Common Pitfalls When Packaging React Apps with Spring Boot
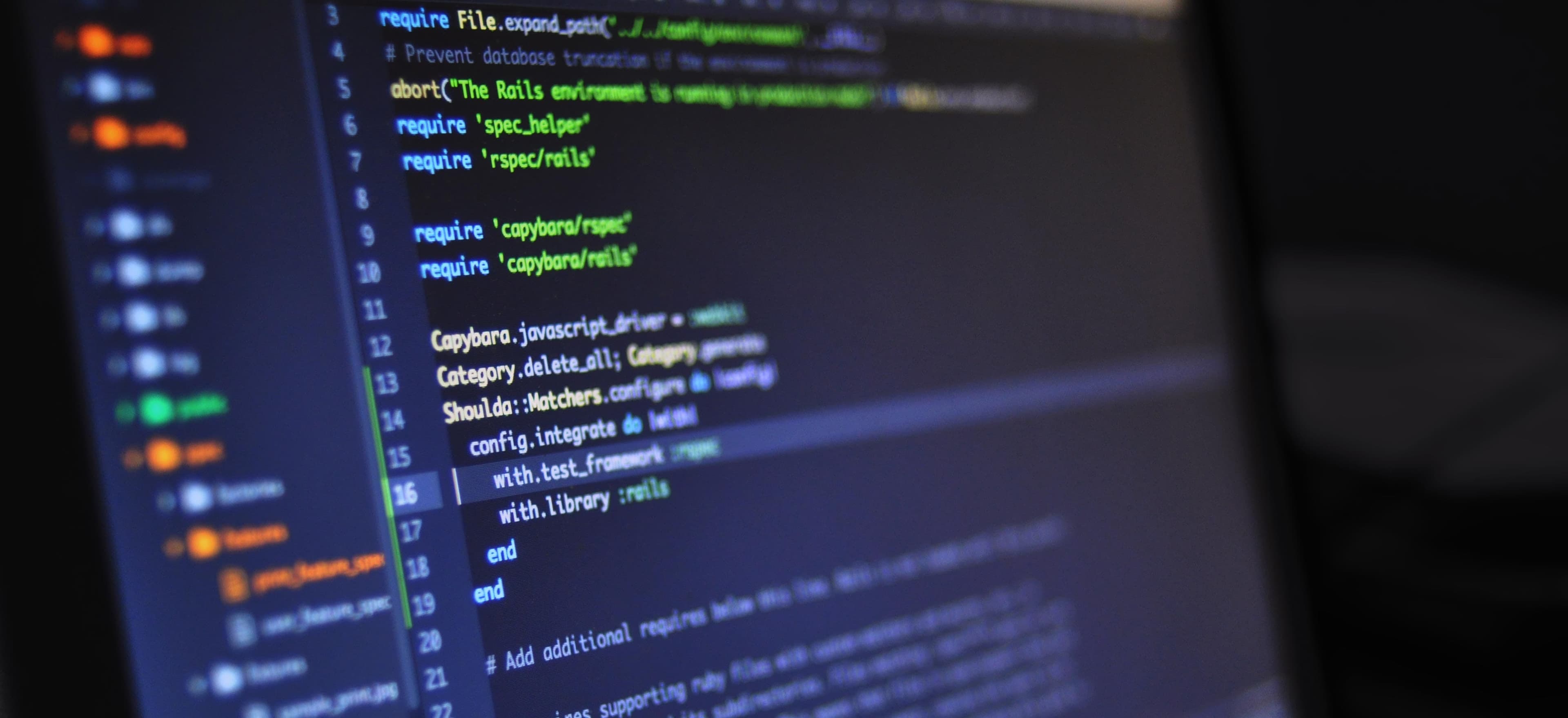
- Published on
Common Pitfalls When Packaging React Apps with Spring Boot
Combining React and Spring Boot has become a popular approach for full-stack development. While both technologies boast significant advantages on their own, integrating them can often present unique challenges. In this article, we will explore common pitfalls you might face when packaging React apps with Spring Boot, and, more importantly, how to avoid them.
Understanding the Basics
Before diving into pitfalls, it's essential to understand the architecture of a React-Spring Boot application. At its core, React serves as the frontend library responsible for rendering UI components, while Spring Boot acts as the backend framework, providing RESTful APIs and handling business logic.
