Common Mistakes in Java Code: How to Avoid Them
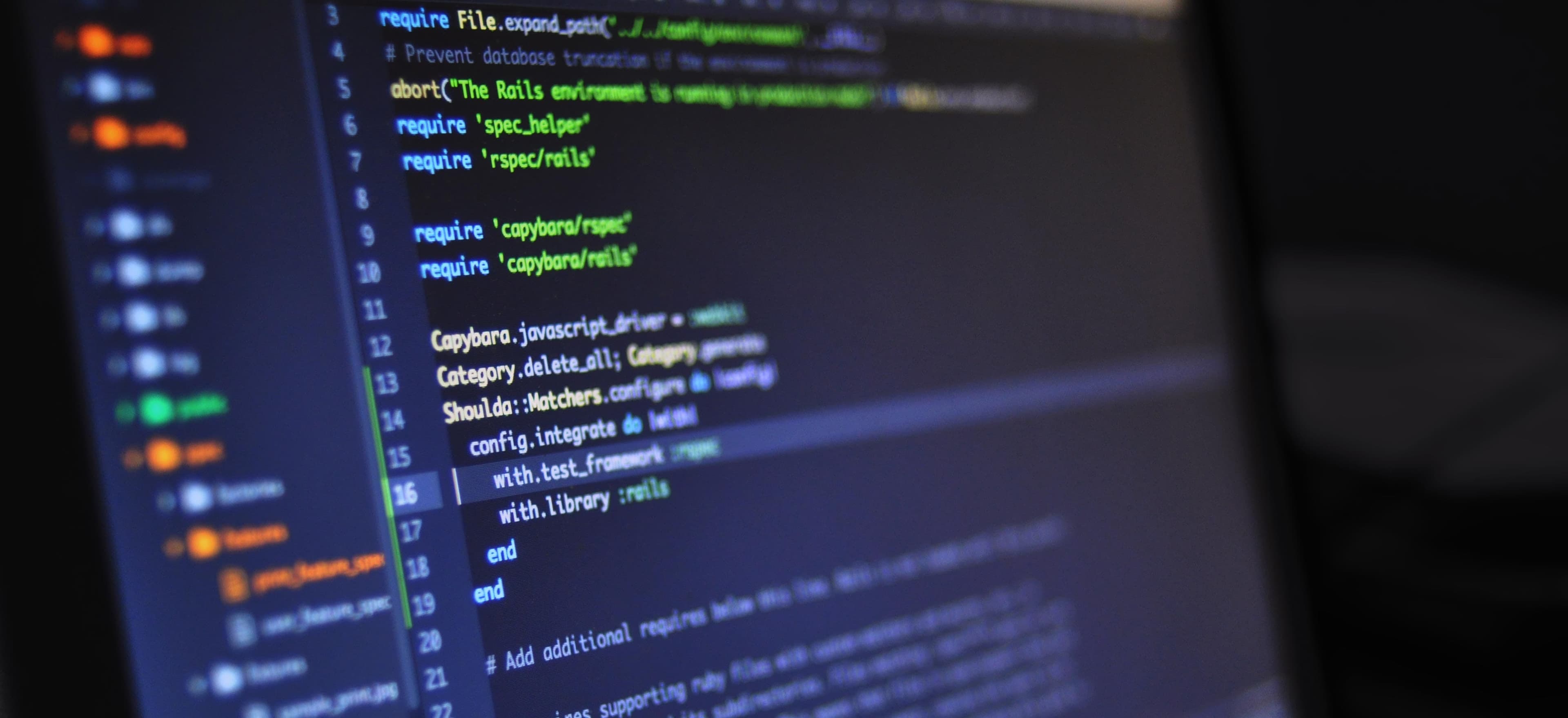
- Published on
Common Mistakes in Java Code: How to Avoid Them
Java is a powerful programming language, widely used for building applications across diverse domains. While its robust syntax and object-oriented principles empower developers to create scalable solutions, common mistakes still lurk in the shadows. This blog post explores frequent pitfalls in Java coding, providing you with actionable insights on how to avoid them, ensuring more efficient and error-free programming.
Table of Contents
- Overlooking NullPointerExceptions
- Improper Use of Collections
- Ignoring Exception Handling
- Mismanaging Memory with Static Variables
- Neglecting Access Modifiers
- Failing to Follow Naming Conventions
- Incorrectly Implementing Interfaces
- Conclusion
Overlooking NullPointerExceptions
One of the most notorious issues in Java is the NullPointerException
. It occurs when a program attempts to use an object reference that has not been initialized.
Example:
public class Sample {
private String name;
public void printName() {
System.out.println(name.length()); // Potential NullPointerException.
