Overcoming Redis Pub/Sub Message Loss in Spring Applications
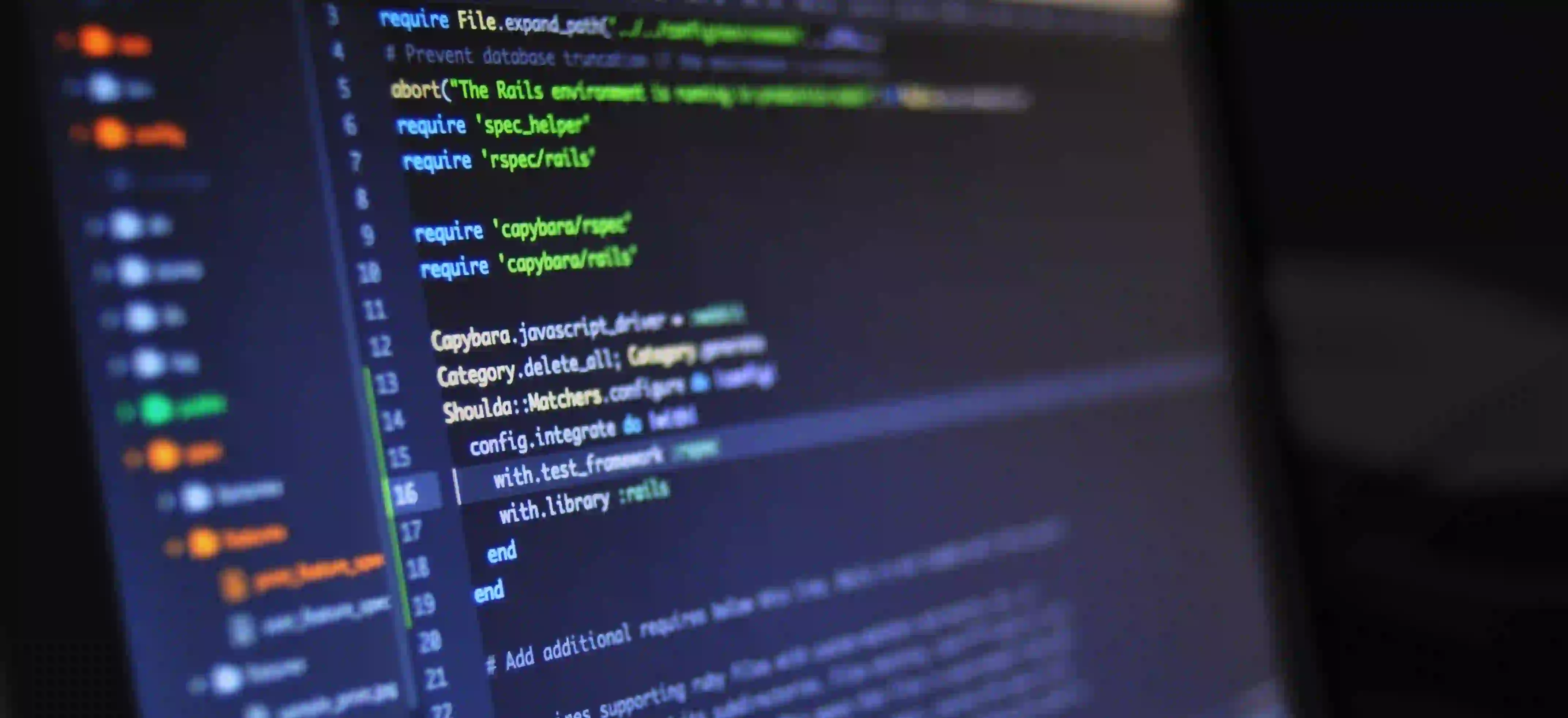
Overcoming Redis Pub/Sub Message Loss in Spring Applications
Redis is an incredibly fast, in-memory data structure store often used as a database, cache, and message broker. It provides a lightweight way for microservices to communicate through its Publish/Subscribe (Pub/Sub) messaging paradigm. However, as efficient as Redis Pub/Sub can be, it comes with its own set of challenges - one of which is message loss.
In this blog post, we'll explore how to mitigate message loss in Redis Pub/Sub when integrating it into a Spring application. By the end of this post, you'll have a clear understanding of the main issues surrounding Redis Pub/Sub, strategies to handle potential message loss, and code snippets that showcase best practices.
Understanding Redis Pub/Sub
In Redis, Pub/Sub is a messaging paradigm where a sender (publisher) pushes messages to one or more receivers (subscribers). Subscribers listen to channels and receive messages pushed to those channels. The beauty of this model is speed and simplicity.
However, the downside is that if a subscriber is not connected at the moment a message is published, it will lose that message. This issue of message loss can be critical in applications where data consistency and reliability are paramount.
Why Does Message Loss Happen?
In a typical scenario, a Redis Pub/Sub message could be lost due to various reasons:
- Subscriber Offline: If a subscriber disconnects and a message is published, that message is lost.
- Network Interruptions: Network issues can cause subscribers to miss messages.
