Troubleshooting Mutual TLS Issues in Java Connections
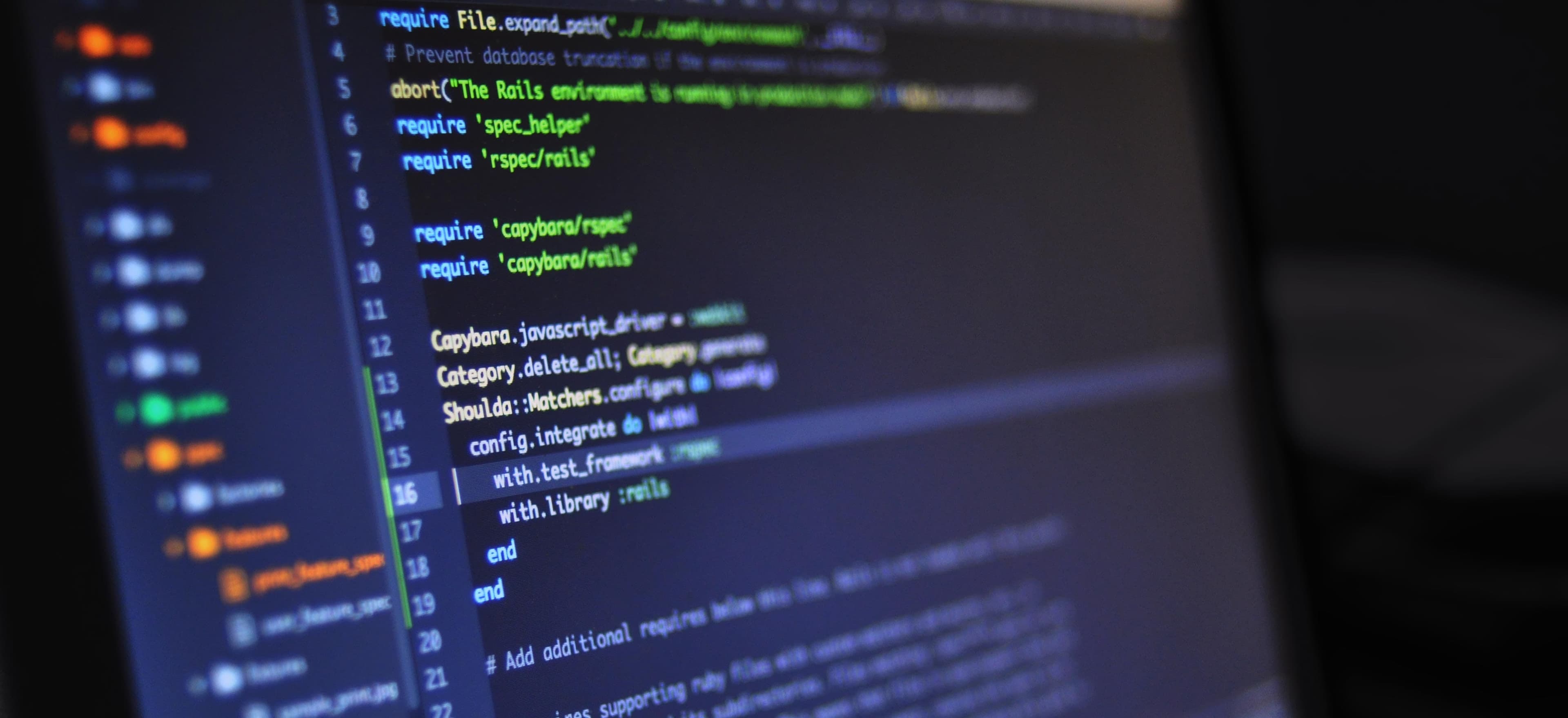
- Published on
Troubleshooting Mutual TLS Issues in Java Connections
A Brief Overview
Mutual TLS (mTLS) is an extension of the TLS (Transport Layer Security) protocol that enforces two-way authentication between a client and server. This means both parties verify the other's identity using digital certificates. While mTLS enhances security, it can also introduce a range of complexities that developers often need to navigate. In this blog post, we’ll explore common issues related to mTLS when building applications in Java, and provide troubleshooting strategies to help ensure seamless connections.
Table of Contents
- Understanding Mutual TLS
- Common Issues in mTLS
- Setting Up Java with mTLS
- Troubleshooting Steps
- Conclusion
Understanding Mutual TLS
Before diving deep into troubleshooting, it's crucial to understand how mTLS works.
- Server Authentication: The server presents its certificate to the client to prove its identity.
- Client Authentication: The client presents its certificate to the server, which verifies the client's identity.
In Java, these interactions typically involve the use of the javax.net.ssl
package. The client and server need their respective keystores for their certificates, and truststores for trusted certificate authorities (CAs).