}
}
In this example, calling printName()
will throw NullPointerException
because name
is never initialized.
How to Avoid:
- Initialize Variables: Always initialize your variables.
public class Sample {
private String name = ""; // Initialize with an empty string
public void printName() {
System.out.println(name.length());
}
}
- Use Optional: Java 8 introduced
Optional
to avoid null checks.
import java.util.Optional;
public class Sample {
private Optional<String> name = Optional.empty();
public void printName() {
System.out.println(name.map(String::length).orElse(0));
}
}
Improper Use of Collections
Collections are a core part of Java. Misusing them can lead to performance issues or unexpected behavior.
Example:
List<String> list = new ArrayList<>();
list.add("A");
list.add("B");
System.out.println(list.get(2)); // IndexOutOfBoundsException
In this case, trying to access an index that does not exist will throw an IndexOutOfBoundsException
.
How to Avoid:
- Check Size Before Access: Always check the size of the collection.
if (index < list.size()) {
System.out.println(list.get(index));
}
- Use Appropriate Collection Types: Choose the right type based on your need (e.g.,
ArrayList
vsLinkedList
).
Ignoring Exception Handling
Exception handling is a fundamental aspect of robust applications. Neglecting it can lead to application crashes and poor user experiences.
Example:
public void readFile(File file) {
FileReader reader = new FileReader(file);
// No exception handling here
}
This code does not handle potential FileNotFoundException
, leading to crashes.
How to Avoid:
- Use Try-Catch Blocks: Always wrap code that could throw exceptions in try-catch blocks.
public void readFile(File file) {
try {
FileReader reader = new FileReader(file);
} catch (FileNotFoundException e) {
e.printStackTrace(); // Log the exception
}
}
- Throw Specific Exceptions: Be clear about what your method can throw, allowing callers to handle exceptions accordingly.
Mismanaging Memory with Static Variables
Static variables persist for the lifetime of the application. Mismanagement can lead to memory leaks or unintentional data retention.
Example:
public class Configuration {
public static String configValue;
public void setConfigValue(String value) {
configValue = value; // Potentially retain old data
}
}
Here, configValue
retains its data even after the instance of Configuration
is no longer needed.
How to Avoid:
-
Limit Static Usage: Use static variables judiciously.
-
Encapsulate State: Consider using instance variables instead.
public class Configuration {
private String configValue;
public void setConfigValue(String value) {
this.configValue = value; // Instance specific
}
}
Neglecting Access Modifiers
Access modifiers are crucial for encapsulating your classes and their members. Neglecting them can expose your code to unintended misuse.
Example:
public class User {
public String userName; // Too permissive
// Method without access control
void displayInfo() {
System.out.println(userName);
}
}
This code exposes userName
to modifications from outside the class.
How to Avoid:
- Use private Variables: Limit access to class members.
public class User {
private String userName; // Encapsulated
public void setUserName(String userName) {
this.userName = userName; // Controlled access
}
public void displayInfo() {
System.out.println(userName);
}
}
Failing to Follow Naming Conventions
Naming conventions play a significant role in code readability and maintainability. Skipping them leads to confusion.
Example:
public class UsrInfo {
private String nme; // Not clear
public void updtNme(String newName) {
this.nme = newName; // Ambiguity increases
}
}
Small mistakes like this can lead to misunderstandings among team members.
How to Avoid:
- Stick to Conventions: Use clear, relevant names that follow Java conventions (camelCase for variables and methods, PascalCase for classes).
public class UserInfo {
private String name; // Clear and descriptive
public void updateName(String newName) {
this.name = newName; // Easy to understand
}
}
Incorrectly Implementing Interfaces
Interfaces are foundational for achieving polymorphism in Java. Improper implementation can lead to runtime errors and unpredictable behavior.
Example:
public interface Animal {
void makeSound();
}
public class Dog implements Animal {
public void bark() { // Incorrect method signature
System.out.println("Woof");
}
}
This implementation misses the makeSound
method declaration.
How to Avoid:
- Implement All Interface Methods: Ensure you implement all methods defined in an interface.
public class Dog implements Animal {
public void makeSound() {
System.out.println("Woof");
}
}
- Use IDE Features: Most IDEs can automatically implement interface methods for you.
In Conclusion, Here is What Matters
Avoiding common mistakes in Java requires diligence and mindfulness. By paying attention to details and using the best practices outlined in this post, you can create cleaner, more maintainable code. Whether you’re building a simple application or working on a complex project, always remember the importance of code quality.
For further reading and in-depth understanding, explore resources such as Java Documentation and books like "Effective Java". Keep learning, coding, and producing exceptional Java applications!