Avoiding Common Pitfalls When Testing with Mockito
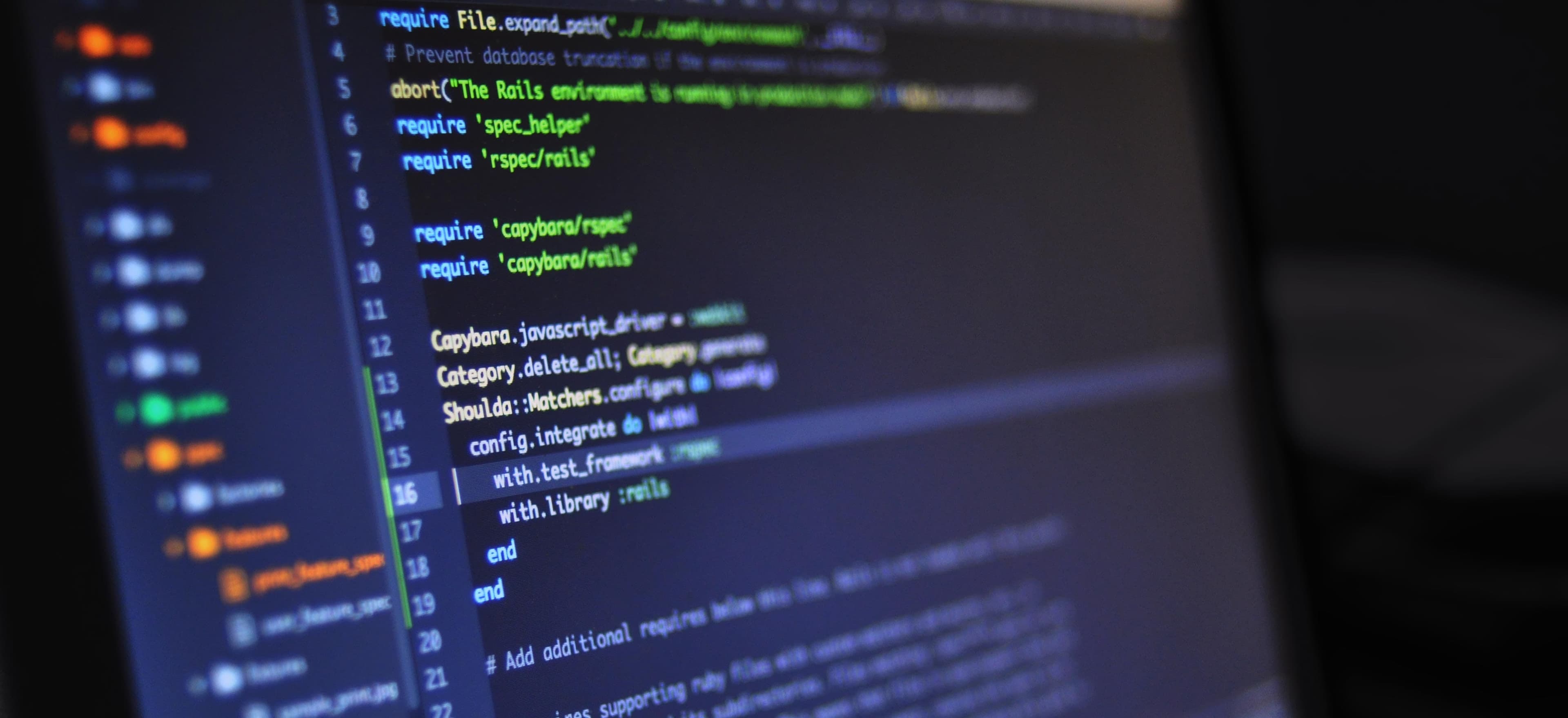
- Published on
Avoiding Common Pitfalls When Testing with Mockito
Mockito is a powerful mocking framework for Java, widely used in unit testing to create test doubles—objects that mimic the behavior of real objects in controlled ways. Its user-friendly syntax and seamless integration with JUnit make it a preferred choice among developers. However, as with any powerful tool, it's easy to fall into common traps that can affect the reliability and maintainability of your tests. In this blog post, we will explore these pitfalls, how to avoid them, and demonstrate some best practices using Mockito.
Why Use Mockito?
Mockito enhances the testing experience by allowing developers to isolate the unit of work. When we decouple our tests from the actual implementation of dependent objects, we open the door to faster execution times and a more focused testing strategy. However, if misused, it can lead to fragile tests that are difficult to debug.
