Overcoming Common Spring Test Framework Pitfalls
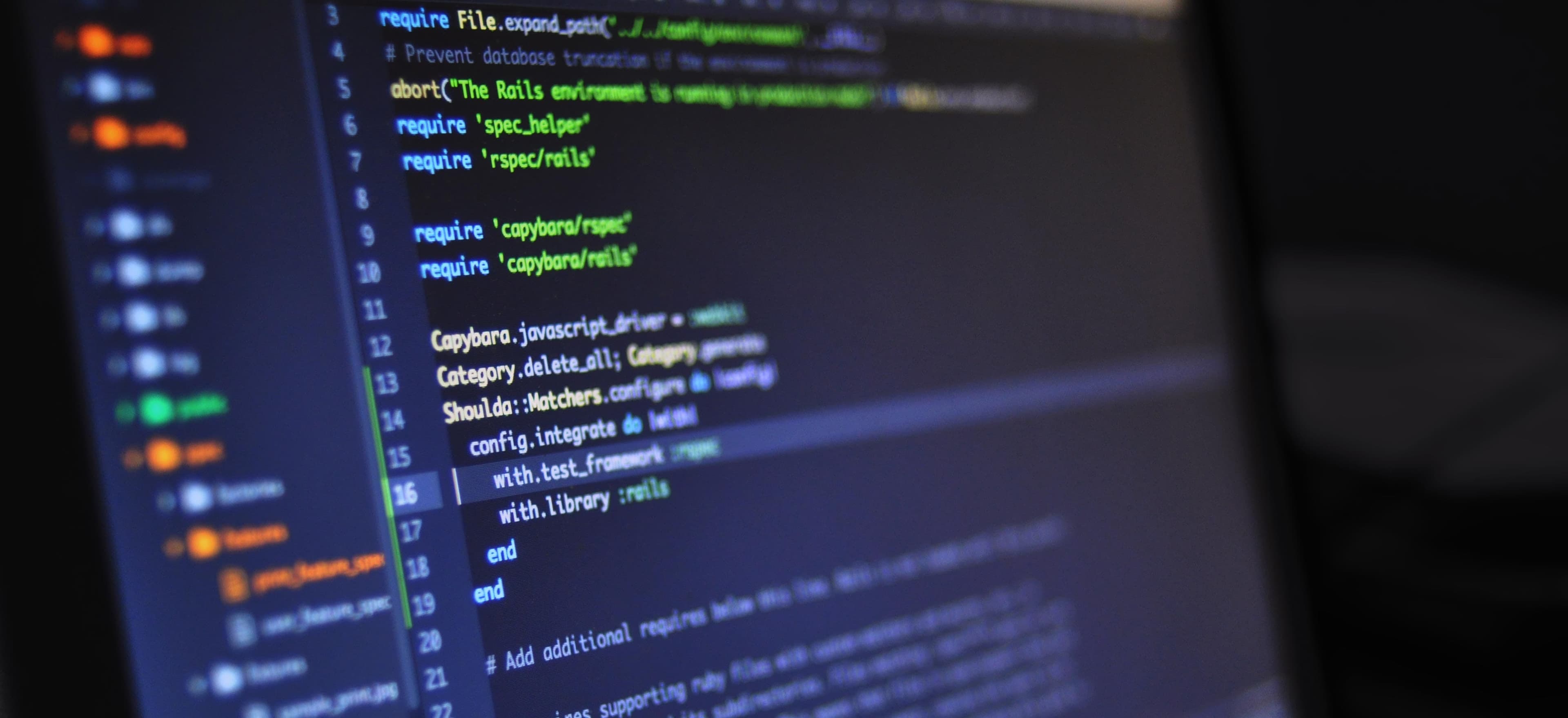
- Published on
Overcoming Common Spring Test Framework Pitfalls
Testing is an essential part of any software development process, especially when using complex frameworks such as Spring. The Spring Test Framework provides a robust environment for testing Spring components. However, developers often face several common pitfalls. In this blog post, we will discuss these pitfalls, how to avoid them, and provide practical code snippets to help you understand effectively how to implement tests using the Spring Test Framework.
Understanding the Spring Test Framework
Before we dive into common pitfalls, it's important to understand what the Spring Test Framework offers. With features like dependency injection, context loading, and configuration management, the framework simplifies testing by allowing for clean, understandable test code.
Key Features of the Spring Test Framework:
- Dependency Injection for Test Classes: This makes it easy to inject mocks and other dependencies.
- Transaction Management: Tests can be rolled back to maintain a clean state.
- Context Configuration: Load and configure the application context, which simulates an entire Spring environment.
