Dealing with AsyncTask Memory Leaks in Android ListViews
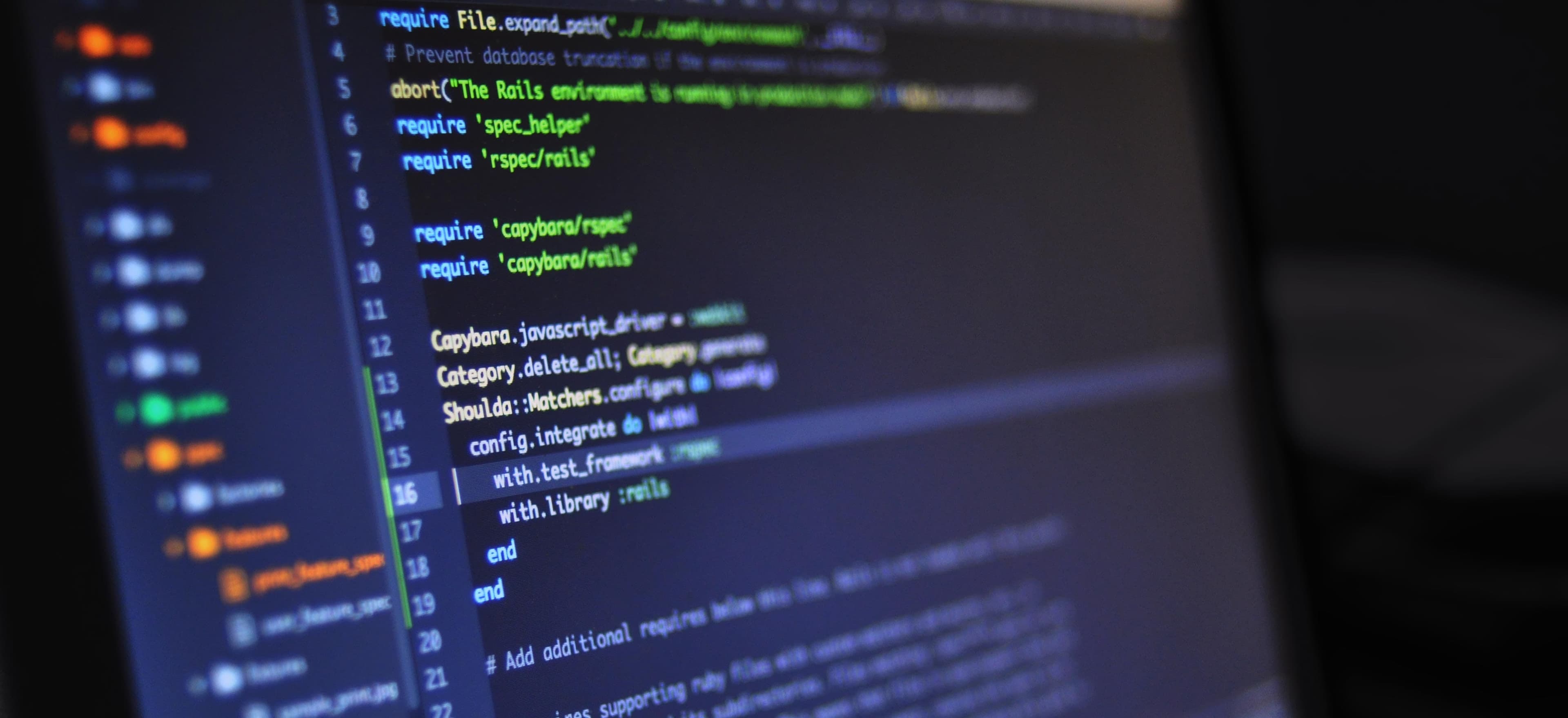
- Published on
Dealing with AsyncTask Memory Leaks in Android ListViews
In the dynamic world of Android development, managing memory efficiently is crucial, especially when dealing with asynchronous tasks. One prevalent issue is the memory leak that can occur when using AsyncTask in conjunction with ListViews. In this blog post, we will explore the causes of these memory leaks, their potential consequences, and effective strategies to avoid them.
