Mastering Command Pattern with Java 8 Lambda Expressions
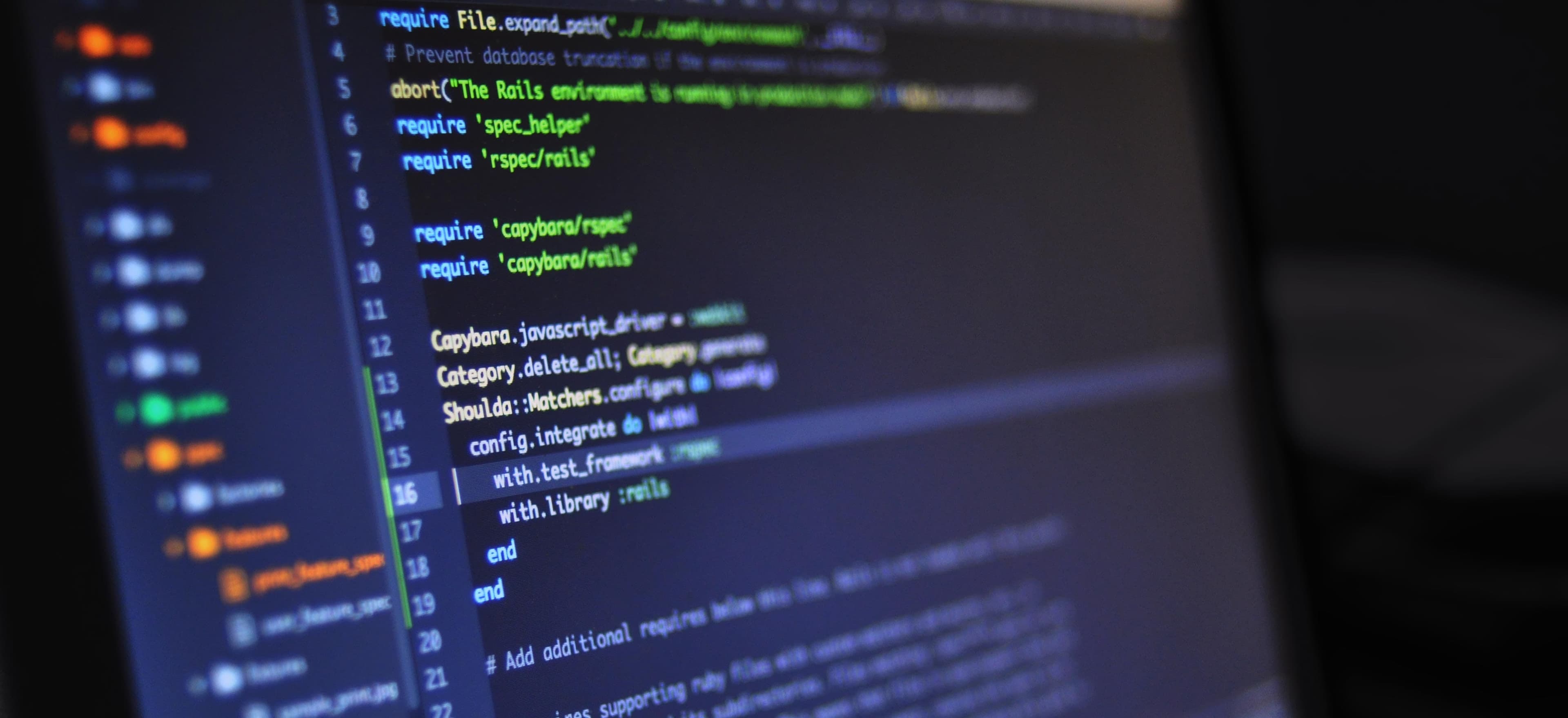
- Published on
Mastering the Command Pattern with Java 8 Lambda Expressions
The Command Pattern is a behavioral design pattern that encapsulates a request as an object, thereby allowing you to parameterize clients with different requests, queue requests, and log the requests. With the introduction of Java 8 and its lambda expressions, implementing the Command Pattern has become more succinct and intuitive. In this blog post, we'll explore the Command Pattern, how to implement it using traditional classes, and then streamline our approach using Java 8's lambda expressions.
Understanding the Command Pattern
Before diving into code, let’s take a moment to dissect the Command Pattern. This design pattern follows a few essential components:
- Command Interface: This defines a simple method (e.g.,
execute()
). - Concrete Command: This implements the Command interface, carrying out the actual request by invoking methods on the receiver.
- Receiver: The object that carries out the action when the command is executed.
- Invoker: The object that holds the commands and triggers their execution.
