Mastering Java 7: Common File Filtering Mistakes to Avoid
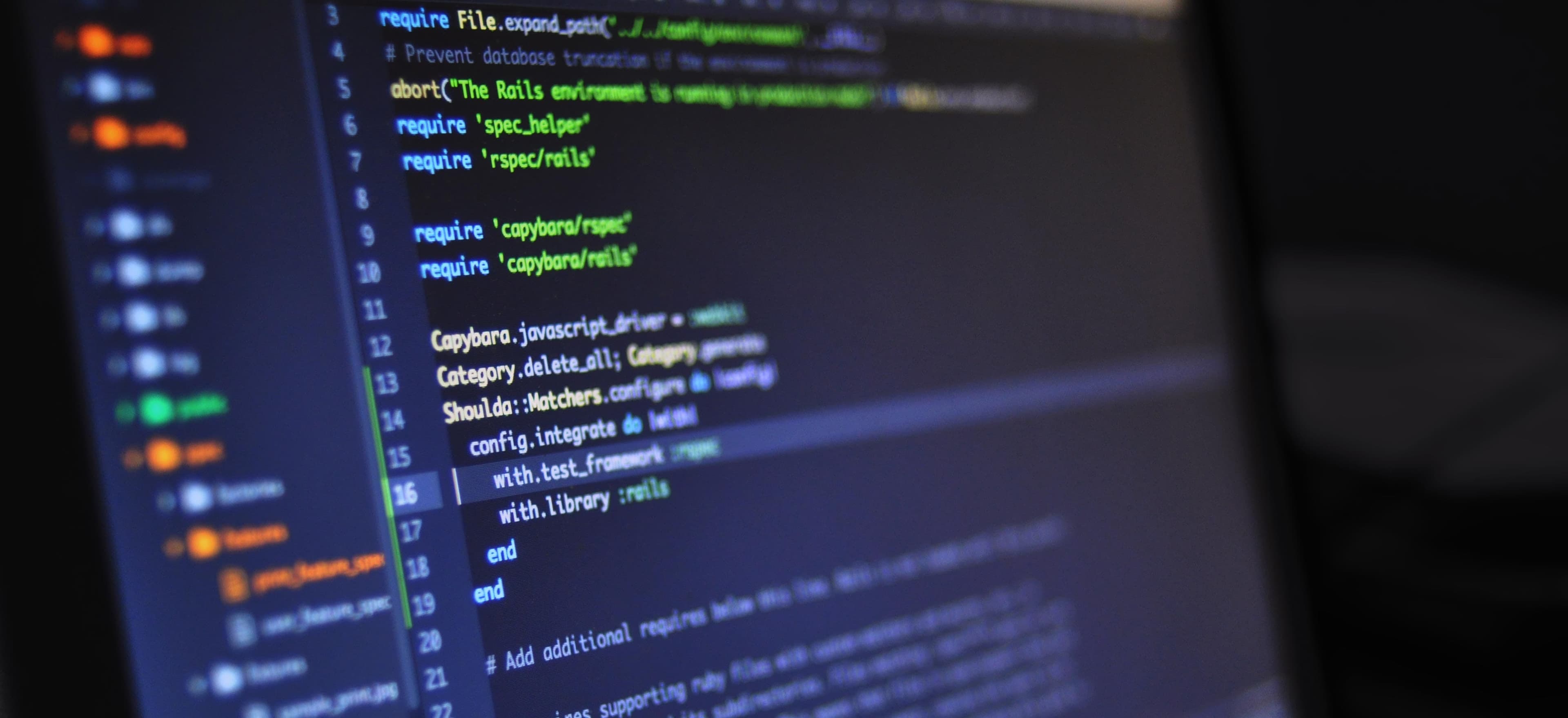
- Published on
Mastering Java 7: Common File Filtering Mistakes to Avoid
Java 7 introduced some robust features to the Java ecosystem, especially with the new I/O package, java.nio.file
. With enhancements like the Files
class, file filtering became more streamlined. However, even seasoned developers can make mistakes when writing file filtering code. In this blog post, we will explore common mistakes to avoid when performing file filtering in Java 7, providing tips and code snippets to guide you.
Understanding Java's File Filter Mechanism
Before we delve into the pitfalls of file filtering, it is essential to clarify how file filtering operates in Java 7. The Files
class contains several methods for file handling, including filtering capabilities through DirectoryStream
and PathMatcher
.
