Common Pitfalls in Multi-Tier Java App Deployments on Docker
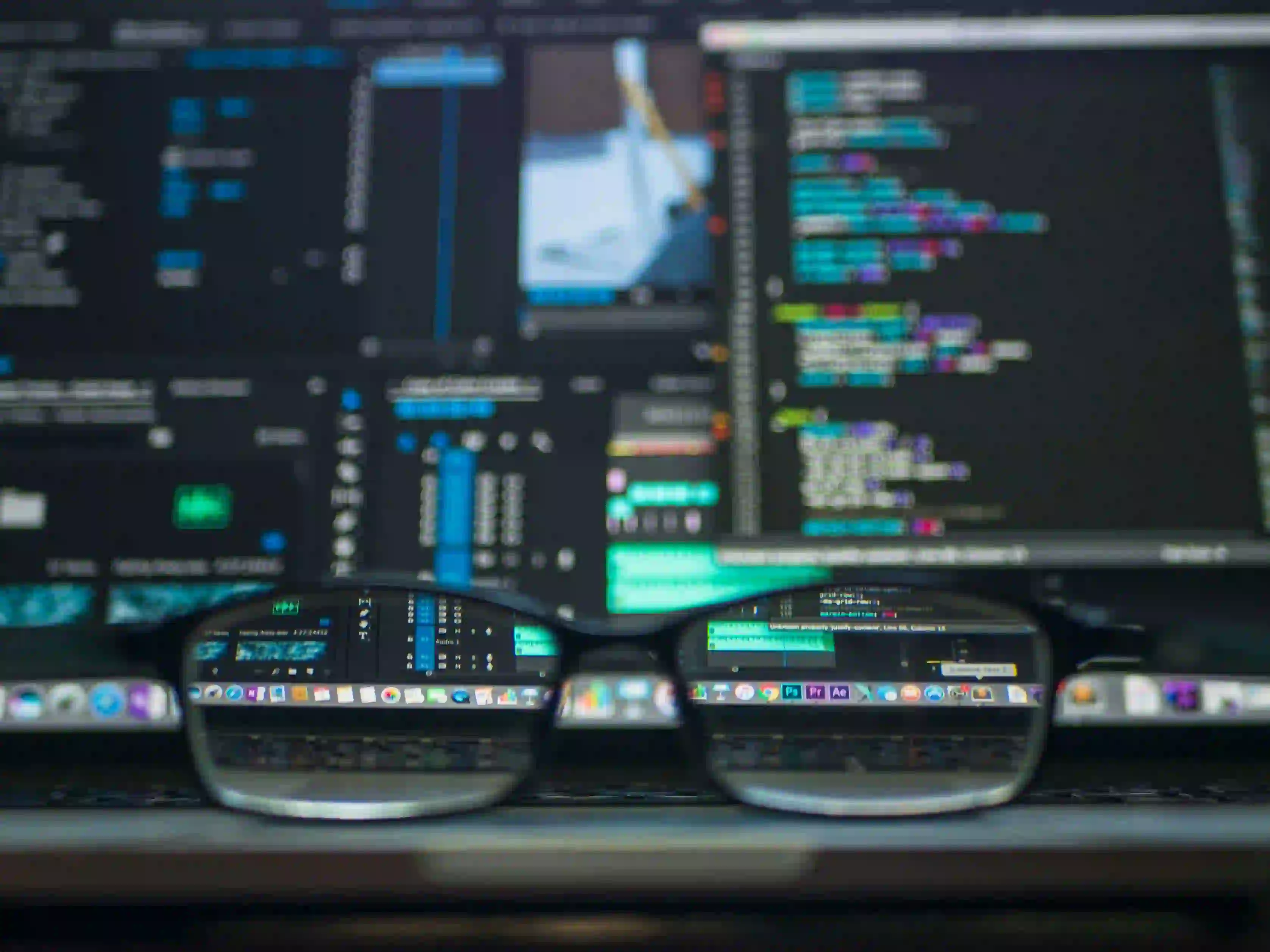
Common Pitfalls in Multi-Tier Java App Deployments on Docker
As the cloud-native approach gains traction in the software industry, deploying Java applications using Docker has become a popular choice. However, organizations often encounter hurdles when deploying multi-tier Java applications in Docker containers. In this blog post, we will explore common pitfalls associated with these deployments, provide practical solutions, and illustrate clear code snippets where relevant.
