Overcoming Challenges in Stubbing Key-Value Stores for Testing
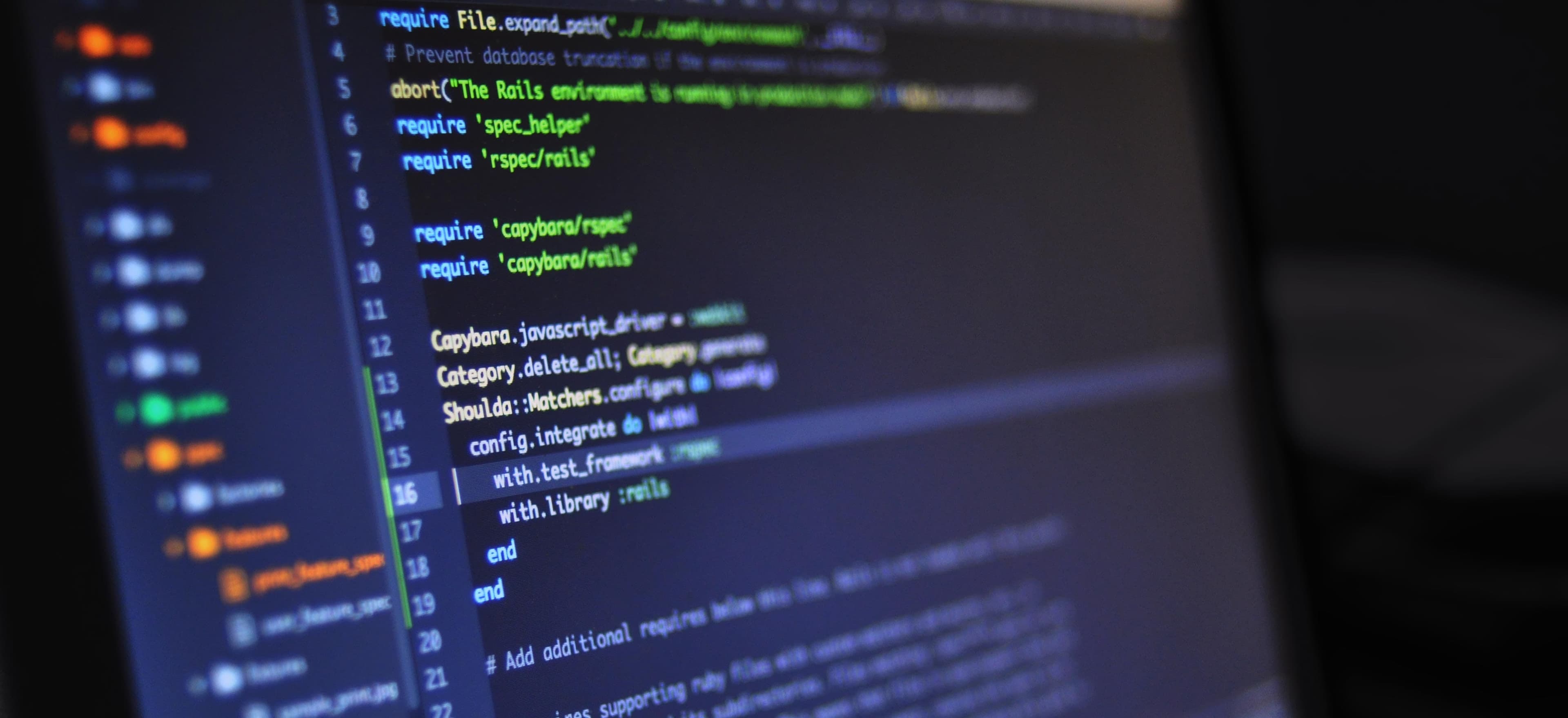
- Published on
Overcoming Challenges in Stubbing Key-Value Stores for Testing
Testing is an integral part of the software development lifecycle. However, when it comes to testing applications that rely on key-value stores, developers often face unique challenges. Stubbing a key-value store can help simulate interactions without the need to connect to a real database, enhancing the efficiency and reliability of tests. In this post, we will explore the hurdles developers encounter, strategies to overcome them, and practical examples using Java.
