Struggling with Apache CXF and CDI? Here's a Quick Fix!
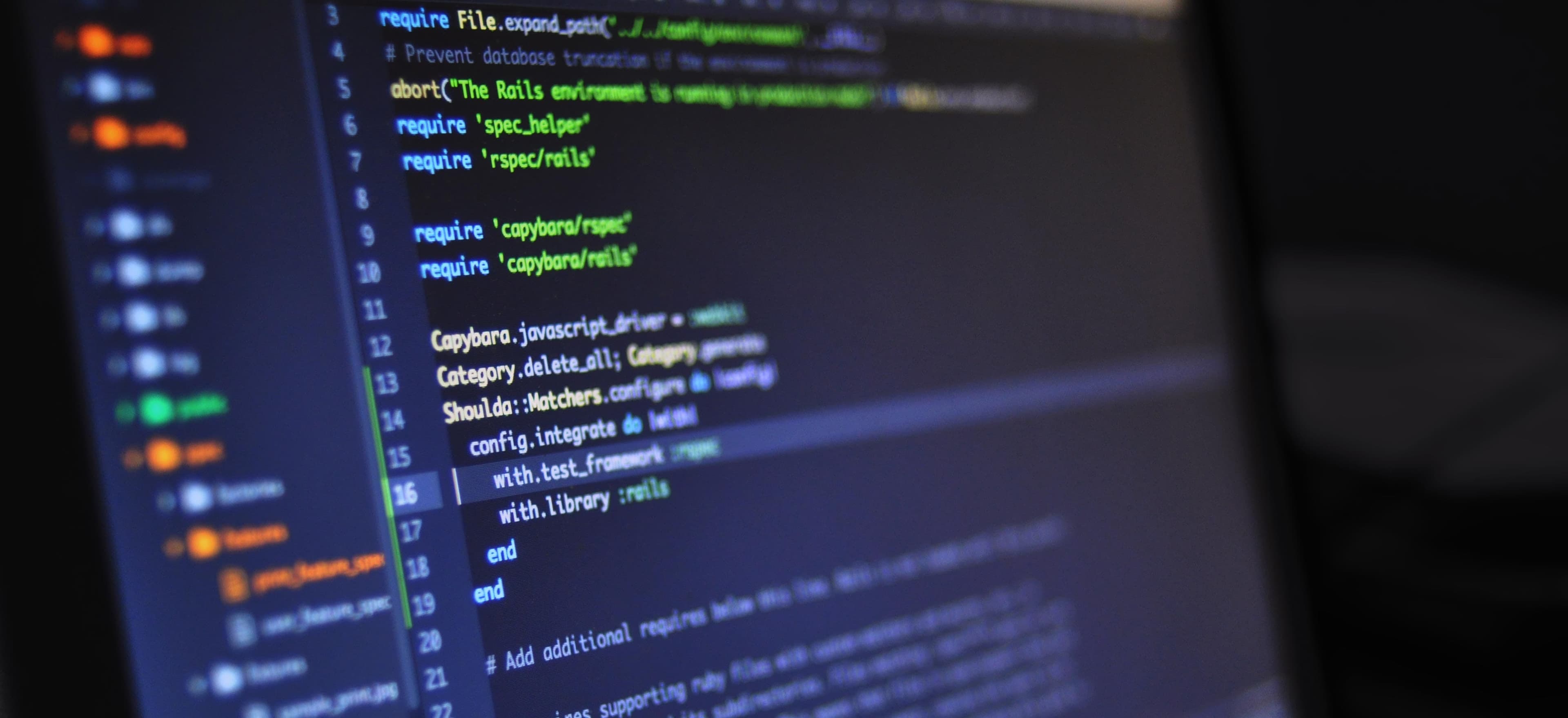
- Published on
Struggling with Apache CXF and CDI? Here's a Quick Fix!
When it comes to building web services in Java, Apache CXF stands out as a robust framework. Its support for various protocols, rich feature set, and integration capabilities make it a popular choice. However, when combining it with Contexts and Dependency Injection (CDI), developers often encounter unexpected challenges. If you're struggling with this integration, you're not alone! But fear not—this blog post will guide you through quick fixes to common issues and help you get back on track.
What is Apache CXF?
Apache CXF is an open-source framework that simplifies the development of web services. It supports both SOAP and REST web services and provides tools to handle security, logging, and transactions. This versatility makes it a go-to framework for many Java developers.
What is CDI?
Contexts and Dependency Injection (CDI) is part of the Java EE specification. It allows developers to manage the lifecycle of beans and perform dependency injection seamlessly. CDI promotes a cleaner architecture and encourages the use of loosely coupled components.
