Common Pitfalls When Deploying Java Web Applications
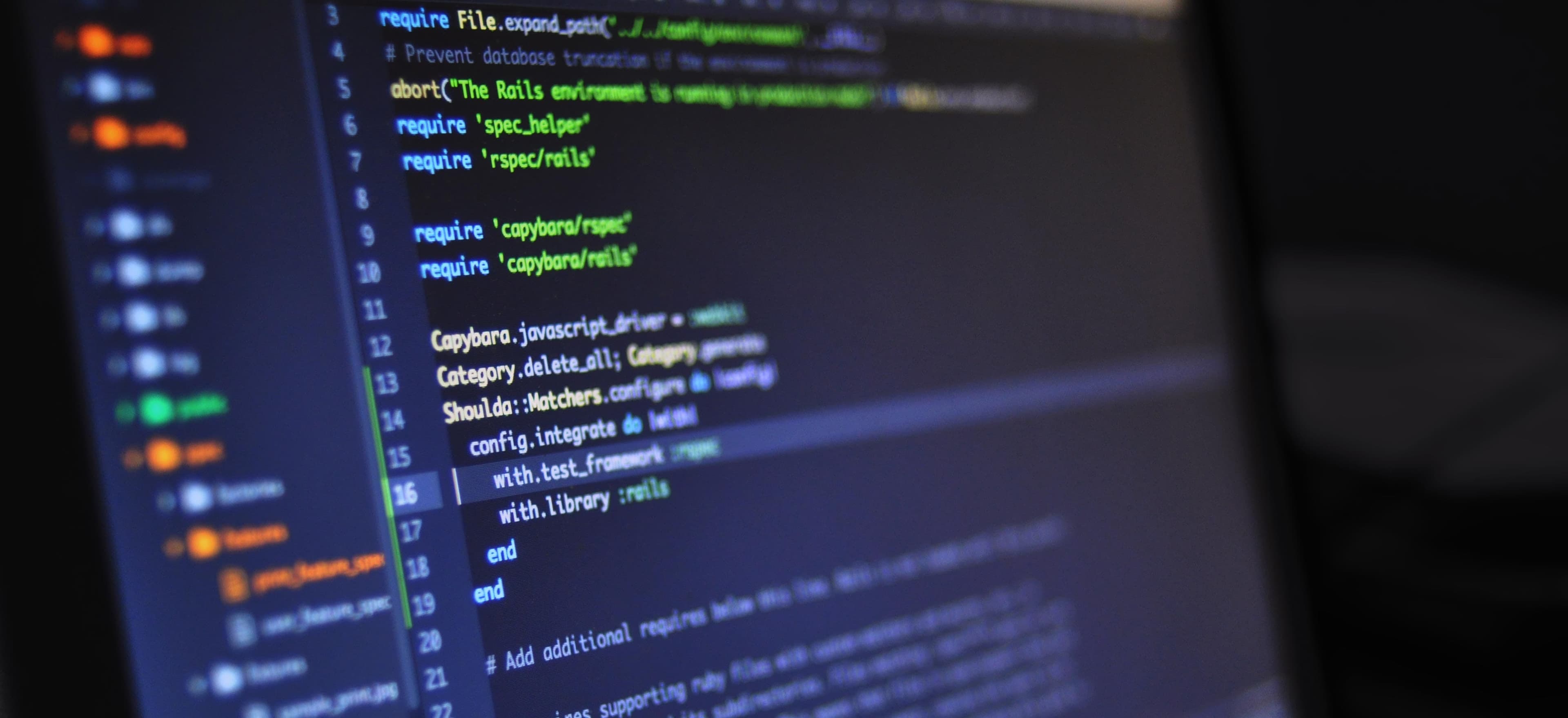
- Published on
Common Pitfalls When Deploying Java Web Applications
Deploying Java web applications can be a rewarding but challenging process. Java is a robust programming language known for its portability and scalability. However, several common pitfalls can occur during the deployment process, which may lead to performance issues, security vulnerabilities, and downtime. In this blog post, we will explore these pitfalls in detail while providing code snippets and best practices to help avoid them.
Table of Contents
- Configuration Errors
- Poor Resource Management
- Inadequate Security Measures
- Insufficient Testing
- Ignoring Logging and Monitoring
Configuration Errors
What Are Configuration Errors?
Configuration errors occur when the application settings are improperly set during deployment. These settings may involve file paths, database connection strings, environmental variables, and application properties.
Example of a Configuration Mistake
public class DatabaseConnection {
private String url = "jdbc:mysql://localhost:3306/mydb";
private String username = "user";
private String password = "pass";
public Connection connect() throws SQLException {
return DriverManager.getConnection(url, username, password);
}
}
In the above example, hardcoding sensitive information such as usernames and passwords can be a major pitfall. Instead, consider storing configuration data in environment variables or using external properties files:
# application.properties
db.url=jdbc:mysql://localhost:3306/mydb
db.username=${DB_USER}
db.password=${DB_PASS}
Why This Matters: Hardcoding sensitive information exposes your application to potential security breaches. Using external configuration files or environment variables prevents this.
Best Practices
- Use Version Control: Keep your configuration files under version control to track changes.