Understanding AsyncTask
AsyncTask is an Android component that allows us to do background operations without having to manage threads manually. It is a great aid for performing operations such as network requests, database queries, or heavy computations:
private class MyAsyncTask extends AsyncTask<Void, Void, String> {
@Override
protected String doInBackground(Void... voids) {
// Background operation, e.g., downloading data
return downloadData();
}
@Override
protected void onPostExecute(String result) {
// Update UI with result
textView.setText(result);
}
}
The Issue with Memory Leaks
While AsyncTask is designed to minimize complexity, misusing it can lead to memory leaks. A common pitfall is when an AsyncTask holds a reference to an Activity
or Fragment
. If the task is executed and the associated activity is destroyed (e.g., during configuration changes like screen rotations), the AsyncTask continues to hold an inactive reference, preventing the garbage collector from reclaiming memory.
Why Memory Leaks Matter
Memory leaks can lead to severe performance issues in Android applications, manifesting as sluggish performance, increased battery usage, and ultimately, application crashes. This can dampen user experience and lead to negative feedback, potentially harming your app's reputation.
Identifying Memory Leaks in AsyncTask
To spot memory leaks, developers can utilize tools like LeakCanary and Android Profiler. A typical leak would appear in the following manner:
Activity com.example.MainActivity has leaked:
* static AsyncTask com.example.MyAsyncTask
This indicates that your AsyncTask is retaining a reference to the instance of MainActivity
, preventing it from being garbage collected.
Strategies for Avoiding Memory Leaks
1. Use WeakReference
A common approach to avoid memory leaks is to use a WeakReference
to refer to the Activity
. This allows the garbage collector to reclaim the Activity
without hindrance when needed.
Here’s an example:
private class MyAsyncTask extends AsyncTask<Void, Void, String> {
private WeakReference<MainActivity> activityReference;
MyAsyncTask(MainActivity context) {
activityReference = new WeakReference<>(context);
}
@Override
protected String doInBackground(Void... voids) {
return downloadData();
}
@Override
protected void onPostExecute(String result) {
MainActivity activity = activityReference.get();
if (activity == null || activity.isFinishing()) {
return; // Activity is no longer valid
}
activity.textView.setText(result);
}
}
By using WeakReference
, we ensure that the AsyncTask
does not prevent the corresponding activity from being garbage collected.
2. Cancel AsyncTask on Activity Destroy
Another approach is to cancel the AsyncTask
when the Activity
is destroyed. This can be implemented in the onDestroy
method of the activity:
@Override
protected void onDestroy() {
super.onDestroy();
if (myAsyncTask != null) {
myAsyncTask.cancel(true);
}
}
This way, if the activity is destroyed, the AsyncTask will not attempt to update the UI, which is invalid in a destroyed state.
3. Use Lifecycle-Aware Components
With Android's Jetpack libraries, consider using ViewModel
and LiveData
combined with Coroutine
or RxJava
. These components are lifecycle-aware and allow tasks to survive configuration changes without the risk of memory leaks.
A simple example using Kotlin Coroutines would look like:
class MyViewModel : ViewModel() {
private val _data = MutableLiveData<String>()
val data: LiveData<String> get() = _data
fun fetchData() {
viewModelScope.launch {
val result = downloadData()
_data.value = result
}
}
}
This approach avoids memory leaks entirely, as viewModelScope
is tied to the ViewModel
, which is destroyed only when the Activity
or Fragment
is fully cleared.
4. Utilize FragmentManager for Fragments
If you are dealing with Fragment
, ensure to use retainInstance
or FragmentManager
. Instead of directly using AsyncTask instances, you may prefer to have data-loading logic within your fragments using these patterns.
Lessons Learned
Memory leaks due to mismanaged AsyncTasks can severely impact the performance and reliability of your Android applications. By understanding the mechanisms behind AsyncTask and applying strategies such as using WeakReference
, cancelling tasks appropriately, employing lifecycle-aware components, and better managing your fragments, developers can design robust and efficient applications.
For more information on memory management in Android, check out Android Developer Documentation and Best Practices for Memory Management in Android.
By implementing these practices, you’re not just avoiding memory leaks; you’re also ensuring a better user experience, a fundamental goal for any Android developer.
Feel free to share your experiences with AsyncTask and memory management in the comments!
Checkout our other articles