Unlocking Java Concurrency: Fixing Hidden Thread Deadlocks
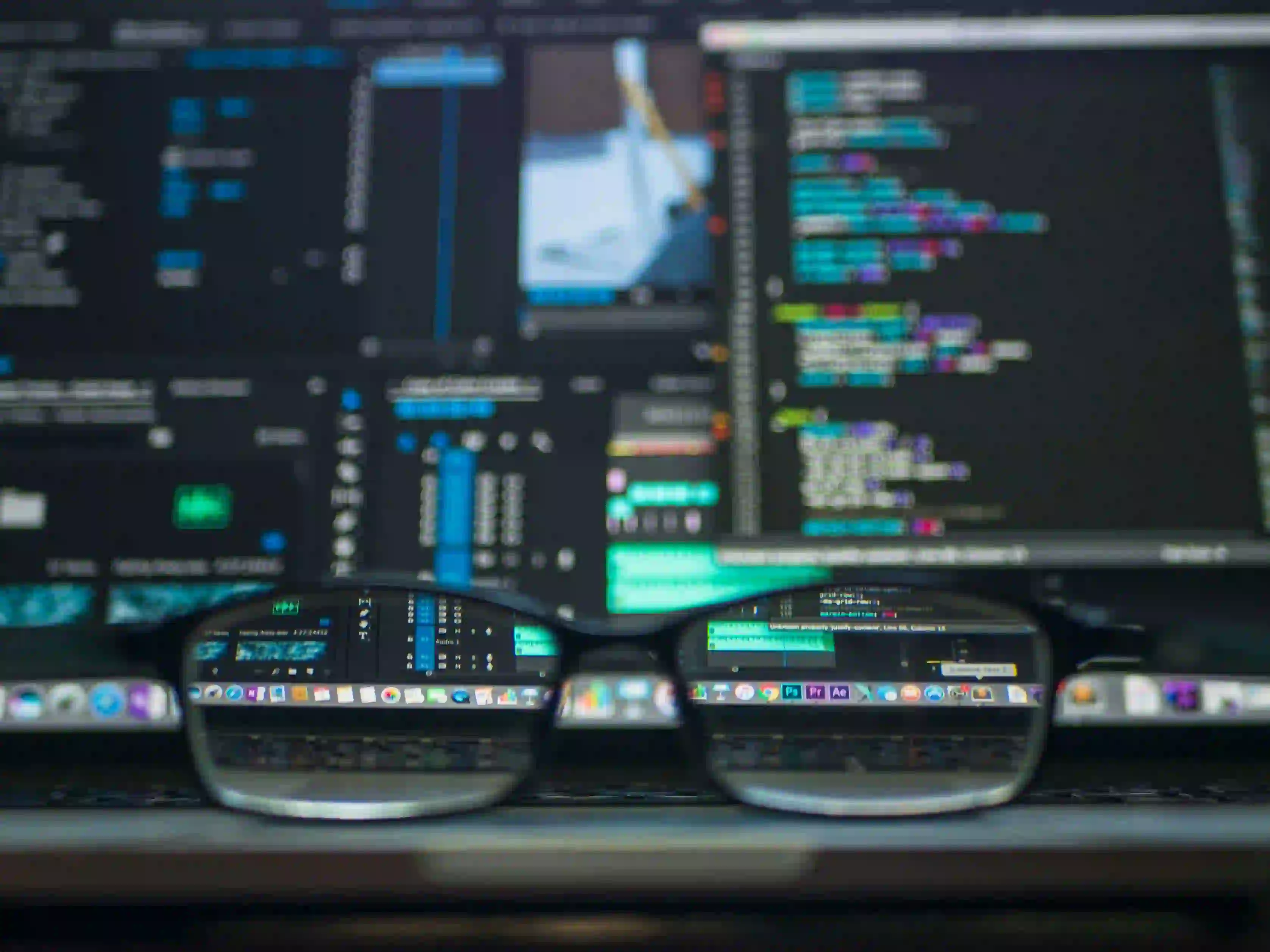
Unlocking Java Concurrency: Fixing Hidden Thread Deadlocks
Java concurrency is a powerful feature that allows multiple threads to execute in tandem, leading to efficient resource utilization and improved application performance. However, the complexities of multithreading can give rise to issues such as thread deadlocks, which can be elusive and challenging to diagnose.
In this blog post, we’ll understand thread deadlocks, identify their causes, and provide effective strategies to fix and prevent them. Whether you are a budding Java developer or a seasoned programmer, this guide aims to enhance your understanding of concurrency in Java.
Table of Contents
- What is a Thread Deadlock?
- How Deadlocks Occur
- Detecting Deadlocks
- Avoiding Deadlocks
- Using Java's Concurrency Utilities
- Conclusion
What is a Thread Deadlock?
A thread deadlock occurs when two or more threads are unable to proceed because each is waiting for the other to release a resource. Imagine two people trying to move around one another while holding tightly onto a single object. In such a scenario, neither can move forward until one relinquishes the hold.
