Mastering Java Spark RDD: Common Reduce Pitfalls to Avoid
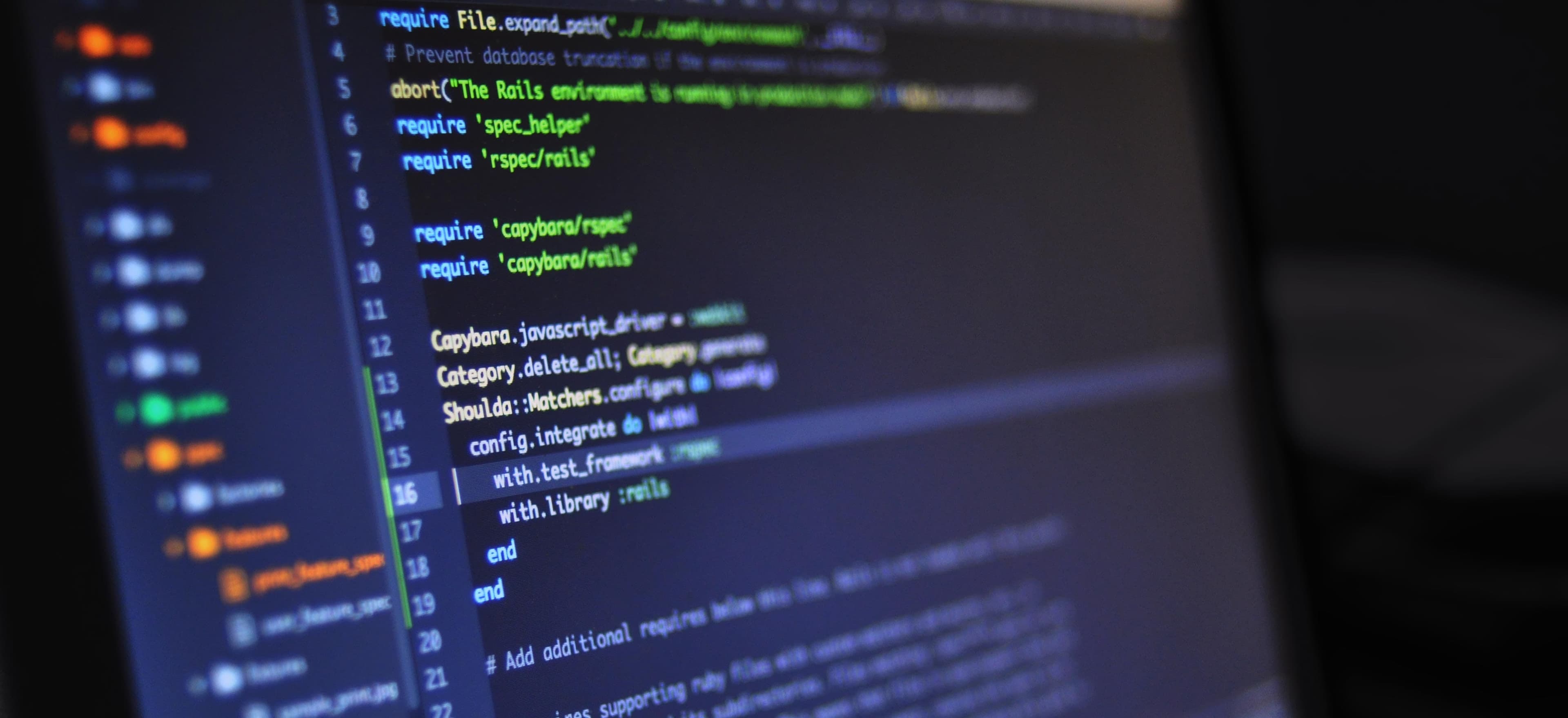
- Published on
Mastering Java Spark RDD: Common Reduce Pitfalls to Avoid
Apache Spark is a powerful engine for data processing, and it offers several abstractions for working with data. One of the most vital components of Spark is the Resilient Distributed Dataset (RDD). While RDDs provide flexible and efficient transformations and actions, developers often encounter pitfalls when using reduce operations. In this post, we will explore common reduce pitfalls and how to avoid them, ensuring your Spark applications run smoothly and efficiently.
Table of Contents
- What is Spark RDD?
- The Reduce Operation in RDD
- Common Pitfalls in Using Reduce
- 3.1 Improper Use of the Reduce Function
- 3.2 Forgetting to Handle Nulls
- 3.3 Using Reduce Instead of Aggregate
- 3.4 Assuming Identity for Reduce
- 3.5 Misunderstanding Data Distribution
- Best Practices for Reduce in Spark RDD
- Conclusion
1. What is Spark RDD?
Resilient Distributed Datasets (RDDs) are Spark's fundamental data structure. They are a distributed collection of objects, allowing for fault-tolerant parallel processing. RDDs can be created from existing data in storage or derived from transformations of other RDDs, such as maps or filters.
RDDs provide a series of operations, including transformations (e.g., map
, filter
) and actions (e.g., collect
, count
). They allow developers to work with large datasets across a distributed cluster efficiently.
