Rebranding Defensive Programming: Overcoming Misconceptions
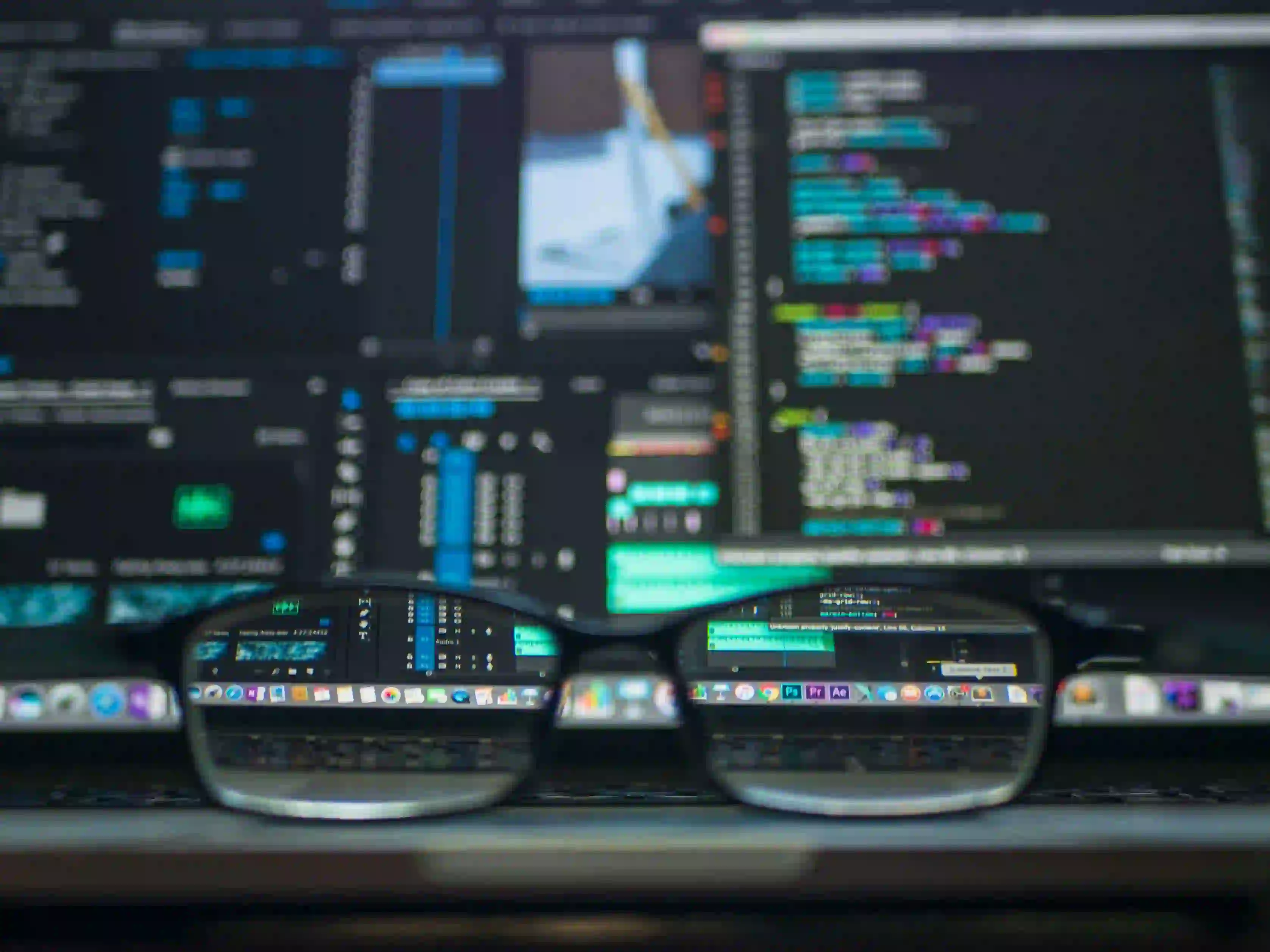
Rebranding Defensive Programming: Overcoming Misconceptions
Defensive programming is often viewed as a tedious process, laden with excessive checks and validations that some developers consider unnecessary. Yet, this approach plays a critical role in creating robust and maintainable code. In this blog post, we will explore the true value of defensive programming, debunk common misconceptions, and provide practical examples to illustrate its importance.
What is Defensive Programming?