For a comprehensive overview of certificates and keystores, check out the [Oracle Documentation on Key and Certificate Management](https://docs.oracle.com/javase/8/docs/technotes/guides/security/crypto/Crypto Spec.html).
Common Issues in mTLS
While implementing or connecting via mTLS, you might encounter various issues, such as:
- Certificate Issues: Expired, self-signed, or incorrectly issued certificates.
- Truststore Configuration: Missing or incorrect truststore configurations in your application.
- Keystore Problems: Issues with loading keystores or key passwords.
- Firewall Settings: Network-related issues blocking specific ports.
- Version Mismatches: Mismatches in TLS versions between client and server.
Certificate Issues
One of the first things to check is the validity of the certificates. An expired certificate will lead to connection failures. Always ensure you are working with current and valid certificates.
Truststore Configuration
The truststore must contain a valid certificate for the server the client is attempting to connect to. This often becomes an oversight when setting up the mTLS.
Keystore Problems
If the keystore cannot be loaded, the Java application will not authenticate properly. Always double-check the file path and password of the keystore.
Setting Up Java with mTLS
To set up a Java application for mTLS, you need to configure the key managers and trust managers properly. Below is an example of how organization code might look:
Example Code Snippet for mTLS in Java
import javax.net.ssl.*;
import java.io.FileInputStream;
import java.security.KeyStore;
public class MTLSClient {
public static void main(String[] args) throws Exception {
// Load Client KeyStore
KeyStore clientStore = KeyStore.getInstance("PKCS12");
clientStore.load(new FileInputStream("client-keystore.p12"), "client-password".toCharArray());
// Load TrustStore
KeyStore trustStore = KeyStore.getInstance("JKS");
trustStore.load(new FileInputStream("truststore.jks"), "truststore-password".toCharArray());
// Create KeyManagerFactory
KeyManagerFactory keyManagerFactory = KeyManagerFactory.getInstance(KeyManagerFactory.getDefaultAlgorithm());
keyManagerFactory.init(clientStore, "client-password".toCharArray());
// Create TrustManagerFactory
TrustManagerFactory trustManagerFactory = TrustManagerFactory.getInstance(TrustManagerFactory.getDefaultAlgorithm());
trustManagerFactory.init(trustStore);
// Create SSLContext
SSLContext sslContext = SSLContext.getInstance("TLS");
sslContext.init(keyManagerFactory.getKeyManagers(), trustManagerFactory.getTrustManagers(), null);
// Create SSLSocketFactory
SSLSocketFactory sslSocketFactory = sslContext.getSocketFactory();
SSLSocket sslSocket = (SSLSocket) sslSocketFactory.createSocket("server-address", 443);
// Start handshake
sslSocket.startHandshake();
System.out.println("Connection established successfully");
}
}
Commentary on the Code
-
Loading the Keystore: The client keystore is loaded first. Ensure the keystore type matches its actual format, e.g.,
PKCS12
vs.JKS
. -
Loading the Truststore: A corresponding truststore must also be loaded, which contains the root certifications that authenticate the server.
-
Creating Key and Trust Managers: This is vital for establishing a secure connection. A failure at this point indicates a significant configuration error.
-
SSL Context: This establishes the security context necessary for the protected communication.
-
Socket Creation and Handshake: Finally, a secure socket connection is created, and an SSL handshake is initiated.
Troubleshooting Steps
If you're experiencing issues despite a correct setup, consider the following steps:
1. Validate Certificate Chain
Use tools like openssl
to validate the certificate chain presented by the server.
openssl s_client -connect server-address:443
This command shows the certificate chain; make sure there are no expired certificates.
2. Check Port Accessibility
Make sure your network allows traffic on the TLS port, typically 443
. Use tools like telnet
or curl
to verify this.
3. Enable Detailed Logging
Enable SSL debugging in Java. This can help catch issues in the handshake process. Start your application like this:
java -Djavax.net.debug=ssl,handshake -jar your-app.jar
You will gain detailed logs that can point out where the problem lies in the mTLS handshake process.
4. Compare TLS Versions
Check the supported TLS versions on both the client and server side. Use the following command to check supported protocols on your server:
openssl s_client -connect server-address:443 -tls1_2
If there’s a mismatch, you may need to update your server configuration or your Java application settings.
5. Consult Documentation and Resources
Always keep updated with documentation related to your libraries (like Apache HttpClient and Spring Boot). They may provide additional insights and examples that you need.
My Closing Thoughts on the Matter
Making use of mTLS adds an additional layer of security to your application. However, troubleshooting issues can be daunting. By following the right steps and understanding the setup, you can effectively address problems as they arise. Remember, validating your certificates, ensuring proper configuration of keystores and truststores, and leveraging detailed logging are crucial steps in a successful mTLS implementation in Java.
If you have more questions or want to delve deeper into the topic, feel free to leave comments below!
Now that you have a clearer understanding, go ahead and implement your mTLS solutions with confidence. Happy coding!
Checkout our other articles