- Utilize Environment Variables: Store sensitive information in environment variables or use secrets management tools like HashiCorp Vault.
- Automate Configuration: Use automated tools like Ansible or Terraform for consistent and error-free deployment configurations.
Poor Resource Management
Understanding Resource Management
Failure to manage application resources effectively can lead to memory leaks, unnecessary CPU usage, and degraded performance. Java applications require appropriate handling of resources such as database connections, file I/O, and network sockets.
Connection Pooling Example
Using connection pools can enhance resource management in Java applications. Here is a simple implementation using HikariCP:
import com.zaxxer.hikari.HikariConfig;
import com.zaxxer.hikari.HikariDataSource;
public class DataSource {
private static HikariDataSource dataSource;
static {
HikariConfig config = new HikariConfig();
config.setJdbcUrl("jdbc:mysql://localhost:3306/mydb");
config.setUsername("user");
config.setPassword("pass");
config.setMaximumPoolSize(10); // Configurable max pool size
dataSource = new HikariDataSource(config);
}
public static Connection getConnection() throws SQLException {
return dataSource.getConnection();
}
}
Why This Matters: Implementing connection pooling reduces the overhead of continuously opening and closing database connections, thereby improving application performance.
Best Practices
- Optimize Garbage Collection: Use JVM flags to tune garbage collection based on your application's memory usage pattern.
- Implement Connection Pooling: Use connection pooling libraries like HikariCP or Apache DBCP to manage database connections efficiently.
- Profile Resource Usage: Use profiling tools like VisualVM or JProfiler to monitor your application's resource utilization.
Inadequate Security Measures
Recognizing Security Risks
Without proper security measures, your Java application may become vulnerable to various attacks, including SQL injection, XSS, and CSRF.
Example of Input Validation
Using input validation can help alleviate SQL injection risks. The following example uses Prepared Statements to prevent such attacks:
public void fetchUserData(String userId) {
String sql = "SELECT * FROM users WHERE id = ?";
try (Connection connection = DataSource.getConnection();
PreparedStatement statement = connection.prepareStatement(sql)) {
statement.setString(1, userId); // Prevents SQL injection
ResultSet rs = statement.executeQuery();
while (rs.next()) {
// Process result
}
} catch (SQLException e) {
e.printStackTrace();
}
}
Why This Matters: By using Prepared Statements, you mitigate SQL injection risks posed by user input, thus improving the application's overall security.
Best Practices
- Implement Security Frameworks: Incorporate security frameworks like Spring Security to handle authentication and authorization.
- Validate User Input: Always validate and sanitize user inputs to protect against malicious attacks.
- Keep Dependencies Updated: Regularly update third-party libraries and frameworks to patch any known security vulnerabilities.
Insufficient Testing
Importance of Comprehensive Testing
Insufficient or inadequate testing can lead to unresolved bugs, negatively affecting the user experience and application performance.
Implementing Unit Tests
Implementing unit tests should be part of your deployment process. Here’s a simple example using JUnit:
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.assertEquals;
public class MathUtilsTest {
@Test
public void testAdd() {
MathUtils utils = new MathUtils();
assertEquals(5, utils.add(2, 3), "Adding 2 + 3 should equal 5");
}
}
Why This Matters: Unit tests ensure that your code functions as expected, catching bugs early in the development process. They also improve the maintainability of your codebase.
Best Practices
- Conduct Automated Tests: Use tools like JUnit or TestNG to write and run automated tests.
- Perform Load Testing: Employ load testing tools like Apache JMeter to simulate multiple users and identify performance bottlenecks.
- Test in Production-like Environments: Always have a staging environment that mirrors your production setup to conduct comprehensive tests.
Ignoring Logging and Monitoring
Importance of Logging and Monitoring
Failing to implement logging and monitoring can make it exceptionally difficult to diagnose issues when they arise, leading to prolonged downtime and user frustration.
Example of Logging with SLF4J
Using a logging framework like SLF4J is essential for understanding application behavior:
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class UserService {
private static final Logger logger = LoggerFactory.getLogger(UserService.class);
public void findUser(String userId) {
logger.info("Fetching user with ID: {}", userId);
// Fetch user logic...
}
}
Why This Matters: Logging provides insight into your application's operations, allowing you to track issues and performance over time.
Best Practices
- Use Centralized Logging: Implement centralized logging using tools like ELK Stack (Elasticsearch, Logstash, Kibana) or Splunk.
- Enable Application Monitoring: Utilize application performance monitoring (APM) tools like New Relic or Dynatrace to keep an eye on application performance and user interactions.
- Set Up Alerts: Configure alerts for critical errors or performance metrics to respond promptly to issues.
To Wrap Things Up
Deploying Java web applications requires careful planning and execution. By avoiding common pitfalls such as configuration errors, poor resource management, inadequate security measures, insufficient testing, and ignoring logging and monitoring, you can ensure a smoother deployment process and create a more stable and secure application.
For additional insights and resources, consider checking out the following links:
- Spring Boot Documentation
- Effective Java by Joshua Bloch
- Java Performance Tuning
By incorporating these practices into your deployment strategy, you will enhance the reliability and effectiveness of your Java applications. Happy coding!