Common Pitfalls in Spring Testing
1. Not Using Annotations Properly
One of the biggest drawbacks new Spring users experience is misusing testing annotations. The most crucial annotations are @RunWith
, @ContextConfiguration
, and @Autowired
.
Why It Matters
Using the wrong annotations can lead to issues with context loading, resulting in IllegalStateException
, or failed tests due to improper dependency management. This leads to confusion and wasted time.
Example Code Snippet
@RunWith(SpringJUnit4ClassRunner.class)
@ContextConfiguration(classes = { AppConfig.class })
public class UserServiceTest {
@Autowired
private UserService userService;
@Test
public void testFindUserById() {
User user = userService.findUserById(1);
assertNotNull(user); // Ensure user is retrieved
}
}
In this example, we utilize @RunWith
to specify the Spring Test Runner. @ContextConfiguration
ensures that the necessary Spring configurations are loaded, providing our test with the required application context.
2. Ignoring @MockBean
One of the most powerful features of Spring, particularly in tests, is the @MockBean
annotation from Spring Boot. Many developers forget to utilize this feature, opting instead for manual mock object creation or not mocking at all.
Why It Matters
Mocking dependencies allows you to isolate the class under test. This leads to more focused tests, improving test reliability and reducing side effects.
Example Code Snippet
@RunWith(SpringJUnit4ClassRunner.class)
@ContextConfiguration(classes = { UserService.class })
public class UserServiceTest {
@MockBean
private UserRepository userRepository;
@Autowired
private UserService userService;
@Test
public void testFindUserById() {
User user = new User(1, "John Doe");
when(userRepository.findById(1)).thenReturn(Optional.of(user));
User foundUser = userService.findUserById(1);
assertEquals("John Doe", foundUser.getName());
}
}
In this code snippet, we've used @MockBean
to mock the UserRepository
. This allows us to dictate the behavior of the repository while testing UserService
.
3. Not Cleaning Up After Tests
Another common pitfall is failing to clean up the database or state after tests. Without cleanup, tests can influence one another, causing flaky results—making it hard to determine if a test failure is due to the code or the state carried over from a previous test.
Why It Matters
Keeping tests isolated allows for repeatability and maintains test integrity. Failing to do so leads to missed bugs that could appear in production.
Example Code Snippet
@AfterEach
public void cleanup() {
userRepository.deleteAll(); // Clear the repository after each test
}
In this snippet, using the @AfterEach
annotation ensures that each test runs in a clean environment, making it less prone to side effects from prior tests.
4. Using Too Much Configuration
The Spring framework is incredibly powerful, but it can also become complex with excessive configuration. Over-configuration of the test context can cause tests to run slower than necessary, leading to developer frustration.
Why It Matters
Keeping your configurations simple leads to faster execution and clearer tests, making it easier to identify where problems are occurring.
Example Code Snippet
@TestConfiguration
static class TestConfig {
@Bean
public UserService userService() {
return new UserService();
}
}
@RunWith(SpringJUnit4ClassRunner.class)
@ContextConfiguration(classes = { TestConfig.class })
public class UserServiceTest {
// Tests here
}
In this example, we’ve encapsulated configuration in a static inner class, isolating it from production code. This practice makes it easier to manage the test context while maintaining simplicity.
5. Failing to Utilize Test Slices
Spring Boot provides various test slices, such as @WebMvcTest
, @DataJpaTest
, etc., that load specific parts of your application context without the overhead of other parts. Not using these slices can lead to slower, more complex tests.
Why It Matters
Using test slices cuts down on context loading time and improves test clarity. You only load what's necessary for the test, reducing complexity.
Example Code Snippet
@WebMvcTest(UserController.class)
public class UserControllerTest {
@Autowired
private MockMvc mockMvc;
@MockBean
private UserService userService;
@Test
public void testGetUser() throws Exception {
when(userService.findUserById(1)).thenReturn(new User(1, "John Doe"));
mockMvc.perform(get("/users/1"))
.andExpect(status().isOk())
.andExpect(jsonPath("$.name").value("John Doe"));
}
}
In this snippet, we utilize @WebMvcTest
to test only the UserController
. It isolates the controller and allows us to mock the service without setting up the entire application context.
To Wrap Things Up
Testing in Spring can be efficient and straightforward, but common pitfalls can hinder development. Avoiding misused annotations, neglecting mocking, failing to clean up, over-configuration, and not utilizing test slices significantly contributes to a smoother, more productive testing process.
For more detailed information on effective techniques with Spring Testing, visit Spring Testing Documentation or check out Spring Boot Testing Documentation.
By understanding and overcoming these common pitfalls, you can maintain high standards in your testing practices, ensuring better software quality and reliability. Keep testing, keep coding!