Troubleshooting JAX-RS Request Method Matching Issues
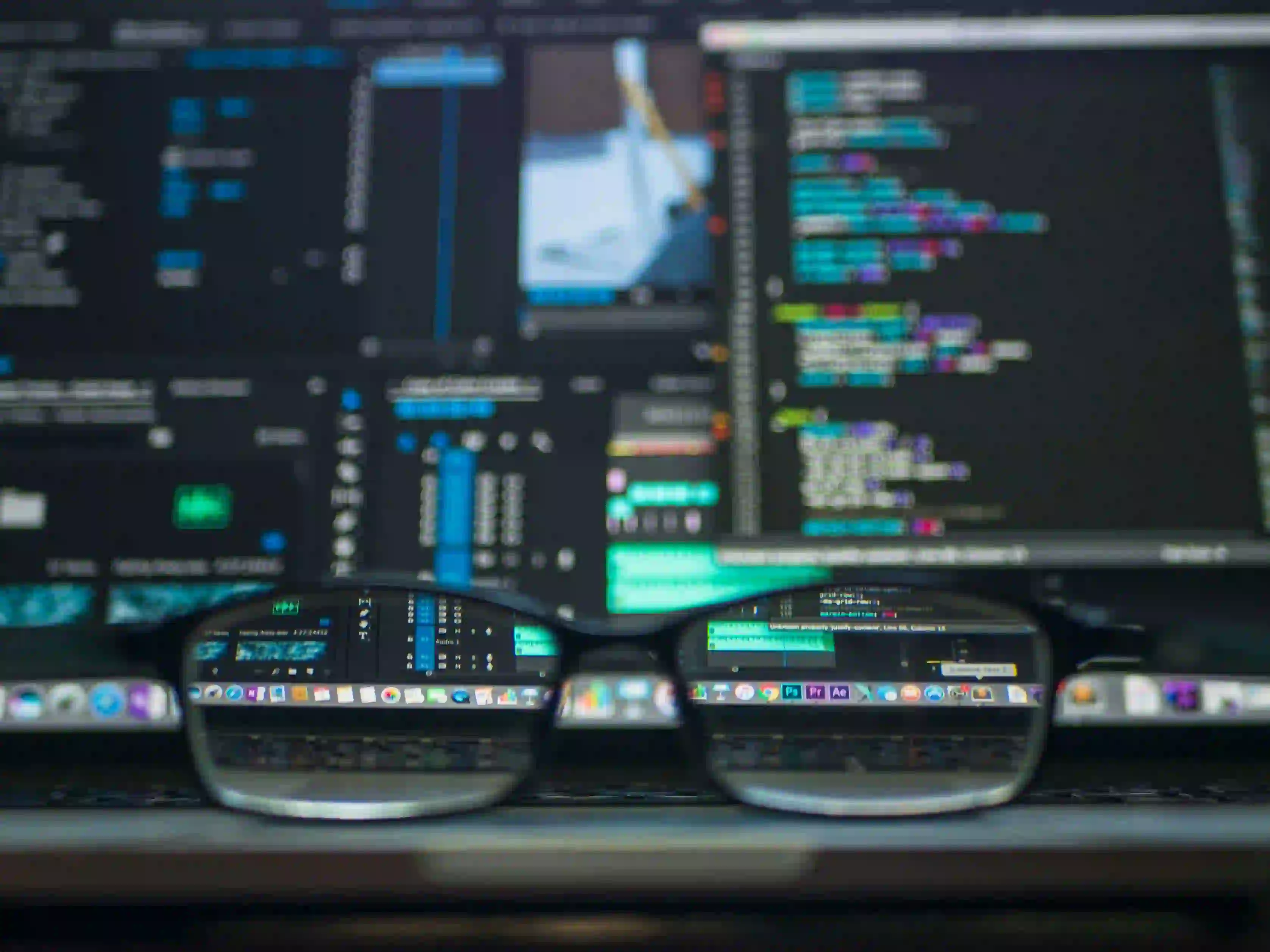
Troubleshooting JAX-RS Request Method Matching Issues
In today's RESTful architecture, JAX-RS (Java API for RESTful Web Services) plays a pivotal role in building web services in Java. However, even seasoned developers can run into issues when request methods aren’t being matched as expected. This can lead to frustrating debugging sessions. In this blog post, we’ll explore some common causes of request method matching issues and how to resolve them effectively.
