Mastering Resource Access in Grails: Tips and Tricks
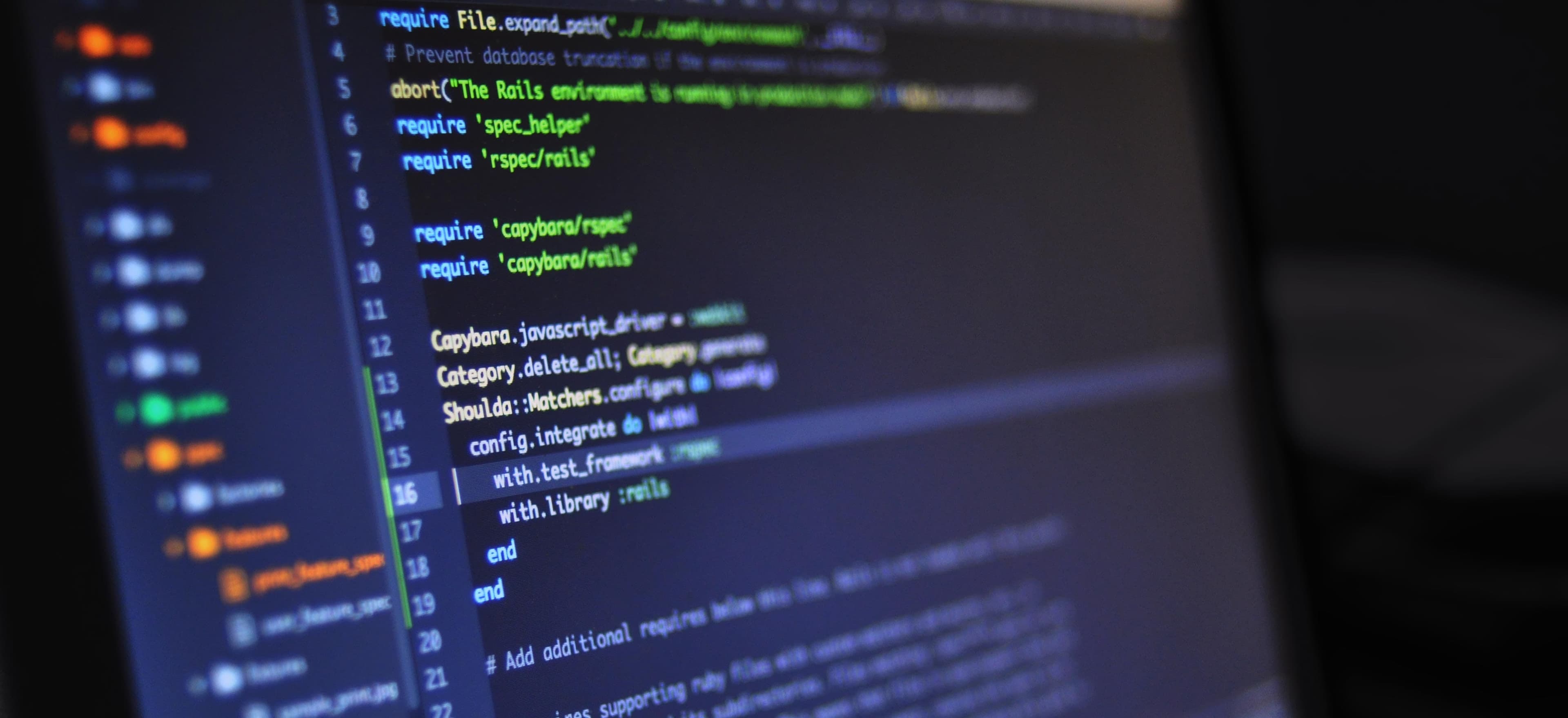
- Published on
Mastering Resource Access in Grails: Tips and Tricks
Grails, the powerful web application framework that is built on top of the Spring framework, allows for rapid development with a focus on convention over configuration. However, like any framework, mastering resource access in Grails requires a good understanding of both the framework itself and its underlying principles.
In this blog post, we will cover the essential tips and tricks to efficiently manage and access resources in Grails applications. This discussion will not only enhance your coding experience but also improve your application’s performance.
Understanding Resource Management in Grails
Grails offers a streamlined way to manage static resources such as JavaScript files, CSS files, images, and more. These resources are typically located in the grails-app/assets
directory, and they can be served directly to clients without any additional configuration.
