Memory Leaks: The Hidden Cost of Object Creation in Java
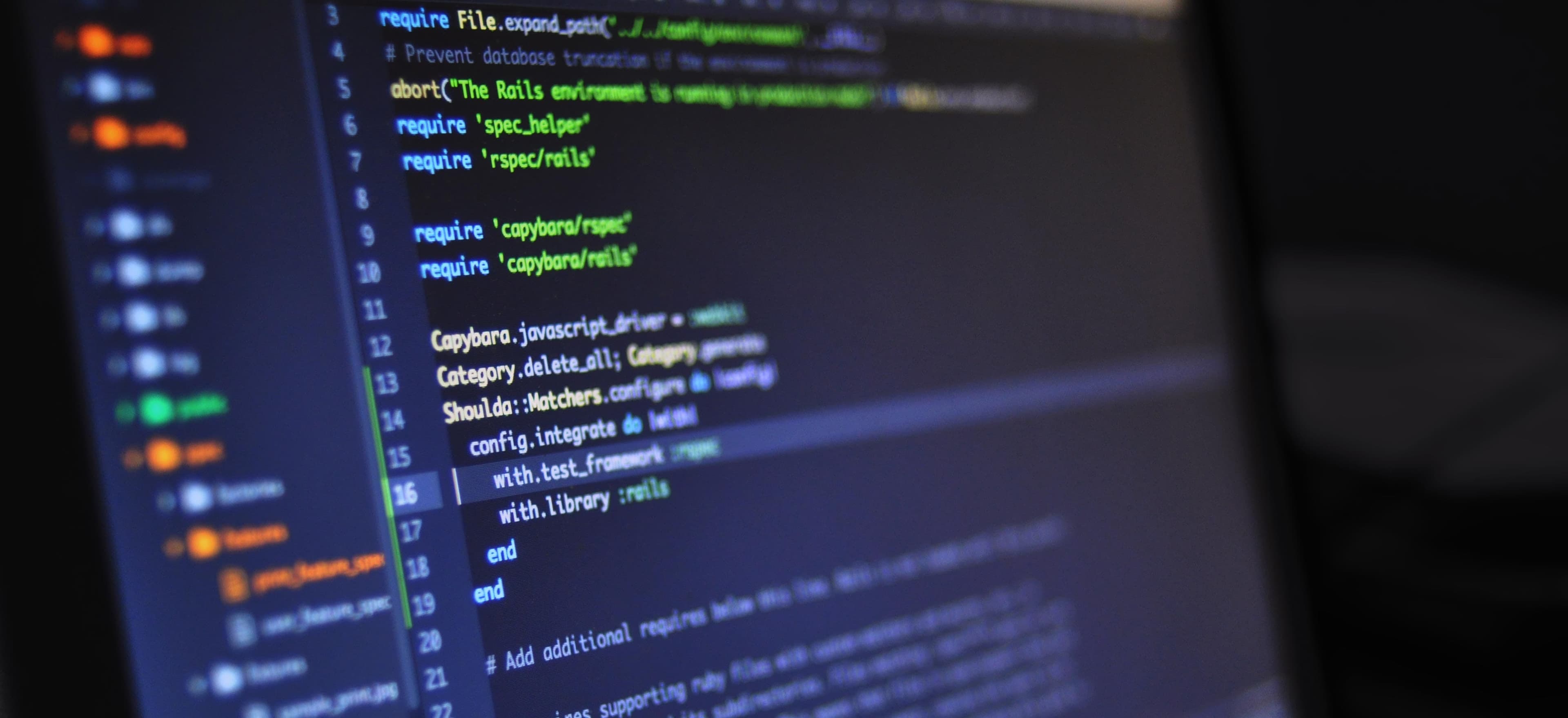
- Published on
Memory Leaks: The Hidden Cost of Object Creation in Java
As developers, we often dedicate numerous hours to writing clean, efficient code, but one issue that can derail our best efforts is memory leaks. They are sneaky, often going unnoticed until they culminate in performance bottlenecks or application crashes. In this blog post, we will delve deep into memory leaks in Java, exploring what they are, how they occur, and strategies to prevent them.
What is a Memory Leak?
A memory leak in Java occurs when objects are no longer needed by a program but are still referenced, preventing the garbage collector (GC) from reclaiming that memory. This leads to increased memory consumption over time, ultimately resulting in performance degradation or potential application failure.
Why Should You Care?
