Mastering the Receptionist Pattern in Akka Typed Actors
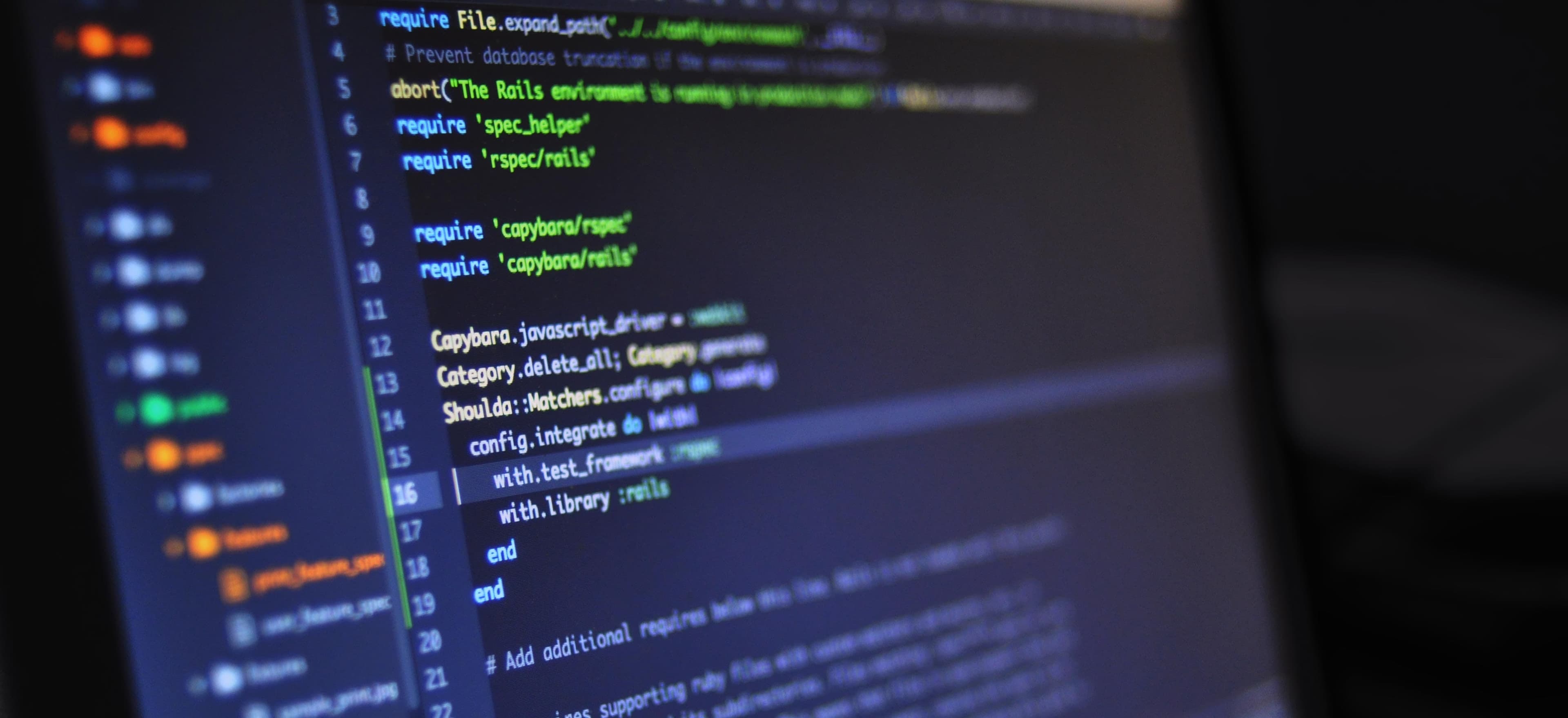
- Published on
Mastering the Receptionist Pattern in Akka Typed Actors
Akka Typed provides a powerful framework for building concurrent applications in a scalable way. One of its fundamental paradigms is the Actor Model, which simplifies complex operations by defining an isolated unit of computation. Within this architectural framework, the Receptionist pattern plays a pivotal role. This blog post will explore the Receptionist pattern in Akka Typed Actors, explaining its purpose, use cases, and providing code snippets to demonstrate its practical applications.
What is the Receptionist Pattern?
The Receptionist pattern allows actors to automatically manage the registration and lookup of other actors in a system. In essence, it provides a way to register actors and allows other actors—usually clients or controllers—to discover and interact with them without needing direct references.
