Troubleshooting Maven Integration Tests in Spring Applications
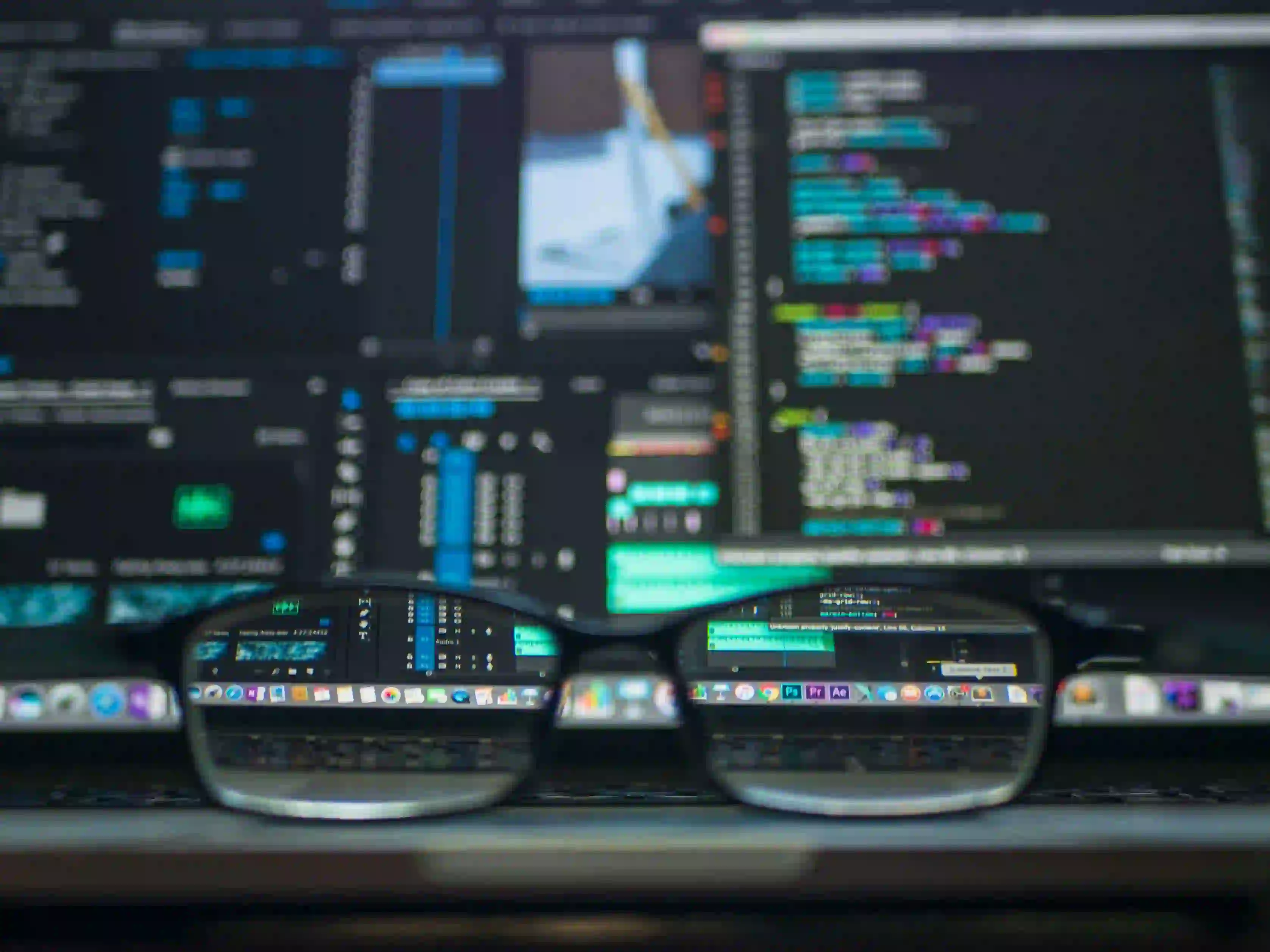
Troubleshooting Maven Integration Tests in Spring Applications
Integration tests are a crucial aspect of any software engineering phase, particularly within Spring applications. These tests aid in validating that different parts of your application work together as expected. However, like any development process, you might encounter issues that can lead to frustrations and wasted time. This blog post will discuss common pitfalls in Maven integration tests for Spring applications and present solutions with clear examples.
Understanding Maven and Spring Integration Tests
Before we delve into troubleshooting, it is essential to understand the interplay between Maven, Spring, and integration tests.
- Maven is a build automation tool used primarily for Java projects, which manages project dependencies, build processes, and lifecycle management.
