How to Prevent Guava Cache Overflowing and Losing Data
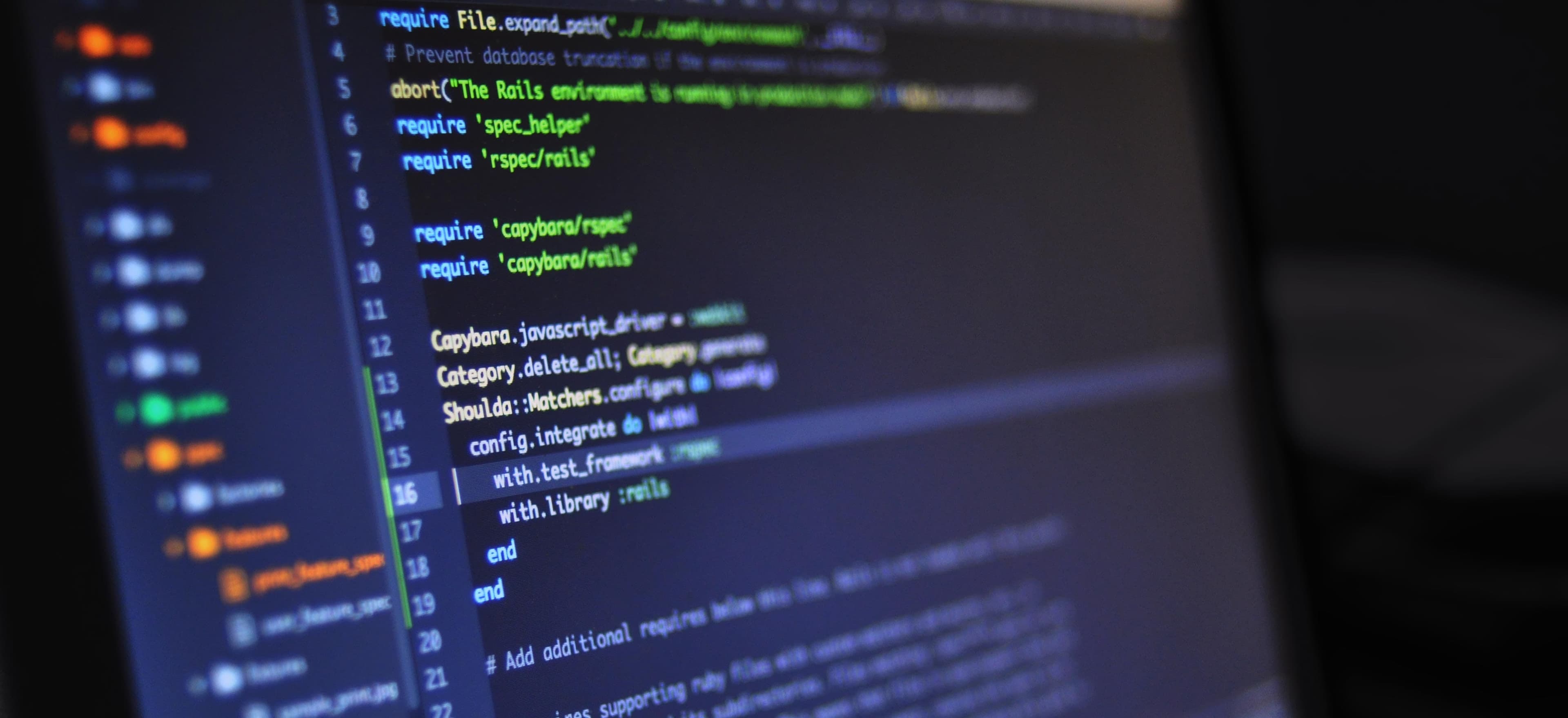
- Published on
Preventing Guava Cache Overflowing and Losing Data
In today's world of high-performance applications, caching plays a vital role in optimizing data retrieval. When it comes to Java, Guava Cache from Google's Guava library has become a go-to for developers due to its powerful features. However, one common issue developers encounter is cache overflow, which can lead to data loss. In this blog post, we will explore strategies for preventing Guava Cache overflow, ensuring that you can maintain your application's efficiency without sacrificing data integrity.
Understanding Guava Cache
First, let's clarify what Guava Cache is. Guava Cache is an in-memory caching library that helps store objects temporarily for quick retrieval. It's designed to handle a variety of caching strategies, including:
- Time-based expiration (TTL)
- Size-based eviction
- Concurrency controls
Caching significantly speeds up data access times and offloads your database or external service, thereby enhancing performance.
Why Does Cache Overflow Occur?
Cache overflow usually occurs when the size of the cache exceeds the allocated space. The consequences are twofold:
- Data Loss: Older entries may be evicted to make room for new data, leading to the unexpected loss of cached values that could have been useful.