Understanding Key-Value Stores
Key-value stores are a popular type of NoSQL database that provide a simple abstraction for storing data as key-value pairs. Databases like Redis, Amazon DynamoDB, and Memcached are classic examples. Applications often depend on these stores for:
- Scalability: They can handle large amounts of data efficiently.
- Performance: Providing quick access to values via keys.
- Flexibility: Enabling various data formats.
However, stubbing these systems for testing can present specific challenges that developers need to navigate.
Challenges in Stubbing Key-Value Stores
-
Complex Data Structures: Unlike traditional databases, key-value stores can handle diverse data types. Stubbing them means simulating these complex structures accurately.
-
Concurrency Issues: Many applications perform read and write operations concurrently. Ensuring your stub handles these scenarios can be tricky.
-
Operational Environment: Your testing environment may differ from production. This discrepancy can lead to false negatives in tests if not managed correctly.
-
Performance Metrics: When testing caching solutions like Redis, it's important to simulate performance metrics. How do you measure effectiveness without a real key-value store?
-
Data Persistence: Often, testing requires a level of data persistence that may not be straightforward to replicate in a stub.
Best Practices for Stubbing Key-Value Stores
To mitigate the challenges above, developers can employ several strategies:
1. Utilize In-Memory Data Structures
By leveraging in-memory data structures, you allow your stub to behave like a key-value store, thus preserving performance and data retrieval speeds.
Example Implementation:
import java.util.HashMap;
import java.util.Map;
public class InMemoryKeyValueStore {
private final Map<String, String> store = new HashMap<>();
public void put(String key, String value) {
store.put(key, value);
}
public String get(String key) {
return store.get(key);
}
public boolean exists(String key) {
return store.containsKey(key);
}
}
Why This Works: This implementation allows for quick lookups, mirroring the efficient access patterns in real key-value stores while maintaining simplicity in your tests. This is especially useful for unit tests requiring setup and teardown of state before and after execution.
2. Simulate Concurrency
A common pitfall in stubbing is failing to simulate concurrent operations. A stub should be capable of handling multi-threaded access patterns to adequately mimic production behavior.
Example Implementation:
import java.util.concurrent.ConcurrentHashMap;
public class ConcurrentKeyValueStore {
private final ConcurrentHashMap<String, String> store = new ConcurrentHashMap<>();
public void put(String key, String value) {
store.put(key, value);
}
public String get(String key) {
return store.get(key);
}
public boolean exists(String key) {
return store.containsKey(key);
}
}
Why This Works: The ConcurrentHashMap
allows safe multi-threaded access, reflecting how your application might interact with a real key-value store under load.
3. Leverage Mocking Frameworks
In scenarios where you want to isolate tests from the real interaction with a key-value store, using mocking frameworks such as Mockito can provide a streamlined approach.
Example Usage:
import static org.mockito.Mockito.*;
public class KeyValueServiceTest {
@Test
public void shouldStoreValueInKeyValueStore() {
// Arrange
InMemoryKeyValueStore mockStore = mock(InMemoryKeyValueStore.class);
when(mockStore.get("key1")).thenReturn("value1");
// Act
String result = mockStore.get("key1");
// Assert
assertEquals("value1", result);
}
}
Why This Works: Mocking frameworks allow you to create behavior for your dependencies without having to instantiate a full implementation. This leads to quicker tests focused on aspects of your application logic rather than integrations with external systems.
4. Create a Local Testing Environment
To better mimic production behavior, consider setting up a local key-value store for testing. Solutions like Docker simplify this process.
Relevant Resource: For a deeper dive into Dockerizing databases, you can visit the Docker documentation.
Lessons Learned
Stubbing key-value stores for testing is an essential skill for modern developers. By understanding the core challenges, applying in-memory structures, simulating concurrency, utilizing mocking frameworks, and setting up local testing environments, you can effectively create robust tests that mimic the behavior of your application in production.
By adopting these practices, your testing process will not only become transparent but also ensure that your application remains reliable in its interactions with key-value stores. Remember, the ultimate goal is to create a testing setup that closely resembles the production environment while maintaining efficiency in test execution. Happy coding!
Checkout our other articles