Common Pitfalls When Integrating Spring Cloud with Kubernetes
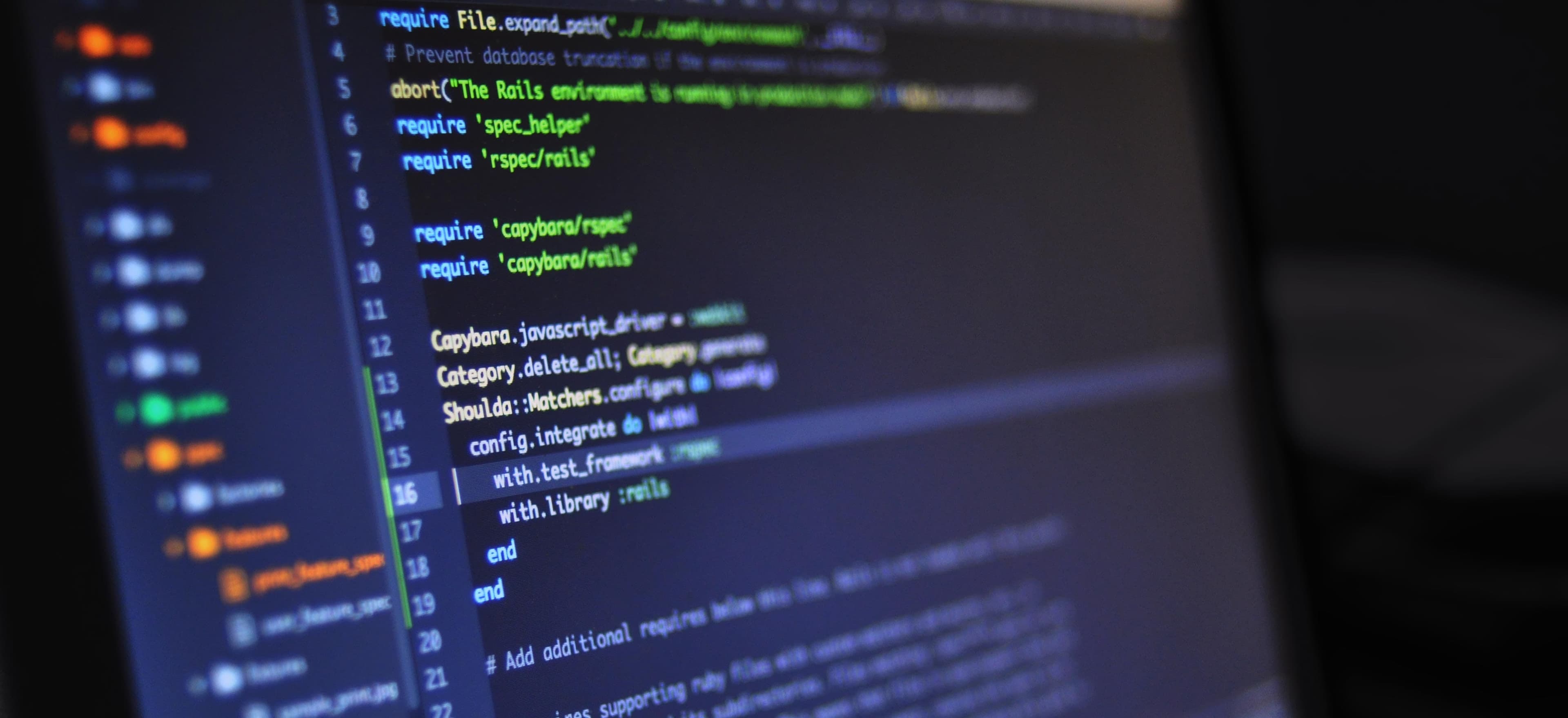
- Published on
Common Pitfalls When Integrating Spring Cloud with Kubernetes
Integrating Spring Cloud with Kubernetes can significantly enhance your microservices architecture by leveraging the power of cloud-native applications. While the benefits are substantial, several common pitfalls can arise during this integration process. In this blog post, we will explore these pitfalls, provide actionable solutions, and share code snippets to help you avoid these issues.
Understanding Spring Cloud and Kubernetes
Before diving into pitfalls, it's essential to understand what Spring Cloud and Kubernetes bring to the table. Spring Cloud simplifies the development of microservices, providing features such as configuration management, service discovery, circuit breakers, and more. Kubernetes, on the other hand, is an orchestration platform that automates the deployment, scaling, and management of containerized applications.
Understanding how these two technologies interact is fundamental to a successful integration.
