Unveiling the Challenges of Java 20 Panama API Integration
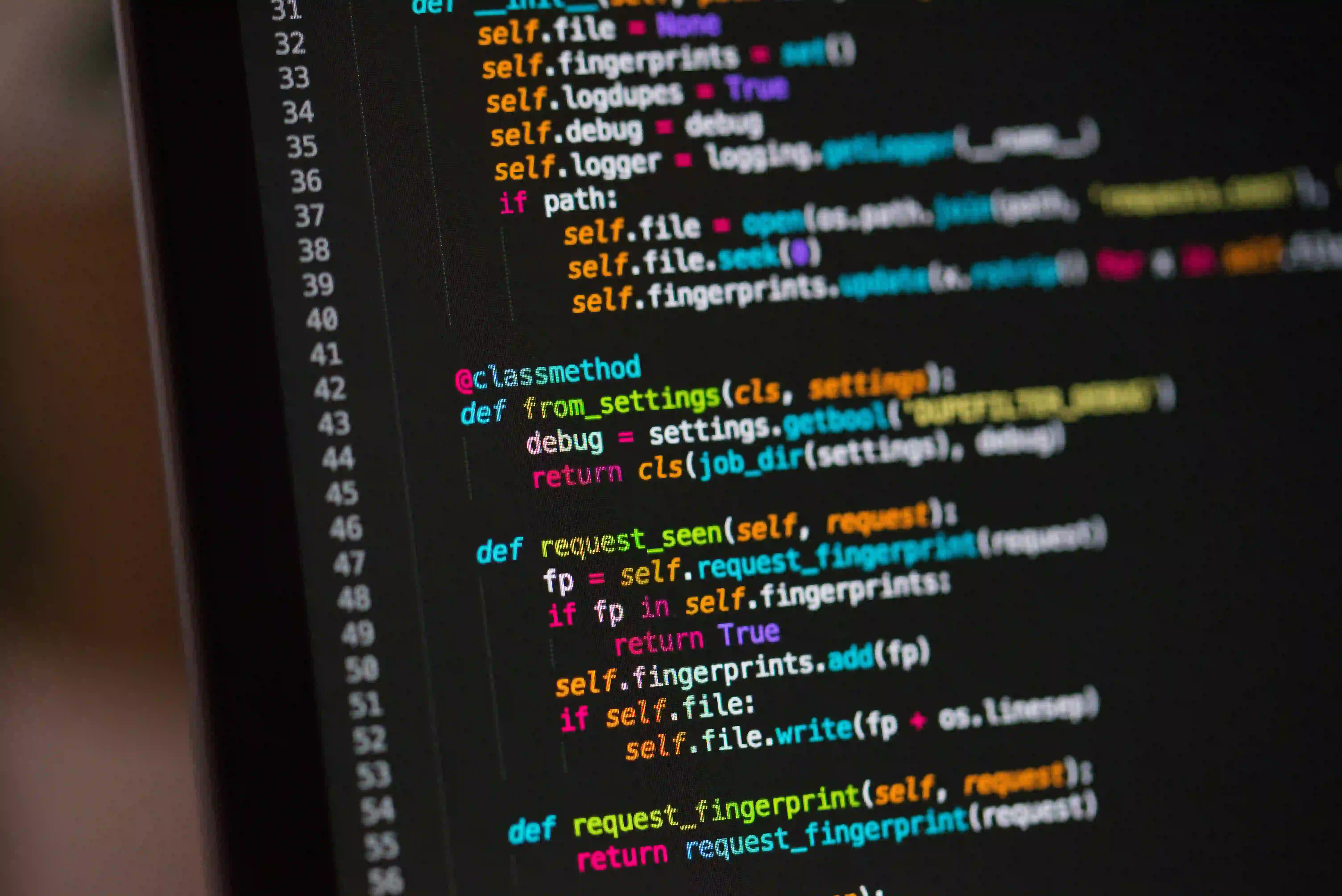
Unveiling the Challenges of Java 20 Panama API Integration
Java has long been a staple in the programming world, celebrated for its portability, efficiency, and extensive libraries. With every new release, the language introduces features aimed at enhancing performance, improving developer experience, and expanding its capabilities. One of the most anticipated features in Java 20 is the Panama API—a revolutionary integration designed to facilitate seamless interaction between Java and native code libraries. While its promise is impressive, the integration of the Panama API is not without challenges.
In this blog post, we will explore the benefits and challenges of using the Java 20 Panama API, provide practical code snippets to illustrate key concepts, and offer insights that developers should keep in mind when adopting this new feature.
What is the Panama API?
The Panama API, part of Project Panama, is an initiative to improve the interaction between Java and native languages like C and C++. This API allows Java programs to interact with native libraries without the overhead typically associated with Java's native interface (JNI). This direct interaction paves the way for running performance-critical applications with native code while still leveraging Java's platform independence.
