Unlocking Complex Logic: The Interpreter Pattern Explained
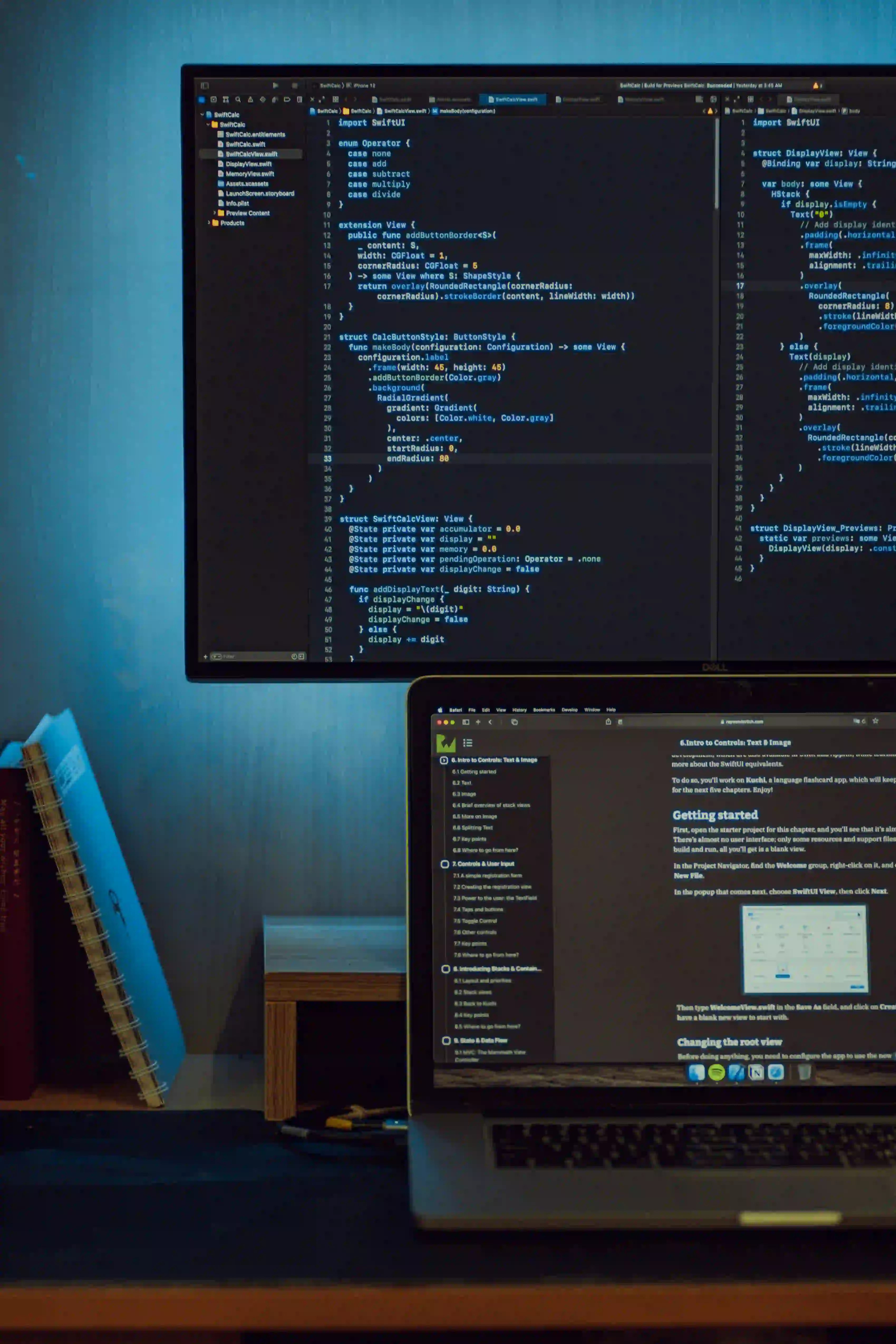
Unlocking Complex Logic: The Interpreter Pattern Explained
The Interpreter Pattern is one of the lesser-known design patterns in software engineering, yet it provides significant value in specific contexts, especially when dealing with complex logic or language-like structures in your application. As software engineers, understanding patterns can streamline our code and improve maintainability, making our projects simpler and more efficient to work on. In this blog post, we will explore the Interpreter Pattern in depth and illustrate its implementation with clear Java examples.
What is the Interpreter Pattern?
The Interpreter Pattern is a behavioral design pattern that defines a representation for a grammar and provides an interpreter to evaluate sentences in that grammar. Simply put, it allows us to define a language's syntax and semantics and then create a systematic way to interpret and evaluate expressions in that language.
