Avoiding Communication Breakdowns in Pair Programming
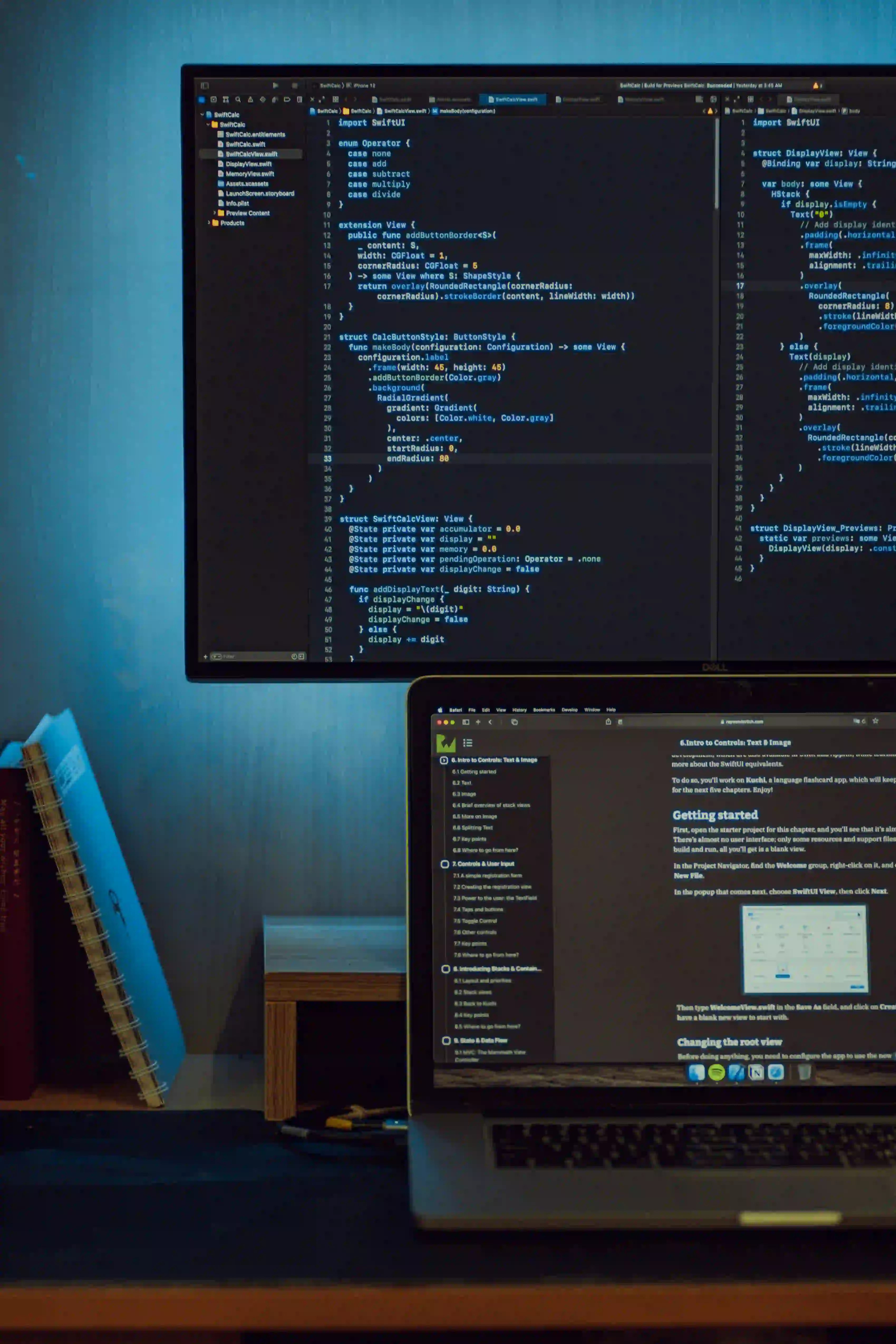
Avoiding Communication Breakdowns in Pair Programming
Pair programming is a powerful collaboration technique in software development that allows two programmers to work together at a single workstation. It fosters shared knowledge, immediate feedback, and ultimately leads to better software. However, effective communication is vital. In this blog post, we'll explore methods to avoid communication breakdowns in pair programming, enhancing both productivity and the quality of the code produced.
What is Pair Programming?
Pair programming involves two roles: the Driver and the Navigator.
- Driver: The person who actively writes the code. They are responsible for translating ideas into actual software.
- Navigator: The individual who reviews the work of the Driver, suggests improvements, and future directions.
This arrangement leads to a constant exchange of ideas and perspectives, enhancing creativity and reducing the chance for errors.