Sample Code: Creating an RDD
Here’s a simple example to illustrate how to create an RDD in Java:
import org.apache.spark.api.java.JavaSparkContext;
import org.apache.spark.api.java.JavaRDD;
import org.apache.spark.SparkConf;
public class RDDExample {
public static void main(String[] args) {
SparkConf conf = new SparkConf().setAppName("RDD Example").setMaster("local");
JavaSparkContext sc = new JavaSparkContext(conf);
// Create an RDD from a list of integers
JavaRDD<Integer> rdd = sc.parallelize(Arrays.asList(1, 2, 3, 4, 5));
sc.close();
}
}
In this code snippet, we set up a Spark context and create an RDD from a list of integers. We'll explore how to apply reduce operations on this dataset.
2. The Reduce Operation in RDD
The reduce
operation in Spark takes two elements and merges them together. This operation is typically used for aggregating data. The result reduces the dataset to a single output value by applying a binary operator.
Sample Reduce Code
Here's how you can use the reduce
operation in Java:
import org.apache.spark.api.java.JavaSparkContext;
import org.apache.spark.api.java.JavaRDD;
import org.apache.spark.SparkConf;
public class ReduceExample {
public static void main(String[] args) {
SparkConf conf = new SparkConf().setAppName("Reduce Example").setMaster("local");
JavaSparkContext sc = new JavaSparkContext(conf);
JavaRDD<Integer> rdd = sc.parallelize(Arrays.asList(1, 2, 3, 4, 5));
// Reduce the RDD to a single sum
Integer sum = rdd.reduce((a, b) -> a + b);
System.out.println("Sum: " + sum);
sc.close();
}
}
In this code, we calculate the total sum of the RDD. Understanding the nuances of the reduce operation can save you from several pitfalls.
3. Common Pitfalls in Using Reduce
3.1 Improper Use of the Reduce Function
One common mistake is applying the reduce
function incorrectly. The reduce
operation must be both associative and commutative. If it isn't, the result can vary based on how Spark partitions the data.
Example of a Pitfall
Suppose you have the following:
JavaRDD<Integer> rdd = sc.parallelize(Arrays.asList(1, 2, 3, 4, 5));
Integer wrongResult = rdd.reduce((a, b) -> a - b); // Not associative
In this instance, a - b
may yield different results based on the order of calculations. Always ensure your function is associative & commutative.
3.2 Forgetting to Handle Nulls
Null values can lead to unexpected behavior during the reduce operation. If your dataset contains nulls and you do not handle them, you may encounter NullPointerExceptions
.
Solution
Ensure to filter out null values before reduction:
JavaRDD<Integer> rdd = sc.parallelize(Arrays.asList(1, null, 3, 4, 5));
Integer sum = rdd.filter(x -> x != null).reduce((a, b) -> a + b);
3.3 Using Reduce Instead of Aggregate
Using reduce
instead of aggregate
can limit your ability to return complex data structures. If you need to maintain additional statistics or outputs, consider using aggregate
.
JavaRDD<Integer> rdd = sc.parallelize(Arrays.asList(1, 2, 3, 4, 5));
Tuple2<Integer, Integer> result = rdd.aggregate(new Tuple2<>(0, 0),
(acc, value) -> new Tuple2<>(acc._1 + value, acc._2 + 1),
(acc1, acc2) -> new Tuple2<>(acc1._1 + acc2._1, acc1._2 + acc2._2));
In this scenario, aggregate
allows you to build a tuple that holds both the sum and the count of items, yielding richer insight from your operation.
3.4 Assuming Identity for Reduce
Many developers make the mistake of assuming that the identity element for reduction is zero, especially when summing values. It's crucial to remember that the identity element depends on the operation you're performing.
For sum, the identity is 0, but if you're conducting a multiplication, the identity would be 1.
3.5 Misunderstanding Data Distribution
Not considering data distribution can significantly impact performance. If a spark job requires a lot of shuffling data between partitions during a reduce step, you may run into performance issues.
Solution
One solution is to ensure your data is partitioned properly, which can enhance performance during reduce operations:
JavaRDD<Integer> rdd = sc.parallelize(Arrays.asList(1, 2, 3, 4, 5), 2); // specify partitions
4. Best Practices for Reduce in Spark RDD
To avoid the pitfalls discussed earlier, follow these best practices:
- Test Associativity and Commutativity: Always check whether your reduce functions can handle varying data orders.
- Null Safety: Always filter nulls for meaningful calculations.
- Choose the Right Operation: Use
aggregate
when you need more than just single output. - Correct Identity Values: Validate the correct identity element for the function you’re using.
- Optimize Partitions: Leverage Spark's partitioning to minimize data shuffle, thus speeding up processing times.
5. Conclusion
Understanding the intricacies of applying reduce operations on RDDs can make the difference between fast, efficient Spark applications and those riddled with errors and poor performance. By familiarizing yourself with common pitfalls and adhering to best practices, you can harness the full potential of Spark for your data processing needs.
For further reading on RDDs, check out the Apache Spark documentation and explore more about the aggregate functions.
Happy coding!