One of the most common use cases for the Interpreter Pattern is in situations where you want to evaluate expressions or perform operations based on a set of predefined rules or grammars. This can include things like parsing mathematical expressions, defining configuration parameters, or even interpreting simple scripting languages.
Key Components of the Interpreter Pattern
The Interpreter Pattern consists of several key components:
-
Abstract Expression: This defines an interface that will be used to interpret the grammar.
-
Terminal Expression: This implements the abstract expression and defines the basic elements of the grammar.
-
Non-terminal Expression: This implements the abstract expression and uses one or more terminal expressions to interpret larger constructs of the grammar.
-
Context: This carries information that is global to the interpreter.
Why Use the Interpreter Pattern?
-
Decoupling Logic: The interpreter separates the grammar from the execution, allowing easier updates and enhancements to the syntax without changing the interpreter.
-
Reusable Components: Once defined, the grammar elements can be reused across various parts of the application.
-
Clear Structure: By segregating logic into classes, the application adheres to the Single Responsibility Principle, enhancing overall maintainability and readability.
Implementation of the Interpreter Pattern in Java
In this section, we'll walk through a practical example of the Interpreter Pattern using Java. Suppose we want to interpret boolean expressions made up of true
, false
, and logical operators like AND
and OR
.
Step 1: Define the Context
Before diving into expressions, we need to establish a context. In this simple example, let's create a basic context that can store variables.
import java.util.HashMap;
import java.util.Map;
class Context {
private Map<String, Boolean> variables = new HashMap<>();
public void assign(String name, Boolean value) {
variables.put(name, value);
}
public Boolean get(String name) {
return variables.get(name);
}
}
Why this code is essential: The Context
class serves as a storage place for variable states, which will help evaluate our expressions.
Step 2: Define the Abstract Expression
Next, we need an abstract expression interface that will define how each expression will be interpreted.
interface Expression {
boolean interpret(Context context);
}
Step 3: Implement Terminal Expressions
Now, we will create terminal expressions for True
and False
.
class TrueExpression implements Expression {
@Override
public boolean interpret(Context context) {
return true;
}
}
class FalseExpression implements Expression {
@Override
public boolean interpret(Context context) {
return false;
}
}
Commentary: These classes implement the interpret
method, returning the boolean values directly and demonstrating how simple elements can be added to our language.
Step 4: Create Non-terminal Expressions
Now we need to implement non-terminal expressions that will use terminal expressions for our logical operators.
class OrExpression implements Expression {
private Expression left;
private Expression right;
public OrExpression(Expression left, Expression right) {
this.left = left;
this.right = right;
}
@Override
public boolean interpret(Context context) {
return left.interpret(context) || right.interpret(context);
}
}
class AndExpression implements Expression {
private Expression left;
private Expression right;
public AndExpression(Expression left, Expression right) {
this.left = left;
this.right = right;
}
@Override
public boolean interpret(Context context) {
return left.interpret(context) && right.interpret(context);
}
}
Why non-terminal expressions are crucial: They allow the combination of simple expressions to form more complex logical constructs, creating a syntax tree of interpretations.
Step 5: Example Usage
Let’s put everything together in a main class, which demonstrates how to use our interpreter:
public class InterpreterPatternDemo {
public static void main(String[] args) {
// Create context
Context context = new Context();
context.assign("A", true);
context.assign("B", false);
// A or B
Expression expression1 = new OrExpression(new TrueExpression(), new FalseExpression());
System.out.println("A or B: " + expression1.interpret(context)); // Output: true
// A and (not B)
Expression expression2 = new AndExpression(new TrueExpression(), new FalseExpression());
System.out.println("A and B: " + expression2.interpret(context)); // Output: false
}
}
Final Considerations
The Interpreter Pattern is a powerful tool that enables us to execute complex logic and define grammars in our applications with ease. By breaking down your logic into terminal and non-terminal expressions, you can maintain a clear and modular codebase.
As you explore more patterns and architectures in your software development journey, the Interpreter Pattern might just become your go-to strategy when dealing with complex language expressions or logic.
For a more comprehensive understanding of design patterns, you can check out Martin Fowler's Analysis Patterns and the Gang of Four book, "Design Patterns: Elements of Reusable Object-Oriented Software." By mastering design patterns, you will elevate your programming expertise, preparing you for the challenges of modern software development.
Let us know in the comments if you've implemented the Interpreter Pattern before and any interesting insights from your experience!