Why Use the Command Pattern?
- Decoupling: The invoker is separated from the receiver.
- Undo/Redo Operations: You can easily implement undo/redo functionality by keeping a history of commands.
- Complex Command Sequences: Commands can be queued, logged, or combined to create more complex operations.
Traditional Implementation of the Command Pattern
Let’s illustrate the Command Pattern using a simple example: an electronic devices control system that can turn on and off.
Step 1: Command Interface
public interface Command {
void execute();
}
Step 2: Receiver
public class Light {
public void turnOn() {
System.out.println("The light is ON");
}
public void turnOff() {
System.out.println("The light is OFF");
}
}
Step 3: Concrete Commands
public class LightOnCommand implements Command {
private Light light;
public LightOnCommand(Light light) {
this.light = light;
}
@Override
public void execute() {
light.turnOn();
}
}
public class LightOffCommand implements Command {
private Light light;
public LightOffCommand(Light light) {
this.light = light;
}
@Override
public void execute() {
light.turnOff();
}
}
Step 4: Invoker
public class RemoteControl {
private Command command;
public void setCommand(Command command) {
this.command = command;
}
public void pressButton() {
command.execute();
}
}
Step 5: Client Code
public class Client {
public static void main(String[] args) {
Light livingRoomLight = new Light();
Command lightsOn = new LightOnCommand(livingRoomLight);
Command lightsOff = new LightOffCommand(livingRoomLight);
RemoteControl remote = new RemoteControl();
remote.setCommand(lightsOn);
remote.pressButton(); // Output: The light is ON
remote.setCommand(lightsOff);
remote.pressButton(); // Output: The light is OFF
}
}
Streamlining with Java 8 Lambda Expressions
Now that we've seen the conventional approach, let’s explore how we can implement the Command Pattern using lambda expressions in Java 8. Lambdas provide a cleaner and more readable way to implement functional interfaces.
Step 1: The Command Interface
The Command
interface remains the same:
public interface Command {
void execute();
}
Step 2: Using Lambda Expressions to Define Commands
We can directly use lambda expressions to create instances of Command
without needing to create explicit classes for each command.
public class Client {
public static void main(String[] args) {
Light livingRoomLight = new Light();
// Using lambda expressions for command implementation
Command lightsOn = () -> livingRoomLight.turnOn();
Command lightsOff = () -> livingRoomLight.turnOff();
RemoteControl remote = new RemoteControl();
remote.setCommand(lightsOn);
remote.pressButton(); // Output: The light is ON
remote.setCommand(lightsOff);
remote.pressButton(); // Output: The light is OFF
}
}
Benefits of Using Lambda Expressions
- Conciseness: Reduces the boilerplate code of creating new classes.
- Readability: Makes the code easier to read and understand.
- Flexibility: Allows for more dynamic command definitions on-the-fly.
Example with Undo Functionality
Let’s extend our example to implement undo functionality using the command pattern with lambda expressions. This can be done by maintaining a history of commands executed.
import java.util.Stack;
public class EnhancedRemoteControl {
private Command command;
private Stack<Command> commandHistory = new Stack<>();
public void setCommand(Command command) {
this.command = command;
}
public void pressButton() {
command.execute();
commandHistory.push(command);
}
public void undoButton() {
if (!commandHistory.isEmpty()) {
commandHistory.pop();
System.out.println("Last command undone");
} else {
System.out.println("No commands to undo");
}
}
}
Client Code with Undo
public class Client {
public static void main(String[] args) {
Light livingRoomLight = new Light();
EnhancedRemoteControl remote = new EnhancedRemoteControl();
Command lightsOn = () -> livingRoomLight.turnOn();
Command lightsOff = () -> livingRoomLight.turnOff();
remote.setCommand(lightsOn);
remote.pressButton(); // Output: The light is ON
remote.undoButton(); // Output: Last command undone
remote.setCommand(lightsOff);
remote.pressButton(); // Output: The light is OFF
remote.undoButton(); // Output: Last command undone
}
}
Wrapping Up
The Command Pattern is a powerful design pattern that enables you to encapsulate requests as objects and decouple invokers from receivers. With Java 8's lambda expressions, implementing this pattern has become more straightforward and expressive. You can quickly define commands inline and keep your code clean and maintainable.
For a deeper dive into design patterns, check out the Design Patterns in Java resource. Whether you're developing a small application or a large enterprise system, mastering such patterns is invaluable.
Implementing the Command Pattern with lambda expressions not only enhances readability but also embraces modern Java programming practices. Experiment with variations of this pattern in your applications, and you'll likely discover more use cases and benefits. Happy coding!