- Performance Degradation: An overflowing cache can lead to increased memory consumption and, ultimately, application performance issues.
Strategies to Prevent Cache Overflow
Preventing cache overflow involves a combination of configuration settings and best practices. Here are some effective methods to ensure your Guava Cache remains efficient and data integrity is maintained.
1. Set Size Limits on your Cache
One of the most direct methods to prevent overflow is to define size constraints on your Guava Cache. This ensures that the cache will evict older entries when it reaches a specific size.
Code Example:
import com.google.common.cache.CacheBuilder;
import com.google.common.cache.Cache;
public class CacheExample {
private final Cache<String, String> cache;
public CacheExample() {
cache = CacheBuilder.newBuilder()
.maximumSize(1000) // Setting the maximum size
.build();
}
public void cacheData(String key, String value) {
cache.put(key, value); // Storing key-value pairs in the cache
}
public String getData(String key) {
return cache.getIfPresent(key); // Retrieving value if present
}
}
Why Use Maximum Size?
By limiting the maximum size, you balance memory usage and data retention. However, you will need to determine an appropriate cache size based on your application’s data access patterns.
2. Implement Expiration Policies
Another strategy is to set expiration times on cached entries. Guava Cache provides configurations for both access-based and write-based expiration.
Code Example:
import com.google.common.cache.CacheBuilder;
import com.google.common.cache.Cache;
public class ExpiringCacheExample {
private final Cache<String, String> cache;
public ExpiringCacheExample() {
cache = CacheBuilder.newBuilder()
.maximumSize(1000) // Also setting maximum size
.expireAfterAccess(10, TimeUnit.MINUTES) // Expire entries after 10 minutes of access
.build();
}
// Methods to add and get data remain the same
}
Why Use Expiration Policies?
Setting expiration helps manage memory more effectively by automatically cleaning out old entries, which might not be accessed again, thus preventing overflow.
3. Use a Loader Function
A loader function can automatically populate cache whenever data is missing. This reduces the chances of application failures due to cache misses, allowing you to manage the cache efficiently.
Code Example:
import com.google.common.cache.CacheBuilder;
import com.google.common.cache.CacheLoader;
import com.google.common.cache.LoadingCache;
public class CachingWithLoader {
private final LoadingCache<String, String> cache;
public CachingWithLoader() {
cache = CacheBuilder.newBuilder()
.maximumSize(1000)
.build(new CacheLoader<String, String>() {
@Override
public String load(String key) {
return loadDataFromSource(key); // Custom method to fetch data
}
});
}
private String loadDataFromSource(String key) {
// Simulating a data fetch
return "data for " + key;
}
}
Why Use a Loader?
Using a loader ensures that when a cache miss occurs, the application can still retrieve the necessary data. This can prevent scenarios where you would encounter incomplete data due to cache eviction.
4. Monitor Cache Usage
Monitoring cache metrics is vital. Regular checks on how often cache entries are hit versus missed can inform adjustments to cache configurations.
You can use Guava’s built-in statistics using:
cache.stats();
Why Monitor?
Monitoring allows you to gain insights into your application's performance, making you aware of issues before they result in cache overflow.
5. Use Asynchronous Loading
Consider using asynchronous loading with Guava Cache, which can enhance performance and resource management.
Code Example:
import com.google.common.cache.CacheBuilder;
import com.google.common.cache.LoadingCache;
public class AsyncCacheExample {
private final LoadingCache<String, String> cache;
public AsyncCacheExample() {
cache = CacheBuilder.newBuilder()
.maximumSize(1000)
.build(new CacheLoader<String, String>() {
@Override
public String load(String key) throws Exception {
return loadAsyncData(key); // Custom async method to fetch data
}
});
}
private CompletableFuture<String> loadAsyncData(String key) {
return CompletableFuture.supplyAsync(() -> {
// Simulate a data fetch
try {
Thread.sleep(100);
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
return "data for " + key;
});
}
}
Why Use Asynchronous Loading?
Asynchronous loading can improve user experience by allowing your application to remain responsive while data is being fetched, minimizing the time users wait for important information.
Closing the Chapter
Guava Cache can significantly enhance your Java application's performance. However, it is crucial to implement strategies to prevent cache overflow, which can lead to data loss and performance degradation. By setting size limits, employing expiration policies, using loader functions, monitoring usage, and considering asynchronous loading, you can ensure that your cache remains efficient and reliable.
For more detailed information about Guava Cache and its capabilities, you can refer to the Guava Documentation.
By carefully implementing these strategies, you can harness the full power of Guava Cache without the fear of overflowing and losing valuable data. Happy coding!