Mastering Edit Distance: Simplifying Dynamic Programming
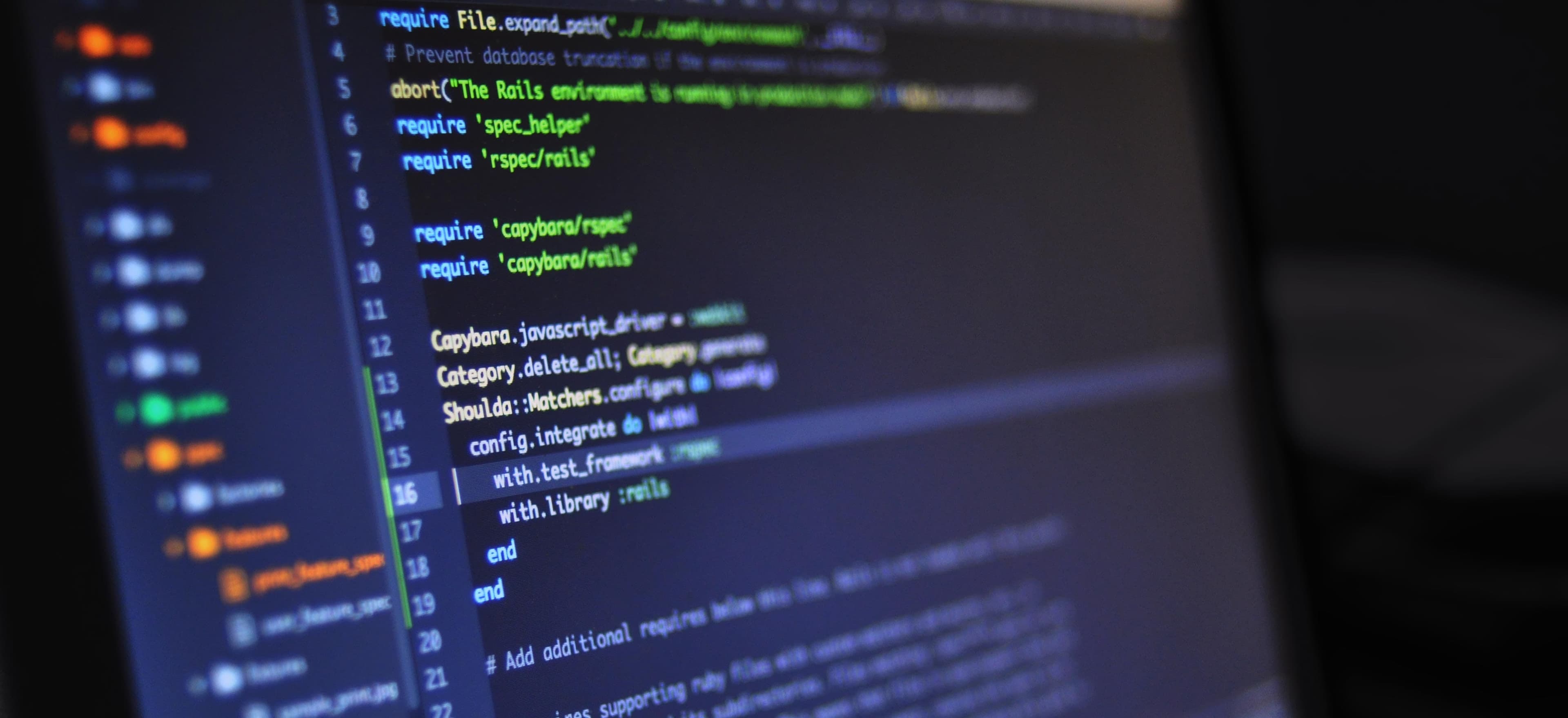
- Published on
Mastering Edit Distance: Simplifying Dynamic Programming
Dynamic programming is a powerful technique in algorithm design that can solve complex problems by breaking them down into simpler subproblems. One critical application of dynamic programming is the calculation of Edit Distance. In this blog post, we will explore the concept of Edit Distance, its significance, how to compute it using dynamic programming, and the rationale behind the approach.
What is Edit Distance?
Edit Distance (also known as Levenshtein Distance) measures how dissimilar two strings are by counting the minimum number of operations required to transform one string into another. The allowed operations typically include:
- Insertion: Adding a character to the string.
- Deletion: Removing a character from the string.
- Substitution: Replacing one character with another.
For example, transforming "kitten" to "sitting" involves the following operations:
- Substitute 'k' with 's' → "sitten"
- Substitute 'e' with 'i' → "sittin"
- Insert 'g' at the end → "sitting"
The Edit Distance for this example is 3.
Why is Edit Distance Important?
Edit Distance is widely used in various fields, including:
- Spell Checking: Determining how close a misspelled word is to valid words.
