Overcoming Common Obstacles in Test-Driven Development
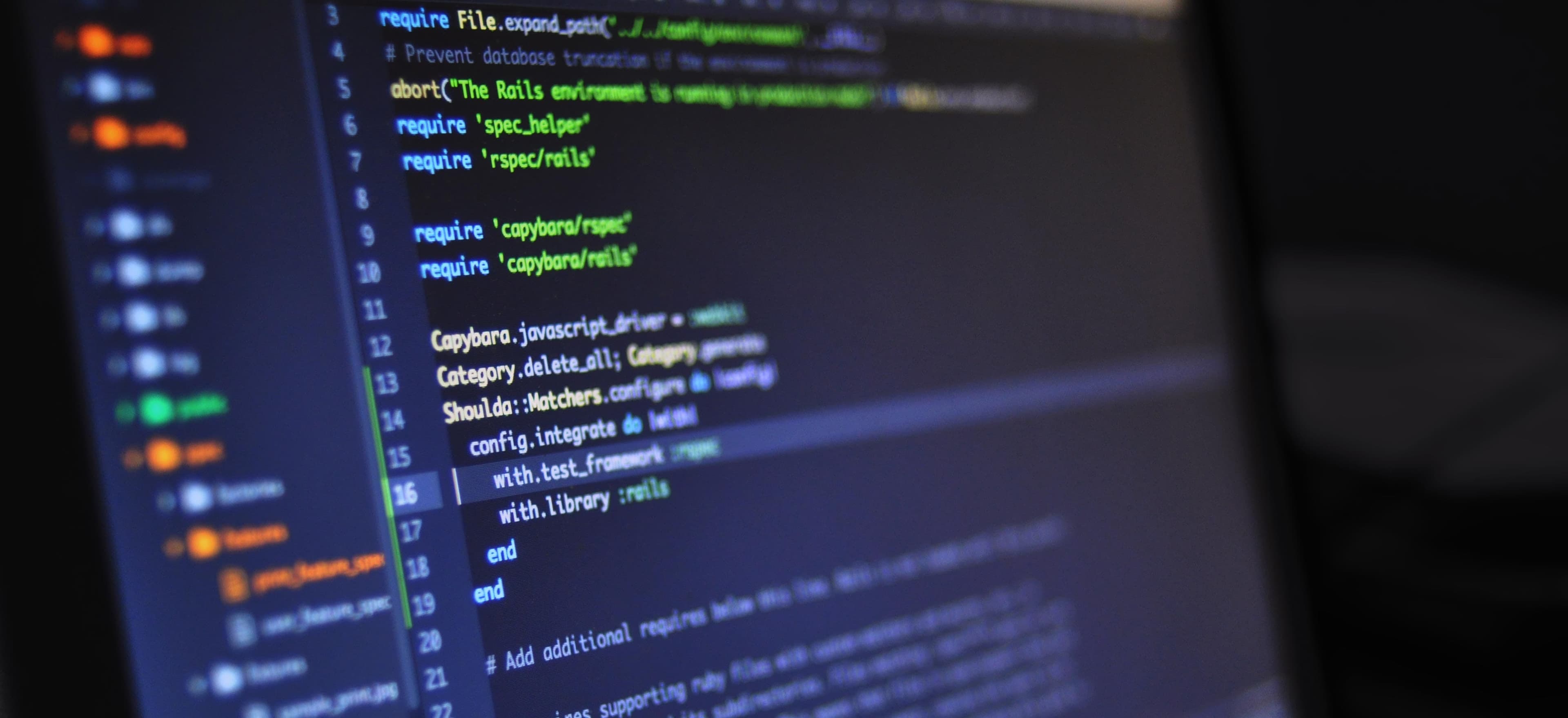
- Published on
Overcoming Common Obstacles in Test-Driven Development
Test-Driven Development (TDD) is a software development methodology that emphasizes writing tests before writing the actual code. This approach can lead to clearer requirements, better-designed software, and an overall higher level of code quality. However, many developers face obstacles while attempting to integrate TDD into their workflows. In this blog post, we will discuss some of the most common challenges of TDD and provide strategies to overcome them. We will also explore Java code examples to solidify these concepts.
What is Test-Driven Development?
Before diving into the common obstacles, it's essential to understand what TDD is and the basic workflow it entails. TDD follows a cycle commonly referred to as the “Red-Green-Refactor” cycle:
- Red: Write a failing test based on the requirements.
- Green: Write the minimum code necessary to pass the test.
- Refactor: Clean up the code while ensuring that the tests still pass.
Using TDD encourages developers to consider the requirements and design upfront, leading to fewer bugs and improved code maintainability.
Common Obstacles and Solutions
1. Lack of Understanding of TDD Principles
Obstacle: Many developers may not fully grasp the principles of TDD or how it differs from traditional development methodologies. This lack of understanding can lead to frustration and improper implementation.
