Common API Gateway Pitfalls in Microservices Architecture
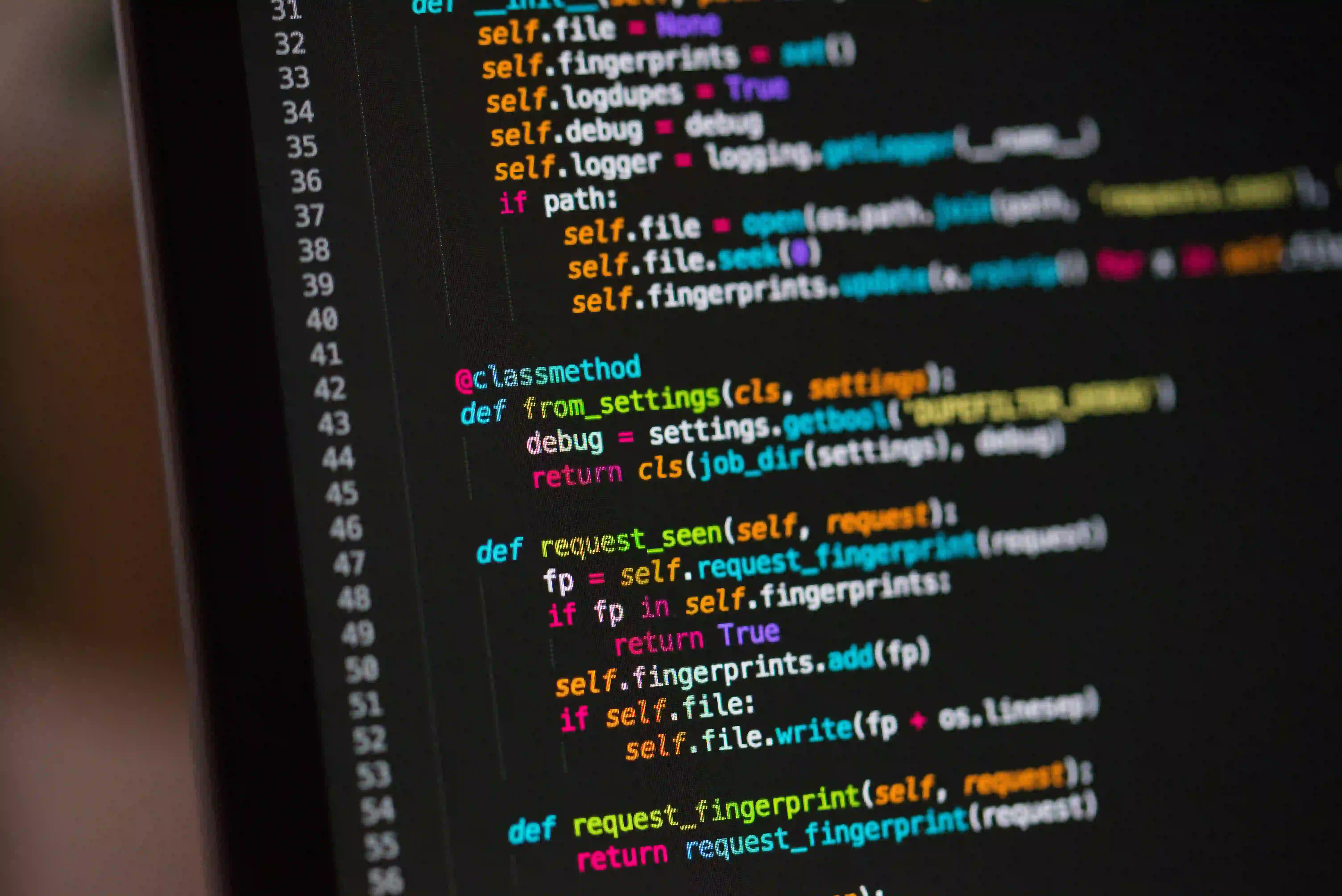
Common API Gateway Pitfalls in Microservices Architecture
In the world of microservices architecture, the API Gateway serves as a critical component that aggregates internal services, facilitates communication, and encapsulates business logic. An effective API Gateway enhances performance, improves security, and provides a single entry point for client applications. However, its importance comes with numerous challenges. In this blog post, we will explore common pitfalls encountered when implementing an API Gateway in microservices architecture and advise on how to avoid these issues.
Understanding the Role of an API Gateway
Before delving into the pitfalls, it's vital to grasp what an API Gateway does. Think of it as a traffic control system that directs requests from clients to the appropriate microservice endpoints. Some of its responsibilities include:
- Request routing
- Request aggregation
- Rate limiting
- Authentication and authorization
- Response transformation
To get a deeper understanding of how microservices interact with API Gateways, consider reviewing this resource on microservices architecture.
