Common Pitfalls in Microservices Adoption and How to Avoid Them
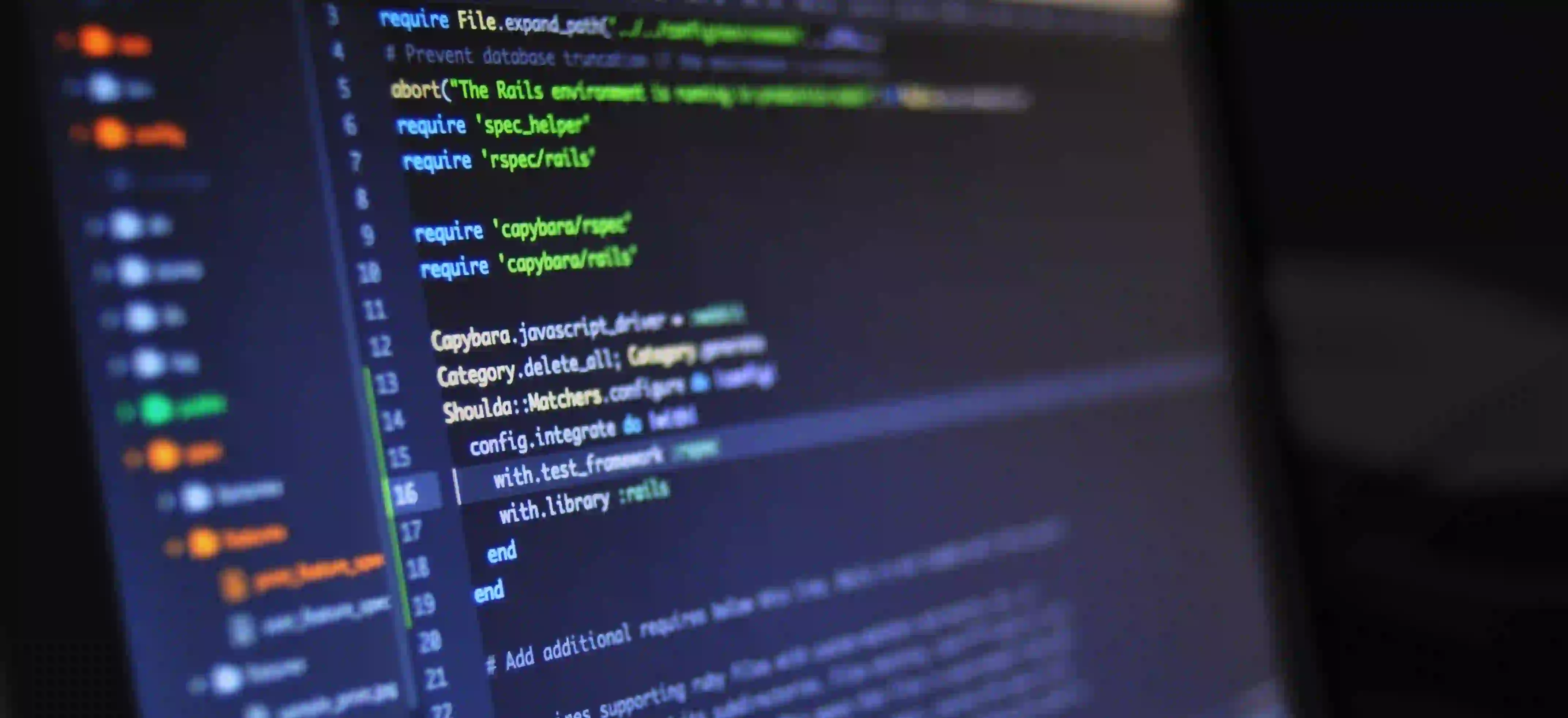
Common Pitfalls in Microservices Adoption and How to Avoid Them
The rise of microservices architecture has revolutionized the way applications are built and deployed. However, the adoption of microservices is not without challenges. Organizations often find themselves grappling with various pitfalls that can hinder the full potential of this architecture. In this blog post, we will explore common pitfalls associated with microservices adoption and provide actionable strategies to avoid them.
Understanding Microservices Architecture
Microservices architecture is an approach to software development where an application is structured as a collection of loosely coupled, independently deployable services. Each service is focused on delivering a single business function, enabling teams to develop, test, and deploy services independently. This modular approach offers several benefits, including improved scalability, faster deployments, and enhanced fault isolation.
However, while the benefits of microservices are compelling, organizations must tread carefully. Let's dive into some of the most common pitfalls in microservices adoption.
1. Lack of Clear Objectives
The Problem
Many organizations rush into microservices without a clear understanding of why they are adopting this architecture. This can lead to confusion, wasted resources, and ultimately, a failure to achieve desired outcomes.
The Solution
Before diving in, establish clear objectives regarding what you want to achieve with microservices. This can include improving scalability, enhancing deployment speed, or increasing team autonomy. Defining these objectives helps to align your team, set realistic expectations, and evaluate the success of your implementation.