Java has robust garbage collection mechanisms to reclaim unused objects. However, even Java is not immune to memory leaks. Awareness of this concept is crucial for developers, as it directly impacts application performance and user experience. Ignoring memory leaks can result in slow applications and frustrated users. In a professional environment, they can lead to costly downtime and unhappy stakeholders.
How Do Memory Leaks Occur in Java?
Memory leaks can manifest in various ways. Here are some common scenarios:
-
Static Fields: Objects referenced from static fields stay in memory for the lifetime of the application.
-
Listeners and Callbacks: Failing to unregister listeners or callbacks can lead to a memory leak, as the referenced objects are still kept alive.
-
Long-lived Collections: Using collections (like lists or maps) to hold objects without proper cleanup can lead to leaks.
-
Thread Locals: These are often overlooked. If ThreadLocal variables are not cleaned up, they can maintain references to objects longer than necessary.
How to Diagnose Memory Leaks
Understanding how to identify memory leaks is key for developers. Here are effective tools and strategies:
1. Profiling Tools
Using a profiling tool like VisualVM or Eclipse Memory Analyzer (MAT) can help spot leaks. These tools allow you to monitor heap memory usage in your applications and analyze memory consumption.
2. Garbage Collection Logs
Enabling GC logs can provide insight into how the garbage collector is functioning. Use JVM arguments such as:
-XX:+PrintGCDetails -XX:+PrintGCTimeStamps -Xloggc:gc.log
These flags generate detailed logs of the GC process, helping you identify which objects are not being collected.
Code Examples: A Deeper Dive
Let’s explore some coding practices that can introduce memory leaks, and we'll provide solutions to mitigate these risks.
Example 1: Static Fields
Having static fields can lead to unintentional retention of objects.
public class MemoryLeakExample {
private static List<SomeObject> leakedObjects = new ArrayList<>();
public void addObject(SomeObject obj) {
leakedObjects.add(obj); // This keeps objects alive
}
}
The Fix
Avoid static fields for non-essential references. If global access is needed, consider using weak references:
import java.lang.ref.WeakReference;
public class FixedMemoryLeakExample {
private static List<WeakReference<SomeObject>> leakedObjects = new ArrayList<>();
public void addObject(SomeObject obj) {
leakedObjects.add(new WeakReference<>(obj)); // Only weakly referenced
}
}
Example 2: Listeners and Callbacks
Failing to unregister listeners leads to memory retention.
public class EventPublisher {
private List<EventListener> listeners = new ArrayList<>();
public void registerListener(EventListener listener) {
listeners.add(listener);
}
// This method should potentially be called!
public void removeListener(EventListener listener) {
listeners.remove(listener);
}
}
The Fix
Always unregister listeners to remove references when they are no longer needed:
public class EventClient {
private EventPublisher publisher;
public EventClient(EventPublisher publisher) {
this.publisher = publisher;
publisher.registerListener(this::handleEvent);
}
private void handleEvent(Event event) {
// Handle the event
}
public void cleanup() {
publisher.removeListener(this::handleEvent); // Clean up when done
}
}
Example 3: Thread Locals
Thread-local variables can also contribute to memory leaks.
public class ThreadLocalExample {
private static ThreadLocal<SomeObject> threadLocalObject = new ThreadLocal<>();
public void setObject(SomeObject obj) {
threadLocalObject.set(obj); // obj remains referenced
}
}
The Fix
Make sure to clear the ThreadLocal variable when you’re done:
public class ImprovedThreadLocalExample {
private static ThreadLocal<SomeObject> threadLocalObject = new ThreadLocal<>();
public void setObject(SomeObject obj) {
threadLocalObject.set(obj);
}
public void clearObject() {
threadLocalObject.remove(); // Clear reference
}
}
Preventing Memory Leaks Through Best Practices
-
Prefer Weak References: Utilize
WeakReference
for caches and large objects that can be garbage collected. -
Use Listeners Wisely: Always remove listeners when they are no longer necessary.
-
Monitor Memory Usage: Regularly profile your application and analyze the heap dumps.
-
Leverage Tools: Make use of tools like SonarQube for static analysis to identify potential leaks in your code.
My Closing Thoughts on the Matter
Memory leaks may not be visible on the surface, but they can accumulate, leading to significant performance issues. By understanding their causes and potential points of failure, developers can take proactive measures to avoid them. Adopting best practices and employing diagnostic tools will ensure that your applications remain efficient and reliable.
For further reading on garbage collection in Java, consider checking this article or explore more about memory management in Java.
Embrace these techniques and continue refining your skills as a Java developer to write robust, leak-free applications. Happy coding!
Checkout our other articles