizing headaches, it’s essential to keep tests focused, to use sensible defaults, and to maintain the simplicity of test setups.
By leveraging these strategies, you can navigate the nuances of the Builder Pattern without sinking into unmanageable test complexity.
For further reading on design patterns, you might find Refactoring Guru and Baeldung useful resources. Happy coding!
Checkout our other articles