Mastering Google Guava Multisets: Common Pitfalls Uncovered
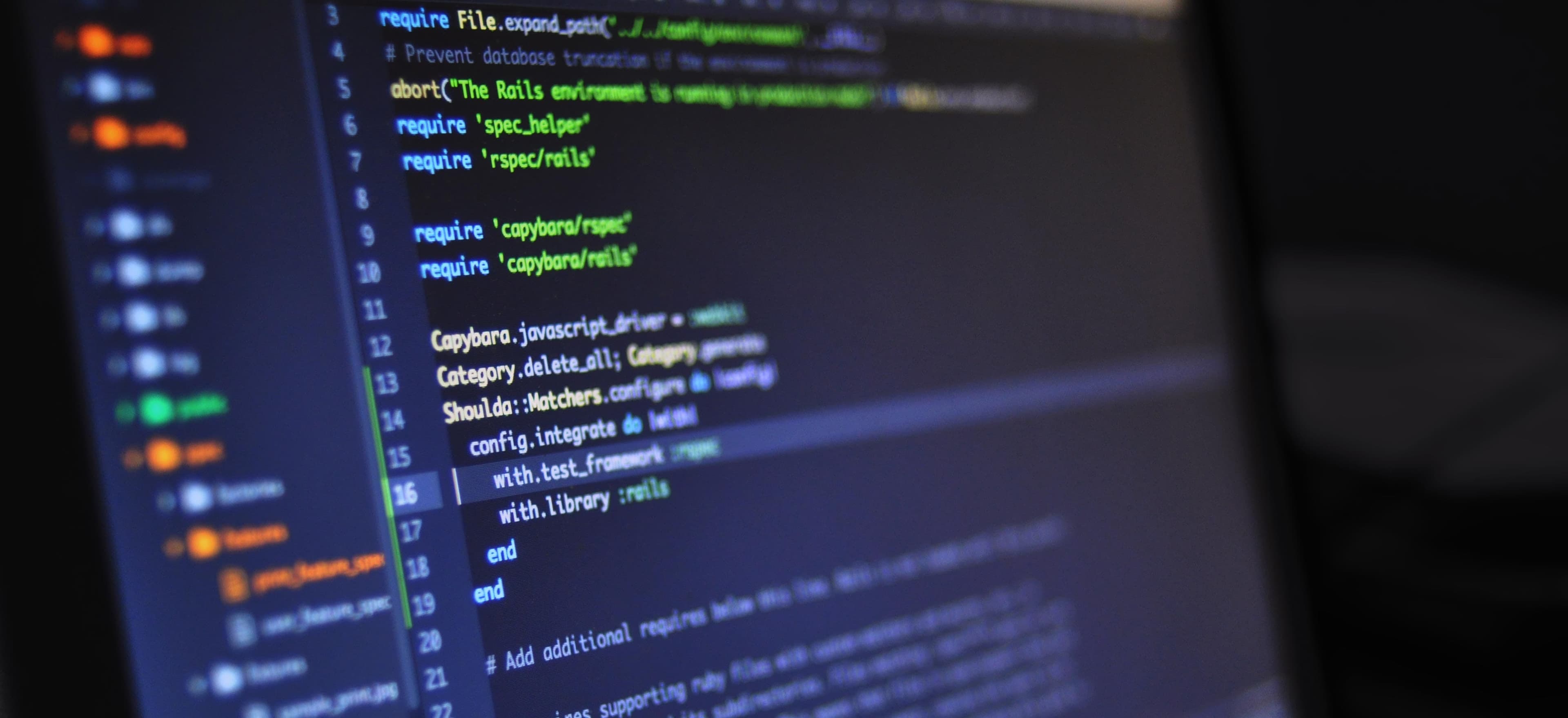
- Published on
Mastering Google Guava Multisets: Common Pitfalls Uncovered
Google Guava is a set of core libraries for Java that enhances the standard Java libraries. Among its features, the Multiset provides a powerful way to manage collections of elements, allowing for duplicate entries while maintaining the characteristics of a set. This post will guide you through mastering Guava's Multiset, highlighting common pitfalls you might encounter and how to avoid them.
What is Guava Multiset?
A Multiset is a collection that allows duplicates. Unlike a regular Set
, which only keeps unique elements, a Multiset counts occurrences. For instance, when counting the number of words in a document, using a Multiset allows you to store the count of each word effectively.
