Streamline Your Code: Clean Up Equals and ToString Methods
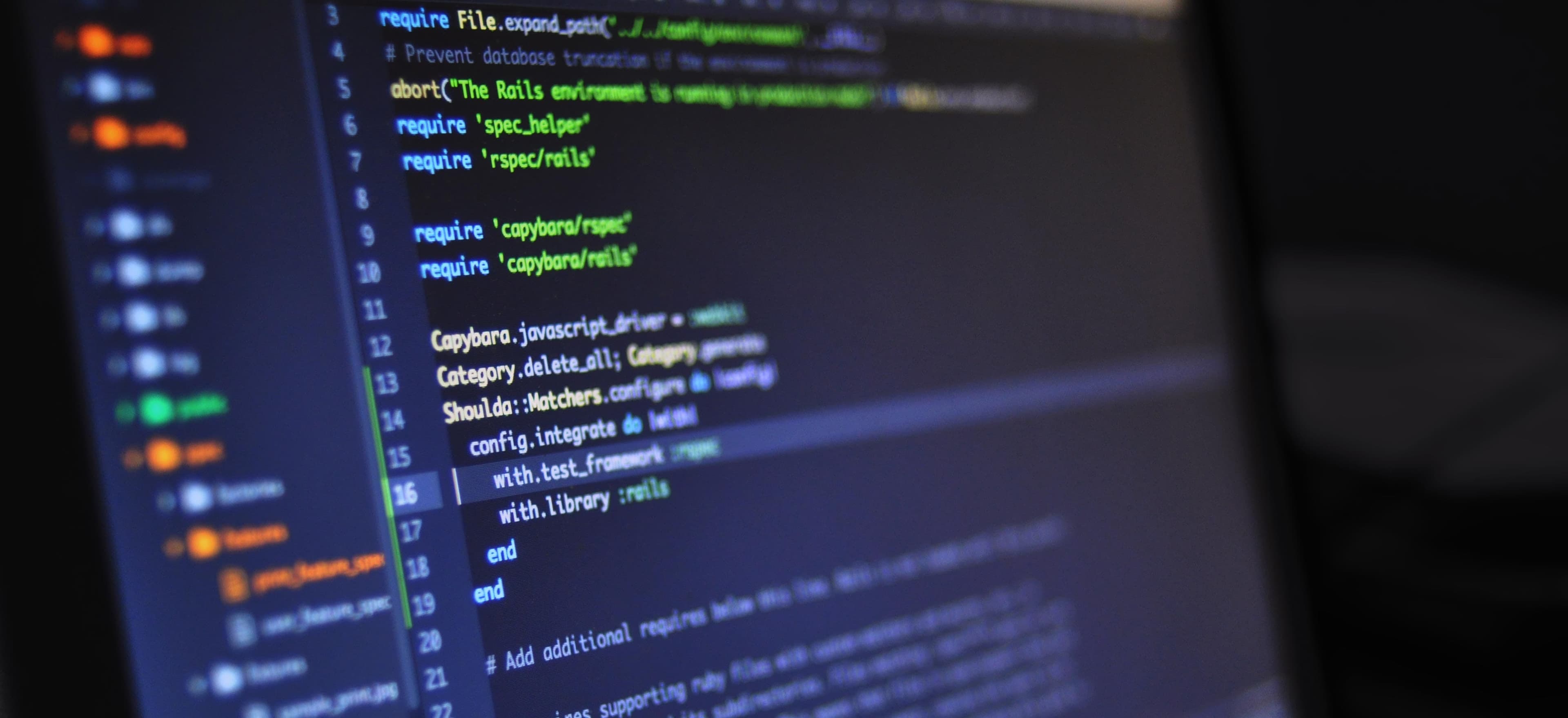
- Published on
Streamline Your Code: Clean Up Equals and ToString Methods in Java
In any Java application, maintaining clean and efficient code is paramount to ensure a smooth development process and ease future updates. Two methods that often require attention are equals()
and toString()
. By refining these methods, you can significantly enhance the readability and functionality of your classes. In this blog post, we will explore best practices for overriding these methods in Java, along with code snippets to illustrate the concepts.