Example of a Deadlock Situation
Consider the following snippet of Java code:
public class DeadlockDemo {
private static final String resource1 = "Resource1";
private static final String resource2 = "Resource2";
public static void main(String[] args) {
Thread thread1 = new Thread(() -> {
synchronized (resource1) {
System.out.println("Thread 1: Holding resource 1...");
try { Thread.sleep(100); } catch (InterruptedException e) { }
synchronized (resource2) {
System.out.println("Thread 1: Acquired resource 2!");
}
}
});
Thread thread2 = new Thread(() -> {
synchronized (resource2) {
System.out.println("Thread 2: Holding resource 2...");
try { Thread.sleep(100); } catch (InterruptedException e) { }
synchronized (resource1) {
System.out.println("Thread 2: Acquired resource 1!");
}
}
});
thread1.start();
thread2.start();
}
}
In this example, Thread 1
locks Resource 1
and waits for Resource 2
, while Thread 2
locks Resource 2
and waits for Resource 1
. The result? A deadlock.
How Deadlocks Occur
Deadlocks generally occur due to the following reasons:
- Mutual Exclusion: A resource can only be held by one thread at a time.
- Hold and Wait: A thread holding at least one resource is waiting to acquire additional resources.
- No Preemption: Resources cannot be forcibly taken from threads.
- Circular Wait: There is a circular chain of threads, each waiting for a resource held by the next thread.
When these conditions are met, a deadlock can occur, leading to significant application performance issues.
Detecting Deadlocks
Deadlocks can be especially hard to identify. However, Java provides tools like Thread Dumps which can help analyze the current state of threads in an application.
To take a thread dump, you can use the following command:
jstack <pid>
Where <pid>
is the process ID of your Java application. The output will show you all the threads and their states, including which threads are blocked and which resources they are waiting for.
Example Output Analysis
"Thread 1" #10 prio=5 os_prio=0 tid=0x0000000001234000 nid=0x4c04 waiting for monitor entry (blocked)
java.lang.Thread.State: BLOCKED (on object monitor)
- waiting to lock 0x000000007f982d80 (a java.lang.String),
which is held by "Thread 2" #11 prio=5 os_prio=0 tid=0x0000000001238000 nid=0x4c05
"Thread 2" #11 prio=5 os_prio=0 tid=0x0000000001238000 nid=0x4c05 waiting for monitor entry (blocked)
java.lang.Thread.State: BLOCKED (on object monitor)
- waiting to lock 0x000000007f982d90 (a java.lang.String),
which is held by "Thread 1" #10 prio=5 os_prio=0 tid=0x0000000001234000 nid=0x4c04
From this output, it's clear that Thread 1
is blocked waiting for a lock held by Thread 2
and vice versa, thereby confirming a deadlock condition.
Avoiding Deadlocks
Preventing deadlocks is often more effective than diagnosing and resolving them after they occur. Here are several strategies to avoid deadlocks:
1. Lock Ordering
Establish a global order in which locks should be acquired. For example, if all threads acquire locks in the order of resource1 and then resource2, the circular wait condition can be avoided.
synchronized (resource1) {
synchronized (resource2) {
// Do something
}
}
2. Timeout on Lock Acquisition
Use a timeout mechanism when attempting to acquire a lock. If a thread cannot acquire the lock within a specified duration, it can back off and try again later.
boolean acquired = lock.tryLock(100, TimeUnit.MILLISECONDS);
if (acquired) {
try {
// Critical Section
} finally {
lock.unlock();
}
}
3. Reduce Lock Scope
Keep the locked section of the code as small as possible. This minimizes the chances of multiple threads acquiring locks in a conflicting manner.
synchronized (resource) {
// Only lock what’s necessary
performAction();
}
Using Java's Concurrency Utilities
The Java Concurrency framework (java.util.concurrent
) provides high-level abstractions to manage threads more efficiently and prevent common pitfalls like deadlocks.
Example: Using ReentrantLock
The ReentrantLock
class not only provides the same capabilities as synchronized
but also includes features that can help avoid deadlocks. This lock allows you to specify an optional timeout when trying to acquire the lock.
import java.util.concurrent.locks.ReentrantLock;
public class LockExample {
private final ReentrantLock lock1 = new ReentrantLock();
private final ReentrantLock lock2 = new ReentrantLock();
public void methodA() {
try {
if (lock1.tryLock(100, TimeUnit.MILLISECONDS)) {
try {
if (lock2.tryLock(100, TimeUnit.MILLISECONDS)) {
try {
// critical section
} finally {
lock2.unlock();
}
}
} finally {
lock1.unlock();
}
}
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
}
}
In this example, methodA
tries to acquire lock1
and, on success, attempts to acquire lock2
. If either lock cannot be acquired, methodA
will back off, helping to prevent a potential deadlock.
Key Takeaways
Concurrency is a vital aspect of Java programming, enabling developers to build high-performance applications. However, with increased power comes increased responsibility; managing concurrency without falling prey to thread deadlocks is critical.
By understanding the causes of deadlocks, employing proper detection mechanisms, and using concurrency utilities such as ReentrantLock
, developers can write robust, deadlock-free Java applications.
For further reading, you may want to consult the official Java Documentation on Concurrency for comprehensive details and advancements.
Keep exploring, and happy coding!