- Redis Server Overload: Under heavy load, Redis can drop messages from the Pub/Sub queue.
These factors highlight the importance of implementing strategies to ensure message delivery is no longer an Achilles' heel in systems reliant on Redis Pub/Sub.
Strategies for Mitigating Message Loss
1. Acknowledged Delivery with a Queue
One effective way to ensure that messages are not lost is to introduce a secondary data store (like a Redis list or sorted set) to act as a queue for messages. Imagine a publisher that writes to both a Redis Pub/Sub channel and a Redis list thereby keeping a copy of the message. Subscribers can read from this list upon reconnection.
Code Example
Let's take a look at a simple setup using Spring's RedisTemplate:
@Service
public class MessagingService {
private final RedisTemplate<String, String> redisTemplate;
private final String PUB_SUB_CHANNEL = "my_channel";
private final String MESSAGE_QUEUE = "message_queue";
@Autowired
public MessagingService(RedisTemplate<String, String> redisTemplate) {
this.redisTemplate = redisTemplate;
}
public void publishMessage(String message) {
// Push the message to a list for reliable storage
redisTemplate.opsForList().rightPush(MESSAGE_QUEUE, message);
// Publish the message to the Pub/Sub channel
redisTemplate.convertAndSend(PUB_SUB_CHANNEL, message);
}
}
Why This Approach?
By storing each message in a list before publishing, you ensure that no message goes missing. Subscribers can check the list upon reconnection and process unacknowledged messages.
2. Message Acknowledgement from Subscribers
An effective pattern to ensure reliability is to require subscribers to acknowledge the receipt of messages. This way, if an acknowledgment is not received, you can retry delivering the message.
Code Example
The subscriber can send an acknowledgment back to a dedicated channel:
@Component
public class Subscriber {
@Autowired
private RedisTemplate<String, String> redisTemplate;
@PostConstruct
public void subscribe() {
redisTemplate.convertAndSend("my_channel", new MessageListener() {
@Override
public void onMessage(Message message, byte[] pattern) {
// Process the message
String msg = message.toString();
boolean success = processMessage(msg);
// Acknowledge or handle failure
if (success) {
redisTemplate.convertAndSend("ack_channel", msg + " acknowledged");
} else {
redisTemplate.convertAndSend("retry_channel", msg); // for retry
}
}
});
}
public boolean processMessage(String message) {
// Process your message here
return true; // return success or failure
}
}
Why Acknowledge Messages?
By implementing a simple acknowledgment mechanism, you ensure that your application can decide how to handle unsuccessful message processing. It also allows for a more robust error handling mechanism, making your messaging system much more resilient.
3. Using a Message Broker
While Redis is a powerful tool, it might not always be the best fit for scenarios that require guaranteed message delivery. Tools like Apache Kafka or RabbitMQ provide built-in support for persistent messaging queues, which ensures that messages are retained until they can be successfully consumed.
Exploring Alternatives
If you're facing persistent issues with Redis Pub/Sub regarding message loss, consider integrating with a dedicated message broker. For more information on when to use which, you can check out this guide to RabbitMQ with Spring Boot.
4. Graceful Handling of Disconnections
By implementing a mechanism that detects disconnections and managing reconnections, you can help ensure that subscribers are always prepared to rebalance:
public void subscribeWithReconnectionHandling(String channel) {
while (true) {
try {
// Attempt to subscribe
redisTemplate.getConnectionFactory().getConnection().subscribe(new MessageListener() {
@Override
public void onMessage(Message message, byte[] pattern) {
// Handle the message
}
}, channel.getBytes());
break; // Exit loop when successful
} catch (Exception e) {
System.err.println("Failed to subscribe, retrying in 5 seconds");
try {
Thread.sleep(5000); // wait before retrying
} catch (InterruptedException ie) {
Thread.currentThread().interrupt();
}
}
}
}
Why Graceful Handling?
This code showcases an effective reconnection strategy. By waiting and retrying, you minimize disruption when a subscriber unexpectedly disconnects.
5. Configuration and Monitoring
Lastly, ensuring that your Redis configuration is optimized for the needs of your application can go a long way in mitigating message loss. Set appropriate values for parameters like maxmemory-policy
and monitor your Redis instance for performance metrics.
Furthermore, integrating application-level logging and health checks can help preempt potential issues. The Spring Boot Actuator can be particularly beneficial for monitoring.
The Last Word
While Redis Pub/Sub is a useful component in a Spring application, it inherently exposes your system to message loss challenges. By implementing strategies such as acknowledged delivery, message queuing, and robust error handling, you can significantly reduce the likelihood of losing critical messages.
If you're encountering persistent issues with Redis Pub/Sub, consider exploring alternative messaging systems tailored to your specific workload requirements, like RabbitMQ or Apache Kafka.
By practicing good coding habits, implementing robust error handling, and constantly monitoring system performance, you can create a reliable communication pipeline in your Spring applications.
Feel free to share your experiences and any additional insights you have into mitigating message loss in Redis Pub/Sub applications in the comments below!