Key Features of Mockito:
- Easy to Use: With its fluent API, creating mocks, stubs, and verifications is straightforward.
- No Need for Boilerplate Code: Mockito reduces the need for excessive setup and teardown code.
- Combines Well with Other Libraries: It works seamlessly with JUnit, TestNG, and other testing libraries.
Common Pitfalls
Now, let's dive into some of the common pitfalls associated with using Mockito and provide solutions to avoid them.
1. Over-Mocking
Problem: Creating mocks for everything can lead to fragile tests that are difficult to maintain. When we mock too many dependencies, we risk losing track of the behavior of the system under test.
Solution: Focus on mocking only the collaborators that have non-trivial behavior, or those whose interactions you want to verify. Use real instances for simple collaborations.
import static org.mockito.Mockito.*;
import static org.junit.Assert.assertEquals;
public class CalculatorTest {
@Test
public void testAdditionWithMockedService() {
// Arrange
MathService mathService = mock(MathService.class);
when(mathService.add(2, 3)).thenReturn(5);
Calculator calculator = new Calculator(mathService);
// Act
int result = calculator.add(2, 3);
// Assert
assertEquals(5, result);
verify(mathService).add(2, 3); // Verify that the called method was indeed invoked
}
}
In this example, we only mock the MathService
which has a defined behavior we want to isolate. This keeps the test focused and relevant.
2. Misunderstanding Verification
Problem: A common mistake is to rely solely on verification. Verification should be used to confirm interactions, not to define the test's success.
Solution: Use assertions to validate the outcomes of your tests. Verification should supplement your assertions, providing a clearer picture of the interaction between components.
public class UserServiceTest {
@Test
public void testRegisterUser() {
// Arrange
UserRepository userRepository = mock(UserRepository.class);
UserService userService = new UserService(userRepository);
User user = new User("John", "Doe");
// Act
userService.register(user);
// Assert
verify(userRepository).save(any(User.class)); // Verification
assertTrue(userService.isUserRegistered(user)); // Assertion on the outcome
}
}
In this snippet, we verify that the repository's save method was called while also asserting that our service behaves as expected.
3. Neglecting Argument Matchers
Problem: Using specific argument values in verifications can lead to brittle tests. If there's a change in the input or behavior, tests can fail unexpectedly.
Solution: Use argument matchers that add flexibility. This way, your tests are more resilient to changes and focus on behaviors rather than strict input.
public class OrderServiceTest {
@Test
public void testProcessOrderWithMatchers() {
// Arrange
PaymentProcessor paymentProcessor = mock(PaymentProcessor.class);
OrderService orderService = new OrderService(paymentProcessor);
Order order = new Order(100);
// Act
orderService.processOrder(order);
// Assert
verify(paymentProcessor).charge(argThat(amount -> amount > 0)); // Flexible matcher
}
}
In this example, we use argThat()
to verify that the method charge is called with a positive amount. This creates resilience against minor changes in the parameters.
4. Ignoring Cleanup
Problem: When tests are not properly cleaned up, it can lead to memory leaks, inconsistencies, or intermittent failures in other tests.
Solution: Use @Before
and @After
annotations to prepare and clean up any state that might persist between tests.
public class DatabaseConnectionTest {
private DatabaseConnection dbConnection;
@Before
public void setUp() {
dbConnection = mock(DatabaseConnection.class);
}
@After
public void tearDown() {
dbConnection = null; // Clearing up the mock
}
@Test
public void testConnect() {
// Implement connection test
}
}
Establishing a clear setup and teardown process ensures that tests do not inadvertently interfere with each other's results.
5. Using Mockito in Production Code
Problem: Mockito should primarily be used in the testing layer. Using Mockito in production code leads to unclear design decisions and possible reliance on mock behaviors at runtime.
Solution: Keep your mocks where they belong: in tests. Design your classes to be test-friendly without relying on Mockito or other mocking frameworks.
Final Thoughts
While Mockito is a powerful tool for unit testing in Java, avoiding common pitfalls ensures that your tests remain effective, reliable, and maintainable. By following the best practices outlined in this blog post, you'll be able to harness the strength of Mockito without falling prey to its vulnerabilities.
With your new understanding, you can confidently leverage Mockito to write robust tests, enhancing your Java applications' quality. For further reading, check out Mockito's official documentation and consider looking into JUnit for a comprehensive testing framework.
Embrace these strategies and avoid the traps of over-mocking, improper verification, and mismanagement of argument matchers. Strong tests lead to strong applications. Happy testing!
Checkout our other articles