When Design Patterns Become Anti-Patterns: Recognizing the Signs
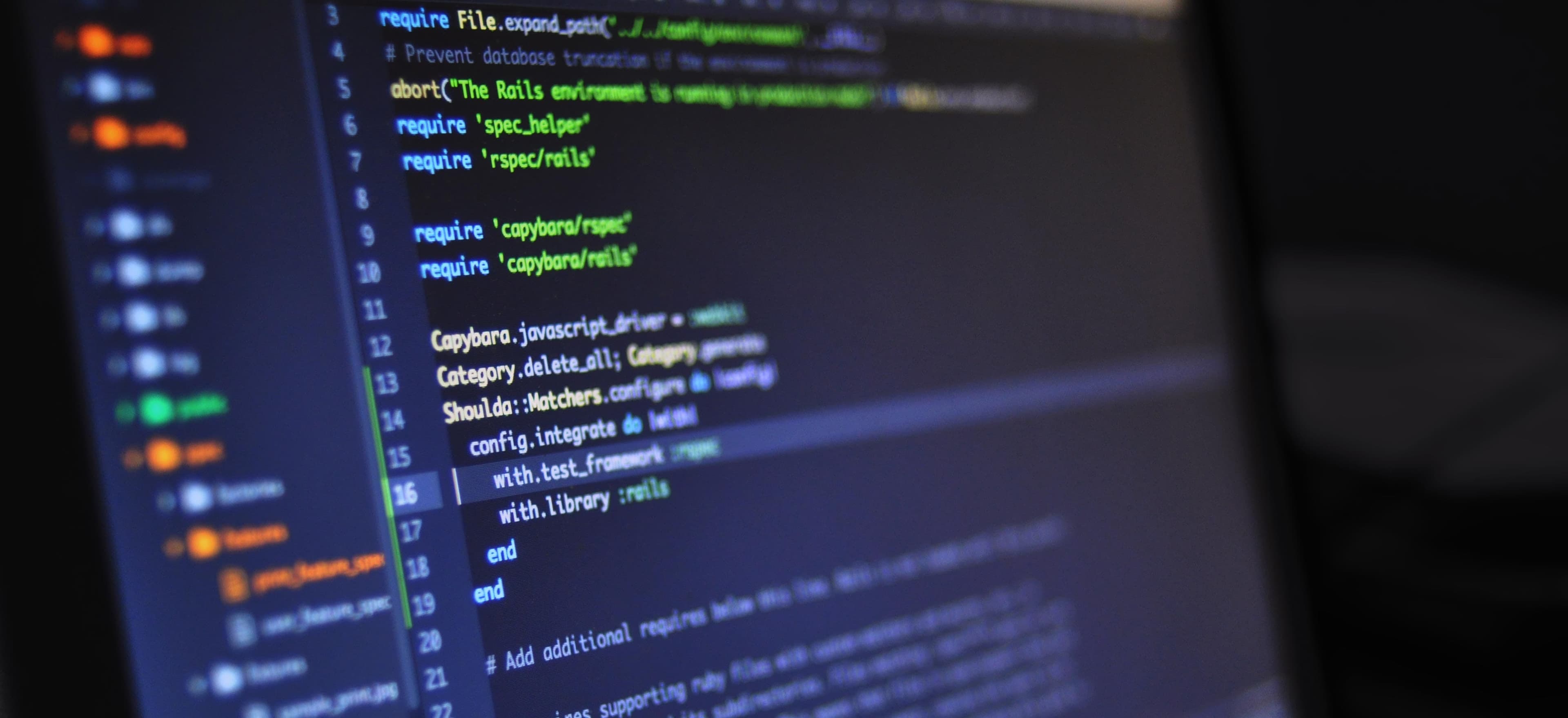
- Published on
When Design Patterns Become Anti-Patterns: Recognizing the Signs
In software development, design patterns serve as proven solutions to common problems. They provide a shared vocabulary, facilitate communication among developers, and promote best practices. However, the misuse of these patterns can lead to what are known as anti-patterns. In this blog post, we will explore how design patterns can become anti-patterns, recognize the telltale signs, and discuss strategies to avoid these pitfalls.
Understanding Design Patterns
Design patterns are typically classified into three categories: Creational, Structural, and Behavioral patterns. Each serves a specific purpose and addresses distinct problems in software design.
- Creational Patterns: These patterns deal with object creation mechanisms. Examples include Singleton, Factory Method, and Builder patterns.
- Structural Patterns: These patterns deal with object composition. Notable examples include Adapter, Composite, and Decorator patterns.
- Behavioral Patterns: These focus on how objects interact and communicate. Examples include Observer, Strategy, and Command patterns.
While these patterns offer great benefits, adhering strictly to them without considering the context can lead to complications and inefficiencies in your codebase.
