Maximizing Efficiency: Common Pitfalls in Resource Bundles
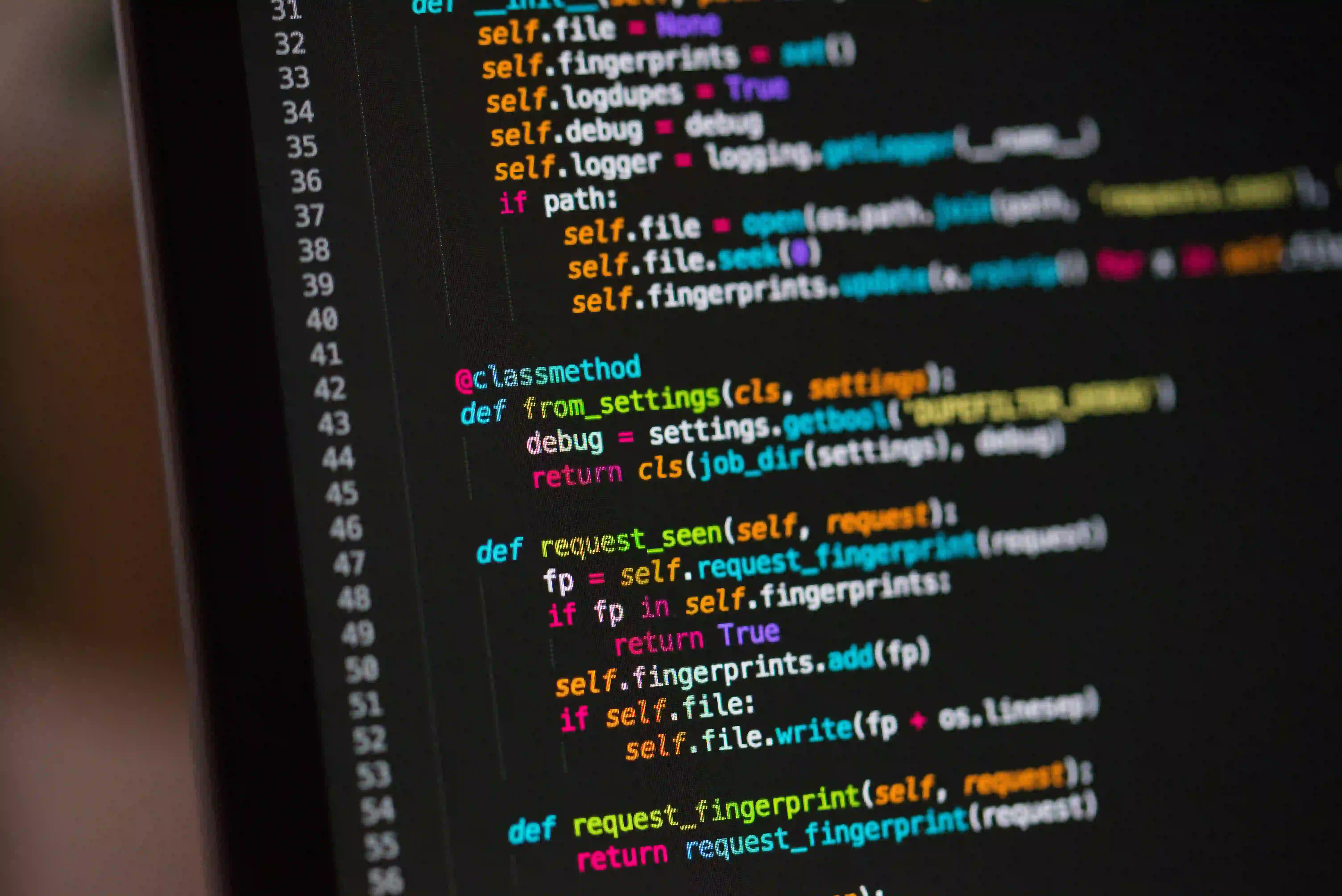
Maximizing Efficiency: Common Pitfalls in Resource Bundles in Java
As developers, we continually seek ways to optimize our applications, and one area that often gets overlooked is resource bundles. Resource bundles are used for internationalization (i18n) and localization (l10n) in Java applications. While they are incredibly powerful, mismanaging them can lead to inefficiencies, performance issues, and increased maintenance costs.
In this article, we will delve into common pitfalls when dealing with Java resource bundles and how to avoid them. We will also discuss best practices to maximize efficiency and maintain clarity in your code. By the end, you should be equipped with actionable insights to help you leverage resource bundles effectively in your Java applications.
Understanding Resource Bundles
Before diving into the pitfalls, it's crucial to understand what resource bundles are. In Java, resource bundles are used to store locale-specific objects, which can include strings, images, or even configuration data. They allow developers to create applications that can adapt to various languages and regions without hardcoding values, making the application more flexible and user-friendly.
Resource bundles in Java are typically implemented using properties files. For instance, you may have a base properties file named messages.properties
and localized versions like messages_fr.properties
for French or messages_de.properties
for German.