- Spring Framework is a comprehensive programming and configuration model that simplifies Java development.
- Integration Tests are designed to test the connections between different parts of your application or between your application and external services.
In a Spring application, integration tests are often annotated with @SpringBootTest
, making it easier to test complex modules by loading the entire application context.
Setting Up Your Integration Tests
Basic Example
To illustrate how integration tests can be set up in a Spring application, consider the following code snippet:
@RunWith(SpringRunner.class)
@SpringBootTest
public class MyServiceIntegrationTest {
@Autowired
private MyService myService;
@Test
public void testServiceMethod() {
String result = myService.performAction();
assertEquals("Expected output", result);
}
}
Why This Works:
- The
@RunWith(SpringRunner.class)
annotation enables Spring's testing support. @SpringBootTest
loads the full application context, allowing beans to be autowired.
Common Pitfalls and Troubleshooting Strategies
Despite best efforts, integration tests can fail or behave unexpectedly. Here are some common issues and their solutions.
1. ApplicationContext Load Failures
Symptoms: When running tests, you might encounter errors stating that the ApplicationContext could not be loaded.
Solution: Ensure that the configuration classes or properties files are available. Use the @TestPropertySource
annotation to specify any configurations if necessary. For example:
@TestPropertySource(locations = "classpath:application-test.properties")
public class MyServiceIntegrationTest {
//...
}
Why This Works: It directs the test to use a specific property source during its run. This is crucial in scenarios where default properties do not suffice or lead to misconfigurations.
2. Bean Creation Exceptions
Symptoms: Errors related to bean creation failures can arise, particularly if a required bean is not available.
Solution: Check if all the components are being scanned. If necessary, explicitly specify component scanning using @ComponentScan
.
@SpringBootTest
@ComponentScan(basePackages = "com.example")
public class MyServiceIntegrationTest {
//...
}
Why This Works: It tells Spring where to find your components, ensuring all necessary beans are created.
3. Persistent Storage Issues
Symptoms: Tests that interact with a database sometimes fail due to issues like connection failures or data state problems.
Solution: Leverage an embedded database for testing purposes, such as H2. Configure it in your test properties:
spring.datasource.url=jdbc:h2:mem:testdb
spring.datasource.driverClassName=org.h2.Driver
spring.datasource.username=sa
spring.datasource.password=
spring.h2.console.enabled=true
Why This Works: An embedded database simplifies testing by eliminating the need for a separate database service, thus minimizing the overhead.
4. Dependency Injection Failures
Symptoms: Tests might fail due to null references or instances being unavailable.
Solution: Always ensure that your tests are consistently using Spring’s dependency injection. If you manually instantiate components instead of allowing Spring to inject them, then you’ll encounter null pointer exceptions.
Example for Proper Dependency Injection
@Service
public class MyService {
private final MyRepository myRepository;
@Autowired
public MyService(MyRepository myRepository) {
this.myRepository = myRepository;
}
}
5. Timeout Issues
Symptoms: Integration tests that make network calls (to an external API, for example) often face timeout issues.
Solution: Use mocks for external service calls in tests.
Example Mocking an External Service
Here’s how to mock an external service using Mockito:
@MockBean
private ExternalApiService externalApiService;
@Test
public void testServiceMethodWithMock() {
when(externalApiService.call()).thenReturn("Mocked Response");
String result = myService.performAction();
assertEquals("Expected output", result);
}
Why This Works: Mocking prevents timeouts and allows tests to run swiftly by eliminating real network calls.
6. CI/CD Integration
Symptoms: Sometimes your tests pass locally but fail in a Continuous Integration/Continuous Deployment (CI/CD) environment.
Solution: Ensure that your CI/CD environment is configured correctly with all necessary dependencies, configurations, and permissions. Use tools like Docker for isolated environments to simulate production closely.
Diagnostic Logging
When issues arise, effective logging can be your best friend. Ensure that you have configured logging correctly in your application by using Spring’s logging support or frameworks like Log4j. This will allow you to trace the flow and diagnose problems effectively.
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
@Service
public class MyService {
private static final Logger logger = LoggerFactory.getLogger(MyService.class);
public String performAction() {
logger.info("Executing performAction");
// Method implementation
}
}
Why This Works: Proper logging helps trace issues in production or testing scenarios and provides visibility into the application's behavior.
Wrapping Up
Troubleshooting Maven integration tests in Spring applications can be daunting but is manageable with the right approaches. By understanding common pitfalls and employing best practices, you can ensure that your integration tests are stable, reliable, and provide meaningful validation of your application.
Further Reading
By the end of this journey, you should feel empowered to tackle integration test issues head-on and keep your application robust and reliable. Happy testing!