1. Overloading the API Gateway
One significant pitfall is overloading the API Gateway with too many responsibilities. While it can handle routing and security, it's not designed to manage business logic extensively. Packing it with numerous functions can lead to:
- Performance bottlenecks
- Increased latency
- Complexity in maintenance
Solution
Keep the API Gateway focused on its core functionalities. Use separate components or services for tasks like caching, complex orchestration, or heavy analytics. For instance, offloading authorization checks to a dedicated service can significantly enhance response times.
Code Example
@RestController
@RequestMapping("/api")
public class GatewayController {
@GetMapping("/users/{id}")
@PreAuthorize("hasRole('USER')")
public ResponseEntity<User> getUser(@PathVariable String id) {
// Here we just route the request without heavy logic
User user = userService.fetchUserById(id);
return ResponseEntity.ok(user);
}
}
In the example above, the @PreAuthorize
annotation ensures that authorization logic is handled through Spring Security, keeping the Gateway's responsibilities minimal.
2. Ignoring Versioning and Backward Compatibility
APIs evolve over time. Ignoring versioning can lead to breaking changes that affect clients relying on the current API version. If clients cannot access their required services due to compatibility issues, it can severely impact user experience.
Solution
Implement API versioning right from the beginning. Use versioning strategies like URI versioning, query parameters, or header versioning.
URI Versioning Example
@GetMapping("/v1/users/{id}")
public ResponseEntity<User> getUserV1(@PathVariable String id) {
// Logic for version 1
}
@GetMapping("/v2/users/{id}")
public ResponseEntity<UserV2> getUserV2(@PathVariable String id) {
// Logic for version 2 with new features
}
By versioning your APIs, you ensure that clients can gradually adapt to changes in a managed fashion. This fosters healthy, long-term relationships with your API consumers.
3. Neglecting Security Measures
With APIs facilitating numerous client interactions, neglecting security can expose the microservices to various vulnerabilities. Rate-limiting, authentication, and encryption are often overlooked aspects that can lead to catastrophic failures.
Solution
Deploy multiple layers of security measures. Implement robust authentication methods like OAuth2, along with rate limiting to prevent abuse.
Rate Limiting Implementation Example
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
import org.springframework.http.ResponseEntity;
@RestController
public class RateLimitController {
@GetMapping("/api/resource")
@RateLimit(limit = 10, duration = 60) // Limit to 10 requests per minute
public ResponseEntity<String> getResource() {
return ResponseEntity.ok("Accessed the protected resource!");
}
}
In this code, we've created a rate-limiting decorator to monitor and restrict API usage. This measure significantly reduces the likelihood of abuse.
4. Lack of Monitoring and Logging
In microservices, where multiple instances communicate with one another, failing to monitor API Gateway traffic can lead to blind spots in performance tracking and debugging.
Solution
Integrate application performance monitoring (APM) tools like Prometheus or ELK Stack for log aggregation. These tools will help you maintain visibility into your services.
Logging Example
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
@RestController
public class LogController {
private static final Logger logger = LoggerFactory.getLogger(LogController.class);
@GetMapping("/api/data")
public ResponseEntity<String> getData() {
logger.info("Data requested at {}", LocalDateTime.now());
return ResponseEntity.ok("Here is your data");
}
}
This logging method captures useful information whenever clients access the API, which can be pivotal for diagnosing issues.
5. Poor Performance Management
If not managed properly, the API Gateway can become a performance bottleneck. High latency in processing requests can stem from blocking operations, synchronous calls, or even excessive logging.
Solution
Optimize performance by using asynchronous communication. Consider implementing message queues for handling heavy operations.
Asynchronous Example
import org.springframework.kafka.annotation.KafkaListener;
import org.springframework.kafka.core.KafkaTemplate;
import org.springframework.stereotype.Service;
@Service
public class AsyncService {
private final KafkaTemplate<String, String> kafkaTemplate;
public AsyncService(KafkaTemplate<String, String> kafkaTemplate) {
this.kafkaTemplate = kafkaTemplate;
}
public void sendAsyncMessage(String message) {
kafkaTemplate.send("topic", message);
}
@KafkaListener(topics = "another-topic")
public void listenGroupFoo(String message) {
// Handle incoming messages efficiently
}
}
Utilizing a messaging system like Kafka allows your system to handle operations asynchronously, drastically improving performance.
Bringing It All Together
The API Gateway is a powerful tool in any microservices architecture, but its implementation comes with challenges. By avoiding common pitfalls such as overloading the gateway, neglecting versioning, implementing weak security measures, failing to monitor usage, and mismanaging performance, you can create a robust and scalable microservices system.
Through this blog post, we have emphasized the importance of keeping the API Gateway's role clear, implementing best practices early on, and incorporating tools that enhance performance and security.
For further insights on scaling your microservices architecture, you may find this helpful article on microservice patterns valuable.
By following the guidelines outlined in this post, you'll be well on your way to building a resilient API Gateway capable of supporting your microservices ecosystem effectively.