Why Communication Breakdowns Happen
Communication breakdowns in pair programming can arise from various sources:
- Different Communication Styles: Programmers have different ways of expressing ideas or thoughts. These differences can lead to misunderstandings.
- Stress or Time Pressure: In high-pressure environments, communication can often be terse or defensive.
- Lack of Clarity: If the objectives or the task at hand are not clearly defined, it can result in both team members pulling in different directions.
- Technical Jargon: Using specialized terms without ensuring mutual understanding can leave one partner feeling alienated.
Framework for Effective Communication
To enhance communication in pair programming, consider the following strategies:
- Establish Clear Goals and Roles
- Engage in Active Listening
- Use Collaborative Tools
- Foster a Non-judgmental Environment
- Agree on Terminology
- Regular Check-ins
- Practice Empathy
Establish Clear Goals and Roles
Before diving into the code, both programmers should clarify their goals for the session. Make sure to articulate what you hope to achieve clearly. Setting expectations can eliminate confusion later.
Code Example
Here is a simple Java method as a demonstration to solidify this concept:
public int add(int a, int b) {
return a + b;
}
Why This Matters: When you both understand that your goal is to create an add
method that processes input properly, you're better equipped to collaborate. If one programmer thinks they are writing a complex mathematical function while the other thinks they are just implementing simple addition, you’ll have confusion.
Goals in Code Development
- Make clear the expected outcome.
- Discuss testing and performance requirements.
Engage in Active Listening
Active listening involves being fully present in the conversation and responding thoughtfully. Often, in technical environments, one partner might focus so much on coding that they miss crucial points.
Code Review Example
public void greetUser(String username) {
System.out.println("Hello, " + username);
}
Discussion: The Navigator might suggest this code needs more input validation. Rather than saying, "That won't work," the Navigator should focus on why it needs improvement: "Let's ensure we check if the username is empty to avoid runtime errors."
Use Collaborative Tools
In remote pair programming situations, tools such as Visual Studio Code Live Share or JetBrains Code With Me allow both programmers to interact with the codebase effectively.
Benefits of Collaborative Tools
- Real-time Editing: Both programmers can see changes as they're made.
- Shared Debugging Sessions: When errors come up, they can troubleshoot them together.
For a more in-depth look into setting up collaborative tools, you might want to check out Visual Studio Code Live Share.
Foster a Non-judgmental Environment
Encouraging open feedback is essential. Programmers should feel safe sharing their thoughts without fear of dismissal or ridicule. This openness invites more creative ideas and solutions.
Example of Encouraging Feedback
When discussing a piece of code, initiate discussions like:
What do you think about this approach?
I’m curious to hear your thoughts before we finalize it.
Instead of:
This doesn’t make sense. Change it.
Why This Matters: Communicating constructively ensures both parties feel respected and valued, which is crucial for a collaborative environment.
Agree on Terminology
Technical language can sometimes create barriers. Spend a few minutes at the outset of a session agreeing on specific terminology related to the project, especially if new technologies or methodologies are involved.
Example
Before discussing a Refactor, you might define it as:
- What is refactoring?: "The process of restructuring existing code without changing its external behavior."
This clarifies what you mean and ensures both programmers are on the same page.
Regular Check-ins
As the session progresses, it is vital to have brief pauses to ensure both programmers are aligned. Regular check-ins allow you to refine the direction of development together.
Sample Check-in Questions
- Are we on track to meet our goals?
- Is there anything unclear about the code we've written?
These questions can promote ongoing dialogue that keeps both programmers engaged and aware.
Practice Empathy
Empathy is crucial during pair programming. Understand and respect the other programmer’s experience level and perspective.
Demonstrating Empathy
If the Navigator realizes the Driver struggles with a concept, suggest breaking it down:
// Pseudo-code for breaking down complex logic
if (conditionNotMet) {
System.out.println("Let’s simplify this part.");
}
Why Practice Empathy?: Practicing empathy fosters a sense of partnership, ensuring that both parties feel respected and understood.
The Bottom Line
Effective communication is the backbone of successful pair programming. By focusing on clear goals, active listening, collaborative tools, and promoting a healthy atmosphere of feedback, you will significantly reduce the chances of communication breakdowns.
Pair programming, when executed with effective communication strategies, not only drives better code quality but also strengthens the skillset of both programmers. Embrace the challenges and rewards of this powerful development practice!
For further reading on pair programming's advantages, check out Martin Fowler's blog on Pair Programming.
Call to Action
Are you currently pair programming, or considering it? Join the conversation in the comments below and share your experiences and tips on avoiding communication breakdowns in this technique!