Solution: Educational resources and training can help bridge this knowledge gap. Workshops, online courses, and reading materials about TDD fundamentals can provide the necessary understanding.
Example: Let's consider a simple Java class for managing a bank account. Before writing any code, we should write tests checking for expected behaviors.
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.*;
public class BankAccountTest {
@Test
public void testDeposit() {
BankAccount account = new BankAccount();
account.deposit(100);
assertEquals(100, account.getBalance());
}
}
In this snippet, we begin by creating a failing test to define our expectations for a deposit method. This aligns with TDD principles, guiding our coding process.
2. Inadequate Test Coverage
Obstacle: Developers may struggle with writing comprehensive tests. This often leads to critical areas of the code remaining untested.
Solution: Utilize tools for code coverage analysis, and establish guidelines for acceptable test coverage percentages. Encourage writing tests for edge cases to ensure robustness.
Example: Building on the previous bank account example, let's write a test for withdrawals:
@Test
public void testWithdraw() {
BankAccount account = new BankAccount();
account.deposit(200);
account.withdraw(150);
assertEquals(50, account.getBalance());
}
By expanding our test suite to include withdrawal functionality, we enhance the robustness of our tests and ensure that we consider various scenarios.
3. Resistance to Change
Obstacle: Team members may be accustomed to traditional methods, making them resistant to adopting TDD. They may perceive it as disruptive or unnecessary.
Solution: Promote the long-term benefits of TDD, including reduced debugging time and increased confidence in code changes. Share success stories from other teams or projects that successfully implemented TDD.
4. Time Constraints
Obstacle: Developers often feel pressured to deliver features quickly, and TDD can seem time-consuming compared to coding without tests.
Solution: Emphasize the efficiency of TDD in the long run. While upfront time investment is required, it typically leads to faster debugging and maintenance, which ultimately saves time.
Example: Consider a scenario where we need to implement a method that calculates early withdrawal penalties from a bank account. The test-driven approach could look like this:
@Test
public void testEarlyWithdrawalPenalty() {
BankAccount account = new BankAccount();
account.deposit(1000);
assertThrows(IllegalArgumentException.class, () -> {
account.withdraw(500); // Assuming there's a rule that penalizes early withdrawal
});
}
Even though we spent time writing the test first, it forces us to think through the requirements and edge cases (e.g., rules about penalties). This attention to detail can prevent future issues and delays.
5. Poorly Designed Tests
Obstacle: Writing tests that are too complex or coupled with the implementation can make them brittle. When the code changes, these tests might fail for the wrong reasons.
Solution: Follow best practices for writing unit tests. Keep tests isolated, and ensure they are easy to read and maintain. Each test should focus on one specific behavior.
Example: Using mocks can help isolate tests. For example, when testing a service that interacts with a database, we can use mocking frameworks to simulate the database:
@Test
public void testServiceWithMock() {
Database mockDatabase = mock(Database.class);
when(mockDatabase.getAccountBalance(anyInt())).thenReturn(1000);
BankService service = new BankService(mockDatabase);
assertEquals(1000, service.getAccountBalance(1));
}
Here, we created a mock database to test our service, ensuring that our tests focus solely on the service logic without being affected by the database implementation.
Additional Best Practices for TDD
-
Keep Test Code Clean: Maintain the same code quality in your tests as you do in production code. This includes proper naming conventions and avoiding code duplication.
-
Continuously Refactor: Regularly revisit and refactor both your production and test code as you gain a better understanding of the requirements and the design.
-
Run Tests Frequently: Make it a habit to run your tests frequently—ideally, before every commit. This constant feedback loop helps catch bugs early and reinforces the TDD methodology.
-
Involve the Team: Encourage all team members to be involved in the TDD process. This fosters a culture of collaboration and knowledge sharing.
-
Leverage Automation Tools: Utilize tools like Jenkins, Travis CI, or GitHub Actions for continuous integration to automate running tests. This can further streamline your DevOps pipeline and catch errors as they arise.
Closing Remarks
Integrating Test-Driven Development into your Java projects can greatly enhance code quality and reduce technical debt. While the road to TDD may present some challenges—including misunderstandings, time constraints, and resistance to change—there are practical strategies to overcome these obstacles. By staying committed to writing thorough tests, understanding the principles of TDD, and embracing best practices, you can cultivate a development environment that prioritizes quality and maintainability.
If you're interested in learning more about TDD and best practices, consider checking the Test-Driven Development Overview for more in-depth coverage on the topic and JUnit for the framework that aids in writing tests.
By following the TDD approach, you'll not only improve your coding skills but also contribute to delivering reliable and maintainable software.
This blog post aims to educate and empower developers at any stage in their journey towards mastering TDD. Implementing these strategies will not only enhance your code quality but foster an environment of continuous improvement and learning. Happy coding!
Checkout our other articles