Grails uses Asset Pipeline, which simplifies the process of managing these resources. It compiles, bundles, and serves the assets to ensure optimal performance while maintaining a clean and organized asset structure.
Key Features of the Asset Pipeline
-
Automatic Compilation: Grails automatically compiles resources stored in the
grails-app/assets
directory, which implies that you do not need to worry about manually compiling your CSS or JavaScript files. -
Minification: The Asset Pipeline minifies code for production, meaning extra spaces, comments, and unnecessary characters are stripped out to reduce file size, thus improving load times.
-
Versioning: Automatically adds a hash to your file names, which assists in cache busting. When you update a resource, the hash changes, prompting the browser to fetch the latest version.
-
Type Support: Supports various file types, including SCSS, Less, TypeScript, and more.
Configuring Resource Access
To access your resources in a Grails application, you can do as follows:
-
JavaScript Files:
- Place your JavaScript files in the
grails-app/assets/javascripts
directory. - Use the
assetPath
tag in your Groovy views to link to your scripts.
<script src="${assetPath('application.js')}" type="text/javascript"></script>
- Place your JavaScript files in the
-
CSS Files:
- Store your CSS files in
grails-app/assets/stylesheets
. - Similar to JavaScript, link them using the
assetPath
tag.
<link rel="stylesheet" href="${assetPath('styles.css')}" />
- Store your CSS files in
-
Images:
- Images can be placed in
grails-app/assets/images
. - Use the
assetPath
to reference them in your views.
<img src="${assetPath('logo.png')}" alt="Logo" />
- Images can be placed in
Why Use Asset Pipeline?
Using the asset pipeline is crucial for maintaining an efficient codebase. Not only does it allow for easy organization, but it also optimizes resource loading times. This can significantly affect the performance of your application.
Advanced Configuration Tips
While the basic usage of resource access is straightforward, there are advanced configurations that can enhance your Grails application.
Custom Asset Directories
You can configure additional asset directories by modifying the Asset Pipeline
configuration. This can be particularly useful for larger projects that require a more complex directory structure.
Example of Custom Configuration
In grails-app/conf/application.groovy
, you can add custom directories as follows:
grails.assets {
// Custom image directory
imageDir = 'assets/images'
// Custom css directory
cssDir = 'assets/css'
// Custom js directory
jsDir = 'assets/js'
}
Lazy Loading of Assets
For very large applications, consider lazy loading your assets. Lazy loading ensures that resources are loaded only when required, improving initial page load speed.
You can achieve this by using asynchronous JavaScript loading libraries, which allows certain scripts to load after the main content is rendered.
Cache Control
Use cache control headers to manage browser caching of your assets. Proper cache management can greatly improve load times for repeated visitors.
Sample Configuration
grails.assets {
cache = {
enabled = true
duration = 31536000 // Cache for a year
}
}
Best Practices for Resource Management
To further enhance resource management in your Grails application, consider the following best practices:
-
Organize Files Logically: Group related resources together. For example, all components of a specific feature should reside in the same subdirectory.
-
Clear Redundant Files: Regularly audit and clean up your assets. Remove any unused files to avoid unnecessarily bloating your application.
-
Optimize Images: Always optimize your images for the web before adding them to your assets. Tools like TinyPNG can significantly decrease image size without a loss in quality.
-
Use CDN: For larger applications, consider serving static assets via a Content Delivery Network (CDN) to decrease load times globally.
Debugging Resources
Occasionally, resource loading issues may occur. Debugging can be done through various methods:
-
Browser Developer Tools: Use the built-in developer tools to monitor network activity and ensure that resources are loading correctly.
-
Log Statements: Add log statements in the
Grails
UrlMappings
or controllers to check if the routes for your resources are correctly defined. -
Fallbacks: Define fallback resources in your application to serve in case the primary resources fail.
To Wrap Things Up
Understanding and mastering resource access in Grails is vital for creating efficient and performant web applications. From leveraging the asset pipeline to implementing advanced configurations, these tips and tricks will help you streamline your resource management efforts.
By keeping best practices in mind and staying updated with the latest features of Grails, you can ensure that your applications are not only functional but also optimized for speed and user experience.
For further reading on Grails and resource management, check out the official Grails documentation and the Asset Pipeline documentation. Embrace these practices and watch your Grails applications thrive!
Checkout our other articles