Code Example: Basic Resource Bundle Setup
Here’s a simple setup for resource bundles.
import java.util.Locale;
import java.util.ResourceBundle;
public class MessageProvider {
private ResourceBundle resourceBundle;
public MessageProvider(Locale locale) {
resourceBundle = ResourceBundle.getBundle("messages", locale);
}
public String getMessage(String key) {
return resourceBundle.getString(key);
}
public static void main(String[] args) {
MessageProvider messageProvider = new MessageProvider(Locale.FRENCH);
String greeting = messageProvider.getMessage("greeting");
System.out.println(greeting); // Outputs: Bonjour
}
}
In this example, the MessageProvider
retrieves messages based on the provided locale. This showcases the flexibility of resource bundles in adapting the user interface to different languages.
Common Pitfalls
1. Overloading Resource Bundles
One of the biggest mistakes is overloading a single resource bundle with too many keys, making it difficult to manage.
Why It Matters:
- Maintainability: Larger files can lead to confusion in tracking specific keys.
- Performance: Loading an extensive resource bundle may impact performance, especially if keys are not relevant in certain contexts.
Solution:
- Modularize Resource Bundles: Split your resource bundles based on functionality or module. For instance, you might have different properties files for UI messages, error messages, and system messages.
2. Inconsistent Key Naming Conventions
Inconsistent naming conventions can lead to confusion and errors.
Why It Matters:
- Readability: Clear key names increase the readability of your code.
- Accessibility: Developers should quickly understand what each key represents.
Solution:
- Establish and Follow Key Naming Conventions: Use clear, descriptive names for your keys, and follow a consistent style (e.g.,
user.name
,error.notFound
).
3. Neglecting to Include Fallback Mechanisms
Many developers forget to implement fallback mechanisms, which can lead to uncaught errors.
Why It Matters:
- User Experience: Missing translations can leave users confused.
- Error Handling: If a key doesn't exist in the resource bundle, it should gracefully handle the situation.
Solution:
- Implement Fallbacks: Java provides built-in support for fallback mechanisms in resource bundles. If a specific Locale resource bundle is not found, it automatically falls back to the default bundle.
4. Ignoring Performance Implications
Resource bundles can impact performance, especially when they are loaded multiple times.
Why It Matters:
- Loading Time: Repeatedly loading resource bundles can slow down application performance.
- Memory Usage: Each instance of a resource bundle consumes memory.
Solution:
- Cache Resource Bundles: Implement a caching mechanism to ensure that resource bundles are loaded once and reused.
Code Snippet: Caching Resource Bundles
import java.util.HashMap;
import java.util.Locale;
import java.util.Map;
import java.util.ResourceBundle;
public class CachedMessageProvider {
private static Map<Locale, ResourceBundle> cache = new HashMap<>();
public static String getMessage(String key, Locale locale) {
ResourceBundle bundle = cache.computeIfAbsent(locale, loc -> ResourceBundle.getBundle("messages", loc));
return bundle.getString(key);
}
public static void main(String[] args) {
System.out.println(getMessage("greeting", Locale.FRENCH)); // Outputs: Bonjour
}
}
In this snippet, we use a HashMap
to cache resource bundles, ensuring we only load each bundle when necessary.
5. Lack of Proper Documentation
Another pitfall is the absence of thorough documentation for resource bundles.
Why It Matters:
- Onboarding: New developers can struggle to understand the structure and usage of resource bundles.
- Maintenance: Lack of documentation can lead to errors when modifying or adding new entries.
Solution:
- Document Key Usage: Use comments to explain each key's purpose and ensure that your resource files are accompanied by documentation on how to extend or modify them.
6. Not Testing Localizations
Finally, failing to test localizations can lead to missed errors.
Why It Matters:
- User Experience: Badly translated items can hurt your application's reputation.
- Functionality: If strings are too long or too short, they may break UI layouts.
Solution:
- Incorporate Localization Testing: Use tools and frameworks that specialize in testing internationalized applications. Ensure that you test not only the translations but how they impact the overall UI.
Best Practices for Resource Bundles
After discussing the common pitfalls, let's shift to best practices for managing resource bundles effectively:
- Keep Resource Files Organized: Follow a systematic folder structure for different locales.
- Adopt Version Control for Resource Files: Track changes to your resource bundles just like you would with code.
- Use IDE Tools: Most Java IDEs, like IntelliJ IDEA or Eclipse, provide tools for managing properties files. Familiarize yourself with these tools.
- Consider using a Translation Management System (TMS): If your project requires many translations, a TMS can help manage translations efficiently and keep them consistent.
- Regularly Review Keys: Periodically audit your resource files to remove unused keys and update existing ones.
Closing Remarks
Navigating the complexities of resource bundles might seem daunting, but by recognizing common pitfalls and adhering to best practices, you can maximize both performance and maintainability in your Java applications. Remember that proper management of resource bundles not only streamlines development but also enhances user experience across diverse locales. By taking these strategies into account, you are well on your way to creating an efficient and robust application.
For more in-depth reading on Java resource bundles and internationalization, you can check the official Java Documentation or browse through books like Core Java Volume I, which cover internationalization in detail.
Now go forth and build applications that respect and embrace the variety of languages and cultures users bring to the table!