Common Pitfalls in Deploying Akka HTTP Apps on Cloud Foundry
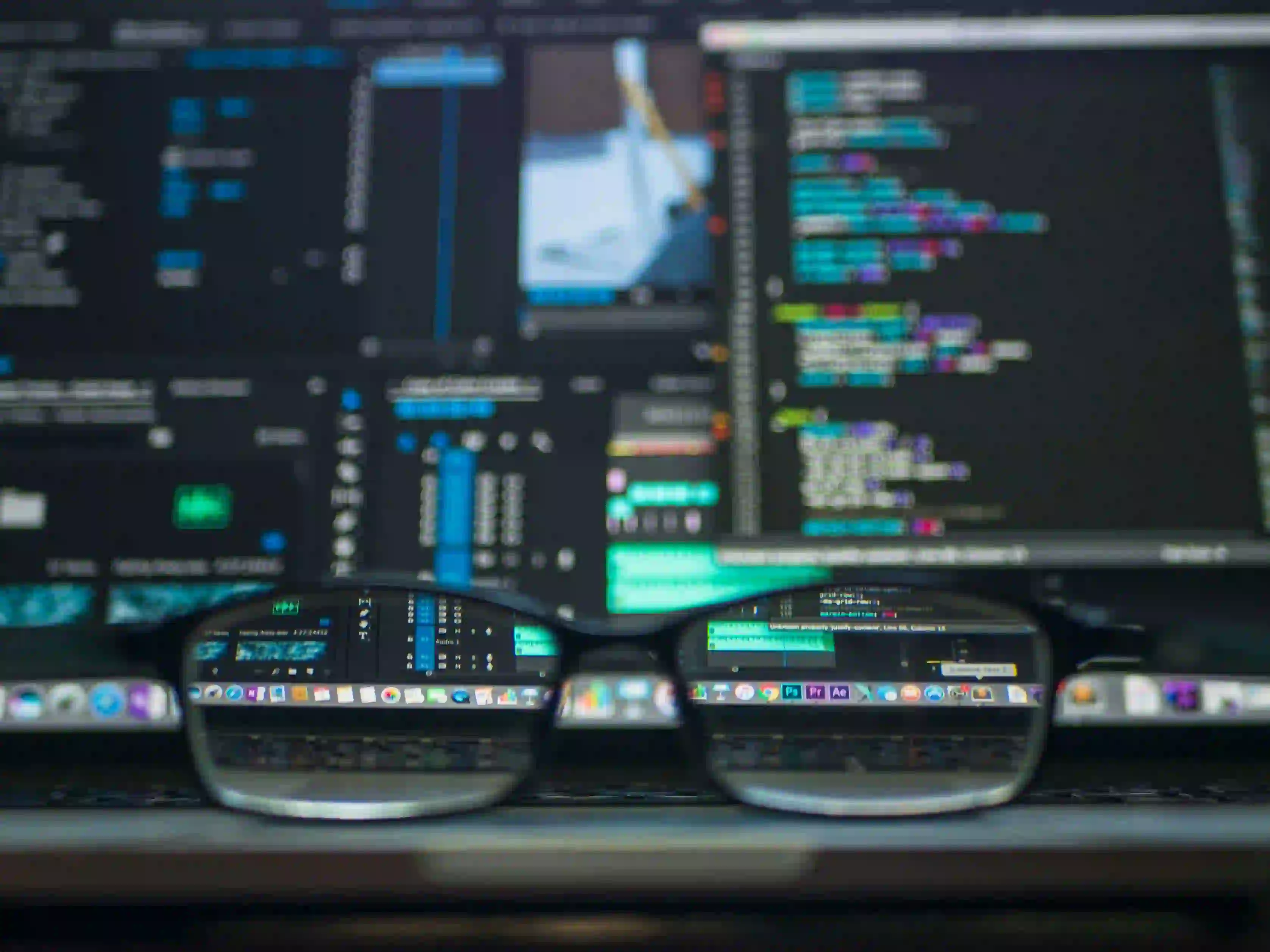
Common Pitfalls in Deploying Akka HTTP Apps on Cloud Foundry
Deploying applications to cloud environments can be challenging, especially for complex frameworks like Akka HTTP. Akka HTTP is a powerful toolkit for building HTTP-based applications in Scala. However, when you decide to host such applications on platforms like Cloud Foundry, you might encounter several pitfalls that could derail your deployment process. In this blog post, we'll explore common pitfalls associated with deploying Akka HTTP applications on Cloud Foundry, offering solutions and insights based on best practices.
Understanding Akka HTTP and Cloud Foundry
Before diving into the common pitfalls, it’s essential to understand what Akka HTTP and Cloud Foundry offer.
What is Akka HTTP?
Akka HTTP is an asynchronous, streaming toolkit for building HTTP-based applications. Its flexibility and capability to handle concurrent users effectively make it a popular choice among developers. The main features include:
- Routing: A powerful, extensible routing DSL.
- Streaming: Built around reactive streams to handle a large number of concurrent connections.
- Integration: Seamlessly integrates with Akka Actors for better concurrency management.
What is Cloud Foundry?
Cloud Foundry is an open-source cloud application platform that provides a choice of cloud services to facilitate application deployment. It abstracts away the underlying infrastructure, allowing developers to focus on writing code rather than managing servers. Key features include:
- Multi-Cloud Support: Runs on major public and private clouds.
- Rapid Deployment: Continuous delivery support to quickly push updates.
- Scaling: Effortless horizontal scaling of services.