2. Poor Service Design
The Problem
One of the critical aspects of microservices is defining boundaries for each service. Poorly designed services can lead to unnecessary complexity, performance bottlenecks, and increased inter-service communication.
The Solution
To avoid this pitfall, embrace domain-driven design (DDD) principles. Focus on identifying business capabilities and group them into services based on business functionality. A good rule of thumb is to ensure that each service has a clear domain responsibility.
Here's a simple example. Consider an eCommerce application with user management, product catalog, and order processing functionalities:
public class UserService {
public User findUserById(String userId) {
// Implementation to retrieve user details
}
}
public class ProductService {
public List<Product> listProducts() {
// Implementation to retrieve product list
}
}
public class OrderService {
public Order createOrder(Order order) {
// Implementation to create a new order
}
}
Commentary
In this example, each service is responsible for a specific part of the application. This separation of concerns allows teams to work independently and deploy without impacting others.
3. Ignoring the Data Management Strategy
The Problem
In traditional monolithic applications, shared databases are common. However, microservices often require each service to have its own database. Ignoring this can lead to data consistency issues and challenges in transactions across multiple services.
The Solution
Adopt a decentralized data management strategy. This involves each service managing its data store, using asynchronous communication (like messaging queues) to deal with data changes. Event sourcing and CQRS (Command Query Responsibility Segregation) can be helpful patterns.
Consider a scenario in which an order is placed, and both the OrderService and InventoryService need to update their respective data stores:
public class OrderService {
public void createOrder(Order order) {
// Save order to the Order database
// Publish an event for inventory update
messagingService.publish("ORDER_PLACED", order);
}
}
public class InventoryService {
@EventListener
public void handleOrderPlaced(Order order) {
// Update inventory based on the order
}
}
Commentary
By using an event-driven approach, you avoid tight coupling between services and maintain data integrity across them.
4. Overlooking Monitoring and Observability
The Problem
Microservices can lead to complex architectures where numerous services communicate with each other. Without proper monitoring, diagnosing issues can become increasingly challenging.
The Solution
Invest in observability tools that allow you to monitor service interactions, log errors systematically, and track performance metrics. Tools like Prometheus, Grafana, and Jaeger are popular for ensuring that you have a holistic view of your microservices.
To effectively monitor your services, establish logging practices across all services:
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class UserService {
private static final Logger logger = LoggerFactory.getLogger(UserService.class);
public User findUserById(String userId) {
logger.info("Fetching user with ID: {}", userId);
// Implementation to retrieve user details
}
}
Commentary
By incorporating structured logging, you can improve your ability to diagnose issues, track user behavior, and monitor application health.
5. Neglecting DevOps Practices
The Problem
Microservices demand a more sophisticated deployment strategy than monolithic applications. Organizations often overlook the importance of DevOps practices, which can lead to deployment headaches.
The Solution
Implement CI/CD (Continuous Integration/Continuous Deployment) pipelines to automate the building, testing, and deployment of your microservices. Utilize tools like Jenkins, GitLab CI, or CircleCI to facilitate this process. Ensure that your pipeline includes:
- Automated Tests: Validate the functionality of your services before deployment.
- Code Quality Checks: Maintain code standards and prevent regressions.
- Deployment Strategies: Consider blue-green deployments or canary releases to manage risk during deployments.
Example CI/CD Pipeline Snippet
pipeline:
stages:
- build
- test
- deploy
build:
script:
- mvn clean package
test:
script:
- mvn test
deploy:
script:
- deploy_script.sh # a script to deploy your service
Commentary
Automation in the deployment process not only speeds up releases but also minimizes the risk of human error.
6. Underestimating Network Latency
The Problem
Microservices communicate over a network, and as the number of services grows, so does network latency. Assuming that inter-service calls are instantaneous can lead to performance issues and degradation of user experience.
The Solution
Optimize communication strategies via asynchronous messaging systems like RabbitMQ or Kafka. This minimizes the wait times associated with service calls and allows for better resource utilization. Additionally, consider caching strategies for frequently accessed data.
Here’s an example of using a cache:
public class UserService {
private Cache<User> userCache = new Cache<>();
public User findUserById(String userId) {
// Check in the cache first
if (userCache.contains(userId)) {
return userCache.get(userId);
}
// Otherwise, fetch from the database
User user = fetchFromDatabase(userId);
userCache.put(userId, user);
return user;
}
}
Commentary
Caching frequently accessed data can significantly reduce the need for inter-service communication, thereby lowering network latency and improving performance overall.
The Last Word
While microservices can greatly enhance the scalability and maintainability of applications, organizations must be vigilant in avoiding common pitfalls. By defining clear objectives, employing sound design principles, implementing a robust data management strategy, ensuring observability, adopting DevOps practices, and being mindful of network latency, organizations can set themselves up for a successful microservices journey.
To dive deeper into microservices architecture and business strategies, you might find Martin Fowler's article on Microservices and AWS's guide on Microservices useful.
Adopting microservices is not just a technological shift but a cultural one. Embrace the change thoughtfully, and you'll reap the rewards that come with it.
Happy coding!