Understanding JAX-RS Annotations
JAX-RS uses annotations to convey HTTP request methods. The primary annotations in this context are:
@GET
@POST
@PUT
@DELETE
@HEAD
@OPTIONS
@PATCH
Here’s a simple example of how these annotations are utilized to create a RESTful resource:
import javax.ws.rs.GET;
import javax.ws.rs.Path;
import javax.ws.rs.Produces;
import javax.ws.rs.core.MediaType;
@Path("/hello")
public class HelloWorldResource {
@GET
@Produces(MediaType.TEXT_PLAIN)
public String sayHello() {
return "Hello, World!";
}
}
In the code snippet above, a simple REST service responds with "Hello, World!" when a GET request is made to /hello
. If this method isn't matched correctly, it could be due to several issues.
Common Reasons for Request Method Matching Issues
1. Incorrect HTTP Method
The HTTP method specified in the request must precisely match the method in your resource class. For example, a POST request will not trigger a method annotated with @GET
.
Solution:
Verify the client sends the correct HTTP method. You can use tools like Postman or curl to check this.
curl -X GET http://localhost:8080/yourapi/hello
2. Incorrect URL Path
The path defined using @Path
annotations can lead to mismatches if they aren't aligned with the client requests. Pay close attention to the casing, trailing slashes, and parameters.
Solution:
Ensure the URL in the client matches the exact path. If your resource class is defined as follows:
@Path("/hello")
public class HelloWorldResource { ... }
A request to /Hello
or /hello/
may not match this resource.
3. Parameter Mismatch
If your methods expect parameters (either query parameters or path parameters), and the client request doesn’t include them, the matching may fail.
Example of a method expecting a path parameter:
@GET
@Path("/{id}")
public Response getItem(@PathParam("id") String id) {
// Your logic here
}
If you request /item
, it won't match since it expects an id
.
Solution:
Check that your request includes the required parameters in the correct format.
4. Ambiguous Method Signatures
Sometimes, you might have multiple methods that appear to match a request. JAX-RS may get confused about which method to invoke.
@GET
@Path("/items")
public List<Item> getItems() { ... }
@GET
@Path("/items/{id}")
public Item getItem(@PathParam("id") int id) { ... }
Solution:
Use more specific paths to prevent ambiguities. Explicitly define parameters or modify method names.
5. Default Methods in Interfaces
If you are using interfaces with default methods in your JAX-RS application, it can lead to unexpected behavior:
public interface ItemService {
@GET
List<Item> getItems();
}
This can confuse the JAX-RS engine when it’s trying to resolve the method matching.
Solution:
Define your resource methods only in concrete classes, and avoid default methods from interfaces as they can introduce complications.
6. Content Negotiation Issues
Content negotiation allows clients to specify what media type they can handle. If your resource responds with a format that the client doesn't accept, it leads to a 406 Not Acceptable error.
@GET
@Produces({MediaType.APPLICATION_JSON, MediaType.APPLICATION_XML})
public Response getItem() { ... }
Solution:
Check the Accept
headers in your requests. Ensure clients provide acceptable media types like:
Accept: application/json
7. Deployment Descriptor Issues
If your application is packaged in a WAR file, the web.xml file can affect request method matching if not configured properly.
Solution:
Make sure the web.xml
is correctly mapping your JAX-RS servlet. For example:
<servlet>
<servlet-name>JAXRSServlet</servlet-name>
<servlet-class>org.glassfish.jersey.servlet.ServletContainer</servlet-class>
<init-param>
<param-name>jersey.config.server.provider.packages</param-name>
<param-value>com.yourpackage</param-value>
</init-param>
<load-on-startup>1</load-on-startup>
</servlet>
<servlet-mapping>
<servlet-name>JAXRSServlet</servlet-name>
<url-pattern>/api/*</url-pattern>
</servlet-mapping>
Make sure the servlet mapping (/api/*
) prefixes your resource path correctly.
Debugging Techniques
1. Logging HTTP Requests
Configure logging to monitor incoming requests and examine the logs for errors and mismatches. Libraries like SLF4J can be useful:
<dependency>
<groupId>org.slf4j</groupId>
<artifactId>slf4j-api</artifactId>
<version>1.7.30</version>
</dependency>
2. Use an HTTP Proxy
Tools like Fiddler or Wireshark can help you see exactly what traffic is leaving your client, allowing you to validate HTTP methods, parameters, and headers.
3. Debugging with Breakpoints
Leverage your IDE’s debugging capabilities. Set breakpoints in your resource methods to inspect the incoming requests and parameters.
Final Thoughts
Troubleshooting JAX-RS request method matching issues can be daunting, but by understanding how each component works and employing some debugging techniques, you can significantly reduce the time spent fixing these issues.
If you find yourself frequently encountering request matching issues, consider reading this resource on JAX-RS best practices for further insights. Always ensure that your annotations, methods, and mappings are configured accurately to enjoy a smoother development experience.
By following this guidance, you will not only resolve the current issues but also gain a deeper understanding of how JAX-RS operates, ultimately enhancing your ability to build scalable and maintainable RESTful services.