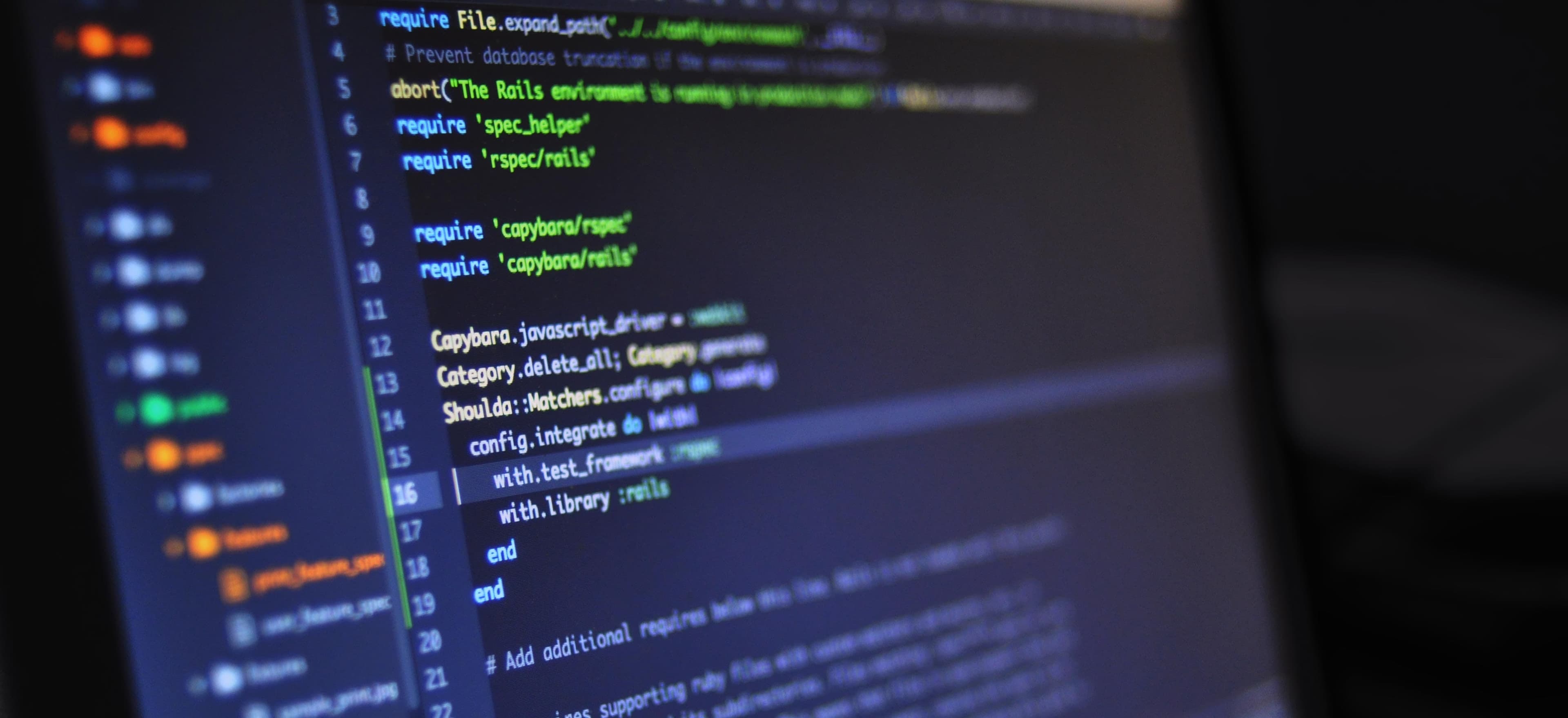
- Published on
Handling External Links in PrimeFaces Dynamic Dialogs
PrimeFaces is a popular UI framework for JavaServer Faces (JSF) that simplifies the creation of web applications. One of its components, the Dynamic Dialog, provides a convenient way to show content in a modal form, making it a favorite among developers for displaying additional information without navigating away from the current view.
However, there are situations where you might want to incorporate external links inside these dialogs. This blog post will explore how to handle external links in PrimeFaces Dynamic Dialogs effectively.
What is PrimeFaces?
Before diving into the implementation, it's essential to understand what PrimeFaces offers. PrimeFaces is an open-source UI library that provides a rich set of UI components for JSF. These components, such as data tables, graphs, and dialogs, promote modern user interface practices that enhance user experience. Dynamic Dialogs are particularly valuable as they enable you to load different views or data on the fly.