Key Features of the Panama API
- Simplified Native Calls: The Panama API reduces the complexity of invoking native libraries.
- Performance Gains: By allowing closer interaction with native code, programs can achieve better performance than traditional JNI calls.
- Safety and Usability: The API incorporates safety features minimizing the likelihood of errors, making it more user-friendly.
For a deeper dive into project Panama, you can check out the OpenJDK Panama project page.
Understanding the Challenges
While the Panama API offers several advantages, integrating it into existing Java applications comes with its challenges:
1. Learning Curve
The Panama API introduces new concepts and methodologies that developers must grasp to utilize its full potential. Java developers familiar only with Java methods will require time and practice to become proficient in native integration.
2. Performance Overhead
Although the Panama API is designed for better performance than JNI, certain scenarios can lead to unexpected performance overhead. For example, passing complex data structures between Java and C can introduce inefficiencies.
3. Compatibility Issues
Java 20 being relatively new means that not all libraries may be fully compatible with the Panama API. Developers might encounter issues with legacy codebases or existing native libraries.
4. Debugging Difficulty
Debugging native code is notoriously challenging. When problems arise in the integration between Java and native libraries, tracing the issue can be complicated, especially if tools for debugging are not readily available.
Getting Started with the Panama API
To illustrate how to work with the Panama API, let’s look at a basic example demonstrating how to invoke a native C function from Java.
Setting Up Your Environment
Assuming Java 20 is installed, you also need to have the following:
- A compatible C compiler (e.g., GCC).
- Your native library (e.g.,
libexample.so
) compiled from C code.
Writing a Simple C Function
Let’s create a simple C function that adds two integers:
// example.c
#include <stdio.h>
int add(int a, int b) {
return a + b;
}
Compile this code into a shared library:
gcc -shared -o libexample.so -fPIC example.c
Java Code with Panama API
Now, let’s write the Java code to invoke this native C function using the Panama API.
import jdk.incubator.foreign.*;
import jdk.incubator.foreign.CLinker;
import static jdk.incubator.foreign.MemoryLayout.*;
import static jdk.incubator.foreign.ValueLayout.*;
import static jdk.incubator.foreign.CLinker.*;
public class NativeExample {
private static final MemoryAddress LIBRARY_PATH = MemoryAddress.ofInt(0); // Update with the correct path to your library
public static void main(String[] args) {
// Load the native library
System.load(LIBRARY_PATH.toString());
// Get the symbol from the native library
SymbolLookup symbolLookup = CLinker.systemLookup();
MemoryAddress addAddress = symbolLookup.find("add").orElseThrow();
// Create a method handle for the native function
MethodHandle addHandle = CLinker.getInstance().downcallHandle(
addAddress,
CLinker.C_INT,
CLinker.C_INT, CLinker.C_INT
);
// Call the native function
try {
int result = (int) addHandle.invoke(5, 3);
System.out.println("Result of native add function: " + result);
} catch (Throwable e) {
e.printStackTrace();
}
}
}
Commentary
-
Loading the Library: Ensure the correct path is set for the native library. Using
System.load()
, we load the shared library into the application’s runtime. -
Finding the Symbol: The
symbolLookup
finds our C functionadd
defined earlier. If the function is missing, it throws an exception, preventing the program from proceeding unexpectedly. -
Creating a Method Handle: The
downcallHandle
method links to the native function, establishing the expected return type and parameters. The usage ofMethodHandle
simplifies calling the native method. -
Executing the Function: Finally, we invoke the native function and print the result. Error handling is crucial here, as exceptions can arise during invocation.
Best Practices for Panama API Integration
As you venture into using the Panama API, consider these best practices:
-
Incremental Adoption: Start by integrating small parts of native functionality and gradually expand. This approach allows for smoother debugging and reduces the risk of significant issues.
-
Thorough Documentation: Maintain clear documentation of native interactions and expected data formats to ease future updates or debugging.
-
Profiling Performance: Regularly profile your application to identify performance bottlenecks that may arise due to native interactions.
-
Community Engagement: Engage with the Java community. Platforms like Stack Overflow or the Java mailing lists can provide valuable insights and support.
Lessons Learned
The Panama API in Java 20 presents a powerful tool for developers looking to optimize performance through native integrations. However, as highlighted in this post, there are challenges to be aware of, including the learning curve, performance overhead, and debugging complexities. By understanding these challenges and following best practices for integration, developers can harness the full potential of the Panama API.
The future looks promising with Project Panama changing how Java interacts with native code. Embrace the journey, and keep exploring the capabilities Java provides.
For further reading, be sure to check out the Java 20 Release Notes and Project Panama's documentation. Happy coding!