Signs That Design Patterns Become Anti-Patterns
Recognizing when a design pattern has transitioned into an anti-pattern is crucial for maintaining a healthy codebase. Here are several signs to look out for:
1. Over-Complexity
One of the most significant indicators is the increased complexity of your code. Patterns like the Facade can lead to over-engineering when used unnecessarily.
class ComplexSystem {
public void operation1() {}
public void operation2() {}
public void operation3() {}
}
class Facade {
private ComplexSystem system;
public Facade(ComplexSystem system) {
this.system = system;
}
public void simplify() {
system.operation1();
system.operation2();
}
}
In this example, using a Facade can simplify client calls to a complex system. However, if the system isn’t truly that complex, adding a facade can introduce unnecessary layers, making your code harder to understand.
2. Excessive Abstraction
While abstraction is essential, too much of it can lead to confusion. Adapter patterns, for instance, can abstract away important details, making the code harder to follow.
interface Target {
void request();
}
class Adaptee {
public void specificRequest() {}
}
class Adapter implements Target {
private Adaptee adaptee;
public Adapter(Adaptee adaptee) {
this.adaptee = adaptee;
}
public void request() {
adaptee.specificRequest();
}
}
In scenarios where the Adaptee
provides minimal functionality, wrapping it in an adapter may create more layers of abstraction than necessary.
3. Misalignment with Requirements
When the implementation of a design pattern does not align vertically with the requirements of a project, it might be time to reconsider its use.
The Observer pattern can quickly become unwieldy if there are too many observers or if the relationships are not well defined:
class Subject {
private List<Observer> observers = new ArrayList<>();
public void attach(Observer observer) {
observers.add(observer);
}
public void notifyObservers() {
for (Observer observer : observers) {
observer.update();
}
}
}
In projects where observer relationships are too cumbersome, you may want to explore simpler alternatives.
4. Scalability Issues
Using patterns without considering their scalability can lead to issues as your system grows. For instance, the Singleton pattern is usually chosen for its global access. However, it can create bottlenecks in multi-threaded applications.
class Singleton {
private static Singleton instance;
private Singleton() {}
public static synchronized Singleton getInstance() {
if (instance == null) {
instance = new Singleton();
}
return instance;
}
}
The synchronized method may work for a small application, but as load increases, it will decrease performance.
5. Increased Dependencies
Design patterns often introduce new classes and interfaces that can lead to a tightly coupled codebase. The Decorator pattern can bloat the number of classes in your system.
interface Coffee {
String getDescription();
double cost();
}
class SimpleCoffee implements Coffee {
public String getDescription() {
return "Simple Coffee";
}
public double cost() {
return 2.0;
}
}
abstract class CoffeeDecorator implements Coffee {
protected Coffee coffee;
public CoffeeDecorator(Coffee coffee) {
this.coffee = coffee;
}
}
// Example concrete decorator
class MilkDecorator extends CoffeeDecorator {
public MilkDecorator(Coffee coffee) {
super(coffee);
}
public String getDescription() {
return coffee.getDescription() + ", Milk";
}
public double cost() {
return coffee.cost() + 0.5;
}
}
In this example, while decorators allow for flexible additions, each decorator creates a new class. For simple use cases, this can lead to a proliferation of classes that convolute your architectural vision.
Strategies for Avoiding Anti-Patterns
Preventing the evolution of design patterns into anti-patterns is imperative. Here are several strategies to keep in mind:
1. Stay Context-Aware
Always assess the context before implementing a design pattern. Context is key; a solution that fits one problem may not be appropriate for another. A contextual understanding—especially regarding project requirements—is vital.
2. Prioritize Simplicity
When in doubt, choose simpler solutions. One of the core tenets of software design is "Do not over-engineer." Sometimes, a straightforward implementation is better than a complex design pattern.
3. Utilize Code Reviews
Conducting code reviews can support maintaining a healthy design pattern usage. Peers can help identify potential pitfalls and highlight unnecessary complexity.
4. Refactor Continuously
As the project evolves, take time to refactor code that has become unwieldy or reminiscent of an anti-pattern. Regularly revisiting your design strategy can lead to improved clarity and simplicity.
5. Limit Your Use of Patterns
It’s easy to become pattern-obsessed, applying them where they may not be useful. Instead of trying to adhere to all design patterns, only employ those that add real value in each specific scenario.
6. Educate Your Team
Provide training to your team on the appropriate use of design patterns. Knowledge sharing can empower engineers to recognize the signs of anti-patterns before they develop.
Final Thoughts
Design patterns are essential tools that help developers solve challenges efficiently. However, they have the potential to morph into anti-patterns when misapplied or overused. By recognizing the signs and implementing strategies to avoid pitfalls, developers can ensure their systems remain clean, scalable, and easy to maintain.
For more about design patterns and anti-patterns, consider exploring sources like Refactoring Guru and Martin Fowler’s website. Remember, the key to leveraging design patterns effectively lies in understanding when and how to use them.
Happy coding!