Why Use Apache CXF with CDI?
Integrating Apache CXF with CDI can leverage both frameworks' strengths. You benefit from the powerful routing and protocol handling of CXF, combined with CDI’s capability to simplify bean management and enhance modularity. This integration can lead to cleaner, more maintainable code.
Common Issues with CXF and CDI
While the integration promises many advantages, it can lead to complexities. Below are common challenges developers face when using Apache CXF with CDI, along with quick solutions.
1. Missing CDI Beans in CXF Endpoints
One of the most frequent issues arises when trying to inject CDI beans into your CXF service classes. If the service class is not properly recognized as a CDI bean, you will encounter issues like javax.enterprise.inject.spi.CDIException
.
Solution: Ensure that your service class is annotated with @Stateless
, @Singleton
, or @Dependent
, which allows the CDI container to manage its lifecycle.
import javax.ejb.Stateless;
import javax.inject.Inject;
@Stateless
public class MyService {
@Inject
private MyDependency myDependency;
public String sayHello() {
return myDependency.getGreeting();
}
}
In this snippet, MyService
is a CDI-managed bean. By injecting MyDependency
, you can easily use its methods in sayHello()
. Make sure to deploy your application correctly so that your beans are scanned effectively.
2. Configuration Issues Related to the CDI Extension
When working with Apache CXF and CDI, you need to make sure that the CXF CDI extension is correctly configured. If not set up properly, your beans will not work within the CXF environment.
Solution: Add the CXF CDI dependency in your pom.xml
.
<dependency>
<groupId>org.apache.cxf</groupId>
<artifactId>cxf-rt-frontend-jaxws</artifactId>
<version>${cxf.version}</version>
</dependency>
<dependency>
<groupId>org.apache.cxf</groupId>
<artifactId>cxf-rt-frontend-jaxrs</artifactId>
<version>${cxf.version}</version>
</dependency>
<dependency>
<groupId>org.apache.cxf</groupId>
<artifactId>cxf-rt-ws-security</artifactId>
<version>${cxf.version}</version>
</dependency>
<dependency>
<groupId>org.apache.cxf</groupId>
<artifactId>cxf-rt-transports-http</artifactId>
<version>${cxf.version}</version>
</dependency>
Incorporate these dependencies in your project to avoid ClassNotFound
exceptions related to CXF components.
3. Qualifier Conflicts
Sometimes, when you have multiple implementations of a CDI bean, you might face issues regarding ambiguous dependencies.
Solution: Use @Qualifier
annotations to specify which implementation you want to inject.
import javax.inject.Qualifier;
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
import java.lang.annotation.Target;
import static java.lang.annotation.ElementType.*;
@Qualifier
@Retention(RetentionPolicy.RUNTIME)
@Target({METHOD, FIELD, PARAMETER, TYPE})
public @interface CustomQualifier {
}
When applying this qualifier in your beans:
@CustomQualifier
public class MySpecialDependency implements MyDependency {
...
}
You can then use this qualifier in your injection point to resolve ambiguity.
4. Application Context Configuration
If your services are not able to communicate correctly, it may be due to misconfiguration in application context files.
Solution: Ensure that your beans.xml
file is set up correctly. A minimal beans.xml
looks like this:
<beans xmlns="http://xmlns.jcp.org/xml/ns/javaee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee
http://xmlns.jcp.org/xml/ns/javaee/beans_1_1.xsd"
version="1.1">
</beans>
Placing this file in the META-INF
directory ensures CDI will initialize correctly.
5. Logging and Error Handling
Debugging issues with CFX and CDI can sometimes be challenging due to insufficient logging.
Solution: Increase the logging level for your CXF services in your application server or add logging handlers in your beans. Below is an example of how to integrate SLF4J with your service:
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
@Stateless
public class MyService {
private static final Logger logger = LoggerFactory.getLogger(MyService.class);
public void process() {
logger.info("Processing request...");
// your processing logic
logger.info("Processing complete.");
}
}
Using structured logging will help you track down issues more effectively.
Closing Remarks
Apache CXF and CDI integration improves the way we design and implement web services in Java. While obstacles exist, knowing how to overcome them can save you time and frustration. By following the recommendations and code snippets in this post, you will be well on your way to successfully using Apache CXF with CDI.
Additional Resources
For further information, consider exploring the following resources:
- Apache CXF Documentation
- Java EE CDI Specification
- Building a RESTful Web Service with JAX-RS and CDI
By taking these steps, you should be poised to handle the challenges associated with Apache CXF and CDI integration. Happy coding!