Now that we have a brief overview of both technologies, let’s dive into the common pitfalls.
Common Pitfalls in Deploying Akka HTTP Apps on Cloud Foundry
1. Misconfigured Memory Limits
One of the primary challenges when deploying applications on Cloud Foundry is memory management. Akka HTTP applications can be memory-intensive, especially under heavy load. Common mistakes include:
- Underestimating Memory Needs: Failing to allocate sufficient memory.
- Overlimentation: Setting unreasonably high limits, causing inefficient resource use.
Solution: Monitor memory usage in development and conduct load testing to determine the average and peak memory requirements.
// application.conf
akka.http.server {
parsin {
max-content-length = 2MB // Ensure this is configured adequately
}
}
Commentary: Adjust your server settings in the application.conf
file to optimize the application's memory usage effectively.
2. Ignoring Connection Management
Akka HTTP’s asynchronous nature allows for high concurrency. However, not managing connections properly can lead to resource exhaustion.
- Too Many Open Connections: This can cause the system to hang, affecting performance.
Solution: Implement connection limits with strategies to manage backpressure.
import akka.http.scaladsl.Http
import akka.http.scaladsl.server.Route
val route: Route = ??? // Define your routes here
val binding = Http().bindAndHandle(route, "localhost", 8080, connectionContext).map { binding =>
println(s"Server online at http://localhost:${binding.localAddress.getPort}/")
}
Commentary: Make sure to bind your server properly and handle what to do when connections exceed the limits.
3. Networking Issues
When deploying apps, networking configurations can become problematic due to incorrect service bindings.
- Binding Issues: Not binding to the correct host or port.
Solution: Configure your application to bind to the correct ports, usually environment variables in Cloud Foundry.
val host = sys.env.getOrElse("PORT", "8080")
Http().bindAndHandle(route, "0.0.0.0", host.toInt)
Commentary: Using environment variables ensures that your app listens to the right port assigned by the cloud platform.
4. Lack of Health Checks
Deploying applications without health checks can lead to issues going undetected until they become critical.
- Failure to Restart or Scale: Cloud Foundry won’t automatically detect issues with your app.
Solution: Implement health endpoints in your Akka HTTP application.
val healthCheckRoute: Route =
path("health") {
get {
complete("Application is healthy!")
}
}
Commentary: This simple route helps you ensure that your application is running correctly. Cloud Foundry can then use this endpoint for checking the health of your application.
5. Inadequate Logging and Monitoring
Effective logging and monitoring are critical in cloud environments. Akka HTTP provides many logging capabilities, but improper configuration may lead to missing logs or excessive log levels.
- Insufficient Logging: Not capturing enough information for troubleshooting.
Solution: Adjust the logging within the application and integrate it with Cloud Foundry’s logging.
import akka.event.Logging
val log = Logging(system, this)
log.info("Starting the server...")
Commentary: Make sure to use structured logging to get relevant insights. This will significantly help during troubleshooting.
6. Dependency Version Conflicts
When deploying an Akka HTTP app, it’s essential to ensure that all libraries and dependencies are compatible with one another.
- Dependency Hell: Conflict between library versions can cause build and runtime errors.
Solution: Regularly update and audit your dependencies.
// build.sbt
libraryDependencies ++= Seq(
"com.typesafe.akka" %% "akka-http" % "10.2.7",
"com.typesafe.akka" %% "akka-stream" % "2.6.19"
)
Commentary: Ensure to specify the right versions for your dependencies. Use SBT's dependency tree feature to audit conflicts.
7. Unoptimized Build and Deployment Process
The deployment steps can significantly affect application performance. Unoptimized images can lead to longer deployment times, impacting productivity.
- Heavy Docker Images: Making your Docker images too large slows down build time.
Solution: Use multi-stage builds to minimize the size of the final Docker image.
# Stage 1
FROM sbt:latest AS builder
WORKDIR /app
COPY . .
RUN sbt assembly
# Stage 2
FROM openjdk:11-jre
COPY --from=builder /app/target/scala-2.13/your-app.jar /your-app.jar
CMD ["java", "-jar", "/your-app.jar"]
Commentary: This approach significantly reduces the final image size, optimizing deployments in Cloud Foundry.
8. Failing to Utilize Cloud Services
Finally, one of the biggest missed opportunities is not utilizing the native services provided by Cloud Foundry, such as databases, caching, and messaging queues.
- Hard-Coding Services: Creating tightly coupled applications limits scalability.
Solution: Use service bindings and environment variables to access Cloud Foundry services.
val databaseUrl = sys.env("DATABASE_URL")
// Connect to the database using the URL
Commentary: This ensures that your application remains portable and can take full advantage of the cloud's capabilities.
Key Takeaways
Deploying Akka HTTP applications on Cloud Foundry can be a robust solution for modern cloud-native applications. However, it's critical to avoid common pitfalls to ensure a smooth deployment and optimal performance. From memory configurations to effective logging practices, each pitfall can be mitigated with proper understanding and implementation.
By following these guidelines, you can enhance not only the robustness of your Akka HTTP applications but also their resilience when deployed in a cloud environment. For more resources on Akka HTTP, consider checking out the Akka Documentation.
Further Reading:
- Akka HTTP Official Documentation
- Cloud Foundry Documentation
- Effective Akka: A Guide for Scala Developers
By understanding and overcoming these common pitfalls, you can harness the full power of Akka HTTP and Cloud Foundry to build scalable and efficient applications. Happy coding!