When you think of the receptionist in a real office, you might imagine someone who directs visitors to the needed departments. Similarly, in Akka, the Receptionist takes care of routing messages to the appropriate actors based on registered identifiers.
Benefits of the Receptionist Pattern
-
Loose Coupling: Clients can send messages without knowing the underlying implementation details of the actors they are interacting with.
-
Dynamic Discovery: New actors can be registered and existing actors can be easily queried, making the system adaptable to changes at runtime.
-
Actor Lifecycle Management: Helps in managing the lifecycle of actors, as registration and lookup can help control the instances of those actors effectively.
Setting Up Your Akka Typed Project
Before diving into the implementation, ensure you have a Scala project set up with Akka Typed. If you're new to Akka, visit the official Akka documentation for installation instructions.
Add these dependencies to your build.sbt
file:
libraryDependencies += "com.typesafe.akka" %% "akka-actor-typed" % "2.7.0"
Implementation of the Receptionist Pattern
Let’s consider a simple scenario where we manage a set of workers in a system. Our workers will be registered with a receptionist, which clients can consult for worker references.
Step 1: Define the Worker Actor
import akka.actor.typed.ActorRef
import akka.actor.typed.Behavior
import akka.actor.typed.javadsl.Behaviors
object Worker {
sealed trait Command
final case class ReportWork(task: String, replyTo: ActorRef[String]) extends Command
def apply(id: String): Behavior[Command] = Behaviors.receive { (context, message) =>
message match {
case ReportWork(task, replyTo) =>
// Process the task and send a simple response
replyTo ! s"Worker $id completed task: $task"
Behaviors.same
}
}
}
Why This Code?
In this example, the Worker
actor processes tasks it receives. It has a method called ReportWork
, which takes a task as input and sends the response back via the replyTo
ActorRef. This encapsulates the work the actor does while maintaining a clear interface.
Step 2: Define the Receptionist Actor
import akka.actor.typed.scaladsl.Behaviors
import akka.actor.typed.{ActorRef, Behavior, SupervisorStrategy}
object Receptionist {
sealed trait Command
final case class RegisterWorker(id: String, worker: ActorRef[Worker.Command]) extends Command
final case class GetWorker(id: String, replyTo: ActorRef[Option[ActorRef[Worker.Command]]]) extends Command
def apply(): Behavior[Command] = Behaviors.setup { context =>
// Use a map to keep track of registered workers
var workers = Map.empty[String, ActorRef[Worker.Command]]
Behaviors.receiveMessage {
case RegisterWorker(id, worker) =>
workers += (id -> worker)
Behaviors.same
case GetWorker(id, replyTo) =>
replyTo ! workers.get(id)
Behaviors.same
}.onSignal {
case (PreRestart, _) =>
// Logic to handle actor restarts can go here, if required
Behaviors.same
}
}
}
Why This Code?
The Receptionist
collects worker actors into a map using their IDs as keys. The RegisterWorker
message allows a worker to register itself, while GetWorker
returns the worker corresponding to the specified ID. This architecture caters to the dynamic nature of actors using flexible storage and retrieval methods.
Step 3: Main Application
Now, let's see how to wire everything together in a main application.
import akka.actor.typed.ActorSystem
object Main extends App {
val receptionist: ActorRef[Receptionist.Command] =
ActorSystem(Receptionist(), "ReceptionistActorSystem")
val worker1 = receptionist.narrow[Receptionist.Command] ! Receptionist.RegisterWorker("worker1", Worker("worker1"))
val worker2 = receptionist.narrow[Receptionist.Command] ! Receptionist.RegisterWorker("worker2", Worker("worker2"))
// Getting a worker by ID
val workerIdToQuery = "worker1"
receptionist ! Receptionist.GetWorker(workerIdToQuery, replyTo = ActorRef.noSender)
}
Why This Code?
In the Main
object, we create a system that initializes the Receptionist
actor. We register two workers and demonstrate querying for one of them. This showcases how clients can interest and interact with your actors without requiring tight coupling.
The Closing Argument
Leveraging the Receptionist pattern in Akka Typed Actors empowers you to build scalable and flexible applications. By enriching interactions between actors, the Receptionist pattern fosters a loose coupling strategy that significantly enhances system maintainability.
Further Reading
For more advanced usage scenarios and features, consider checking out the following resources:
Mastery over patterns like the Receptionist can lead to more resilient and adaptable architectures, making your applications not only faster but also more efficient. Happy coding!