Understanding the Importance of Equals and ToString
Before diving into the implementation, it’s essential to understand why overriding equals()
and toString()
is crucial in Java.
equals()
: More Than Just Value Comparison
The equals()
method determines whether two objects are logically equivalent. By default, the equals()
method in the Object
class compares object references, which isn't useful for most data types. To illustrate:
Object obj1 = new Object();
Object obj2 = new Object();
System.out.println(obj1.equals(obj2)); // false
To compare values, we need to override this method.
toString()
: Human-Readable Representations
The toString()
method provides a string representation of the object. This is especially useful for debugging and logging. By default, it returns the object's class name followed by a hash code, which can be uninformative.
System.out.println(obj1); // Output: java.lang.Object@5ca881b5
Setting the Stage: A Simple Java Class
Let’s consider a simple class, Person
, to illustrate how to cleanly implement these two methods.
public class Person {
private String name;
private int age;
public Person(String name, int age) {
this.name = name;
this.age = age;
}
}
Implementing the Equals Method
To override the equals()
method, follow these steps:
- Check Reference Equality: First check if both references point to the same object.
- Instance Check: Check if the object is an instance of the current class.
- Type Casting: Safely cast the object to the correct type.
- Field Comparison: Compare the relevant fields to establish logical equivalence.
Here’s how the equals()
method looks for the Person
class:
@Override
public boolean equals(Object obj) {
if (this == obj) return true; // Step 1: Check reference equality
if (obj == null || getClass() != obj.getClass()) return false; // Step 2: Instance check
Person person = (Person) obj; // Step 3: Type casting
// Step 4: Field comparison
return age == person.age && (name != null ? name.equals(person.name) : person.name == null);
}
Why Each Step Matters
- Reference Equality Check (Step 1) ensures that you don’t perform unnecessary checks if both object references point to the same instance.
- Instance Check (Step 2) restricts comparisons to instances of the same class, which prevents ClassCastException.
- Type Casting (Step 3) is safe since we know the object is of the appropriate class.
- Field Comparison (Step 4) effectively checks that all meaningful attributes are logically equivalent for two
Person
instances.
Implementing the ToString Method
Now that we have a robust equals()
method, let’s implement a clean toString()
method. The idea here is to return a string that accurately reflects the state of the object in a readable format.
@Override
public String toString() {
return "Person{" +
"name='" + name + '\'' +
", age=" + age +
'}';
}
Why This Format Works
This implementation uses a format that’s easy to read and understand. It clearly states the class and lists its attributes. This format aids in debugging and provides clarity when logging.
Complete Person Class Example
Let’s combine both overridden methods into our Person
class for clarity:
public class Person {
private String name;
private int age;
public Person(String name, int age) {
this.name = name;
this.age = age;
}
@Override
public boolean equals(Object obj) {
if (this == obj) return true;
if (obj == null || getClass() != obj.getClass()) return false;
Person person = (Person) obj;
return age == person.age && (name != null ? name.equals(person.name) : person.name == null);
}
@Override
public String toString() {
return "Person{" +
"name='" + name + '\'' +
", age=" + age +
'}';
}
}
Best Practices for Equals and ToString
Here are some best practices to keep in mind:
- Consistency: If
equals()
returns true for two objects, ensure thathashCode()
is also consistent. - Null Safety: Always check for null values to avoid
NullPointerException
. - Immutability: If possible, make your classes immutable. This simplifies the implementation of
equals()
andtoString()
. - Use Java 7+ Features: Consider using
java.util.Objects
to simplifyequals()
andhashCode()
implementations
@Override
public boolean equals(Object obj) {
if (this == obj) return true;
if (obj == null || getClass() != obj.getClass()) return false;
Person person = (Person) obj;
return age == person.age && Objects.equals(name, person.name);
}
Bringing It All Together
By implementing clean, robust equals()
and toString()
methods, you improve the maintainability and readability of your Java classes. These methods play a significant role in object comparison and display, which are critical in any software application.
Performance and clarity go hand-in-hand in code quality, and with these best practices, your Java applications will become more efficient and understandable.
As you implement these methods in your own projects, remember to think critically about what equality and string representation mean for your specific context. Happy coding!
For more information on equals()
, toString()
, and object-oriented principles in Java, consider checking out Oracle's official documentation.
Checkout our other articles