Understanding Multi-Tier Architecture
Before we delve into the pitfalls, it is essential to understand what a multi-tier architecture entails. This architecture separates the application into different layers. Typically, these include:
- Presentation Layer: Interface that users interact with.
- Business Logic Layer: Processes data and applies business rules.
- Data Access Layer: Manages data operations and database interactions.
When deploying such a structure in Docker, it is important to recognize how each tier communicates, can be scaled, and is managed.
Common Pitfalls
1. Incorrect Network Configuration
One of the primary issues when deploying multi-tier applications in Docker is misconfiguring networks. By default, containers are isolated, which can lead to communication issues.
Solution: Use a dedicated Docker network.
# Create a user-defined bridge network
docker network create my-network
By running containers within the same network, communication between them becomes seamless. For example, when deploying a Java application along with a database, ensuring both run on my-network
ensures they can communicate without hiccups.
2. Hardcoding Configuration Values
Hardcoding configuration values such as database URLs or API keys is a common mistake. This can lead to challenges in configurations, especially between different environments (development, staging, production).
Solution: Make use of environment variables.
# Dockerfile snippet to utilize environment variables
ENV DATABASE_URL=${DATABASE_URL}
Using Docker secrets and environment variables allows for more flexible configurations that can easily be changed without altering the code. This is especially useful in CI/CD workflows.
3. Dependence on Container State
Containers are ephemeral by design. Assuming that the container will always carry state relevant to the application can lead to issues. For instance, if a Java application relies on a stateful backend system, losing its state could lead to application failures.
Solution: Use external data storage.
You can utilize databases, object storage or similar services to manage application state outside of the container.
// Example of accessing an external database
public Connection connectToDatabase() {
try {
String dbUrl = System.getenv("DATABASE_URL");
Connection conn = DriverManager.getConnection(dbUrl);
return conn;
} catch (SQLException e) {
throw new RuntimeException("Error connecting to the database", e);
}
}
4. Not Implementing Logging and Monitoring
Deploying in a container requires a shift in how you deal with logging. Logs from Docker containers require careful management, and failing to do so can make debugging difficult.
Solution: Use centralized logging services.
Integrating logging libraries like Logback or SLF4J in your Java application can ensure logs are captured properly.
<!-- Logback dependency in pom.xml -->
<dependency>
<groupId>ch.qos.logback</groupId>
<artifactId>logback-classic</artifactId>
<version>1.2.3</version>
</dependency>
5. Ignoring Resource Limits
Containers can be allocated resources like CPU and memory, but not defining these resources can lead to performance degradation or outages.
Solution: Set resource limits in your Docker Compose file or directly in your Docker run commands.
# docker-compose.yml example setting resource limits
services:
java-app:
image: my-java-app
deploy:
resources:
limits:
cpus: '0.50'
memory: 512M
6. Failing to Handle Container Lifecycles
Understanding and managing container lifecycles is crucial. Containers can crash or restart unexpectedly, and not handling these scenarios can lead to service downtime.
Solution: Incorporate health checks.
# Adding a health check in your Dockerfile
HEALTHCHECK CMD curl --fail http://localhost:8080/health || exit 1
Implementing health checks ensures that the orchestration system (like Kubernetes) can manage the container effectively by restarting it when necessary.
7. Poor Image Optimization
Using bloated base images can drastically increase deployment times and resource consumption.
Solution: Use optimized base images like adoptopenjdk:11-jre-slim
.
# Optimize Java base image
FROM adoptopenjdk:11-jre-slim
COPY target/my-app.jar /usr/app/my-app.jar
ENTRYPOINT ["java", "-jar", "/usr/app/my-app.jar"]
To Wrap Things Up
Deploying multi-tier Java applications using Docker provides remarkable flexibility and scalability but can be fraught with mistakes. A thorough understanding of architecture, configuration management, logging, resource allocation, and container lifecycles can streamline your deployment process and mitigate potential pitfalls.
As you embark on your Docker journey, keep best practices at the forefront. It's not just about deploying your application but ensuring its stability and robustness in production.
For additional insights into Docker and Java best practices, check out Docker's Official Documentation and The Twelve-Factor App Methodology that can guide you to develop more resilient applications in the cloud.
Happy coding!