Understanding the Dynamic Dialog
A Dynamic Dialog in PrimeFaces allows you to create a modal that can be used to display additional information or take user input without disrupting the original page's flow. Here's how a minimal example of a PrimeFaces Dynamic Dialog looks:
<p:dialog header="Dynamic Dialog" widgetVar="dynamicDialog" modal="true">
<h:outputText value="This is a dynamic dialog!" />
</p:dialog>
<p:commandButton value="Show Dialog"
onclick="PF('dynamicDialog').show();"
type="button" />
In this snippet, a button is utilized to open the dialog, which contains a simple text message.
Opening External Links in Dynamic Dialogs
Now, let's tackle the handling of external links. If you want to present information that resides on an external site inside your Dynamic Dialog, there are effective approaches you can take.
Approach 1: Using Iframe
One way to display an external page is to embed it within an iframe
. Here’s how you can do this:
<p:dialog header="External Content" widgetVar="externalDialog" modal="true" resizable="false" width="800">
<h:panelGrid columns="1">
<iframe src="https://www.example.com" width="100%" height="400px" frameborder="0"></iframe>
</h:panelGrid>
</p:dialog>
<p:commandButton value="Open External Link"
onclick="PF('externalDialog').show();"
type="button" />
Commentary on Using Iframe
-
Security Concerns: Be cautious; not all websites allow their content to be displayed in an iframe. Check if the X-Frame-Options header of the target site is set to "DENY" or "SAMEORIGIN".
-
User Experience: Embedding can offer a seamless experience. Always ensure the external site is responsive. A static width and height may ruin the UX on mobile devices.
-
SEO Considerations: Since the content is presented within your web application, it might dilute the importance of the external site from an SEO perspective. Consider optimally using iframe based on your content objectives.
Approach 2: Using Anchor Links
Alternatively, if the goal is to redirect users to an external site instead of displaying content within the application, use HTML anchor links within the dialog:
<p:dialog header="External Site" widgetVar="linkDialog" modal="true">
<h:outputText value="Visit our partner website:" />
<h:outputLink value="https://www.example.com" target="_blank">Click Here</h:outputLink>
</p:dialog>
<p:commandButton value="Show Link"
onclick="PF('linkDialog').show();"
type="button" />
Commentary on Anchor Links
-
User Choice: This method provides user choice—whether to open the link in the same tab or a new one.
-
Accessibility: Adding
target="_blank"
ensures that the user maintains access to your application. This practice can enhance the user experience by reducing friction. -
Best Practices: Use
nofollow
on links to external sites if you don't want search engines to pass authority.
Styling and Customization
It is crucial to maintain the overall aesthetic and usability of your dialog. PrimeFaces allows customization through CSS classes. You can define your styles for iframe or content wrapping, which enhances the visual consistency.
Example CSS:
.ui-dialog .ui-dialog-content {
padding: 20px;
font-family: Arial, sans-serif;
}
.ui-dialog .ui-dialog-titlebar {
background-color: #4CAF50;
color: white;
}
Link the CSS file in your XHTML header, ensuring that your changes apply uniformly across your dialogs.
Error Handling and Performance
While dealing with external links, error handling is vital to maintain user trust.
-
Fallback Content: If the iframe fails to load, consider providing fallback content.
-
Loading Indicators: Users appreciate visual feedback. Use PrimeFaces loading indicators when fetching external sites, particularly if it loads asynchronously.
Example of loading indicator in conjunction with an iframe:
<p:dialog header="Load External" widgetVar="loadingDialog" modal="true">
<p:outputPanel id="externalContent">
<p:ajax onstart="PF('loadingDialog').show();"
oncomplete="PF('loadingDialog').hide();" />
<iframe src="https://www.example.com" width="100%" height="400px"></iframe>
</p:outputPanel>
</p:dialog>
Final Thoughts
Incorporating external links into PrimeFaces Dynamic Dialogs enriches the user experience of your web application. Whether choosing to embed an external page or redirecting users, each method holds unique advantages.
As you implement these strategies, remember always to prioritize user experience and security. Consider utilization contexts, potential vulnerabilities, and resource loading implications. With these best practices in mind, you can create a more engaging and informative interface for your users.
For more exhaustive information on PrimeFaces navigation and best practices, visit PrimeFaces Official Documentation and explore deeper into its capabilities.
Happy coding!