Basic Usage of Multiset
To begin using Multiset from Guava, you first need to add the Guava dependency to your project:
<dependency>
<groupId>com.google.guava</groupId>
<artifactId>guava</artifactId>
<version>31.0.1-jre</version>
</dependency>
Creating a Multiset
You can create a Multiset using the HashMultiset
class from Guava:
import com.google.common.collect.HashMultiset;
import com.google.common.collect.Multiset;
public class MultisetExample {
public static void main(String[] args) {
Multiset<String> multiset = HashMultiset.create();
// Adding elements
multiset.add("apple");
multiset.add("banana");
multiset.add("apple"); // Duplicate
System.out.println("Apple count: " + multiset.count("apple")); // Output: 2
System.out.println("Total elements in multiset: " + multiset.size()); // Output: 3
}
}
Why Use Multiset?
The above example illustrates the beauty of Multiset when tracking the number of occurrences in a collection. Its utility shines in scenarios like:
- Counting frequency of items.
- Handling multi-dimensional data.
- Multiset operations in graphs and advanced data structures.
However, misusing Multiset can lead to performance issues or incorrect data representation. Let’s explore some pitfalls.
Common Pitfalls When Using Guava Multiset
1. Forgetting to Handle Null Values
One common mistake is adding null values to a Multiset without proper checks. Null elements can lead to NullPointerException
in your application.
Issue:
multiset.add(null); // NullPointerException
Solution:
You should always validate inputs before adding them. Use condition checks:
if (item != null) {
multiset.add(item);
}
2. Not Understanding the Count Mechanism
Many developers misunderstand how element counting works in Multisets. The count()
method provides the number of occurrences, but performance might degrade if used carelessly.
Issue:
Frequent count checks in a loop can lead to performance drops, particularly for large multisets.
Solution:
Retrieve the count once, store it, and use it within the loop:
String target = "apple";
int appleCount = multiset.count(target);
for (int i = 0; i < appleCount; i++) {
System.out.println("Processing apple #" + (i + 1));
}
3. Unintended Modifications During Iteration
When iterating over a Multiset, it is crucial to remember that modifying the collection during iteration can lead to ConcurrentModificationException
.
Issue:
for (String fruit : multiset) {
if (fruit.equals("apple")) {
multiset.remove(fruit); // Throws ConcurrentModificationException
}
}
Solution:
Use an Iterator
for safe removal during iteration:
Iterator<String> iterator = multiset.iterator();
while (iterator.hasNext()) {
String fruit = iterator.next();
if (fruit.equals("apple")) {
iterator.remove(); // Safe way to remove
}
}
4. Misusing Multisets in a Multithreaded Environment
Multisets are not thread-safe by default. In a concurrent application, this can be a significant pitfall.
Issue:
Multiple threads adding or removing elements can corrupt the Multiset state.
Solution:
Consider using Collections.synchronizedCollection
or ConcurrentHashMap
to manage concurrent access. Alternatively, you can use concurrent collections from java.util.concurrent
.
Multiset<String> concurrentMultiset = HashMultiset.create();
Collections.synchronizedCollection(concurrentMultiset);
5. Confusing Multiset with Multimap
Another common mistake is confusing Multiset with Multimap. While they both allow multiple values for a single key, their behavior and intended use cases differ significantly.
Issue:
Trying to use Multiset as you would Multimap can lead to data inaccuracies.
Solution:
Understand the functional differences: use Multiset when you need to count occurrences of elements and use Multimap when you're associating keys with multiple values.
import com.google.common.collect.ArrayListMultimap;
import com.google.common.collect.ListMultimap;
ListMultimap<String, Integer> multimap = ArrayListMultimap.create();
multimap.put("apple", 1);
multimap.put("apple", 2); // Stores 2 values for "apple"
Lessons Learned
Google Guava Multisets offer a powerful way to manage collections with duplicate entries efficiently. However, understanding its pitfalls is crucial for effective usage. By being aware of these common mistakes—such as handling null values correctly, understanding the count mechanism, and ensuring safe modifications during iterations—you can harness the full power of Multisets in your Java applications.
For more information on advanced Guava usage, consider checking out the Google Guava Documentation and explore further on its collection functionalities.
By following the tips provided here as you work with Multisets, you can avoid pitfalls that can lead to errors and improve your application’s performance. Start mastering Guava Multisets today, and elevate your Java development to the next level!
Checkout our other articles