Taming Coupling Issues in Local Microservices Architecture
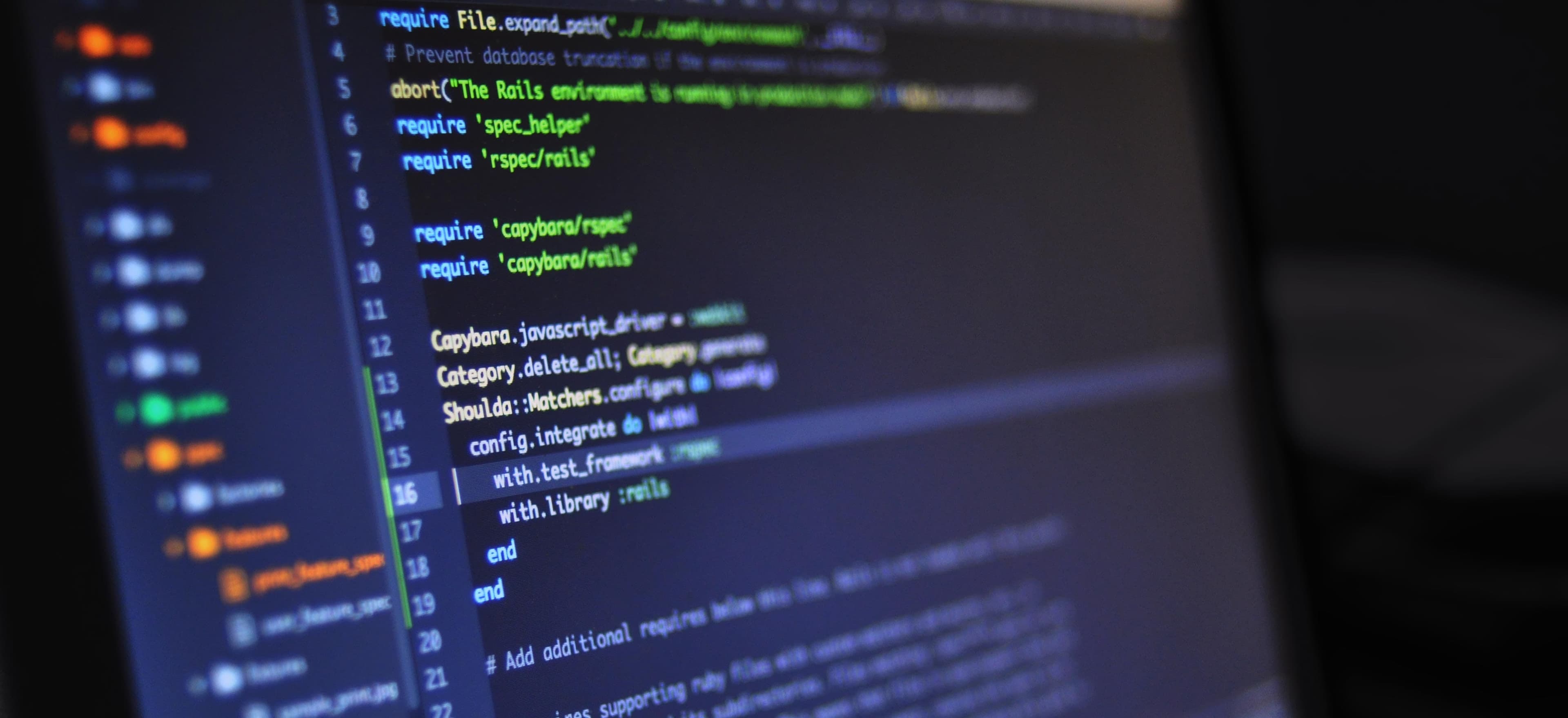
- Published on
Taming Coupling Issues in Local Microservices Architecture
Microservices architecture has become increasingly popular in the software development landscape due to its ability to provide scalability, flexibility, and maintainability. However, as organizations embrace this paradigm, they often face significant challenges, particularly regarding coupling between services. In this blog post, we will explore what coupling is, its implications in microservices, and strategies to mitigate it effectively.
Understanding Coupling in Microservices
At its core, coupling refers to the degree of interdependence between software modules. In a microservices architecture, each service is intended to be an independent unit, but high coupling can undermine this independence, leading to complexities and challenges such as:
- Reduced Flexibility: Changes in one service might require changes in others, which can slow down the deployment pipeline.