- DNA Sequencing: Comparing genetic sequences.
- Natural Language Processing: Measuring similarity or differences in text.
Understanding the edit distance between two strings is foundational in building robust algorithms for these applications.
Calculating Edit Distance Using Dynamic Programming
Dynamic programming simplifies problems by storing the results of subproblems, allowing efficient computation through recursion and overlapping subproblems.
The algorithm for computing Edit Distance can be broken down into the following steps:
-
Create a Matrix: Define a matrix where the cell
(i, j)
represents the Edit Distance between the firsti
characters of stringA
and the firstj
characters of stringB
. -
Initialize the Matrix:
- The first row and column represent transformations from an empty string. For instance, transforming "" to "abc" requires three insertions.
-
Fill the Matrix:
- If the characters at position
i
inA
andj
inB
are the same, no new operation is needed. Otherwise, compute the costs for insertion, deletion, and substitution.
- If the characters at position
-
Trace the Result: The bottom-right cell of the matrix contains the Edit Distance between the two strings.
Here is a Java implementation:
public class EditDistance {
// Function to calculate Edit Distance
public static int calculateEditDistance(String str1, String str2) {
int m = str1.length();
int n = str2.length();
// Create a matrix to store results of subproblems
int[][] dp = new int[m + 1][n + 1];
// Initialize the first row and column
for (int i = 0; i <= m; i++) {
dp[i][0] = i; // Deleting all characters from str1
}
for (int j = 0; j <= n; j++) {
dp[0][j] = j; // Adding all characters of str2
}
// Fill the matrix
for (int i = 1; i <= m; i++) {
for (int j = 1; j <= n; j++) {
// If characters are the same, don't incur any cost
if (str1.charAt(i - 1) == str2.charAt(j - 1)) {
dp[i][j] = dp[i - 1][j - 1];
} else {
// Calculate costs for insertion, deletion, and substitution
int insertCost = dp[i][j - 1] + 1; // insert str2[j-1]
int deleteCost = dp[i - 1][j] + 1; // remove str1[i-1]
int substituteCost = dp[i - 1][j - 1] + 1; // substitute str1[i-1] with str2[j-1]
dp[i][j] = Math.min(insertCost, Math.min(deleteCost, substituteCost));
}
}
}
// The edit distance is in the bottom-right cell
return dp[m][n];
}
public static void main(String[] args) {
String str1 = "kitten";
String str2 = "sitting";
int distance = calculateEditDistance(str1, str2);
System.out.println("Edit Distance between " + str1 + " and " + str2 + " is: " + distance);
}
}
Explanation of the Code
- The matrix dp is created to store the intermediate results, reducing the computation time.
- The initialization steps set up the base cases, allowing us to start with simple transformations (from an empty string).
- The nested loops fill out the matrix by comparing characters and calculating the costs of operations using the previously computed values.
Through these systematic calculations, we can achieve an efficient solution to the Edit Distance problem, which runs in O(m * n) time complexity, where m and n are the lengths of the two strings.
Visualizing the Edit Distance Calculation
It helps to visualize the dynamic programming matrix during execution. For "kitten" and "sitting", our matrix transformation would look as follows:
| | | s | i | t | t | i | n | g | |----|---|---|---|---|---|---|---|---| | | 0 | 1 | 2 | 3 | 4 | 5 | 6 | 7 | | k | 1 | 1 | 2 | 3 | 4 | 5 | 6 | 7 | | i | 2 | 2 | 1 | 2 | 3 | 4 | 5 | 6 | | t | 3 | 3 | 2 | 1 | 2 | 3 | 4 | 5 | | t | 4 | 4 | 3 | 2 | 1 | 2 | 3 | 4 | | e | 5 | 5 | 4 | 3 | 2 | 2 | 3 | 4 | | n | 6 | 6 | 5 | 4 | 3 | 3 | 2 | 3 |
The bottom-right cell contains the final Edit Distance, which in this case is 3.
Practical Applications
Edit Distance has vast applications across various domains. Here are some use cases:
- Text Comparison Tools: Applications like diff find the difference between files in programming and documentation.
- Search Query Autocomplete: Search engines can suggest words based on user input.
- Genomic Research: In bioinformatics, DNA sequence alignment employs Edit Distance.
You can further explore dynamic programming and sequence alignment in more detail on resources like GeeksforGeeks.
Closing Remarks
Understanding Edit Distance not only illuminates dynamic programming principles but also equips developers with the tools to solve complex string manipulation problems. The technique offers imperturbable accuracy in various applications, enhancing user experience across technologies.
With this knowledge, you've taken a significant step towards mastering dynamic programming — a skill that will open new avenues in your programming journey. Continue exploring more algorithms and their applications, and you will find endless possibilities for applying dynamic programming in creative ways.
Happy coding!