1. Misconfigured Service Discovery
One of the primary benefits of using Spring Cloud with Kubernetes is service discovery. By default, Spring Cloud Kubernetes uses Kubernetes' built-in service discovery. However, many developers misconfigure their services, leading to communication failures.
Solution
When using Spring Cloud Kubernetes, ensure that the spring.cloud.kubernetes.discovery.enabled
property is set to true
in your application properties.
# application.yml
spring:
cloud:
kubernetes:
discovery:
enabled: true
This property tells your Spring Boot application to look for services in the Kubernetes cluster automatically. If misconfigured, your application may not discover other microservices, hindering communication.
For more detailed configuration, refer to the official Spring Cloud Kubernetes documentation.
2. Ignoring Health Checks
Health checks are vital in Kubernetes. A common pitfall is neglecting to implement proper health checks in your Spring applications. While Spring Boot offers actuator endpoints for health monitoring, these need to be configured correctly.
Solution
Ensure you expose proper health endpoints by including the Spring Actuator dependency and configuring it in your pom.xml
:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-actuator</artifactId>
</dependency>
Next, modify your application.yml
to enable health checks:
management:
endpoints:
web:
exposure:
include: health, info # Expose health and info endpoints
Kubernetes can then utilize these endpoints for periodic health checks, which will restart unhealthy pods automatically. For additional information on health endpoints, check out the Spring Boot Actuator documentation.
3. Overlooking Configuration Management
In a cloud environment, configuration management can become tedious if not automated. Spring Cloud Config Server is typically used for centralized configuration management. However, in a Kubernetes environment, many developers overlook it or misconfigure the configuration provider.
Solution
Utilize Kubernetes ConfigMaps to manage configurations. This reduces files in your repository and keeps sensitive data hidden. Here’s a basic example of using a ConfigMap in Kubernetes:
apiVersion: v1
kind: ConfigMap
metadata:
name: my-config
data:
application.properties: |
spring.datasource.url=jdbc:mysql://mysql:3306/mydb
Ensure that your Spring application is designed to pull configuration from Kubernetes. You can do this by modifying the bootstrap.yml
or application.yml
:
spring:
cloud:
kubernetes:
config:
enabled: true
For more details on managing configuration with Spring Cloud Kubernetes, refer to the Spring Cloud Kubernetes Config documentation.
4. Not Utilizing Load Balancer Properly
Kubernetes offers several types of services, including LoadBalancer, NodePort, and ClusterIP. Overlooking this can lead to undesired availability and accessibility of your services.
Solution
When defining your services, carefully select the right service type based on your requirements. Here is an example of a LoadBalancer service definition:
apiVersion: v1
kind: Service
metadata:
name: my-service
spec:
type: LoadBalancer
ports:
- port: 80
targetPort: 8080
selector:
app: my-app
Ensure that your application is reachable, especially when running in production.
5. Lack of Proper Resource Limits
Kubernetes is designed to ensure that applications utilize resources efficiently. However, many developers skip defining resource limits for their Spring microservices, which can lead to resource contention.
Solution
Make it a standard practice to define resource limits for your microservices. Here’s how you can configure it in your Deployment YAML:
apiVersion: apps/v1
kind: Deployment
metadata:
name: my-app
spec:
template:
spec:
containers:
- name: my-app
image: my-app-image
resources:
requests:
memory: "512Mi"
cpu: "500m"
limits:
memory: "1Gi"
cpu: "1"
Setting proper resource requests and limits ensures that your application can scale efficiently and avoids resource starvation.
6. Ignoring Logs and Monitoring
In a microservices architecture, monitoring and logging cannot be overlooked. Some developers tend to focus solely on application functionality and miss logging important events, which can prove detrimental for troubleshooting.
Solution
Integrate distributed tracing and logging tools like Zipkin or ELK stack (Elasticsearch, Logstash, Kibana). You may need to set up environment variables and additional configurations:
spring:
sleuth:
sampler:
probability: 1.0 # Sample all requests for tracing
By implementing monitoring, you can gain insights into the performance and health of your application, making debugging easier.
Closing the Chapter
While integrating Spring Cloud with Kubernetes can propel your microservices development into a more efficient realm, being mindful of common pitfalls ensures that your applications run smoothly and reliably. By addressing misconfigurations, health checks, configuration management, load balancing, resource limits, and monitoring, your integration efforts will yield higher performance and improved maintainability.
If you want to dive deeper into these technologies, consider visiting the official documentation for Spring Cloud and Kubernetes.
By avoiding these common pitfalls, you set the stage for a robust and cloud-native microservices solution. Happy coding!
Checkout our other articles