- Increased Complexity: Debugging becomes challenging since issues may arise from interactions between tightly-coupled services.
- Poor Resilience: If one service fails, it may cascade, impacting others that depend on it.
This is a critical concept that everyone transitioning to microservices should understand.
Types of Coupling
Understanding the types of coupling can help pinpoint the specific challenges you might face. Here are three common types:
-
Tight Coupling: Services are highly dependent on each other, often sharing data models or invoking each other directly. This increases the fragility of the system.
-
Loose Coupling: Services operate independently, with minimal dependencies. They communicate through well-defined interfaces or APIs, enhancing resilience.
-
Event-driven Coupling: Services communicate through events, allowing asynchronous interactions and facilitating scalability.
Example of Tight Coupling vs. Loose Coupling
In tight coupling, service A might directly call service B's methods, exposing it to changes in service B:
// Tight Coupling Example
public class ServiceA {
private final ServiceB serviceB;
public ServiceA(ServiceB serviceB) {
this.serviceB = serviceB;
}
public void process() {
serviceB.performAction(); // Direct dependency
}
}
In contrast, with loose coupling, service A publishes an event that service B listens for:
// Loose Coupling Example
public class ServiceA {
private final EventPublisher eventPublisher;
public ServiceA(EventPublisher eventPublisher) {
this.eventPublisher = eventPublisher;
}
public void process() {
// Instead of calling service B directly,
// we'll publish an event
eventPublisher.publish(new ActionPerformedEvent());
}
}
In the second example, service A does not need to know the specifics of how service B performs its action.
Strategies for Reducing Coupling
1. Utilize APIs
One of the primary methods of achieving loose coupling in microservices is through well-defined APIs.
- RESTful APIs: Using HTTP for inter-service communication, they follow standard conventions, making it easier for services to evolve independently.
- gRPC: This lightweight framework offers efficient communication between services and may provide performance benefits over REST.
2. Adopt Asynchronous Communication
Instead of synchronous calls where services wait for responses, asynchronous communication promotes decoupling.
- Message Queues: Integrate message brokers like RabbitMQ or Apache Kafka to facilitate message passing, which can enhance resiliency and performance.
Code Snippet: Using RabbitMQ
import com.rabbitmq.client.*;
public class MessagePublisher {
private static final String QUEUE_NAME = "task_queue";
public void sendMessage(String message) throws IOException {
ConnectionFactory factory = new ConnectionFactory();
factory.setHost("localhost");
try (Connection connection = factory.newConnection();
Channel channel = connection.createChannel()) {
channel.queueDeclare(QUEUE_NAME, true, false, false, null);
channel.basicPublish("", QUEUE_NAME, null, message.getBytes());
System.out.println(" [x] Sent '" + message + "'");
}
}
}
3. Embrace Domain-Driven Design (DDD)
Domain-Driven Design encourages encapsulating the data and behavior relevant to a specific domain within a service. This practice can help clearly define boundaries between services, reducing the chances of tight coupling.
- Identify and create bounded contexts that delineate service responsibilities.
- Implement clear interfaces at the boundaries of services to ensure they communicate effectively yet independently.
4. Service Discovery & Load Balancing
Implementing a service discovery mechanism can support flexibility and adaptability in your system architecture. By using tools like Eureka or Consul, services can find and communicate with each other without relying on hardcoded IP addresses or hostnames. This not only reduces coupling but also allows for dynamic adjustments to service locations.
5. Circuit Breaker Pattern
A robust system is one that can handle failures gracefully. The circuit breaker pattern allows a service to fail fast and return an error rather than waiting for a slow response, thereby preventing systemic failures. Frameworks like Netflix Hystrix or Resilience4j enable you to implement this pattern effectively.
In Conclusion, Here is What Matters
Taming coupling issues in local microservices architecture is a critical undertaking, one that can significantly affect the scalability and maintainability of your application. By embracing APIs, asynchronous communications, DDD, service discovery, and circuit breakers, you can foster a more resilient architecture.
If you are looking for additional reading on microservices architecture and reducing coupling, check out Martin Fowler's microservices article and the 12-factor app methodology, which provides powerful principles for building modern, scalable applications.
By understanding and applying the strategies highlighted in this post, you can ensure that your microservices are decoupled, resilient, and ready to face the challenges of today's rapidly changing software landscape.
Checkout our other articles