Boosting Performance: Master JMH in Java with Gradle
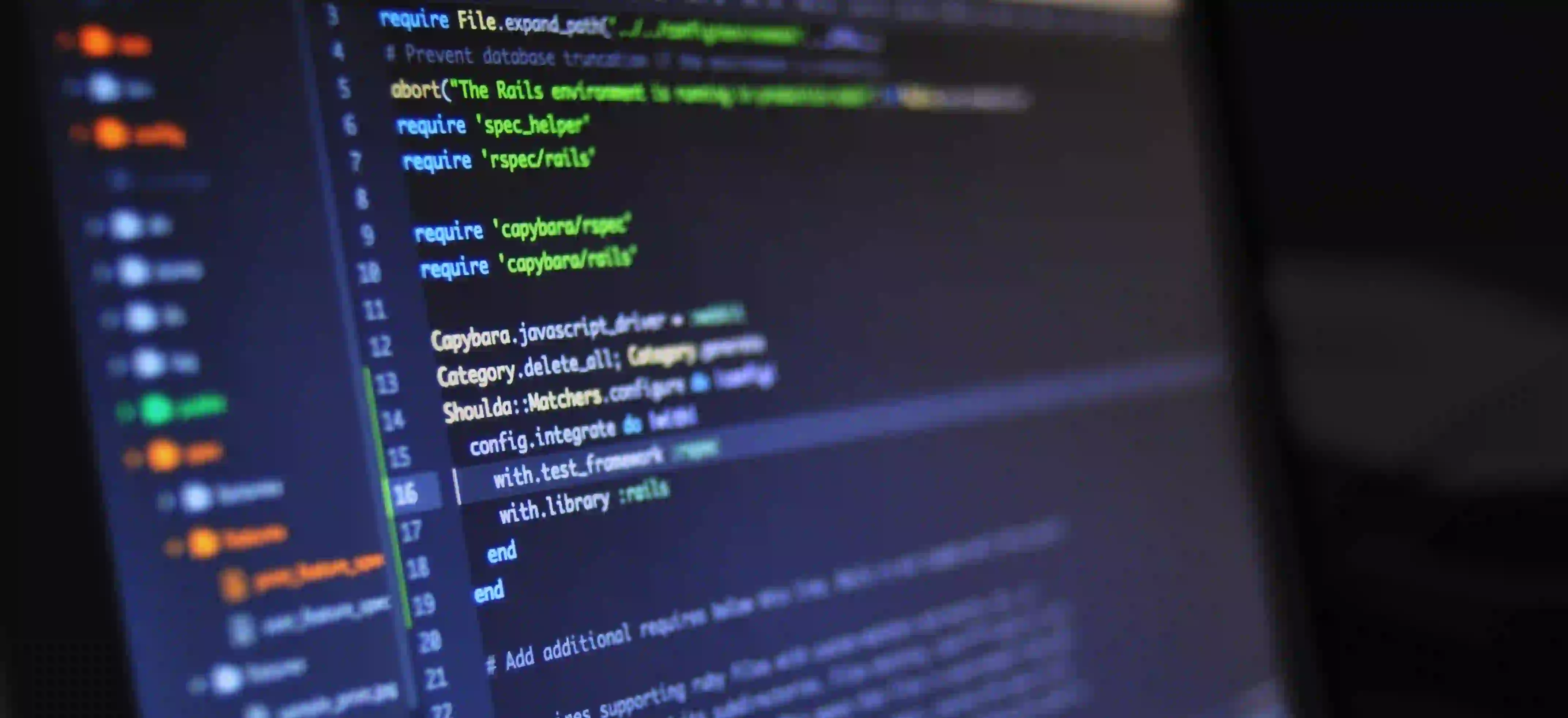
Boosting Performance: Master JMH in Java with Gradle
In Java programming, performance is often a critical factor. Whether you are developing a high-frequency trading system, an enterprise application, or simply optimizing your code, understanding how different aspects of your code affect performance is crucial. The Java Microbenchmark Harness (JMH) is a tool specifically designed for this purpose. In this blog post, we will explore how to effectively use JMH with Gradle to measure the performance of your Java code, alongside best practices and examples.
What is JMH?