Typical Project Structure
A well-organized project structure might look like this:
my-app/
│
├── backend/
│ ├── src/
│ ├── pom.xml
├── frontend/
│ ├── src/
│ ├── package.json
Here, backend
holds your Spring Boot project, while frontend
contains your React application. This separation of concerns is crucial for maintainability and ease of development.
Common Pitfalls
1. Confusing the Build Processes
One of the most prevalent issues developers face is misunderstanding the separate build processes for React and Spring Boot.
Why This Matters
Each application has its own build mechanism: Yarn or NPM for React, and Maven or Gradle for Spring Boot. If not handled correctly, it can lead to complexities during deployment.
Solution
Make sure to streamline the build processes. For instance, you can use a multi-stage Docker build to facilitate seamless integration:
# Stage 1: Build the React app
FROM node:14 AS react-build
WORKDIR /frontend
COPY frontend/package.json ./
RUN npm install
COPY frontend/ ./
RUN npm run build
# Stage 2: Package with Spring Boot
FROM openjdk:11
WORKDIR /app
COPY --from=react-build /frontend/build /app/static
COPY backend/target/backend.jar ./
ENTRYPOINT ["java", "-jar", "backend.jar"]
This Dockerfile clearly separates the build of the frontend and backend, ensuring that the final image contains the built React app served by Spring Boot.
2. API Endpoint Conflicts
When both apps run on the same server, API endpoint conflicts can arise, especially if both the frontend and backend are hosted on the same domain.
Why This Matters
If both React and Spring Boot make similar routes, like /api
, it can lead to unexpected behaviors.
Solution
To avoid endpoint conflicts, configure your application.properties
in Spring Boot to set a base path for your REST endpoints:
server.port=8080
server.servlet.context-path=/api
Then, make sure your React app is aware of this change, leading to cleaner API calls:
fetch('/api/users')
.then(response => response.json())
.then(data => console.log(data));
3. CORS Issues
Cross-Origin Resource Sharing (CORS) issues frequently pop up when your React app communicates with the Spring Boot backend.
Why This Matters
Browsers implement CORS to prevent requests from potentially harmful sources. If your frontend and backend run on different ports during development, without proper CORS configurations, you may face unexpected rejections of your requests.
Solution
In your Spring Boot application, you can easily enable CORS for specific endpoints using the @CrossOrigin
annotation:
@RestController
@CrossOrigin(origins = "http://localhost:3000")
public class UserController {
@GetMapping("/api/users")
public List<User> getUsers() {
return userService.getAllUsers();
}
}
This solution allows your React frontend to call the backend API without encountering CORS issues.
4. Ignoring Build Optimizations
When deploying your application, skipping optimizations can lead to slow load times and a poor user experience.
Why This Matters
A poorly optimized build will not only increase the size of your application but can drastically slow page loads. Users expect fast and responsive applications.
Solution
Make sure to run the React build in production mode:
npm run build
This command creates an optimized build for deployment in the build
directory.
In your Spring Boot application, also ensure that the static files are properly served:
@SpringBootApplication
public class MyApplication {
public static void main(String[] args) {
SpringApplication.run(MyApplication.class, args);
}
}
5. Mismanaging Environment Configurations
Not properly managing environment variables can lead to confusion and mistakes, especially when handling multiple environments (development, staging, production).
Why This Matters
Environment variable management is crucial for separating settings such as API URLs, database connections, and any sensitive data.
Solution
You can use .env
files for your React application, alongside handling the application.properties
or application.yml
for Spring Boot. For example, the .env
file may look like:
REACT_APP_API_URL=http://localhost:8080/api
Then in your React app, you can access this variable like so:
const apiUrl = process.env.REACT_APP_API_URL;
fetch(`${apiUrl}/users`)
.then(response => response.json())
.then(data => console.log(data));
For Spring Boot, keep your sensitive data secure, using tools like Vault or AWS Secrets Manager.
Lessons Learned
Integrating React with Spring Boot can be a rewarding journey, but it's vital to navigate the common pitfalls that can arise during the process. By paying attention to build processes, endpoint management, CORS configuration, optimization strategies, and environment handling, you can ensure a smoother development and deployment experience.
Additional Resources
For further reading, consider checking out:
By following the guidelines and solutions discussed in this article, you can streamline your workflow and create a robust, full-stack application that delights both developers and users. Happy coding!
Checkout our other articles