Defensive programming is a coding paradigm that protects software from both bugs and malicious attacks. By implementing checks and validations at various stages of development, developers can better anticipate potential issues, improving the quality and security of their applications.
The Purpose of Defensive Programming
The primary goal of defensive programming is to build software that can endure unpredictable usage and unexpected behaviors. This notion stems from the belief that not only do developers write code, but users interact with it in unforeseen ways.
Common Misconceptions
1. It Slows Down Development
One prevailing myth is that implementing defensive programming slows down the development process. While it may initially seem like adding extra lines of code increases development time, the reality is that thorough validation leads to fewer bugs, ultimately speeding up the process in the long run.
Consider the following Java method as an illustration:
public int divide(int numerator, int denominator) {
if (denominator == 0) {
throw new IllegalArgumentException("Denominator cannot be zero");
}
return numerator / denominator;
}
Why This is Important: The check for a zero denominator prevents a potential ArithmeticException
from being thrown at runtime. When developers encounter unexpected errors in production, it often consumes far more time and resources to fix than investing time to prevent them during the initial coding phase.
2. It's Extraneous for Small Projects
Many developers argue that defensive programming is unnecessary for small projects or prototypes. However, even small applications can benefit immensely from reliability and maintainability.
Take a look at this snippet where we implement input validation:
public void processUserInput(String input) {
if (input == null || input.isEmpty()) {
throw new IllegalArgumentException("Input cannot be null or empty");
}
// Process input
}
Why This is Important: This simple validation transcends the project scale. A small oversight can lead to flaws that compromise user experience. Imagine the frustration when users provide input only to find your application crashes without feedback.
3. It Adds Complexity Without Benefits
Another misconception is that defensive programming introduces unnecessary complexity. However, when implemented correctly, defensive programming can help maintain clarity and prevent future confusion.
For example:
public void readFile(String filePath) {
if (filePath == null || !Files.exists(Paths.get(filePath))) {
throw new IllegalArgumentException("File path cannot be null and must exist");
}
// Continue reading the file
}
Why This is Important: The explicit checks provide transparency about what conditions must be satisfied for the method to execute correctly. This prevents misunderstanding by future developers, leading to fewer surprises.
The Benefits of Defensive Programming
1. Enhanced Software Reliability
Defensive programming does much more than catch runtime errors; it enhances reliability. When your application can handle unexpected inputs or states gracefully, it becomes more robust.
2. Improved Security
As we move into an era where cyber threats are rampant, the need for security is paramount. Defensive programming prevents common vulnerabilities such as SQL injection and buffer overflows.
3. Simplified Maintenance
Code that anticipates failures is often easier to maintain. When requirements change or new features are added, a defensively programmed system will adapt better. It provides a safety net that makes developers feel more confident.
4. User Experience
Users prefer applications that just work. A well-handled error, such as an informative message guiding users back to the right track, enhances the overall user experience.
Practical Applications in Java
Exception Handling
Exception handling is a fundamental aspect of defensive programming in Java. By structuring your code to anticipate exceptions, you position your application to respond intelligently.
public void saveUser(User user) {
try {
// Attempt to save user to the database
database.save(user);
} catch (DatabaseException e) {
// Handle database-related issues
logger.error("Database error while saving user: " + e.getMessage());
throw new UserSaveException("Error saving user");
}
}
Why This is Important: By handling exceptions gracefully, any issues are logged and the user remains informed, rather than greeted with abrupt crashes or unhandled errors.
Input Validation
Validating input is paramount. It ensures that your application only processes data conforming to expected integrity, thus improving reliability.
public void addProduct(Product product) {
if (product.getName() == null || product.getPrice() < 0) {
throw new IllegalArgumentException("Invalid product details");
}
// Add product logic
}
Why This is Important: This kind of validation prevents potential issues later in the program where invalid product names or prices could cause cascading failures.
The Last Word
Defensive programming is a fundamental practice that alleviates many issues developers often face. By questioning the misconceptions surrounding it, we can acknowledge its significance in crafting high-quality software.
The investment of time and effort in defensive programming pays off in multiple ways: increased reliability, user satisfaction, security, and ease of maintenance.
For a deeper look at software reliability, you might find these resources helpful:
- Software Reliability Engineering
- Principles of Secure Coding
Embrace defensive programming not as a burden but as a key to enhancing your software quality. Your future self, along with countless users, will thank you.