JMH is a Java library designed for benchmarking code snippets. It handles the intricacies of JVM optimizations, ensuring that your benchmarks are accurate and reliable. This means you won't end up with misleading results that can arise from just running methods in a simple loop.
Key Features of JMH
- Precision: JMH eliminates many common issues that developers face when writing benchmarks.
- Configurability: Supports various benchmarking scenarios, allowing you to configure parameters specific to your needs.
- Ease of Use: Integrates seamlessly with Java projects, making the setup and execution of benchmarks straightforward.
Getting Started with Gradle and JMH
Setting Up Your Gradle Project
-
Create a new Gradle project. If you haven’t already, create a new Gradle project. You can use the command line:
🔧snippet.shmkdir JMHExample cd JMHExample gradle init --type java-library
-
Add the JMH Dependency. Update your
build.gradle
file to include JMH as a dependency.📄snippet.txtplugins { id 'java' id 'me.champeau.jmh' version '0.6.6' } repositories { mavenCentral() } dependencies { // Add JMH dependency jmh 'org.openjdk.jmh:jmh-core:1.35' jmh 'org.openjdk.jmh:jmh-generator-annprocess:1.35' }
The
me.champeau.jmh
plugin simplifies the process of using JMH by automatically generating the necessary classes for benchmarking.
Creating Your First Benchmark
-
Create a Benchmark Class. Create a new Java class in
src/main/java
calledMyBenchmark.java
.☕snippet.javaimport org.openjdk.jmh.annotations.*; import java.util.concurrent.TimeUnit; @BenchmarkMode(Mode.AverageTime) @OutputTimeUnit(TimeUnit.MILLISECONDS) @State(Scope.Thread) public class MyBenchmark { @Benchmark public void testMethod() { // Simulate some workload long sum = 0; for (int i = 0; i < 1000; i++) { sum += i; } } }
Why This Code?
- @BenchmarkMode(Mode.AverageTime): This annotation specifies that the benchmark will measure the average time taken by the
testMethod
. - @OutputTimeUnit(TimeUnit.MILLISECONDS): We want the output in milliseconds for clearer insight into time spent.
- @State(Scope.Thread): This manages the state of the benchmark. It's convenient for preserving state across multiple invocations in a single thread.
Running the Benchmark
To run your benchmarks, execute the following command in your terminal:
./gradlew jmh
This will compile your code and execute the benchmarks. You should see output that reflects the performance of your method.
Interpreting Results
The output might look like this:
[info] Benchmark Mode Cnt Score Error Units
[info] MyBenchmark.testMethod avgt 5 0.234 ± 0.012 ms/op
This indicates that the average time taken for testMethod
is approximately 0.234 milliseconds.
Advanced Benchmarking
In real-world scenarios, benchmarking often requires comparison between different implementations or configurations. You can extend your benchmarking class to investigate performance further.
For instance, let’s add another method that uses streams for calculation:
@Benchmark
public void testStreamMethod() {
long sum = IntStream.range(0, 1000).sum();
}
Complete Benchmarking Class
Your complete benchmark class will look like this:
import org.openjdk.jmh.annotations.*;
import java.util.concurrent.TimeUnit;
import java.util.stream.IntStream;
@BenchmarkMode(Mode.AverageTime)
@OutputTimeUnit(TimeUnit.MILLISECONDS)
@State(Scope.Thread)
public class MyBenchmark {
@Benchmark
public void testMethod() {
long sum = 0;
for (int i = 0; i < 1000; i++) {
sum += i;
}
}
@Benchmark
public void testStreamMethod() {
long sum = IntStream.range(0, 1000).sum();
}
}
Rerun and Compare
Re-run your benchmarks to compare testMethod
and testStreamMethod
. JMH will show the average execution time for both, allowing you to identify which approach is more efficient.
In Conclusion, Here is What Matters
By incorporating JMH to benchmark your Java code, you gain precise insights that allow you to optimize your code effectively. This is essential for maintaining performance in production systems. As you experiment with different implementations, you will be equipped to make better-informed decisions.
Further Reading
Mastering performance in Java is a journey, and using tools like JMH, coupled with Gradle, makes it achievable. Dive into your benchmarking endeavors, and elevate the performance of your applications! Happy coding!