For example, using DirectoryStream
, you can filter files in a directory based on their names and types. Here's a quick snippet to illustrate this:
import java.io.IOException;
import java.nio.file.DirectoryStream;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
public class FileFilterExample {
public static void main(String[] args) {
Path dir = Paths.get("your/directory/path");
try (DirectoryStream<Path> stream = Files.newDirectoryStream(dir, "*.txt")) {
for (Path entry : stream) {
System.out.println(entry.getFileName());
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
Explanation
In this example, we filter all .txt files within a specified directory. The Files.newDirectoryStream
method takes a path and a globbing pattern (in this case, "*.txt"), allowing us to retrieve all text files.
Mistake 1: Ignoring IOException
One of the fundamental principles of Java is its strict handling of exceptions. Many developers hastily write file filtering code without properly managing IOException
. This can lead to unexpected software behavior. The snippet provided earlier demonstrates a try-with-resources
block, ensuring that resources are appropriately closed.
Best Practice
Always catch exceptions and consider logging them to understand where things went wrong. Utilize try-with-resources
to automatically close streams.
try {
// your file filter code
} catch (IOException e) {
System.err.println("Error occurred: " + e.getMessage());
}
Mistake 2: Overlooking Hidden Files
Developers often forget that files can be hidden depending on the operating system. Windows and UNIX/Linux systems allow file designation as hidden, and filtering by *.txt
might overlook crucial files.
Solution
To exclude or include hidden files, you might consider implementing a custom FileVisitor
or simply using a path filter:
Files.walk(Paths.get("your/directory/path"))
.filter(path -> !Files.isHidden(path) && path.toString().endsWith(".txt"))
.forEach(System.out::println);
Explanation
In this example, Files.walk()
is used to traverse the directory structure, applying a filter that checks if a file is hidden. This way, you ensure comprehensive filtering.
Mistake 3: Inefficient Filtering Methods
Some developers resort to applying filters individually, leading to inefficient execution. Filtering files in a loop incurs a performance cost, especially with large directories.
Optimized Filtering
Instead of applying multiple filters in succession, use composite filtering techniques. The following snippet uses a predicate:
Files.list(Paths.get("your/directory/path"))
.filter(path -> !Files.isHidden(path) && path.toString().endsWith(".txt"))
.forEach(System.out::println);
Explanation
This code snippet filters visible .txt files efficiently in a single operation, reducing overhead and enhancing performance.
Mistake 4: Not Familiarizing with PathMatcher
Java 7 introduced the PathMatcher
interface as a powerful tool for matching file paths more flexibly than relying solely on glob expressions. However, it remains underutilized.
Example
import java.nio.file.FileSystems;
import java.nio.file.PathMatcher;
import java.nio.file.Files;
import java.nio.file.Paths;
public class PathMatcherExample {
public static void main(String[] args) {
PathMatcher matcher = FileSystems.getDefault().getPathMatcher("glob:**/*.txt");
try {
Files.list(Paths.get("your/directory/path"))
.filter(matcher::matches)
.forEach(System.out::println);
} catch (IOException e) {
e.printStackTrace();
}
}
}
Explanation
The PathMatcher
evaluates if each file matches the specified glob pattern, enabling you to define sophisticated file-matching rules, such as ignoring directory hierarchies or types.
Mistake 5: Failing to Test Edge Cases
Another common issue arises from the lack of testing for edge cases. For instance, if a directory is empty or has restricted permissions, your program should gracefully handle these scenarios.
Recommended Approach
Always test your filtering logic with various datasets including:
- Empty directories.
- Directories without read permissions.
- Edge cases where files do not match your filters.
In such conditions, you may notice how your application behaves.
try {
Files.list(Paths.get("empty_directory"))
.filter(path -> path.toString().endsWith(".txt"))
.forEach(System.out::println);
} catch (NoSuchFileException e) {
System.err.println("The directory does not exist: " + e.getMessage());
} catch (IOException e) {
System.err.println("I/O error occurred: " + e.getMessage());
}
My Closing Thoughts on the Matter
File filtering in Java 7 has made significant strides, offering developers convenient classes and interfaces. However, common mistakes can lead to inefficient code and application failure.
By understanding and avoiding these pitfalls—ensuring proper exception handling, being aware of hidden files, optimizing filtering methods, employing PathMatcher
, and testing edge cases—you will improve your application’s robustness and performance.
For a deeper dive into Java I/O concepts, check out the official Java documentation. Additionally, you can further explore file handling with the Java 7 New Features curated by Oracle.
Start implementing these best practices today, and master file filtering in Java 7! Happy coding!
Checkout our other articles